How Can You Create a Website Using Python?
Creating a website can seem like a daunting task, especially if you’re new to programming or web development. However, with the right tools and a little guidance, you can harness the power of Python to build a dynamic and engaging website that showcases your ideas, products, or services. Python, known for its simplicity and versatility, has become a popular choice among developers for web applications, thanks to its robust frameworks and libraries that streamline the development process. Whether you’re looking to create a personal blog, an e-commerce platform, or a portfolio site, Python offers the flexibility and functionality to bring your vision to life.
In this article, we will explore the essential steps and concepts involved in making a website using Python. From understanding the foundational components of web development to selecting the right framework that suits your project needs, we will guide you through the process. You’ll learn about the various tools available, how to set up your development environment, and the importance of both front-end and back-end development in creating a seamless user experience.
As we delve deeper into the world of Python web development, you’ll discover how to leverage popular frameworks like Flask and Django, which simplify the process of building web applications. By the end of this journey, you’ll not only have the knowledge to create your own website but also
Choosing the Right Framework
When creating a website with Python, selecting the appropriate framework is critical as it will dictate the development process and the capabilities of your web application. The two most popular web frameworks in Python are Flask and Django.
Flask is a micro-framework that is lightweight and flexible, making it ideal for smaller applications or projects where you want to maintain control over the components you include. It allows for rapid development and is easy to learn for beginners.
Django, on the other hand, is a high-level framework that encourages rapid development and clean, pragmatic design. It comes with built-in features such as an ORM, authentication, and an admin interface, which can save significant development time.
Comparison Table: Flask vs. Django
Feature | Flask | Django |
---|---|---|
Type | Micro-framework | Full-stack framework |
Flexibility | High | Moderate |
Built-in Features | Minimal | Extensive |
Learning Curve | Easy | Moderate |
Setting Up Your Development Environment
Before you start coding, you need to set up your development environment. This typically includes installing Python, the chosen framework, and any additional libraries or dependencies.
- Install Python: Ensure you have Python installed on your system. You can download it from the [official Python website](https://www.python.org/downloads/).
- Create a Virtual Environment: It’s recommended to use a virtual environment to manage dependencies. This can be done using the following commands:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
“`
- Install Framework:
- For Flask:
“`bash
pip install Flask
“`
- For Django:
“`bash
pip install Django
“`
Building Your First Application
After setting up your environment, you can proceed to create your first web application. Below are the basic steps for both Flask and Django.
Flask Example:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == ‘__main__’:
app.run(debug=True)
“`
Django Example:
- Create a new Django project:
“`bash
django-admin startproject myproject
cd myproject
python manage.py runserver
“`
- Create an application within the project:
“`bash
python manage.py startapp myapp
“`
- Add the following code in `views.py` of the app:
“`python
from django.http import HttpResponse
def home(request):
return HttpResponse(“Hello, Django!”)
“`
- Configure your URL routing in `urls.py`.
Deploying Your Website
Once your website is ready, deployment is the next step. Common platforms for hosting Python web applications include Heroku, AWS, and DigitalOcean.
- Heroku: Simple to use and offers a free tier for small applications. You can deploy using Git.
- AWS: Offers more control and scaling options but has a steeper learning curve.
- DigitalOcean: Provides a straightforward way to deploy applications with its Droplets.
Deployment Steps on Heroku:
- Install the Heroku CLI.
- Log in to your Heroku account.
- Create a new Heroku application.
- Push your code using Git.
These steps provide a solid foundation for creating and deploying a website using Python, enabling you to build a variety of web applications tailored to your needs.
Choosing a Framework
Selecting the right framework is crucial for building a website in Python. Here are some popular frameworks, each suited for different project types:
- Flask: A micro-framework that is lightweight and easy to use. Ideal for small to medium-sized applications.
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. Best suited for larger applications requiring a robust structure.
- FastAPI: Excellent for building APIs with asynchronous capabilities, making it suitable for high-performance applications.
Setting Up Your Development Environment
To begin building your website, establish a proper development environment:
- Install Python: Ensure you have Python installed on your machine. Use the latest version for better features and compatibility.
- Create a Virtual Environment: This helps manage dependencies for your project separately from other projects. Use the following command:
“`bash
python -m venv myenv
“`
- Activate the Virtual Environment:
- On Windows:
“`bash
myenv\Scripts\activate
“`
- On macOS/Linux:
“`bash
source myenv/bin/activate
“`
Installing Necessary Packages
Depending on the framework chosen, install the required packages using `pip`. Here’s how to do it for Flask and Django:
- Flask:
“`bash
pip install Flask
“`
- Django:
“`bash
pip install Django
“`
Creating a Basic Application
The creation of a basic application varies based on the framework selected. Below are examples for Flask and Django.
Flask:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run(debug=True)
“`
Django:
“`bash
django-admin startproject myproject
cd myproject
python manage.py runserver
“`
Visit `http://127.0.0.1:8000/` in your browser to see the default welcome page.
Routing and Views
Routing defines how URLs are mapped to views in your application.
Flask Routing Example:
“`python
@app.route(‘/about’)
def about():
return ‘This is the About page.’
“`
Django URL Configuration:
In `myproject/urls.py`, add:
“`python
from django.urls import path
from . import views
urlpatterns = [
path(‘about/’, views.about, name=’about’),
]
“`
Templates and Static Files
Integrate HTML templates and static files (CSS, JavaScript) for a polished user interface.
Flask:
- Create a `templates` directory and add HTML files.
- Use the `render_template` function to render them.
Django:
- Create a `templates` directory in your app folder.
- Reference templates in views using `render`.
Static Files:
Both frameworks handle static files differently, requiring specific directories and configurations.
Database Integration
Web applications often require data persistence through databases.
- Flask: Use SQLAlchemy or Flask-SQLAlchemy for ORM support.
- Django: Comes with built-in ORM. Define models in `models.py` and run migrations.
Example for Django Model:
“`python
from django.db import models
class Post(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
“`
Deployment
Once your website is ready, deployment options include:
- Heroku: A platform as a service that supports both Flask and Django applications.
- AWS Elastic Beanstalk: Provides services for deploying applications in the cloud.
- DigitalOcean: A cloud infrastructure provider that offers simpler setup for small projects.
Each of these platforms has its own documentation for deployment, ensuring you can follow the steps specific to your project’s needs.
Expert Insights on Building a Website with Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When creating a website using Python, it is essential to choose the right framework. Django is an excellent choice for building robust applications quickly, while Flask offers more flexibility for smaller projects. Understanding the strengths of each framework can significantly impact your development process.”
Michael Thompson (Web Development Instructor, Code Academy). “Learning how to make a website in Python involves grasping not only the language itself but also web technologies like HTML, CSS, and JavaScript. Integrating these technologies with Python will enhance your website’s functionality and user experience.”
Sarah Patel (Lead Developer, Digital Solutions Corp). “Security should be a priority when developing a website with Python. Implementing best practices such as using HTTPS, validating user inputs, and regularly updating dependencies can help protect your site from vulnerabilities and attacks.”
Frequently Asked Questions (FAQs)
How do I start making a website using Python?
To start making a website using Python, you should choose a web framework such as Flask or Django. Install the framework using pip, set up a project structure, and create your application by defining routes, views, and templates.
What are the best Python frameworks for web development?
The best Python frameworks for web development include Django, Flask, FastAPI, and Pyramid. Django is feature-rich and suitable for larger applications, while Flask is lightweight and ideal for smaller projects.
Do I need to know HTML and CSS to create a website with Python?
Yes, knowledge of HTML and CSS is essential when creating a website with Python. These technologies are used for structuring and styling the web pages, while Python handles the backend logic.
Can I host a Python-based website for free?
Yes, you can host a Python-based website for free using platforms like Heroku, PythonAnywhere, or Replit. These services offer free tiers that allow you to deploy small applications without cost.
How do I connect a database to my Python web application?
To connect a database to your Python web application, you can use an Object-Relational Mapping (ORM) tool like SQLAlchemy or Django’s built-in ORM. Configure the database connection in your application settings and use the ORM to interact with the database.
What are some common challenges when building a website with Python?
Common challenges include managing dependencies, ensuring security, handling user authentication, and optimizing performance. Proper planning and using established frameworks can help mitigate these issues.
Creating a website using Python involves several key steps, including selecting the appropriate web framework, setting up the development environment, and deploying the site. Popular frameworks such as Flask and Django offer robust features that cater to different project needs. Flask is ideal for smaller applications due to its simplicity and flexibility, while Django is suited for larger, more complex projects with its built-in functionalities and admin interface.
In addition to choosing a framework, it is essential to understand the basics of HTML, CSS, and JavaScript, as these technologies are integral to web development. Python handles the backend logic, while the frontend is typically constructed using these web technologies. Furthermore, incorporating a database, such as SQLite or PostgreSQL, is crucial for managing data effectively, allowing for dynamic content and user interaction.
Deployment is another critical aspect of building a website with Python. Various platforms, such as Heroku, AWS, and DigitalOcean, provide hosting services that can help you launch your site. Additionally, utilizing version control systems like Git ensures that your code is well-managed and collaborative, facilitating smoother development processes.
In summary, building a website with Python requires a combination of selecting the right framework, understanding web technologies, and effectively deploying your application. By
Author Profile
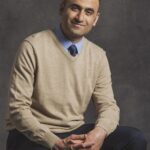
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?