How Can You Import Another Python File in Your Project?
In the world of Python programming, efficiency and organization are paramount. As projects grow in complexity, the need to manage multiple files and modules becomes increasingly important. One of the most fundamental skills every Python developer must master is the ability to import another Python file. This seemingly simple task opens the door to modular programming, allowing you to reuse code, maintain clean structures, and collaborate seamlessly with others. Whether you’re building a small script or a large application, understanding how to effectively import and utilize external Python files is a game-changer.
When you import another Python file, you are essentially bringing in the functions, classes, and variables defined in that file into your current workspace. This not only saves time by preventing code duplication but also enhances readability and maintainability. By structuring your code into separate files, you can focus on specific functionalities while keeping your main program uncluttered. Moreover, this practice encourages a systematic approach to coding, where each module can be tested and updated independently.
In this article, we will explore the various methods of importing Python files, from basic imports to more advanced techniques. We will also discuss best practices for organizing your code and the importance of namespaces. Whether you’re a novice just starting your programming journey or a seasoned developer looking to refine your skills,
Using the Import Statement
To import another Python file, you primarily use the `import` statement. This allows you to access functions, classes, and variables defined in other Python files (modules). The general syntax for importing is straightforward:
“`python
import module_name
“`
This method imports the entire module, and you can access its contents using the dot notation. For example, if you have a module named `my_module.py` with a function `my_function`, you can call it as follows:
“`python
import my_module
my_module.my_function()
“`
Importing Specific Elements
If you only need specific functions or classes from a module, you can import them directly. This can make your code cleaner and reduce the overhead of importing everything from the module. The syntax for importing specific elements is:
“`python
from module_name import specific_function
“`
For instance, if you only want to use `my_function` from `my_module.py`, you would write:
“`python
from my_module import my_function
my_function()
“`
Aliasing Modules and Functions
In some cases, you might want to use an alias for a module or function to shorten your code or avoid naming conflicts. This can be accomplished using the `as` keyword. Here’s how you can create an alias:
“`python
import module_name as alias_name
from module_name import specific_function as alias_function
“`
For example:
“`python
import my_module as mm
mm.my_function()
“`
Organizing Your Python Files
When working with multiple Python files, maintaining a structured organization is vital. Here are some common practices:
- Directory Structure: Keep related modules in the same folder.
- Naming Conventions: Use descriptive names for your files to reflect their content.
- Use `__init__.py`: This file can be used to mark a directory as a Python package, allowing for easier imports.
Using Relative Imports
In a larger project, you might want to import modules from the same package or subpackage. Relative imports allow you to do this easily. You can use a dot (`.`) to indicate the current package or use double dots (`..`) for the parent package. The syntax is as follows:
“`python
from . import sibling_module
from .. import parent_module
“`
Here’s a simple example of a directory structure and how to use relative imports:
“`
my_project/
│
├── __init__.py
├── main.py
└── utils/
├── __init__.py
└── helper.py
“`
In `main.py`, you can import `helper.py` as follows:
“`python
from utils import helper
“`
Common Import Errors
When importing modules, you may encounter several common errors. Below is a table summarizing these errors and their potential solutions.
Error | Possible Cause | Solution |
---|---|---|
ModuleNotFoundError | The module does not exist in the specified path. | Check the module name and its location. |
ImportError | A specific function or class cannot be found. | Verify that the function or class name is correct and exists in the module. |
Circular Import | Modules import each other, creating a loop. | Refactor the code to eliminate circular dependencies. |
By following these guidelines and understanding the import mechanisms, you can effectively manage and utilize multiple Python files within your projects.
Importing Python Files
Importing another Python file allows you to use functions, classes, and variables defined in that file. This modular approach enhances code organization and reuse. The methods to import files vary depending on their locations and the context of your project.
Basic Import Syntax
To import a Python file, use the `import` statement followed by the filename without the `.py` extension. For example, if you have a file named `module.py`, you can import it as follows:
“`python
import module
“`
After importing, you can access its contents using the dot notation:
“`python
module.function_name()
module.ClassName()
“`
Importing Specific Elements
If you only need specific functions or classes from a module, you can import them directly using the `from` keyword:
“`python
from module import function_name, ClassName
“`
This allows you to use the imported items without the module prefix:
“`python
function_name()
instance = ClassName()
“`
Importing with Aliases
You can create an alias for a module or function using the `as` keyword. This is particularly useful for modules with long names or when there are naming conflicts:
“`python
import module as m
from module import function_name as fn
“`
Usage becomes simpler:
“`python
m.function_name()
fn()
“`
Relative Imports
When working within a package, you may need to perform relative imports. Use a dot (`.`) to indicate the current package or two dots (`..`) for the parent package. For instance, to import a module from the same package:
“`python
from . import sibling_module
from .. import parent_module
“`
Handling Import Errors
When importing, you might encounter `ImportError`. This can occur due to:
- The module not being in the Python path.
- The file name being incorrect.
- Circular imports.
To troubleshoot:
- Verify the module’s existence and correct spelling.
- Check the directory structure.
- Refactor your code to avoid circular dependencies.
Using `__init__.py` in Packages
If your directory is structured as a package, include an `__init__.py` file to indicate that the directory should be treated as a package. This file can be empty or execute initialization code.
Example structure:
“`
my_package/
__init__.py
module1.py
module2.py
“`
You can then import like this:
“`python
from my_package import module1
“`
Importing Built-in Modules
Python provides a rich library of built-in modules. You can import them similarly to user-defined modules. For example:
“`python
import math
import os
“`
These modules can be utilized directly:
“`python
result = math.sqrt(16)
files = os.listdir(‘.’)
“`
Best Practices for Imports
- Organize imports at the top of the file.
- Use absolute imports when possible for clarity.
- Avoid wildcard imports (e.g., `from module import *`) to prevent namespace conflicts.
- Group imports into three categories: standard library, third-party libraries, and local application imports, separated by blank lines.
Following these practices leads to cleaner, more maintainable code.
Expert Insights on Importing Python Files
Dr. Emily Carter (Senior Software Engineer, Python Innovations Inc.). “To import another Python file, you can use the `import` statement followed by the name of the file without the `.py` extension. Ensure that the file is in the same directory or in the Python path to avoid import errors.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “Utilizing the `from` keyword allows for more granular imports, enabling you to import specific functions or classes from a file. This approach can enhance code readability and maintainability.”
Sarah Thompson (Educational Content Creator, LearnPythonOnline). “When organizing larger projects, consider using packages by placing your Python files in a directory with an `__init__.py` file. This allows you to structure your imports more effectively and avoid naming conflicts.”
Frequently Asked Questions (FAQs)
How do I import a Python file located in the same directory?
To import a Python file in the same directory, use the syntax `import filename` without the `.py` extension. This allows you to access the functions and classes defined in that file.
What if the Python file I want to import is in a different directory?
If the file is in a different directory, you can modify the `sys.path` list to include the directory path or use a relative import. For example, `from ..folder import filename` can be used if the structure allows for it.
Can I import specific functions or classes from another Python file?
Yes, you can import specific functions or classes using the syntax `from filename import function_name`. This allows you to directly use the imported function without prefixing it with the filename.
What is the difference between import and from…import?
Using `import filename` imports the entire module, requiring you to prefix its members with the module name. In contrast, `from filename import member` imports only the specified member, allowing direct access without the module prefix.
How can I import all functions from a Python file?
To import all functions from a Python file, use the syntax `from filename import *`. However, this practice is discouraged as it can lead to naming conflicts and makes code less readable.
What should I do if I encounter an ImportError?
If you encounter an ImportError, check that the file name is correct, ensure the file is in the Python path, and verify that there are no circular imports. Adjusting the Python path or correcting the import statement usually resolves the issue.
Importing another Python file is a fundamental aspect of modular programming that enhances code organization and reusability. Python provides several mechanisms to import code from one file into another, primarily through the use of the `import` statement. This allows developers to break down complex programs into smaller, manageable components, facilitating easier debugging and maintenance. The most common methods include importing entire modules, specific functions or classes, and utilizing relative imports within packages.
When importing a file, it is essential to understand the Python module search path, which determines where Python looks for the modules to import. By default, Python searches in the current directory, the standard library, and any directories listed in the `PYTHONPATH` environment variable. Additionally, developers can create packages by organizing related modules into directories with an `__init__.py` file, allowing for structured imports and better namespace management.
Another important consideration is the use of the `from … import …` syntax, which allows for importing specific elements from a module, thus avoiding namespace conflicts and improving code clarity. Furthermore, the `as` keyword can be utilized to create aliases for imported modules or functions, enhancing readability and convenience. Understanding these import techniques is crucial for effective Python programming and contributes to writing clean
Author Profile
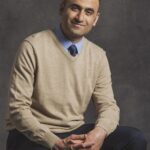
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?