How Can You Create a Simple Password Generator Using Python?
In an increasingly digital world, the importance of strong, unique passwords cannot be overstated. With cyber threats lurking around every corner, creating secure passwords is a fundamental step in protecting personal information and online accounts. But with the multitude of accounts we manage, remembering complex passwords can be a daunting task. This is where a simple password generator comes into play, offering a practical solution to enhance your online security effortlessly. In this article, we will explore how to create a straightforward password generator using Python, a versatile programming language that is beginner-friendly yet powerful enough to handle this task with ease.
Building a simple password generator in Python not only serves as a useful tool for enhancing your security but also provides a fantastic opportunity to sharpen your programming skills. By leveraging Python’s built-in libraries, you can create a program that generates random passwords of varying lengths and complexities, tailored to your specific needs. Whether you want to include uppercase letters, numbers, or special characters, a password generator can help ensure that your passwords are both strong and unpredictable.
As we delve deeper into the process of creating your own password generator, you’ll discover the fundamental concepts of string manipulation, randomization, and user input handling. This project is not just about coding; it’s about understanding the principles of security and the importance of safeguarding your
Understanding Password Components
A password generator requires a clear understanding of the components that will make up the passwords. Typically, effective passwords include a combination of the following:
- Uppercase Letters (A-Z)
- Lowercase Letters (a-z)
- Numbers (0-9)
- Special Characters (e.g., !@#$%^&*)
The inclusion of diverse character types increases the complexity of the password, making it harder to crack. A well-rounded password generator will allow for customization of these components based on user preferences.
Implementing the Password Generator in Python
To create a simple password generator in Python, we can utilize the `random` and `string` libraries. The following code snippet demonstrates a basic implementation:
python
import random
import string
def generate_password(length=12, use_uppercase=True, use_numbers=True, use_special=True):
characters = string.ascii_lowercase
if use_uppercase:
characters += string.ascii_uppercase
if use_numbers:
characters += string.digits
if use_special:
characters += string.punctuation
password = ”.join(random.choice(characters) for _ in range(length))
return password
This function allows users to specify the desired length of the password and whether to include uppercase letters, numbers, and special characters.
Customizing Password Generation
When creating a password generator, customization is key. Users can define their password requirements, including:
- Length: The total number of characters in the password.
- Character Types: Options to include or exclude specific character types.
Here’s a table summarizing the options available for customizing the password generator:
Option | Description |
---|---|
Length | Specify the total length of the generated password. |
Uppercase | Include uppercase letters (A-Z). |
Numbers | Include digits (0-9). |
Special Characters | Include symbols (e.g., !@#$%^&*). |
Example Usage
Here’s how you might call the `generate_password` function in practice:
python
# Generate a 16-character password with all options enabled
print(generate_password(length=16, use_uppercase=True, use_numbers=True, use_special=True))
This example generates a password that is 16 characters long, incorporating uppercase letters, numbers, and special characters, thus ensuring a high level of security.
Testing and Enhancements
After implementing the basic generator, it is essential to test its functionality. Consider the following enhancements:
- Input Validation: Ensure that the user inputs valid parameters (e.g., length must be a positive integer).
- User Interface: Develop a simple command-line or graphical interface for easier interaction.
- Entropy Measurement: Include a method to measure password strength based on entropy, providing users with feedback on their generated passwords.
By considering these enhancements, the password generator can evolve into a more robust tool, providing users with not only secure passwords but also a better user experience.
Understanding Password Requirements
When creating a password generator, it is essential to define the criteria for the passwords you wish to generate. Common requirements include:
- Minimum and maximum length
- Inclusion of uppercase letters
- Inclusion of lowercase letters
- Inclusion of digits
- Inclusion of special characters (e.g., @, #, $, %)
Establishing these guidelines will help ensure that the generated passwords are secure and meet specific use case needs.
Implementation of a Simple Password Generator
Below is a simple implementation of a password generator in Python. This code allows customization based on the user-defined criteria.
python
import random
import string
def generate_password(length=12, use_uppercase=True, use_digits=True, use_special=True):
characters = string.ascii_lowercase # Start with lowercase letters
if use_uppercase:
characters += string.ascii_uppercase
if use_digits:
characters += string.digits
if use_special:
characters += string.punctuation
password = ”.join(random.choice(characters) for _ in range(length))
return password
# Example usage
print(generate_password(length=16))
Code Explanation
- Imports: The `random` and `string` modules are imported. The `random` module helps in selecting random characters, while the `string` module provides easy access to common character sets.
- Function Definition: The `generate_password` function accepts parameters for length and character types. Default values are provided to ensure usability without requiring user input.
- Character Set Construction:
- The base set starts with lowercase letters.
- Conditional checks append uppercase letters, digits, and special characters based on user preferences.
- Password Generation: The password is generated using a list comprehension that selects random characters from the constructed set.
Customization Options
To enhance usability, consider adding the following features:
- User Input for Length: Prompt the user for desired password length.
- Toggle Character Types: Allow users to choose which character types to include.
- Strength Indicator: Implement a function to evaluate password strength based on criteria met.
Example of Enhancing Functionality
Here’s a modified version that includes user input and strength evaluation:
python
def input_password_options():
length = int(input(“Enter password length (8-20): “))
use_uppercase = input(“Include uppercase letters? (y/n): “).lower() == ‘y’
use_digits = input(“Include digits? (y/n): “).lower() == ‘y’
use_special = input(“Include special characters? (y/n): “).lower() == ‘y’
return length, use_uppercase, use_digits, use_special
length, use_uppercase, use_digits, use_special = input_password_options()
print(generate_password(length, use_uppercase, use_digits, use_special))
This code snippet enhances interactivity and allows users to tailor the password generation process to their needs.
Testing and Validation
After implementation, it is crucial to test the generator thoroughly. Consider the following test cases:
Test Case | Expected Outcome |
---|---|
Generate a 12-character password | A password of length 12 with valid chars |
Generate password without digits | A password containing no digits |
Generate password with all types | A password containing all character types |
Testing will ensure that the password generator functions correctly and meets the specified criteria.
Expert Insights on Creating a Simple Password Generator in Python
Dr. Emily Carter (Cybersecurity Analyst, SecureTech Solutions). “When developing a simple password generator in Python, it is crucial to incorporate a mix of uppercase letters, lowercase letters, numbers, and special characters to enhance security. Utilizing the ‘random’ module effectively can help achieve this balance while ensuring the generated passwords are both complex and user-friendly.”
James Liu (Software Engineer, CodeSecure Inc.). “A straightforward approach to creating a password generator in Python involves leveraging built-in libraries such as ‘string’ and ‘random’. By defining a function that randomly selects characters from a predefined set, developers can easily customize the length and complexity of the passwords to meet specific requirements.”
Sarah Thompson (Python Developer, Tech Innovations). “It’s essential to consider user experience when designing a password generator. Implementing features such as user-defined length and the option to include or exclude certain character types can make the tool more versatile. Additionally, ensuring that the generated passwords are displayed in a clear and accessible manner will enhance usability.”
Frequently Asked Questions (FAQs)
What is a simple password generator in Python?
A simple password generator in Python is a program that creates random passwords based on specified criteria, such as length and character types, to enhance security.
How can I create a basic password generator using Python?
You can create a basic password generator by utilizing the `random` and `string` libraries to select characters randomly from a defined set, such as letters, digits, and symbols.
What libraries are commonly used for making a password generator in Python?
The most commonly used libraries for creating a password generator in Python are `random` for random selection and `string` for accessing predefined character sets like letters and digits.
Can I customize the length and complexity of the passwords generated?
Yes, you can customize the length and complexity of the passwords by adjusting parameters in your code, allowing you to specify the total length and which character types to include.
Is it secure to use a simple password generator for sensitive applications?
While a simple password generator can create random passwords, it is advisable to use more robust methods and libraries, such as `secrets`, for generating passwords intended for sensitive applications to ensure higher security.
How do I run a Python script for a password generator?
To run a Python script for a password generator, save the script in a `.py` file and execute it using a Python interpreter in your command line or terminal by typing `python filename.py`.
In summary, creating a simple password generator in Python involves utilizing the built-in libraries to generate random characters. By leveraging the `random` module, developers can easily create passwords that include a mix of uppercase letters, lowercase letters, numbers, and special characters. This approach ensures that the generated passwords are not only secure but also customizable based on user preferences for length and character types.
Moreover, implementing a password generator can be achieved through a straightforward function that accepts parameters such as password length and character set. This modular approach allows for flexibility and scalability, enabling users to adapt the generator to their specific security needs. Additionally, the use of lists and string manipulation enhances the efficiency of the code, making it accessible even for beginners in Python programming.
Ultimately, the key takeaway is that a simple password generator is an essential tool for enhancing security in various applications. By following best practices in coding and utilizing Python’s capabilities, developers can create effective solutions that protect user data. This not only fosters a sense of security but also promotes good habits in password management among users.
Author Profile
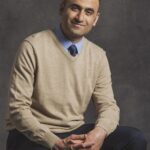
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?