How Can You Create a Table in Python? A Step-by-Step Guide
Creating tables in Python is an essential skill for anyone looking to manage and present data effectively. Whether you’re a data analyst, a software developer, or a researcher, the ability to organize information into a structured format can significantly enhance your projects. In a world where data is king, knowing how to create and manipulate tables can set you apart, making your work not only more efficient but also more visually appealing.
In Python, there are several libraries and tools available that simplify the process of creating tables, each catering to different needs and preferences. From the widely-used Pandas library, which offers robust data manipulation capabilities, to more straightforward options like PrettyTable for quick outputs in the console, the possibilities are vast. Understanding these tools and their functionalities can empower you to choose the right approach for your specific use case, whether it’s for data analysis, reporting, or even web applications.
Moreover, mastering table creation in Python opens the door to more advanced data visualization techniques, allowing you to present your findings in a clear and compelling manner. As we delve deeper into this topic, you’ll discover various methods to create tables, customize their appearance, and leverage them for data analysis, paving the way for more insightful and impactful results. Get ready to transform your data handling skills and elevate your Python
Creating Tables Using Pandas
Pandas is a powerful data manipulation library in Python, widely used for data analysis. It provides an easy way to create and manage tables through its DataFrame structure. To create a table with Pandas, you can start by importing the library and then define your data in a structured format, such as a dictionary or a list of lists.
Here is a simple example of creating a table using a dictionary:
python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
print(df)
This code snippet creates a DataFrame that represents a table with names, ages, and cities. The output will resemble the following:
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
You can also create tables using lists of lists. Here’s how to do it:
python
data = [
[‘Alice’, 25, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]
]
df = pd.DataFrame(data, columns=[‘Name’, ‘Age’, ‘City’])
print(df)
Using PrettyTable for Terminal Output
When you want to display tables in a more visually appealing format in the terminal, you can use the PrettyTable library. This library allows you to create ASCII tables that are easy to read. First, install the library using pip:
bash
pip install prettytable
Then, you can create a table as follows:
python
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
The output will look like this:
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
Creating HTML Tables
For web applications, you might want to create HTML tables. Using Pandas, you can easily convert a DataFrame to HTML format. Here’s how to achieve that:
python
html_table = df.to_html()
print(html_table)
This will generate an HTML representation of the DataFrame. Here’s an example of what the output might look like:
Name | Age | City |
---|---|---|
Alice | 25 | New York |
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
This HTML code can be embedded directly into a web page, allowing for a clean and structured presentation of your data.
Creating a Simple Table Using Pandas
Pandas is a powerful library in Python for data manipulation and analysis, which makes it particularly suitable for creating tables. Below is a step-by-step guide on how to create a simple table using a Pandas DataFrame.
- Install Pandas: If you haven’t already, install the Pandas library using pip.
bash
pip install pandas
- Import the Library: Start by importing Pandas in your Python script.
python
import pandas as pd
- Create a DataFrame: You can create a DataFrame by passing a dictionary or a list of lists.
python
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [24, 30, 22],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
- Display the DataFrame: Use the `print()` function to show your table.
python
print(df)
The output will look like this:
Name Age City
0 Alice 24 New York
1 Bob 30 Los Angeles
2 Charlie 22 Chicago
Creating a Table Using PrettyTable
For a more visually appealing table in the console, PrettyTable can be used. Follow these steps:
- Install PrettyTable: Install the library via pip.
bash
pip install prettytable
- Import PrettyTable: Import it into your script.
python
from prettytable import PrettyTable
- Create a Table: Instantiate a PrettyTable object and add columns.
python
table = PrettyTable()
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 24, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 22, “Chicago”])
- Display the Table: Simply print the table.
python
print(table)
The output will be a formatted table:
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 24 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 22 | Chicago |
+———+—–+————-+
Creating Tables with Matplotlib
Matplotlib allows you to create tables that can be displayed alongside plots. To create a table using Matplotlib, follow these steps:
- Install Matplotlib: Use pip to install.
bash
pip install matplotlib
- Import the Library: Import Matplotlib and NumPy.
python
import matplotlib.pyplot as plt
import numpy as np
- Prepare Data: Define your data in a NumPy array.
python
data = np.array([
[‘Alice’, 24, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 22, ‘Chicago’]
])
- Create a Table: Use the `table()` function to create the table.
python
fig, ax = plt.subplots()
ax.axis(‘tight’)
ax.axis(‘off’)
ax.table(cellText=data, colLabels=[“Name”, “Age”, “City”], cellLoc = ‘center’, loc=’center’)
- Display the Table: Use `plt.show()` to display.
python
plt.show()
This will generate a visual table within a Matplotlib figure.
Using Tabulate for Command Line Tables
Tabulate is a simple library for creating tables in the command line. Here’s how to use it:
- Install Tabulate: Install using pip.
bash
pip install tabulate
- Import Tabulate: Import the library in your script.
python
from tabulate import tabulate
- Prepare Data: Use a list of lists or a list of dictionaries.
python
data = [
[“Alice”, 24, “New York”],
[“Bob”, 30, “Los Angeles”],
[“Charlie”, 22, “Chicago”]
]
- Create and Print the Table:
python
print(tabulate(data, headers=[“Name”, “Age”, “City”], tablefmt=”grid”))
The output will be formatted as follows:
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 24 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 22 | Chicago |
+———+—–+————-+
These methods provide different ways to create tables in Python, catering to various needs and output formats.
Expert Insights on Creating Tables in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When creating tables in Python, utilizing libraries such as Pandas is essential. It allows for efficient data manipulation and provides powerful tools for data analysis, making it a preferred choice among data professionals.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For beginners, starting with the built-in ‘tabulate’ library can simplify the process of displaying tabular data. It offers a straightforward syntax and various output formats, making it an excellent choice for quick table generation.”
Sarah Thompson (Python Instructor, LearnPythonNow). “Understanding the structure of your data is crucial before creating a table. Using libraries like Matplotlib or Seaborn for visualization can complement your tables, providing a more comprehensive understanding of the data at hand.”
Frequently Asked Questions (FAQs)
How can I create a simple table in Python using lists?
You can create a simple table in Python by using nested lists. Each sublist represents a row, and you can print it using a loop to format it neatly.
What libraries can I use to create tables in Python?
You can use libraries such as Pandas for data manipulation, PrettyTable for easy table formatting, and Tabulate for creating simple ASCII tables.
How do I create a DataFrame table using Pandas?
To create a DataFrame in Pandas, import the library, then use `pd.DataFrame(data)` where `data` can be a dictionary, list of lists, or another DataFrame.
Can I customize the appearance of a table in Python?
Yes, you can customize the appearance of tables using libraries like PrettyTable or by applying styles in Pandas with the `style` attribute for DataFrames.
Is it possible to export a table to a file in Python?
Yes, you can export tables to various file formats using Pandas, such as CSV with `DataFrame.to_csv()` or Excel with `DataFrame.to_excel()`.
How do I install the necessary libraries to create tables in Python?
You can install libraries like Pandas and PrettyTable using pip. Run `pip install pandas prettytable` in your command line or terminal.
Creating a table in Python can be accomplished using various libraries, each offering unique features and functionalities. The most commonly used libraries for this purpose include Pandas, PrettyTable, and tabulate. Pandas is particularly powerful for data manipulation and analysis, allowing users to create DataFrames that can be easily converted into tables. PrettyTable is ideal for generating simple ASCII tables, while tabulate offers a range of formatting options for both plain-text and HTML tables.
To create a table using Pandas, one can start by importing the library and then defining a DataFrame with the desired data. This DataFrame can be displayed as a table with just a few lines of code. For instance, using the `pd.DataFrame()` function allows users to create a structured table effortlessly. Similarly, PrettyTable requires initializing a PrettyTable object and adding rows and columns to it, making it straightforward for quick table generation.
When choosing a method for creating tables in Python, it is essential to consider the specific requirements of the project. For data analysis tasks that involve complex datasets, Pandas is the most suitable choice due to its extensive capabilities. Conversely, for simpler applications or when a quick visual representation is needed, PrettyTable or tabulate can be more efficient
Author Profile
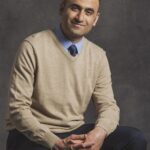
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?