How Can You Create a Timer Using Python?
Have you ever found yourself needing to keep track of time for a specific task, whether it’s a cooking session, a workout routine, or simply a reminder to take a break? In our fast-paced world, timers have become essential tools for productivity and time management. If you’re a Python enthusiast looking to harness the power of programming to create your own timer, you’re in the right place! This article will guide you through the process of building a simple yet effective timer using Python, empowering you to customize it to fit your unique needs.
Creating a timer in Python is not only a practical project but also a fantastic way to enhance your coding skills. With Python’s straightforward syntax and powerful libraries, you can easily implement a timer that counts down from a specified duration or counts up from zero. Whether you’re a beginner eager to learn or an experienced coder wanting to explore new functionalities, this project offers a perfect blend of challenge and creativity.
In the following sections, we’ll delve into the fundamental concepts of time management in programming, explore the various methods available in Python for handling time, and ultimately guide you through the steps to create your very own timer application. Get ready to unlock the potential of Python and take control of your time like never before!
Creating a Simple Timer
To create a simple timer in Python, you can utilize the built-in `time` module. This module provides various functions to work with time-related tasks. A straightforward implementation can be achieved using a combination of a loop and the `time.sleep()` function.
Here’s a basic example of a countdown timer:
“`python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’) Overwrite the timer display
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
Example usage
countdown_timer(10)
“`
In this code:
- The `countdown_timer` function takes the number of seconds as an argument.
- The `divmod` function is used to convert total seconds into minutes and seconds.
- The timer is printed in a formatted manner, and `end=’\r’` ensures that it updates on the same line.
- The `time.sleep(1)` function pauses the execution for one second, creating the countdown effect.
Enhancing the Timer with User Input
To make the timer more interactive, you can accept user input for the countdown duration. Here’s how you can enhance the previous example:
“`python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
try:
total_seconds = int(input(“Enter the time in seconds: “))
countdown_timer(total_seconds)
except ValueError:
print(“Please enter a valid integer.”)
“`
This implementation includes error handling to ensure that the input is a valid integer.
Using Tkinter for a GUI Timer
For those interested in creating a graphical user interface (GUI) timer, Python’s `Tkinter` library is an excellent choice. Below is an example of how to create a simple countdown timer using `Tkinter`:
“`python
import tkinter as tk
import time
def countdown_timer(seconds):
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
label[‘text’] = timer
if seconds > 0:
root.after(1000, countdown_timer, seconds – 1)
else:
label[‘text’] = “Time’s up!”
root = tk.Tk()
root.title(“Countdown Timer”)
label = tk.Label(root, font=(‘Helvetica’, 48), fg=’red’)
label.pack()
total_seconds = 10 Set the duration for the timer
countdown_timer(total_seconds)
root.mainloop()
“`
In this GUI example:
- A `Tk` window is created with a label to display the countdown.
- The `after` method schedules the countdown function to run every second.
- The timer updates in the label until it reaches zero.
Timer Features Overview
When designing a timer application, consider implementing additional features for improved functionality. Below is a summary of potential features:
Feature | Description |
---|---|
Pause/Resume | Allow users to pause the countdown and resume later. |
Reset | Provide an option to reset the timer to a specified duration. |
Sound Notification | Play a sound when the timer reaches zero. |
Multiple Timers | Enable users to run multiple timers simultaneously. |
Incorporating these features can enhance user experience and make your timer application more versatile.
Creating a Simple Timer in Python
To create a timer in Python, you can utilize the built-in `time` module, which provides various time-related functions. The following is a straightforward example of a countdown timer.
“`python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
Example usage
countdown_timer(10)
“`
In this example:
- The function `countdown_timer` takes an integer input representing the number of seconds.
- The `divmod` function divides the total seconds into minutes and seconds.
- The timer is formatted and printed in `MM:SS` format.
- The loop continues until the countdown reaches zero, at which point it prints “Time’s up!”.
Implementing a Timer with User Input
You can modify the timer to accept user input for the countdown duration. Below is an example that includes input validation.
“`python
def get_timer_duration():
while True:
try:
seconds = int(input(“Enter the time in seconds: “))
if seconds < 0:
raise ValueError("Please enter a positive number.")
return seconds
except ValueError as e:
print(e)
Using the previous countdown function
duration = get_timer_duration()
countdown_timer(duration)
```
Key points for this implementation:
- The `get_timer_duration` function prompts the user for input until a valid positive integer is received.
- Exception handling ensures that the program does not crash on invalid input.
Enhancing the Timer with Threading
For applications requiring a timer that doesn’t block the main program, threading can be employed. The following example demonstrates how to implement a timer that allows for concurrent execution.
“`python
import threading
def countdown_timer_thread(seconds):
def run_timer():
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
timer_thread = threading.Thread(target=run_timer)
timer_thread.start()
Example usage
countdown_timer_thread(10)
“`
Considerations for this approach:
- A new thread is created to run the timer, allowing other tasks to run simultaneously.
- This is particularly useful in GUI applications or scripts that require user interaction while the timer is active.
Creating a Graphical Timer Using Tkinter
For a more visual approach, you can create a graphical user interface (GUI) timer using Tkinter. Below is a simple implementation.
“`python
import tkinter as tk
def start_timer():
try:
seconds = int(entry.get())
countdown_timer(seconds)
except ValueError:
label.config(text=”Please enter a valid number.”)
def countdown_timer(seconds):
if seconds >= 0:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
label.config(text=timer)
root.after(1000, countdown_timer, seconds – 1)
else:
label.config(text=”Time’s up!”)
root = tk.Tk()
root.title(“Timer”)
entry = tk.Entry(root)
entry.pack()
start_button = tk.Button(root, text=”Start Timer”, command=start_timer)
start_button.pack()
label = tk.Label(root, font=(‘Helvetica’, 48))
label.pack()
root.mainloop()
“`
In this example:
- The GUI consists of an entry field for user input, a button to start the timer, and a label to display the countdown.
- The `after` method schedules the countdown function to be called after 1000 milliseconds (1 second), allowing for a responsive interface.
Using External Libraries for Advanced Features
For more advanced timer functionalities, consider using external libraries like `pygame` or `dateutil`. These libraries can offer additional features such as sound notifications or more sophisticated time manipulation.
Here’s a simple example using `playsound` for sound notifications:
“`python
from playsound import playsound
def countdown_with_sound(seconds):
countdown_timer(seconds)
playsound(‘alarm.mp3’)
Ensure you have an audio file named ‘alarm.mp3’ in your working directory.
“`
This snippet plays an audio file when the timer ends, adding an auditory cue to the visual countdown.
Implementing timers in Python can range from simple console applications to complex GUI applications, providing flexibility depending on the requirements of your project.
Expert Insights on Creating a Timer in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Creating a timer in Python can be efficiently accomplished using the built-in `time` module. By leveraging functions like `time.sleep()` alongside threading, developers can create responsive timers that enhance user experience.”
Michael Chen (Python Developer Advocate, CodeCraft). “For those looking to implement a countdown timer, utilizing the `tkinter` library can provide a simple graphical interface. This allows for a more interactive approach, making it suitable for educational applications and projects.”
Lisa Patel (Data Scientist, AI Solutions Inc.). “When building a timer for data processing tasks, consider using asynchronous programming with the `asyncio` library. This method allows for more efficient resource management, especially when handling multiple timers or tasks simultaneously.”
Frequently Asked Questions (FAQs)
How can I create a simple timer in Python?
You can create a simple timer in Python using the `time` module. Use `time.sleep(seconds)` to pause execution for a specified number of seconds, and then print the elapsed time.
What libraries can I use to make a more advanced timer in Python?
For a more advanced timer, you can use libraries such as `threading` for concurrent execution or `Tkinter` for a graphical user interface. The `time` and `datetime` modules can also enhance functionality.
How do I implement a countdown timer in Python?
To implement a countdown timer, use a loop that decrements a variable representing time. Inside the loop, use `time.sleep(1)` to create a one-second interval and print the remaining time until it reaches zero.
Can I set a timer to trigger a function after a certain period?
Yes, you can use the `threading.Timer` class to execute a function after a specified delay. Instantiate `Timer` with the delay and the function to call, and then start it with `.start()`.
How can I format the timer output to display minutes and seconds?
You can format the timer output by calculating minutes and seconds using integer division and the modulus operator. For example, `minutes = total_seconds // 60` and `seconds = total_seconds % 60`.
Is it possible to pause and resume a timer in Python?
Yes, you can implement pause and resume functionality by using a flag to control the timer’s state. Store the elapsed time when paused and continue from that point when resumed.
Creating a timer in Python can be accomplished using various methods, depending on the specific requirements and complexity desired. The most straightforward approach involves utilizing the built-in `time` module, which allows for simple countdown timers. By leveraging functions like `time.sleep()`, developers can create a delay that simulates the passage of time, effectively implementing a basic timer.
For more advanced functionality, such as a graphical user interface (GUI), Python libraries like Tkinter can be employed. Tkinter provides a framework for building interactive applications, enabling users to start, stop, and reset timers with visual elements. This approach enhances user experience and makes timers more accessible to those who prefer visual interaction over command-line interfaces.
Additionally, integrating threading can allow timers to run in the background without freezing the main program. This is particularly useful in applications where multiple tasks need to be performed simultaneously. By using the `threading` module, developers can create non-blocking timers that operate alongside other processes, improving overall application performance.
Python offers a versatile environment for creating timers, ranging from simple command-line implementations to complex GUI applications. By understanding the various tools and libraries available, developers can choose the most suitable method for their specific needs,
Author Profile
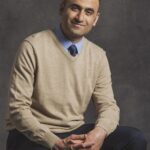
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?