How Do You Initialize a Dictionary in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow you to store data in key-value pairs, making it easy to access and manipulate information efficiently. Whether you’re managing user data, organizing configurations, or handling complex datasets, knowing how to initialize a dictionary is a fundamental skill that can elevate your coding prowess. As you dive into the intricacies of Python, mastering this essential concept will empower you to create more dynamic and responsive applications.
Initializing a dictionary in Python can be accomplished in several straightforward ways, each suited to different scenarios and preferences. From using curly braces to leveraging the `dict()` constructor, these methods provide flexibility in how you structure your data from the outset. Understanding the nuances of each technique not only enhances your coding efficiency but also allows you to choose the most appropriate method for your specific use case.
As you explore the various techniques for initializing dictionaries, you’ll discover how to create empty dictionaries, populate them with initial values, and even use comprehensions for more complex structures. This foundational knowledge will serve as a stepping stone for more advanced topics, such as nested dictionaries and dictionary methods, ultimately enriching your Python programming toolkit. Get ready to unlock the full potential of dictionaries and transform the way you handle data in your projects
Initializing a Dictionary Using Curly Braces
One of the most straightforward methods to initialize a dictionary in Python is by using curly braces `{}`. This method allows you to create a dictionary and populate it with key-value pairs in a single statement.
Example:
“`python
my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
“`
Using the dict() Constructor
Another way to create a dictionary is through the `dict()` constructor. This method is particularly useful when you want to create a dictionary from keyword arguments or from a sequence of key-value pairs.
Example:
“`python
my_dict = dict(key1=’value1′, key2=’value2′)
“`
You can also initialize a dictionary from a list of tuples:
“`python
my_dict = dict([(‘key1’, ‘value1’), (‘key2’, ‘value2’)])
“`
Creating an Empty Dictionary
If you need to create an empty dictionary to populate later, you can initialize it with either curly braces or the `dict()` constructor.
Example:
“`python
empty_dict_1 = {}
empty_dict_2 = dict()
“`
Both approaches yield an empty dictionary that can be populated with key-value pairs afterward.
Initializing a Dictionary with Default Values
You can also initialize a dictionary with default values using dictionary comprehensions or the `fromkeys()` method. This is particularly useful when you want all keys to have the same initial value.
Using `fromkeys()`:
“`python
keys = [‘key1’, ‘key2’, ‘key3’]
default_value = 0
my_dict = dict.fromkeys(keys, default_value)
“`
Using dictionary comprehension:
“`python
my_dict = {key: default_value for key in keys}
“`
Table of Initialization Methods
Method | Example | Notes |
---|---|---|
Curly braces | my_dict = {‘a’: 1, ‘b’: 2} | Simple and commonly used method. |
dict() constructor | my_dict = dict(a=1, b=2) | Useful for dynamic key-value creation. |
fromkeys() | my_dict = dict.fromkeys([‘a’, ‘b’], 0) | Creates keys with the same default value. |
Dictionary comprehension | my_dict = {key: 0 for key in [‘a’, ‘b’]} | Flexible and powerful for complex initializations. |
Populating a Dictionary After Initialization
Once a dictionary is initialized, you can easily add new key-value pairs or update existing ones. This can be done using the assignment operator.
Example:
“`python
my_dict[‘key3’] = ‘value3’ Adding a new key-value pair
my_dict[‘key1’] = ‘new_value’ Updating an existing value
“`
This flexibility allows for dynamic modifications and updates to the dictionary as needed throughout your program.
Initializing a Dictionary in Python
In Python, dictionaries are mutable, unordered collections of key-value pairs. Initializing a dictionary can be accomplished in several ways, each suited to different use cases. Below are the most common methods of dictionary initialization.
Using Curly Braces
The simplest way to create a dictionary is by using curly braces `{}`. This method allows you to define key-value pairs directly.
“`python
my_dict = {
‘key1’: ‘value1’,
‘key2’: ‘value2’,
‘key3’: ‘value3’
}
“`
Using the `dict()` Constructor
You can also use the built-in `dict()` constructor to create a dictionary. This method can take keyword arguments or an iterable of key-value pairs.
“`python
my_dict = dict(key1=’value1′, key2=’value2′, key3=’value3′)
“`
For initializing with an iterable:
“`python
my_dict = dict([(‘key1’, ‘value1’), (‘key2’, ‘value2’)])
“`
Using Dictionary Comprehensions
Dictionary comprehensions provide a concise way to create dictionaries. This method is especially useful for generating dictionaries dynamically.
“`python
my_dict = {x: x**2 for x in range(5)}
“`
This will create a dictionary where each key is a number and each value is its square.
Using Default Factory with `defaultdict`
When you want to initialize a dictionary with default values, the `collections.defaultdict` is particularly useful. It allows you to specify a default type for the dictionary.
“`python
from collections import defaultdict
my_dict = defaultdict(int) Default value will be 0 for non-existent keys
my_dict[‘key1’] += 1 Automatically initialized to 0, then incremented to 1
“`
Initializing an Empty Dictionary
To create an empty dictionary, you can use either method, depending on your preference:
“`python
empty_dict1 = {}
empty_dict2 = dict()
“`
Both `empty_dict1` and `empty_dict2` will be empty dictionaries.
Common Use Cases
Here are some scenarios where initializing dictionaries is commonly used:
- Storing Configuration Settings: Configuration values can be stored as key-value pairs for easy access.
- Counting Frequencies: You can use dictionaries to count occurrences of items in a list.
- Mapping Related Data: Dictionaries are ideal for mapping relationships, such as names to phone numbers.
Summary of Methods
The following table summarizes the different methods to initialize a dictionary in Python:
Method | Syntax Example | Description |
---|---|---|
Curly Braces | `my_dict = {‘key1’: ‘value1’}` | Directly define key-value pairs. |
`dict()` Constructor | `my_dict = dict(key1=’value1′)` | Create a dictionary using keyword arguments. |
Dictionary Comprehension | `my_dict = {x: x**2 for x in range(5)}` | Generate a dictionary dynamically. |
`defaultdict` | `my_dict = defaultdict(int)` | Initialize with a default value for non-existent keys. |
Empty Dictionary | `empty_dict = {}` or `empty_dict = dict()` | Create an empty dictionary. |
By choosing the appropriate method for initializing a dictionary, you can enhance code readability and functionality according to your specific requirements.
Expert Insights on Initializing Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To initialize a dictionary in Python, one can use the curly braces `{}` for an empty dictionary or use the `dict()` constructor. For example, `my_dict = {}` or `my_dict = dict()`. This flexibility allows developers to choose the method that best fits their coding style.”
James Thompson (Python Developer, Tech Innovations). “When initializing a dictionary, it is also possible to prepopulate it with key-value pairs. For instance, `my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}` is a common practice that saves time when the initial data is known.”
Linda Zhang (Data Scientist, Analytics Pro). “Utilizing dictionary comprehensions is an efficient way to initialize dictionaries in Python. For example, `my_dict = {x: x**2 for x in range(5)}` creates a dictionary with squares of numbers from 0 to 4, demonstrating the power of Python’s expressive syntax.”
Frequently Asked Questions (FAQs)
How do I initialize an empty dictionary in Python?
You can initialize an empty dictionary in Python using either curly braces `{}` or the `dict()` constructor. For example, `my_dict = {}` or `my_dict = dict()`.
How can I initialize a dictionary with default values?
To initialize a dictionary with default values, you can use dictionary comprehension or the `fromkeys()` method. For instance, `my_dict = dict.fromkeys([‘key1’, ‘key2’], ‘default_value’)` creates a dictionary with specified keys and a default value.
What is the syntax for initializing a dictionary with key-value pairs?
You can initialize a dictionary with key-value pairs by using curly braces with colon-separated key-value pairs. For example, `my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2’}`.
Can I initialize a dictionary using a list of tuples?
Yes, you can initialize a dictionary using a list of tuples by passing it to the `dict()` constructor. For example, `my_dict = dict([(‘key1’, ‘value1’), (‘key2’, ‘value2’)])`.
Is it possible to initialize a dictionary with different data types for keys and values?
Yes, Python dictionaries can have keys and values of different data types. For instance, `my_dict = {1: ‘one’, ‘two’: 2, (3, 4): [5, 6]}` is a valid dictionary with mixed types.
How do I initialize a nested dictionary in Python?
To initialize a nested dictionary, you can define a dictionary within another dictionary. For example, `my_dict = {‘outer_key’: {‘inner_key’: ‘inner_value’}}` creates a nested dictionary structure.
In Python, initializing a dictionary can be accomplished in several straightforward ways. The most common method is to use curly braces, where key-value pairs are defined within the braces, such as `my_dict = {‘key1’: ‘value1’, ‘key2’: ‘value2′}`. Alternatively, the `dict()` constructor can be utilized, which allows for the creation of dictionaries from keyword arguments or from an iterable of key-value pairs. For example, `my_dict = dict(key1=’value1′, key2=’value2’)` or `my_dict = dict([(‘key1’, ‘value1’), (‘key2’, ‘value2’)])` are both valid approaches.
Another effective method for initializing dictionaries is through dictionary comprehensions, which provide a concise way to create dictionaries from existing iterables. For instance, one can generate a dictionary from a list of tuples using a comprehension like `{k: v for k, v in iterable}`. This method not only enhances readability but also improves performance in scenarios where dictionaries are constructed from large datasets.
In summary, Python offers multiple techniques for dictionary initialization, each with its own advantages. Understanding these various methods allows developers to choose the most appropriate one based on their specific
Author Profile
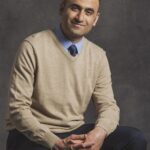
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?