How Can You Import a Python File from Another Directory?
In the world of Python programming, organizing your code efficiently is key to maintaining readability and functionality. As projects grow in complexity, it often becomes necessary to structure your files across multiple directories. However, this can lead to a common challenge: how to import a Python file from another directory. Whether you’re working on a personal project, collaborating with others, or developing a larger application, mastering this skill is essential for effective code management. In this article, we’ll explore the various methods to seamlessly import modules from different locations in your project, empowering you to enhance your Python coding experience.
When you find yourself needing to access functions, classes, or variables from a Python file located in a different directory, understanding the import system becomes crucial. Python’s import mechanism allows you to bring in external modules, but the way you do this can vary based on your project’s structure and the specific requirements of your code. From using relative imports to manipulating the Python path, there are several strategies you can employ to ensure smooth integration of your files.
Additionally, it’s important to consider the implications of your chosen method on code readability and maintainability. While some approaches may seem straightforward, they can introduce complexities that affect how others interact with your code. By the end of this article, you will
Understanding Python Modules and Packages
In Python, a module is simply a file containing Python code, while a package is a directory that contains a special `__init__.py` file along with other modules. When you want to import a Python file from another directory, it’s essential to understand how Python resolves module paths.
To import a module from a different directory, you can either modify the system path or use relative imports if the directories are part of a package.
Modifying the System Path
One common method to import a Python file from another directory is to modify the system path at runtime. This can be done using the `sys` module, which allows you to add the directory containing your target module to the Python path.
Example code snippet:
“`python
import sys
import os
Add the directory to the system path
sys.path.append(os.path.join(os.path.dirname(__file__), ‘path_to_directory’))
Now you can import the module
import my_module
“`
This method is straightforward, but it is often considered a temporary solution. It may lead to code that is difficult to maintain, especially in larger projects.
Using Absolute Imports
If your project structure is organized as a package, you can use absolute imports. An absolute import specifies the full path to the module from the project’s root.
Example directory structure:
“`
my_project/
main.py
package/
__init__.py
my_module.py
“`
You can import `my_module` in `main.py` as follows:
“`python
from package import my_module
“`
This method is cleaner and more maintainable, as it avoids modifying the system path.
Using Relative Imports
Relative imports allow you to import modules based on the current module’s position in the package hierarchy. This is particularly useful for large projects with complex structures.
Example of a relative import:
“`python
from .my_module import my_function
“`
In this case, the dot `.` refers to the current package. To use relative imports, ensure that your script is being executed as part of a package (e.g., using the `-m` flag).
Best Practices for Directory Structure
Maintaining a clear directory structure can greatly simplify the import process. Here are some best practices:
- Organize related modules into packages.
- Use `__init__.py` files to define package boundaries.
- Keep your project’s root directory clear of unnecessary files.
Example directory structure:
“`
my_project/
main.py
utils/
__init__.py
helper.py
models/
__init__.py
model.py
“`
This structure makes it easier to manage imports and avoid conflicts.
Common Issues and Solutions
Issue | Solution |
---|---|
ImportError: No module named | Check the module path and ensure it is correct. |
ImportError: cannot import name | Verify that the target function or class exists in the module. |
ModuleNotFoundError | Ensure the directory is added to `sys.path` or use absolute imports. |
By following these guidelines and strategies, you can effectively manage imports across different directories in your Python projects.
Using Python’s sys.path
To import a Python file from another directory, one common approach is to modify the `sys.path` list. This list contains the directories Python searches for modules. Here’s how to do it:
- Import the `sys` module.
- Append the target directory to `sys.path`.
- Import the desired module.
Example:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_module
“`
This method allows for dynamic imports without permanently altering the Python environment.
Utilizing the PYTHONPATH Environment Variable
Another method is to set the `PYTHONPATH` environment variable, which tells Python where to look for modules. This is particularly useful for larger projects.
- On Linux/MacOS:
“`bash
export PYTHONPATH=”/path/to/your/directory:$PYTHONPATH”
“`
- On Windows:
“`cmd
set PYTHONPATH=C:\path\to\your\directory;%PYTHONPATH%
“`
After setting `PYTHONPATH`, you can simply import the module as if it were in the current directory.
Creating a Package Structure
For more organized projects, consider creating a package structure. This involves the following steps:
- Create a directory for your package.
- Inside this directory, create an `__init__.py` file (can be empty).
- Place your module files in this directory.
For example:
“`
my_package/
__init__.py
my_module.py
“`
You can then import the module using:
“`python
from my_package import my_module
“`
This method ensures that your code is modular and maintainable.
Relative Imports
If you are working within a package, you can use relative imports. This allows you to import modules relative to the current module’s location.
- Use a dot (`.`) for the current package.
- Use two dots (`..`) to go up one package level.
Example:
“`python
from . import sibling_module Imports sibling_module in the same package
from ..parent_module import some_function Imports from a parent package
“`
Relative imports enhance readability and maintainability but should be used within packages only.
Using Importlib
For dynamic imports, `importlib` can be used. This is particularly useful for cases where you need to import modules at runtime based on certain conditions.
Example:
“`python
import importlib.util
import sys
module_name = “your_module”
module_path = “/path/to/your/directory/your_module.py”
spec = importlib.util.spec_from_file_location(module_name, module_path)
your_module = importlib.util.module_from_spec(spec)
sys.modules[module_name] = your_module
spec.loader.exec_module(your_module)
“`
This method offers flexibility and is suitable for plugins or modular applications.
Common Pitfalls
When importing files from another directory, be aware of these common issues:
- Incorrect Path: Ensure the path is correct; otherwise, Python will raise a `ModuleNotFoundError`.
- Circular Imports: This occurs when two modules try to import each other. Refactor the code to avoid circular dependencies.
- Namespace Conflicts: Avoid using the same names for modules in different directories to prevent confusion.
By understanding and applying these methods, you can effectively manage module imports across directories in Python.
Expert Insights on Importing Python Files from Different Directories
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When importing a Python file from another directory, it is essential to ensure that the target directory is included in the Python path. This can be achieved by modifying the `sys.path` list or by using relative imports if the files are part of a package structure.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Utilizing the `importlib` module can simplify the process of importing files from different directories. This approach allows for dynamic imports, making it easier to manage dependencies and maintain clean code structures across larger projects.”
Sarah Patel (Python Instructor, Data Science Academy). “For beginners, I recommend using absolute imports whenever possible. This not only helps in avoiding confusion but also enhances code readability. It’s crucial to maintain a well-organized directory structure to facilitate seamless imports.”
Frequently Asked Questions (FAQs)
How can I import a Python file from a different directory?
You can import a Python file from another directory by modifying the `sys.path` list to include the path of the directory containing the file. Use the following code:
“`python
import sys
sys.path.append(‘/path/to/your/directory’)
import your_file
“`
What is the purpose of using `sys.path`?
The `sys.path` list determines the directories Python searches for modules to import. By appending a directory to this list, you inform Python where to find the modules that are not in the default search paths.
Can I use relative imports to import a file from another directory?
Relative imports are only valid within packages. If your files are structured as a package, you can use relative imports with a dot notation, such as `from ..module import function`, to navigate to the desired module.
What is the role of the `__init__.py` file in importing?
The `__init__.py` file indicates that the directory should be treated as a package. This allows you to use relative imports and organize your modules effectively within that package.
Are there any potential issues when importing files from different directories?
Yes, potential issues include module name conflicts, circular imports, and difficulties in maintaining code readability. It is essential to manage imports carefully to avoid these complications.
What alternative methods exist for importing files from another directory?
Other than modifying `sys.path`, you can use the `PYTHONPATH` environment variable to include additional directories or utilize tools like `virtual environments` and `packages` to manage dependencies and imports more effectively.
In summary, importing a Python file from another directory can be accomplished through several methods, each suited to different scenarios. The most common approaches include modifying the system path, using relative imports, and utilizing the `importlib` module. By understanding the structure of your project and the location of your files, you can effectively manage imports across directories.
One of the simplest methods is to append the target directory to the `sys.path` list, allowing Python to recognize the location of the module you wish to import. Alternatively, if your project is structured as a package, relative imports can be employed to navigate between modules within the same package. For more dynamic import scenarios, the `importlib` module provides a flexible approach to load modules programmatically.
It is essential to consider the implications of each method on code readability and maintainability. While modifying `sys.path` can be straightforward, it may lead to complications in larger projects. On the other hand, using relative imports can enhance clarity but requires a well-defined package structure. Ultimately, the choice of method should align with the overall architecture of your application and the specific requirements of your project.
Author Profile
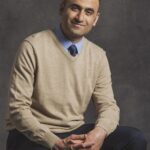
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?