How Can You Create a Matrix in Python? A Step-by-Step Guide
Creating and manipulating matrices is a fundamental skill in programming, particularly in fields such as data science, machine learning, and scientific computing. In Python, a versatile and powerful language, the ability to work with matrices opens up a world of possibilities for data analysis and mathematical modeling. Whether you’re a beginner eager to learn the ropes or an experienced programmer looking to refine your skills, understanding how to make a matrix in Python is essential for tackling complex problems efficiently.
In Python, matrices can be constructed using various methods, each suited for different scenarios and preferences. The most common approach involves utilizing built-in data structures like lists or tuples, while libraries such as NumPy provide more advanced functionalities for handling large datasets and performing intricate mathematical operations. By harnessing these tools, you can create matrices that are not only easy to manipulate but also optimized for performance.
As we delve deeper into the topic, we will explore the different ways to create matrices, from simple lists to leveraging powerful libraries. You’ll learn about the advantages of each method and how they can be applied in real-world situations, setting the stage for more complex operations and analyses. Get ready to unlock the potential of matrices in Python and elevate your programming skills to new heights!
Using Lists to Create a Matrix
Creating a matrix in Python can be accomplished using nested lists. A matrix can be represented as a list of lists, where each inner list represents a row of the matrix. Here’s how you can do it:
python
# Creating a 2×3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6]
]
In this example, `matrix` consists of two lists, each containing three elements. You can access elements using two indices: the first for the row and the second for the column. For example, `matrix[0][1]` will return `2`.
Using NumPy for Matrix Operations
For more complex operations and better performance, the NumPy library is highly recommended. NumPy provides a powerful array structure and functions that simplify matrix manipulation. To create a matrix using NumPy, follow these steps:
- Install NumPy if you haven’t already:
bash
pip install numpy
- Import NumPy and create a matrix:
python
import numpy as np
# Creating a 2×3 matrix using NumPy
matrix_np = np.array([[1, 2, 3], [4, 5, 6]])
Using NumPy allows for efficient calculations and a wide range of matrix operations such as addition, multiplication, and transposition.
Creating a Matrix with Custom Functions
You may also define a custom function to generate matrices based on specific requirements. For example, creating a matrix filled with zeros or ones can be useful for initializing data structures. Here’s a simple function to create a matrix:
python
def create_matrix(rows, cols, fill_value=0):
return [[fill_value for _ in range(cols)] for _ in range(rows)]
# Create a 3×4 matrix filled with zeros
zero_matrix = create_matrix(3, 4)
This function generates a matrix of specified dimensions, initialized with a given fill value.
Matrix Operations Overview
When working with matrices, you may need to perform various operations. Here’s a summary of some common matrix operations:
Operation | Description |
---|---|
Addition | Element-wise addition of matrices. |
Subtraction | Element-wise subtraction of matrices. |
Multiplication | Matrix multiplication (dot product). |
Transposition | Flipping a matrix over its diagonal. |
Determinant | A scalar value that describes the matrix. |
Example: Matrix Multiplication
Matrix multiplication can be performed using NumPy as follows:
python
# Creating two matrices
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
# Multiplying matrices A and B
C = np.dot(A, B)
In this example, `C` will contain the result of multiplying matrices `A` and `B`. Using NumPy ensures that the operation is efficient and straightforward.
Creating a Matrix Using Nested Lists
In Python, one of the simplest ways to create a matrix is by using nested lists. Each sub-list represents a row in the matrix. Here’s how to do it:
python
# Creating a 3×3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
You can access elements in the matrix using the syntax `matrix[row][column]`. For example, `matrix[1][2]` will return `6`.
Using NumPy for Matrix Creation
NumPy is a powerful library for numerical computations in Python, which provides a more efficient way to handle matrices and arrays. To create a matrix with NumPy, you first need to install the library if you haven’t done so already:
bash
pip install numpy
Then, you can create a matrix as follows:
python
import numpy as np
# Creating a 3×3 matrix using NumPy
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
NumPy provides additional functionalities that make operations on matrices straightforward, such as element-wise operations, matrix multiplication, and more.
Creating Matrices with Specific Functions
NumPy includes several built-in functions to create matrices with specific configurations:
- zeros: Create a matrix filled with zeros.
- ones: Create a matrix filled with ones.
- identity: Create an identity matrix.
- arange: Create a matrix with a range of values.
Here’s how to use these functions:
python
# Creating different types of matrices
zeros_matrix = np.zeros((3, 3)) # 3×3 matrix of zeros
ones_matrix = np.ones((2, 4)) # 2×4 matrix of ones
identity_matrix = np.identity(4) # 4×4 identity matrix
range_matrix = np.arange(1, 10).reshape(3, 3) # 3×3 matrix from a range
Manipulating Matrices
Once you have created a matrix, you may want to perform various operations on it. Here are some common manipulations:
- Transposing a Matrix:
python
transposed_matrix = np.transpose(matrix)
- Adding Two Matrices:
python
result_matrix = matrix + np.array([[1, 1, 1], [1, 1, 1], [1, 1, 1]])
- Matrix Multiplication:
python
product_matrix = np.dot(matrix, np.array([[1, 0, 0], [0, 1, 0], [0, 0, 1]]))
- Element-wise Operations:
python
squared_matrix = matrix ** 2 # Squaring each element
Matrix Creation
Creating and manipulating matrices in Python can be efficiently handled using either nested lists or the NumPy library. Depending on the complexity of your operations and the size of your data, choosing the right method will enhance performance and ease of use.
Expert Insights on Creating Matrices in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Creating matrices in Python can be efficiently handled using libraries such as NumPy. This library provides a powerful array object that simplifies matrix operations, making it an essential tool for any data analysis or scientific computing task.”
Michael Chen (Software Engineer, Python Programming Hub). “For beginners, I recommend starting with nested lists to create matrices in Python. However, as projects scale, transitioning to NumPy arrays will enhance performance and provide a broader range of mathematical functions.”
Sarah Thompson (Educator, Python Academy). “Teaching how to make a matrix in Python involves not only the syntax but also the underlying concepts of linear algebra. Using visual aids and practical examples can significantly enhance students’ understanding of matrix operations in Python.”
Frequently Asked Questions (FAQs)
How can I create a simple matrix in Python?
You can create a simple matrix using a list of lists. For example, `matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]` represents a 3×3 matrix.
What libraries can I use to create matrices in Python?
You can use libraries such as NumPy, which provides powerful functions for matrix creation and manipulation. For example, `import numpy as np; matrix = np.array([[1, 2], [3, 4]])`.
How do I create a matrix with NumPy?
To create a matrix with NumPy, first import the library, then use the `np.array()` function. For instance, `matrix = np.array([[1, 2], [3, 4]])` creates a 2×2 matrix.
Can I create a matrix with random values in Python?
Yes, you can create a matrix with random values using NumPy. Use `np.random.rand(rows, columns)` to generate a matrix filled with random floats. For example, `matrix = np.random.rand(3, 3)` creates a 3×3 matrix with random values.
How do I access elements in a matrix created with NumPy?
You can access elements in a NumPy matrix using indexing. For example, `matrix[0, 1]` accesses the element in the first row and second column.
Is it possible to perform matrix operations in Python?
Yes, Python supports various matrix operations using libraries like NumPy. You can perform addition, subtraction, multiplication, and more using built-in functions such as `np.add()`, `np.subtract()`, and `np.dot()` for matrix multiplication.
Creating a matrix in Python can be achieved through various methods, each catering to different needs and preferences. The most common approaches include using nested lists, the NumPy library, and the Pandas library. Nested lists provide a straightforward way to represent matrices, while NumPy offers a more efficient and powerful framework for numerical computations, making it the preferred choice for scientific and engineering applications. Pandas, on the other hand, is ideal for data manipulation and analysis, especially when working with labeled data.
When using nested lists, one can simply create a list of lists, where each inner list represents a row of the matrix. However, this method lacks many of the functionalities provided by specialized libraries. In contrast, NumPy allows for the creation of matrices using the `numpy.array()` function, which not only simplifies the syntax but also enhances performance through optimized operations. Additionally, NumPy provides a wide range of mathematical functions that can be applied directly to matrices.
Pandas offers another layer of abstraction with its DataFrame structure, which can be used to create matrices with labeled axes. This is particularly useful for data analysis tasks where the context of data is crucial. The choice between these methods largely depends on the specific requirements of the task at hand, such
Author Profile
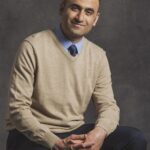
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?