How Can You Create a Hangman Game Using Python?
Have you ever wanted to create your own version of the classic word-guessing game, Hangman, using Python? This timeless game not only sparks nostalgia but also serves as a fantastic project for both beginner and experienced programmers. By building Hangman from scratch, you can enhance your coding skills, learn about string manipulation, and dive into the world of user input and control flow. Whether you’re looking to impress friends with your programming prowess or simply want to challenge yourself with a fun coding exercise, this guide will walk you through the essential steps to bring this iconic game to life.
Creating Hangman in Python is a rewarding endeavor that combines creativity with technical skill. At its core, the game involves selecting a word, allowing players to guess letters, and tracking their progress while managing a limited number of incorrect attempts. As you embark on this project, you’ll explore key programming concepts such as loops, conditionals, and functions, all while crafting an engaging user experience. The beauty of Hangman lies in its simplicity, making it an ideal starting point for those eager to learn the fundamentals of Python programming.
In this article, we will break down the process of developing your very own Hangman game, from setting up the game environment to implementing the game logic. You’ll gain insights
Setting Up the Game
To create a Hangman game in Python, you will need to set up a few essential components. Begin by importing the necessary libraries and defining your main variables.
“`python
import random
List of words to choose from
words = [‘python’, ‘hangman’, ‘programming’, ‘challenge’, ‘development’]
chosen_word = random.choice(words)
guessed_letters = []
attempts = 6
“`
In this setup, you have a list of potential words. The `random.choice()` function selects one word from the list for the player to guess. The `guessed_letters` list tracks the letters that have been guessed, while the `attempts` variable defines how many incorrect guesses are allowed.
Displaying the Game State
The next step is to display the current state of the game to the player. This includes showing the correctly guessed letters and masking the others.
“`python
def display_word(chosen_word, guessed_letters):
displayed_word = ”
for letter in chosen_word:
if letter in guessed_letters:
displayed_word += letter + ‘ ‘
else:
displayed_word += ‘_ ‘
return displayed_word.strip()
“`
This function constructs a string that represents the current state of the guessed word. It replaces unguessed letters with underscores, while correctly guessed letters are shown.
Game Loop
The main game loop handles user input and checks for win/lose conditions. Players will continue guessing until they either reveal the word or run out of attempts.
“`python
while attempts > 0:
print(display_word(chosen_word, guessed_letters))
print(f’Attempts left: {attempts}’)
guess = input(‘Guess a letter: ‘).lower()
if guess in guessed_letters:
print(‘You already guessed that letter.’)
elif guess in chosen_word:
guessed_letters.append(guess)
print(‘Good guess!’)
else:
guessed_letters.append(guess)
attempts -= 1
print(‘Wrong guess.’)
if all(letter in guessed_letters for letter in chosen_word):
print(f’Congratulations! You guessed the word: {chosen_word}’)
break
else:
print(f’Sorry, you lost! The word was: {chosen_word}’)
“`
In this loop, the game checks if the player has already guessed a letter and updates the number of attempts if the guess is incorrect. The game continues until the word is fully guessed or the player runs out of attempts.
Enhancements and Features
To improve the Hangman game experience, consider adding the following features:
- Input Validation: Ensure the user inputs only a single letter and handle invalid inputs gracefully.
- Score Tracking: Implement a scoring system based on the number of attempts remaining.
- Replay Option: Allow players to restart the game without having to rerun the program.
Here’s a simple table summarizing the features:
Feature | Description |
---|---|
Input Validation | Check for valid single-letter guesses. |
Score Tracking | Keep track of scores based on attempts left. |
Replay Option | Enable restarting the game after a session. |
These enhancements can make your Hangman game more engaging and user-friendly. By implementing these features, you create a more polished and complete gaming experience for users.
Setting Up the Game
To create a Hangman game in Python, start by setting up your environment. You will need to have Python installed on your machine. Any version from Python 3.x will work effectively. Open a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode to begin coding.
Basic Game Structure
The basic structure of the Hangman game consists of the following components:
- Word Selection: The game must choose a word from a predefined list.
- User Input: Users will guess letters.
- Game Logic: The game must track correct and incorrect guesses.
- Display: The game must show the current state of the word and number of attempts left.
Code Implementation
Here is a simple implementation of the Hangman game in Python:
“`python
import random
def choose_word():
words = [‘python’, ‘hangman’, ‘challenge’, ‘programming’, ‘development’]
return random.choice(words)
def display_board(word, guessed_letters):
display = ”
for letter in word:
if letter in guessed_letters:
display += letter + ‘ ‘
else:
display += ‘_ ‘
return display.strip()
def hangman():
word = choose_word()
guessed_letters = set()
attempts = 6
print(“Welcome to Hangman!”)
while attempts > 0:
print(“\nCurrent word: “, display_board(word, guessed_letters))
print(f”Attempts left: {attempts}”)
guess = input(“Guess a letter: “).lower()
if guess in guessed_letters:
print(“You’ve already guessed that letter.”)
continue
guessed_letters.add(guess)
if guess in word:
print(“Good guess!”)
else:
attempts -= 1
print(“Wrong guess!”)
if all(letter in guessed_letters for letter in word):
print(f”Congratulations! You’ve guessed the word: {word}”)
break
else:
print(f”You’ve run out of attempts! The word was: {word}”)
if __name__ == “__main__”:
hangman()
“`
Game Features
Consider adding the following features to enhance the game:
- Input Validation: Ensure the user enters a single alphabetic character.
- Score Tracking: Keep track of wins and losses.
- Replay Option: Allow users to play multiple rounds without restarting the program.
- Hints: Provide hints for difficult words.
Enhancements with Functions
Breaking down the game into functions promotes better structure and readability. Here’s how to refactor the game:
Function | Purpose |
---|---|
`choose_word()` | Selects a random word from the list. |
`display_board()` | Generates a string representation of the word. |
`hangman()` | Contains the main game loop and logic. |
`get_user_input()` | Handles user input and validates it. |
Each function can be expanded or modified independently, allowing for easy updates and enhancements.
Testing the Game
Ensure to test the game thoroughly:
- Test with different words to check if the game behaves correctly.
- Check the input validation by entering invalid inputs.
- Test the replay functionality if added.
Adjust the game based on user feedback to improve the overall experience.
Expert Insights on Creating Hangman in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When developing a Hangman game in Python, it is crucial to utilize lists and strings effectively. Implementing functions for word selection, player input, and game state management will enhance the modularity and readability of your code.”
James Liu (Python Programming Instructor, Tech Academy). “To create an engaging Hangman game, consider implementing features like difficulty levels and a graphical interface using libraries such as Tkinter. This not only improves user experience but also allows for deeper learning of Python’s capabilities.”
Sarah Thompson (Game Developer, Indie Game Studio). “Focus on the user experience when coding your Hangman game. Ensure that the prompts are clear and that the feedback on player guesses is immediate. This will keep players engaged and make the game more enjoyable.”
Frequently Asked Questions (FAQs)
What are the basic requirements to create a Hangman game in Python?
To create a Hangman game in Python, you need a basic understanding of Python syntax, control structures (like loops and conditionals), and data structures (such as lists and dictionaries). Familiarity with functions will also be beneficial for organizing your code.
How do I choose a word for the Hangman game?
You can choose a word from a predefined list or use an external file containing a collection of words. Alternatively, you can implement a function that randomly selects a word from a list to enhance the game’s unpredictability.
What libraries or modules can be used to enhance the Hangman game?
While you can create a Hangman game using only built-in Python features, libraries like `random` for word selection and `time` for adding delays can enhance the game’s functionality. Additionally, using `pygame` can help create a graphical version of the game.
How can I implement user input for guessing letters?
You can use the `input()` function to capture user guesses. Ensure to validate the input by checking if the entered character is a single letter and has not been guessed before to maintain the game’s integrity.
What strategies can I use to display the current state of the game?
You can represent the word as a list of underscores, updating it with correctly guessed letters. Display the current state by joining the list into a string and showing it alongside the number of incorrect guesses to inform the player of their progress.
How can I manage the game’s win/lose conditions?
You can check for win conditions by verifying if all letters in the word have been guessed correctly. For lose conditions, compare the number of incorrect guesses to a predefined limit. Implement appropriate messages to inform the player of the game’s outcome.
Creating a Hangman game in Python involves several fundamental programming concepts, including the use of loops, conditionals, and data structures such as lists and strings. The game typically starts by selecting a random word from a predefined list, which the player must guess letter by letter. As the player makes guesses, the program keeps track of correct and incorrect attempts, updating the display of the word and the number of remaining tries accordingly.
To implement the game effectively, developers should focus on structuring the code to handle user input and provide feedback. This includes validating guesses, displaying the current state of the word with correctly guessed letters, and managing a simple user interface that can run in the console. Additionally, using functions to modularize the code can enhance readability and maintainability, making it easier to debug and expand the game in the future.
Key takeaways from developing a Hangman game in Python include the importance of user interaction and error handling. Ensuring that the game responds appropriately to invalid inputs is crucial for a smooth user experience. Furthermore, incorporating features such as a scoring system or the ability to play multiple rounds can enhance the game’s replayability and engagement. Overall, building a Hangman game serves as an excellent exercise for honing programming skills while
Author Profile
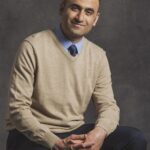
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?