How Can You Easily Load a Dataset in Python?
In the world of data science and machine learning, the ability to effectively load and manipulate datasets is a fundamental skill that can significantly influence the success of any project. Whether you’re a seasoned data scientist or just starting your journey into the realm of data analysis, understanding how to load datasets in Python is crucial. Python, with its rich ecosystem of libraries and tools, offers a variety of methods to import data from different sources, making it a versatile choice for handling data.
Loading a dataset in Python is often the first step in the data analysis process, setting the stage for everything that follows. From CSV files and Excel spreadsheets to databases and web APIs, Python provides numerous libraries such as Pandas, NumPy, and others that simplify the task of data ingestion. Each library comes with its own set of functions and methods tailored to efficiently read and process data, allowing users to focus on analysis rather than the intricacies of data handling.
As you delve deeper into the topic, you’ll discover the nuances of different data formats and the best practices for loading them into your Python environment. You’ll learn how to tackle common challenges, such as dealing with missing values and ensuring data integrity, while also exploring the powerful features that Python offers for data manipulation and exploration. With the right knowledge and tools at your disposal
Loading Datasets with Pandas
Pandas is a powerful data manipulation library in Python, widely used for data analysis. It provides various functions to load datasets from different formats, making it an essential tool for data scientists.
To load a dataset using Pandas, you typically use the `read_*` functions, where `*` corresponds to the file format. Here are some common methods:
- CSV Files: Use `pd.read_csv()`
- Excel Files: Use `pd.read_excel()`
- JSON Files: Use `pd.read_json()`
- SQL Databases: Use `pd.read_sql()`
Here’s an example of loading a CSV file:
“`python
import pandas as pd
Load a CSV file
df = pd.read_csv(‘data.csv’)
“`
This function will read the specified CSV file and return a DataFrame, which is a two-dimensional labeled data structure.
Loading Datasets with NumPy
NumPy is another essential library in Python, particularly for numerical data. It is often used for loading and manipulating arrays. To load datasets, you can utilize functions like:
- Text Files: Use `np.loadtxt()`
- Binary Files: Use `np.load()`
For example, loading a text file using NumPy can be done as follows:
“`python
import numpy as np
Load a text file
data = np.loadtxt(‘data.txt’, delimiter=’,’)
“`
This will read the text file and convert it into a NumPy array, which is optimal for mathematical computations.
Loading Datasets from Online Sources
In some cases, datasets may be available online. You can load these directly into your Python environment using libraries like Pandas. For example:
“`python
url = ‘https://example.com/data.csv’
df = pd.read_csv(url)
“`
This method is efficient as it eliminates the need to download and save the file locally.
Common Parameters for Loading Datasets
When loading datasets, various parameters can be adjusted to tailor the loading process to your needs. Below is a table summarizing some common parameters used in `pd.read_csv()`:
Parameter | Description |
---|---|
sep | Specify a custom delimiter (default is comma). |
header | Row number(s) to use as the column names. |
index_col | Column(s) to set as index. |
usecols | Subset of columns to read. |
dtype | Data type for data or columns. |
These parameters allow for greater control when loading datasets, ensuring that data is formatted correctly for analysis.
Handling Missing Data
When loading datasets, it is not uncommon to encounter missing values. Pandas provides several options to handle missing data right after loading the dataset:
- Drop missing values: Use `df.dropna()`
- Fill missing values: Use `df.fillna(value)`
Example:
“`python
Drop rows with missing values
df_cleaned = df.dropna()
Fill missing values with zero
df_filled = df.fillna(0)
“`
These functions help maintain data integrity and ensure accurate analysis as you proceed with your data science tasks.
Loading Datasets Using Pandas
Pandas is a powerful library in Python for data manipulation and analysis. It provides various functions to load datasets from different file formats.
To load a dataset using Pandas, follow these steps:
- Install Pandas if you haven’t already:
“`bash
pip install pandas
“`
- Import the library in your script:
“`python
import pandas as pd
“`
- Load a dataset from a CSV file:
“`python
df = pd.read_csv(‘path/to/your/file.csv’)
“`
- Load a dataset from an Excel file:
“`python
df = pd.read_excel(‘path/to/your/file.xlsx’, sheet_name=’Sheet1′)
“`
- Load a dataset from a JSON file:
“`python
df = pd.read_json(‘path/to/your/file.json’)
“`
- Load a dataset from a SQL database:
“`python
from sqlalchemy import create_engine
engine = create_engine(‘database_connection_string’)
df = pd.read_sql(‘SELECT * FROM table_name’, con=engine)
“`
Loading Datasets with NumPy
NumPy is another popular library, especially for numerical data. It provides functionalities to handle arrays and matrices efficiently.
To load datasets using NumPy:
- Install NumPy if needed:
“`bash
pip install numpy
“`
- Import the library:
“`python
import numpy as np
“`
- Load a dataset from a CSV file:
“`python
data = np.loadtxt(‘path/to/your/file.csv’, delimiter=’,’)
“`
- Load a dataset from a text file:
“`python
data = np.genfromtxt(‘path/to/your/file.txt’, delimiter=’,’)
“`
Loading Datasets from APIs
When working with datasets from web APIs, the `requests` library is invaluable.
- Install Requests if not installed:
“`bash
pip install requests
“`
- Import the library:
“`python
import requests
“`
- Fetch data from an API:
“`python
response = requests.get(‘https://api.example.com/data’)
data = response.json()
“`
Loading Datasets Using TensorFlow and Keras
For machine learning applications, TensorFlow and Keras offer methods for loading data seamlessly.
- Install TensorFlow:
“`bash
pip install tensorflow
“`
- Load a dataset from Keras datasets:
“`python
from tensorflow.keras.datasets import mnist
(x_train, y_train), (x_test, y_test) = mnist.load_data()
“`
- Load a dataset using TensorFlow data API:
“`python
import tensorflow as tf
dataset = tf.data.Dataset.from_tensor_slices((features, labels))
“`
Loading Datasets from Other Sources
Datasets can also be loaded from various sources like HDF5 files or directly from URLs.
- Load a dataset from HDF5:
“`python
df = pd.read_hdf(‘path/to/your/file.h5’)
“`
- Load a dataset directly from a URL:
“`python
df = pd.read_csv(‘https://example.com/data.csv’)
“`
Each method has its use case, and the choice of the loading technique will depend on your dataset’s format and source.
Expert Insights on Loading Datasets in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Loading datasets in Python is a fundamental skill for any data scientist. Utilizing libraries like Pandas allows for efficient data manipulation and analysis. I recommend using `pd.read_csv()` for CSV files, as it provides various options to handle missing values and data types effectively.”
Michael Thompson (Machine Learning Engineer, AI Solutions Group). “When working with large datasets, consider using Dask or PySpark for loading data. These libraries enable parallel processing, which significantly speeds up the loading time compared to traditional methods. It’s crucial to choose the right tool based on your dataset size and complexity.”
Sarah Patel (Senior Data Analyst, Data Insights Co.). “For loading datasets from databases, the SQLAlchemy library is invaluable. It allows seamless integration with various database systems, enabling you to load data directly into a Pandas DataFrame. This approach not only simplifies the process but also enhances data retrieval efficiency.”
Frequently Asked Questions (FAQs)
How can I load a CSV file in Python?
You can load a CSV file in Python using the `pandas` library with the `read_csv()` function. For example:
“`python
import pandas as pd
data = pd.read_csv(‘file_path.csv’)
“`
What libraries are commonly used to load datasets in Python?
Common libraries for loading datasets in Python include `pandas`, `numpy`, and `dask`. `pandas` is particularly popular for handling structured data.
Can I load Excel files in Python?
Yes, you can load Excel files using the `pandas` library with the `read_excel()` function. Ensure you have the `openpyxl` or `xlrd` library installed for this functionality.
How do I load JSON data in Python?
You can load JSON data using the `pandas` library with the `read_json()` function or the built-in `json` module. For example:
“`python
import pandas as pd
data = pd.read_json(‘file_path.json’)
“`
Is it possible to load data from a SQL database in Python?
Yes, you can load data from a SQL database using the `pandas` library with the `read_sql()` function. You will need a database connector like `sqlite3`, `psycopg2`, or `mysql-connector-python`.
What formats can I load datasets from in Python?
You can load datasets from various formats in Python, including CSV, Excel, JSON, SQL databases, and even HTML tables using libraries like `pandas`.
Loading a dataset in Python is a fundamental skill for data analysis and machine learning. There are various libraries available that facilitate this process, with the most common being Pandas, NumPy, and built-in Python functions. The choice of library often depends on the format of the dataset, such as CSV, Excel, JSON, or SQL databases. Each library provides specific functions tailored to efficiently read and manipulate data from these formats.
Pandas, in particular, is widely regarded for its powerful data manipulation capabilities. The `read_csv()` function is frequently used for loading CSV files, while `read_excel()` serves a similar purpose for Excel files. For JSON data, the `read_json()` function can be employed. Additionally, Pandas supports reading from SQL databases through the `read_sql()` function, making it a versatile tool for data ingestion.
It is also essential to consider data preprocessing after loading the dataset. This includes handling missing values, converting data types, and filtering rows or columns as necessary. By effectively utilizing the appropriate libraries and functions, users can streamline their workflow and prepare their datasets for further analysis or modeling.
In summary, mastering the techniques for loading datasets in Python is crucial for any data-driven professional. Understanding the
Author Profile
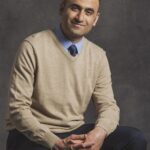
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?