How Can You Create a Directed Graph in Python?
### Introduction
In the world of data visualization and analysis, directed graphs stand out as powerful tools for representing relationships and flows between entities. Whether you’re mapping out complex networks, analyzing social interactions, or visualizing data structures, understanding how to create directed graphs in Python can significantly enhance your ability to convey information effectively. With a plethora of libraries at your disposal, the journey into graph creation is not only accessible but also exciting, unlocking new avenues for data storytelling and insight extraction.
Directed graphs, or digraphs, are a type of graph where the edges have a direction, indicating a one-way relationship between nodes. This characteristic makes them particularly useful in various applications, from web page link structures to dependency graphs in software development. Python, with its rich ecosystem of libraries such as NetworkX and Matplotlib, provides robust tools for constructing and visualizing these graphs, making it easier than ever to translate abstract concepts into tangible representations.
As you delve into the process of creating directed graphs in Python, you’ll discover the flexibility and power these visualizations offer. From defining nodes and edges to customizing layouts and styles, the possibilities are vast. By mastering these techniques, you can elevate your projects, whether they involve academic research, data analysis, or software engineering, and communicate your findings with clarity and impact
Using NetworkX for Directed Graphs
To create directed graphs in Python, one of the most popular libraries is NetworkX. This library provides comprehensive tools for the creation, manipulation, and analysis of complex networks. Here’s how to get started with directed graphs using NetworkX.
First, ensure you have NetworkX installed in your Python environment. You can install it using pip:
bash
pip install networkx
Once installed, you can begin creating directed graphs by importing the library and utilizing its `DiGraph` class. Here’s a simple example:
python
import networkx as nx
import matplotlib.pyplot as plt
# Create a directed graph
G = nx.DiGraph()
# Add nodes
G.add_node(1)
G.add_node(2)
G.add_node(3)
# Add directed edges
G.add_edge(1, 2)
G.add_edge(2, 3)
G.add_edge(3, 1)
# Draw the graph
nx.draw(G, with_labels=True, node_color=’lightblue’, arrows=True)
plt.show()
In this example, we create a directed graph with three nodes and three directed edges. The `nx.draw()` function visualizes the graph.
Adding Attributes to Nodes and Edges
NetworkX allows you to add attributes to nodes and edges, which can enhance the information contained within the graph. Attributes can include weights, labels, or any other relevant metadata.
To add attributes, you can modify the nodes and edges as follows:
python
# Add nodes with attributes
G.add_node(1, label=’A’, value=5)
G.add_node(2, label=’B’, value=3)
# Add edges with attributes
G.add_edge(1, 2, weight=4)
G.add_edge(2, 3, weight=2)
You can retrieve these attributes using:
python
node_label = G.nodes[1][‘label’]
edge_weight = G.edges[1, 2][‘weight’]
Analyzing Directed Graphs
NetworkX provides various algorithms for analyzing directed graphs. Here are a few common metrics you can compute:
- In-degree: The number of incoming edges to a node.
- Out-degree: The number of outgoing edges from a node.
- Shortest path: The shortest path between two nodes.
You can calculate these metrics as follows:
python
in_degree = G.in_degree(1)
out_degree = G.out_degree(1)
shortest_path = nx.shortest_path(G, source=1, target=3)
Table of Common Methods in NetworkX
Method | Description |
---|---|
add_node(n) | Add a node n to the graph. |
add_edge(u, v) | Add a directed edge from node u to node v. |
in_degree(n) | Return the in-degree of node n. |
out_degree(n) | Return the out-degree of node n. |
shortest_path(G, source, target) | Find the shortest path from source to target. |
By leveraging NetworkX, you can efficiently create, manipulate, and analyze directed graphs, making it an invaluable tool for graph theory applications in Python.
Using NetworkX to Create Directed Graphs
NetworkX is a popular Python library for the creation, manipulation, and study of complex networks. It provides a straightforward approach to creating directed graphs.
To install NetworkX, use the following pip command:
bash
pip install networkx
### Basic Operations with Directed Graphs
To create a directed graph using NetworkX, follow these steps:
- Import the library:
python
import networkx as nx
- Create a directed graph:
python
G = nx.DiGraph()
- Add nodes:
Nodes can be added individually or as a list. For example:
python
G.add_node(1)
G.add_nodes_from([2, 3, 4])
- Add directed edges:
Edges can be added between nodes to indicate direction:
python
G.add_edge(1, 2)
G.add_edges_from([(2, 3), (3, 1)])
### Visualizing Directed Graphs
Visualization is crucial for understanding graph structures. NetworkX works well with Matplotlib for this purpose.
- Install Matplotlib:
bash
pip install matplotlib
- Draw the graph:
python
import matplotlib.pyplot as plt
nx.draw(G, with_labels=True)
plt.show()
### Example: Creating and Visualizing a Directed Graph
The following code snippet demonstrates the complete process of creating and visualizing a directed graph.
python
import networkx as nx
import matplotlib.pyplot as plt
# Create directed graph
G = nx.DiGraph()
# Add nodes
G.add_nodes_from([1, 2, 3, 4, 5])
# Add directed edges
G.add_edges_from([(1, 2), (1, 3), (2, 4), (3, 5), (4, 5)])
# Draw the graph
nx.draw(G, with_labels=True, node_color=’lightblue’, arrows=True)
plt.show()
### Additional Features of NetworkX
NetworkX provides numerous features for analyzing and manipulating directed graphs, including:
- Graph algorithms: Shortest path, connectivity, and clustering.
- Graph statistics: Degree distribution, centrality measures, and more.
- Graph transformations: Subgraph extraction and graph merging.
### Performance Considerations
When working with large directed graphs, consider the following:
- Efficiency: Use sparse representations if the graph is large but has few edges relative to the number of nodes.
- Memory usage: Keep track of the number of nodes and edges to ensure that memory constraints are respected.
### Summary of NetworkX Functions
Function | Description |
---|---|
`add_node(node)` | Adds a single node to the graph |
`add_nodes_from(nodes)` | Adds multiple nodes at once |
`add_edge(u, v)` | Adds a directed edge from node u to v |
`add_edges_from(edges)` | Adds multiple directed edges |
`draw()` | Visualizes the graph |
By utilizing these features, you can effectively create and manipulate directed graphs in Python using NetworkX.
Expert Insights on Creating Directed Graphs in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Creating directed graphs in Python can be efficiently achieved using libraries such as NetworkX. This library provides a robust framework for graph creation, manipulation, and analysis, making it ideal for both academic and industrial applications.”
Michael Thompson (Software Engineer, GraphTech Solutions). “For those looking to visualize directed graphs, I recommend using Matplotlib in conjunction with NetworkX. This combination allows for clear and informative representations of complex relationships, which is crucial in data analysis.”
Dr. Sarah Patel (Professor of Computer Science, University of Data Science). “When constructing directed graphs, it is essential to understand the underlying data structure. Python’s adjacency list or adjacency matrix implementations can greatly influence the performance of graph algorithms, so choose wisely based on your specific needs.”
Frequently Asked Questions (FAQs)
How do I create a directed graph in Python?
You can create a directed graph in Python using libraries such as NetworkX. First, install the library using `pip install networkx`, then use `nx.DiGraph()` to create a directed graph object. Add nodes and edges using the `add_node()` and `add_edge()` methods.
What is the difference between a directed graph and an undirected graph?
A directed graph consists of edges that have a direction, indicating a one-way relationship between nodes. In contrast, an undirected graph has edges that represent a two-way relationship, meaning the connection is mutual.
Can I visualize a directed graph in Python?
Yes, you can visualize a directed graph using libraries like Matplotlib in conjunction with NetworkX. After creating your directed graph, use `nx.draw()` or `nx.draw_networkx()` to render the graph visually.
What are some common applications of directed graphs?
Directed graphs are commonly used in various applications, including representing web page links, task scheduling, social network analysis, and modeling relationships in databases.
Are there any performance considerations when working with large directed graphs in Python?
Yes, performance can be affected by the size of the graph. For large directed graphs, consider using efficient data structures, optimizing algorithms, and leveraging libraries designed for scalability, such as Graph-tool or igraph.
How can I save and load a directed graph in Python?
You can save a directed graph using NetworkX’s built-in functions like `nx.write_gpickle()` for binary format or `nx.write_graphml()` for XML format. To load the graph, use `nx.read_gpickle()` or `nx.read_graphml()`, respectively.
Creating directed graphs in Python can be accomplished using various libraries, with NetworkX being one of the most popular and versatile options. This library provides a comprehensive set of tools for the creation, manipulation, and analysis of complex networks. Users can easily create directed graphs by utilizing the `DiGraph` class, which allows for the representation of edges that have a direction, thus enabling the modeling of relationships where directionality is significant.
In addition to NetworkX, other libraries such as Matplotlib for visualization and Graph-tool for performance optimization can be integrated to enhance the functionality of directed graph representations. By combining these tools, users can not only create graphs but also visualize them effectively, making it easier to interpret the relationships and structures within the data. Furthermore, the ability to analyze properties such as connectivity, paths, and centrality metrics adds significant value to the study of directed graphs.
Overall, the process of making directed graphs in Python is straightforward, thanks to the rich ecosystem of libraries available. Users are encouraged to explore the documentation and examples provided by these libraries to gain a deeper understanding of their capabilities. This exploration will enable them to leverage directed graphs effectively in their projects, whether for academic research, data analysis, or software development.
Author Profile
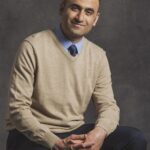
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?