How Can I Import Functions from Another JavaScript File?
Introduction
In the world of JavaScript development, modularity is key to creating efficient, maintainable, and scalable applications. As projects grow in complexity, the need to organize code into manageable chunks becomes increasingly important. One of the fundamental practices in achieving this modularity is the ability to import functions from one JavaScript file into another. Whether you’re building a simple web application or a robust enterprise solution, mastering this technique can significantly streamline your workflow and enhance collaboration among team members. In this article, we will explore the various methods of importing functions, the benefits of modular programming, and best practices to ensure your code remains clean and efficient.
When working with JavaScript, you often find yourself needing to share functionality across different files. This is where the concept of importing functions comes into play. By breaking your code into separate files, you can encapsulate related functionalities, making your codebase easier to navigate and maintain. This modular approach not only promotes reusability but also helps in avoiding code duplication, which can lead to errors and inconsistencies in larger projects.
There are several ways to import functions in JavaScript, each suited to different scenarios and coding styles. From the traditional CommonJS module system to the more modern ES6 modules, understanding these methods is essential for any developer looking
Using ES6 Modules
To import functions from another JavaScript file using ES6 modules, you need to use the `export` and `import` statements. This modern approach allows for cleaner and more maintainable code.
To export a function from a file, use the `export` keyword. For example:
javascript
// file: mathFunctions.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a – b;
}
In the above code, the functions `add` and `subtract` are exported from the `mathFunctions.js` file.
To import these functions in another JavaScript file, use the `import` statement:
javascript
// file: main.js
import { add, subtract } from ‘./mathFunctions.js’;
console.log(add(5, 3)); // Outputs: 8
console.log(subtract(5, 3)); // Outputs: 2
Ensure that the file paths are correctly specified, especially if the files are located in different directories.
Default Exports
In cases where you want to export a single function or variable as the default, you can use the `default` keyword. This allows for a simpler import syntax.
For example, in `mathFunctions.js`:
javascript
// file: mathFunctions.js
export default function multiply(a, b) {
return a * b;
}
To import a default export, the syntax changes slightly:
javascript
// file: main.js
import multiply from ‘./mathFunctions.js’;
console.log(multiply(5, 3)); // Outputs: 15
This method is beneficial when the module contains one primary functionality.
CommonJS Modules
For environments that do not support ES6 modules, such as older versions of Node.js, you can use the CommonJS module system. This method utilizes `module.exports` and `require()`.
In `mathFunctions.js`, you would define your functions like this:
javascript
// file: mathFunctions.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a – b;
}
module.exports = { add, subtract };
Then, in your main file, you would import the functions using `require()`:
javascript
// file: main.js
const mathFunctions = require(‘./mathFunctions.js’);
console.log(mathFunctions.add(5, 3)); // Outputs: 8
console.log(mathFunctions.subtract(5, 3)); // Outputs: 2
Comparison of Module Systems
The choice between ES6 modules and CommonJS modules often depends on the environment and specific project requirements. Below is a comparison of the two:
Feature | ES6 Modules | CommonJS Modules |
---|---|---|
Syntax | import/export | require/module.exports |
Loading | Static (compile-time) | Dynamic (run-time) |
Use in Browsers | Supported natively | Requires bundler |
Use in Node.js | Supported since v12 | Standard |
Understanding these differences can help you select the appropriate module system based on your project’s needs and compatibility requirements.
Using ES6 Modules
To import functions from another JavaScript file using ES6 modules, you need to follow a few straightforward steps. First, ensure that your JavaScript files are properly set up as modules.
- Exporting Functions: In the file where the function is defined, you need to export it using the `export` keyword. You can export functions in two ways:
- Named exports
- Default exports
Example of Named Export:
javascript
// file: mathFunctions.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a – b;
}
Example of Default Export:
javascript
// file: mathFunctions.js
export default function multiply(a, b) {
return a * b;
}
- Importing Functions: In the file where you want to use these functions, you will import them using the `import` statement.
Importing Named Exports:
javascript
// file: app.js
import { add, subtract } from ‘./mathFunctions.js’;
console.log(add(2, 3)); // Outputs: 5
console.log(subtract(5, 2)); // Outputs: 3
Importing Default Exports:
javascript
// file: app.js
import multiply from ‘./mathFunctions.js’;
console.log(multiply(2, 3)); // Outputs: 6
Using CommonJS Modules
For environments like Node.js, you can use CommonJS module syntax.
- Exporting Functions: Use `module.exports` to export functions from a file.
Example:
javascript
// file: mathFunctions.js
function add(a, b) {
return a + b;
}
function subtract(a, b) {
return a – b;
}
module.exports = { add, subtract };
- Importing Functions: Use `require()` to import the functions in another file.
Example:
javascript
// file: app.js
const { add, subtract } = require(‘./mathFunctions’);
console.log(add(5, 10)); // Outputs: 15
console.log(subtract(10, 5)); // Outputs: 5
Using Script Tags in HTML
When working in a browser environment without modules, you can also use script tags to include JavaScript files.
- Including Files: Use multiple script tags to include JavaScript files in your HTML.
Example:
- Global Functions: Ensure that the functions defined in `mathFunctions.js` are attached to the global `window` object, making them accessible in `app.js`.
Example:
javascript
// file: mathFunctions.js
function add(a, b) {
return a + b;
}
window.add = add; // Attach to global scope
Using TypeScript
If you are working with TypeScript, the import/export syntax is similar to ES6.
- Exporting Functions: Use `export` as in JavaScript.
Example:
typescript
// file: mathFunctions.ts
export function add(a: number, b: number): number {
return a + b;
}
- Importing Functions: Use the same import syntax.
Example:
typescript
// file: app.ts
import { add } from ‘./mathFunctions’;
console.log(add(2, 3)); // Outputs: 5
Expert Insights on Importing Functions in JavaScript
Jessica Lin (Senior JavaScript Developer, CodeCraft Solutions). “To import functions from another JavaScript file, you should utilize the ES6 module syntax. This involves exporting the functions using the `export` keyword in the source file and then importing them using the `import` statement in the target file. This approach enhances code organization and reusability.”
Michael Torres (Lead Software Engineer, DevMasters Inc.). “When working with CommonJS modules, which is common in Node.js environments, you can use `module.exports` to export functions and `require()` to import them. It’s crucial to understand the module system you are working with to avoid compatibility issues.”
Emily Carter (Frontend Architect, Web Innovators). “Utilizing dynamic imports can be particularly beneficial for performance optimization. By employing the `import()` function, you can load modules asynchronously, which helps in reducing the initial load time of your application.”
Frequently Asked Questions (FAQs)
How do I export functions from a JavaScript file?
To export functions from a JavaScript file, use the `export` keyword before the function declaration. For example, `export function myFunction() { … }` or `export default function myFunction() { … }` for default exports.
What is the difference between named and default exports?
Named exports allow you to export multiple functions or variables from a file, while default exports allow you to export a single function or variable as the primary export of that module. Named exports require curly braces when importing, whereas default exports do not.
How do I import a named function from another JavaScript file?
To import a named function, use the `import` statement with curly braces. For example, `import { myFunction } from ‘./myFile.js’;` imports the function `myFunction` from `myFile.js`.
Can I import multiple functions from the same file?
Yes, you can import multiple functions from the same file by listing them within the curly braces of the import statement. For example, `import { functionOne, functionTwo } from ‘./myFile.js’;` imports both `functionOne` and `functionTwo`.
What should I do if I encounter an error while importing functions?
Check for common issues such as incorrect file paths, missing file extensions, or mismatched export/import names. Ensure that the module is properly structured and that the functions are exported correctly.
Is it possible to import functions from a third-party library?
Yes, you can import functions from third-party libraries by installing the library via a package manager like npm or yarn and then using the `import` statement. For example, `import { libraryFunction } from ‘library-name’;` imports a specific function from the library.
In JavaScript, importing functions from another file is a fundamental practice that enhances code modularity and reusability. This process typically involves the use of the `import` statement, which allows developers to bring in specific functions, objects, or variables defined in one module into another. This modular approach not only streamlines the development process but also makes it easier to maintain and test code.
There are two primary module systems in JavaScript: CommonJS and ES Modules (ESM). CommonJS is often used in Node.js environments, utilizing the `require()` function to import modules. In contrast, ES Modules, which are now widely supported in modern browsers and JavaScript environments, employ the `import` and `export` syntax. Understanding the differences between these systems is crucial for developers working across various platforms and frameworks.
Key takeaways include the importance of properly exporting functions from the source file using the `export` keyword, whether as named exports or default exports. This ensures that the functions can be easily imported into other files. Additionally, organizing code into modules can significantly improve readability and reduce the likelihood of errors by encapsulating functionality within distinct files.
Ultimately, mastering the import and export mechanisms in JavaScript is essential
Author Profile
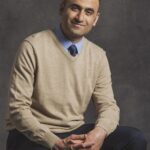
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?