How Can You Effectively Increment Values in JavaScript?
### Introduction
In the world of programming, the ability to manipulate numbers is fundamental, and JavaScript offers a variety of ways to increment values with ease. Whether you’re developing interactive web applications, performing calculations, or managing data, understanding how to increment in JavaScript is a crucial skill that can enhance your coding efficiency and effectiveness. This article will guide you through the various methods of incrementing numbers in JavaScript, helping you to grasp not only the syntax but also the best practices for implementing these techniques in your projects.
When we talk about incrementing in JavaScript, we’re referring to the process of increasing a numerical value, typically by one. This simple yet powerful operation can be performed using different techniques, each suited to different scenarios. From the straightforward increment operator to more complex functions, JavaScript provides a flexible toolkit for developers to manipulate numbers seamlessly.
As we delve deeper into the topic, you’ll discover the nuances of incrementing variables, the implications of using different operators, and how to avoid common pitfalls that can arise during implementation. With practical examples and clear explanations, you’ll be well-equipped to apply these concepts in your own coding endeavors, making your JavaScript experience more dynamic and robust.
Incrementing Values in JavaScript
In JavaScript, incrementing a value typically refers to increasing a number by a specific amount, commonly by one. This operation is fundamental in programming, especially in loops and counters. There are multiple ways to increment a number in JavaScript, each serving different purposes depending on the context.
Using the Increment Operator
The most straightforward method to increment a number is by using the increment operator `++`. This operator can be utilized in two forms: prefix and postfix.
- Prefix Increment (`++variable`): Increments the variable by one and then returns the variable.
- Postfix Increment (`variable++`): Returns the variable’s original value and then increments it by one.
Here’s a concise demonstration:
javascript
let a = 5;
let b = ++a; // a is now 6, b is 6
let c = a++; // a is now 7, c is 6
Using the Addition Assignment Operator
Another common method for incrementing values is the addition assignment operator `+=`. This operator allows you to add a specified value to a variable. For simple increments, you can add one as shown below:
javascript
let count = 0;
count += 1; // count is now 1
This approach is particularly useful when incrementing by values other than one, as you can easily modify the number:
javascript
count += 5; // count is now 6
Incrementing in Loops
Incrementing is frequently used in loops to control iterations. A `for` loop typically employs the increment operator to advance the loop counter.
javascript
for (let i = 0; i < 5; i++) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
}
In this example, the loop starts with `i` at 0 and increments `i` by 1 after each iteration until it reaches 5.
Comparison of Increment Methods
The choice between using the increment operator and the addition assignment operator can depend on readability and specific use cases. Below is a comparison table summarizing their features:
Method | Syntax | Returns | Use Case |
---|---|---|---|
Increment Operator | ++variable or variable++ | Variable after or before increment | Simple increments |
Addition Assignment | variable += value | New value after addition | Flexible increments |
Ultimately, understanding how to increment values in JavaScript is crucial for effective programming. By mastering these techniques, you can enhance your control over numerical data and optimize your code for various applications.
Basic Increment Operators
In JavaScript, incrementing a number can be achieved using the increment operator, which is represented by `++`. There are two forms of this operator: the prefix increment (`++variable`) and the postfix increment (`variable++`).
- Prefix Increment: Increments the variable’s value by one, then returns the new value.
- Postfix Increment: Returns the current value, then increments the variable’s value by one.
Example:
javascript
let a = 5;
let b = ++a; // b is 6, a is 6
let c = a++; // c is 6, a is 7
Using Increment in Loops
Increment operators are commonly used in loops to control iteration. Below are examples of using the increment operator in a `for` loop and a `while` loop.
For Loop Example:
javascript
for (let i = 0; i < 5; ++i) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
}
While Loop Example:
javascript
let i = 0;
while (i < 5) {
console.log(i); // Outputs: 0, 1, 2, 3, 4
i++;
}
Incrementing with Other Values
JavaScript allows for incrementing not only by one but also by any other value using the `+=` operator. This operator adds the specified value to the variable.
Example:
javascript
let num = 10;
num += 5; // num is now 15
Incrementing in Arrays
When working with arrays, you can increment elements directly by accessing their index.
Example:
javascript
let numbers = [1, 2, 3, 4];
for (let i = 0; i < numbers.length; i++) {
numbers[i]++; // Each element is incremented by 1
}
console.log(numbers); // Outputs: [2, 3, 4, 5]
Using Increment in Functions
Functions can also utilize incrementing to modify values passed as parameters.
Example:
javascript
function incrementValue(value) {
return value + 1;
}
let result = incrementValue(10); // result is 11
Incrementing with Objects
When dealing with objects, you can increment properties directly.
Example:
javascript
let obj = { count: 0 };
obj.count++; // obj.count is now 1
Common Pitfalls
While using increment operators, certain issues may arise:
- Using in Conditional Statements: Be cautious when using increment operators within conditions, as the order of evaluation might lead to unexpected results.
- Type Coercion: JavaScript is dynamically typed, so be aware that incrementing a non-integer type may lead to undesired type coercion.
Issue | Description |
---|---|
Increment in condition | Can lead to confusion regarding current value |
Type coercion | Non-integer types may not behave as expected |
Understanding the nuances of incrementing in JavaScript enhances your ability to manipulate numeric values efficiently in various programming contexts.
Expert Insights on Incrementing Values in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, the increment operator (++) is a straightforward and efficient way to increase the value of a variable by one. It’s essential to understand the difference between prefix and postfix forms of the operator, as they can affect the order of operations in your code.”
James Liu (JavaScript Developer Advocate, CodeCraft). “While the increment operator is commonly used, developers should also consider using the more explicit approach of adding one to a variable (e.g., variable += 1). This can enhance code readability, especially for those who may not be as familiar with JavaScript syntax.”
Sarah Thompson (Lead Frontend Developer, Web Solutions Group). “When incrementing values in loops, using a for loop with the increment operator can streamline the process. However, it’s crucial to ensure that your loop conditions are correctly set to avoid infinite loops, which can occur if the increment is not properly implemented.”
Frequently Asked Questions (FAQs)
What is the increment operator in JavaScript?
The increment operator in JavaScript is represented by `++`. It increases the value of a variable by one.
How do you use the increment operator?
You can use the increment operator in two forms: prefix (`++variable`) and postfix (`variable++`). The prefix form increases the value before the expression is evaluated, while the postfix form increases the value after the expression is evaluated.
Can you increment a variable that is not a number?
Yes, you can increment variables of other types, such as strings. However, JavaScript will attempt to convert the variable to a number before performing the increment, which may lead to unexpected results.
What happens if you increment a constant variable?
Attempting to increment a constant variable (declared with `const`) will result in a TypeError, as constants cannot be reassigned.
Is it possible to increment an array element in JavaScript?
Yes, you can increment an element of an array by accessing it via its index and applying the increment operator, such as `array[index]++`.
What are some common use cases for incrementing in JavaScript?
Common use cases include iterating through loops, updating counters, and managing state in applications where a numeric value needs to be increased.
In JavaScript, incrementing a value is a fundamental operation that can be achieved using various methods. The most common approach involves the use of the increment operator, represented by `++`. This operator can be utilized in two forms: the prefix increment (`++variable`) and the postfix increment (`variable++`). Both forms effectively increase the variable’s value by one, but they differ in their behavior regarding the value returned during the operation.
Additionally, developers can increment values using the addition assignment operator (`+=`). This operator allows for more flexibility, enabling the addition of any specified number to a variable. For example, `variable += 5` will increase the value of `variable` by five. This method is particularly useful when incrementing by values other than one, providing a straightforward way to adjust variable values in various contexts.
Understanding these incrementing techniques is essential for efficient programming in JavaScript. They not only simplify code but also enhance readability and maintainability. By mastering these operators, developers can write cleaner and more effective code, ensuring that their applications behave as intended while minimizing potential errors associated with manual incrementation.
Author Profile
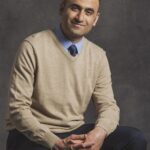
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?