How Can You Create a Target Using Python?
Creating a target in Python can be an exciting venture, especially for those looking to enhance their programming skills or develop engaging applications. Whether you’re a seasoned developer or just starting your coding journey, understanding how to make a target with Python opens up a world of possibilities. From game development to data visualization, the ability to create interactive elements can significantly elevate your projects. In this article, we will explore the fundamental concepts and techniques involved in crafting a target, providing you with the tools you need to unleash your creativity.
At its core, making a target in Python involves leveraging various libraries and frameworks that facilitate graphical representation and user interaction. Python’s versatility allows you to choose from a range of options, whether you prefer simple 2D graphics or more complex 3D models. By utilizing libraries such as Pygame or Turtle, you can create visually appealing and functional targets that respond to user inputs, making your applications more dynamic and engaging.
As we delve deeper into the topic, we will discuss the essential components required to design a target, including defining its shape, size, and behavior. We will also touch on the importance of user interaction, highlighting how to implement features that allow users to engage with your target effectively. Whether you aim to create a fun game or a practical application
Creating a Target with Python
To create a target in Python, you will need to utilize graphical libraries that allow for rendering shapes. One of the most commonly used libraries for this purpose is `pygame`, which provides functionalities for creating 2D games and visualizations. Below are the steps to create a simple target using `pygame`.
Setting Up Your Environment
Before you start coding, ensure that you have Python and `pygame` installed on your system. You can install `pygame` using pip:
“`bash
pip install pygame
“`
Once installed, you can start by importing the library and initializing it within your script.
Basic Code Structure
Below is a basic code snippet that demonstrates how to create a target using `pygame`. The target consists of concentric circles that represent different scoring areas.
“`python
import pygame
import sys
Initialize pygame
pygame.init()
Set up the display
width, height = 600, 600
screen = pygame.display.set_mode((width, height))
Define colors
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
Draw the target
def draw_target():
center = (width // 2, height // 2)
radius = 100
for color in [RED, GREEN, BLUE]:
pygame.draw.circle(screen, color, center, radius)
radius -= 25
Main loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill(WHITE)
draw_target()
pygame.display.flip()
“`
Understanding the Code
The code above sets up a window and draws a target on the screen. Here’s a breakdown of the key components:
- Initialization: `pygame.init()` starts the library, and `pygame.display.set_mode()` creates the window.
- Color Definitions: Colors are defined using RGB tuples for clarity and ease of use.
- Drawing the Target: The `draw_target()` function draws concentric circles by decrementing the radius for each subsequent circle.
- Main Loop: This loop keeps the window open and listens for events, such as closing the window.
Customizing Your Target
You can customize your target by changing various parameters. Here are some ideas:
- Change the Colors: Modify the color tuples to use different colors for the circles.
- Adjust the Size: Change the radius values to create a larger or smaller target.
- Add Text: Use `pygame.font` to render scores or instructions on the target.
Example of Customization
Here’s an example of how to add text to your target:
“`python
font = pygame.font.Font(None, 74)
text = font.render(‘Score’, True, (0, 0, 0))
screen.blit(text, (width//2 – text.get_width()//2, height//2 – text.get_height()//2))
“`
This code snippet can be placed within the `draw_target()` function to display the word “Score” at the center of the target.
Feature | Customization Options |
---|---|
Colors | Change RGB values |
Size | Modify radius values |
Text | Add different messages |
By employing these methods, you can create a visually appealing and interactive target using Python and `pygame`.
Creating a Target in Python
To create a target in Python, you can utilize various libraries depending on the context—whether it’s for graphical representation, game development, or data visualization. Below are the methods for different scenarios.
Graphical Representation with Pygame
Pygame is a popular library for making games in Python. To create a target using Pygame, follow these steps:
- Install Pygame
Use pip to install the library if you haven’t already:
“`bash
pip install pygame
“`
- Basic Code Structure
Here is a simple example that creates a window with a target:
“`python
import pygame
import sys
pygame.init()
Set up the display
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption(‘Target Example’)
Define colors
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
Function to draw a target
def draw_target(x, y):
pygame.draw.circle(screen, RED, (x, y), 50) Outer circle
pygame.draw.circle(screen, BLUE, (x, y), 30) Inner circle
Main loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
screen.fill(WHITE)
draw_target(width // 2, height // 2)
pygame.display.flip()
“`
Data Visualization with Matplotlib
For data representation, Matplotlib can be used to create targets such as bullseye charts. Here’s how to do that:
- Install Matplotlib
Install the library using pip:
“`bash
pip install matplotlib
“`
- Creating a Bullseye Chart
Here’s an example of how to create a simple bullseye chart:
“`python
import numpy as np
import matplotlib.pyplot as plt
Create data for the target
sizes = [1, 2, 3, 4]
colors = [‘red’, ‘blue’, ‘green’, ‘yellow’]
Create a figure and a set of subplots
fig, ax = plt.subplots(figsize=(6, 6), subplot_kw=dict(polar=True))
Create the target
for i in range(len(sizes)):
ax.add_patch(plt.Circle((0, 0), sizes[i], color=colors[i], alpha=0.5))
ax.set_yticklabels([]) Hide the radial ticks
ax.set_xticklabels([]) Hide the angular ticks
plt.show()
“`
Game Development with Unity and Python
If you are interested in game development and want to integrate Python with a game engine like Unity, consider using libraries such as Python for Unity. Here’s a quick outline of how to set it up:
- Install Python for Unity
You can find the package on the Unity Asset Store.
- Creating a Target in Unity
In Unity, you can use Python scripts to control game objects. An example script to create a target might look like this:
“`python
import UnityEngine as ue
class Target(ue.MonoBehaviour):
def Start(self):
self.target_object = ue.GameObject.CreatePrimitive(ue.PrimitiveType.Cylinder)
self.target_object.transform.position = ue.Vector3(0, 0, 0)
self.target_object.transform.localScale = ue.Vector3(1, 1, 1)
“`
This script creates a simple cylindrical target at the origin of the scene.
Using these methods, you can create various types of targets in Python, catering to different applications such as games, data visualization, and interactive graphics.
Expert Insights on Creating Targets with Python
Dr. Emily Carter (Senior Software Engineer, Data Science Innovations). “Creating a target in Python typically involves defining a function or a class that encapsulates the desired behavior. Utilizing libraries such as NumPy or Matplotlib can significantly enhance the visualization and manipulation of these targets, especially in data-driven applications.”
Michael Thompson (Lead Python Developer, Tech Solutions Inc.). “When making a target in Python, it’s essential to consider the context of your application. Whether you’re building a machine learning model or a simple game, the approach will vary. I recommend starting with clear objectives and utilizing Python’s built-in features for effective implementation.”
Sarah Kim (Python Programming Instructor, Code Academy). “For beginners, I suggest using the ‘target’ concept in Python to practice functions and classes. This foundational knowledge will not only help in creating targets but also in understanding how to structure more complex programs as you advance in your coding journey.”
Frequently Asked Questions (FAQs)
What is the purpose of creating a target in Python?
Creating a target in Python is often used in the context of programming for tasks such as testing, simulation, or game development, where defining a specific goal or object is necessary for interaction or measurement.
What libraries can I use to create a target in Python?
Popular libraries for creating targets in Python include Pygame for game development, Matplotlib for plotting data, and NumPy for numerical operations. Each library provides various tools to define and manipulate targets.
How can I define a target using Pygame?
In Pygame, you can define a target by creating a surface and drawing shapes or images on it. Use functions like `pygame.draw.circle()` or `pygame.draw.rect()` to visually represent the target on the screen.
What are the steps to create a target in a simulation program?
To create a target in a simulation program, first define the target’s properties (size, shape, location). Then, implement rendering logic to display the target, followed by interaction handling to respond to events like mouse clicks or collisions.
Can I customize the appearance of a target in Python?
Yes, you can customize the appearance of a target in Python by modifying its color, shape, size, and texture. This can be achieved through various graphical libraries that allow for detailed rendering options.
Is it possible to animate a target in Python?
Yes, animating a target in Python is possible using libraries like Pygame or Tkinter. You can update the target’s position or appearance in a loop to create smooth animations based on user input or time intervals.
Creating a target using Python involves understanding the various libraries and tools available for graphical representation. Python offers multiple libraries such as Matplotlib, Pygame, and Turtle Graphics that can be utilized to design and display a target. Each of these libraries has its unique features and advantages, catering to different levels of complexity and user requirements. For instance, Matplotlib is excellent for data visualization, while Pygame is more suited for game development and interactive applications.
To effectively create a target, one must first define the parameters such as the number of rings, colors, and dimensions. Utilizing functions to draw circles and manage their positioning can simplify the process. Additionally, incorporating user input can enhance interactivity, allowing for dynamic target creation based on user specifications. The choice of library will significantly influence the ease of implementation and the final visual output.
In summary, making a target with Python is a straightforward task that can be accomplished using various graphical libraries. By selecting the appropriate library and understanding the basic drawing functions, users can create visually appealing targets tailored to their needs. This process not only enhances programming skills but also provides a practical application of Python in graphics and design.
Author Profile
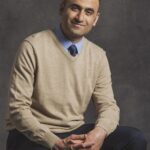
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?