How Can You Create a Snippet That Effectively Targets a Specific Class?
In the ever-evolving landscape of web development and design, the ability to create targeted snippets that enhance user experience and engagement is a crucial skill. Whether you’re a seasoned developer or just starting your journey, understanding how to make snippets that target a specific class can elevate your projects to new heights. These snippets not only streamline your workflow but also allow for greater customization and functionality within your applications. In this article, we will explore the essentials of crafting effective snippets tailored to specific classes, empowering you to optimize your coding practices and improve your website’s performance.
Creating snippets that target a class involves a blend of creativity and technical know-how. At its core, this process allows developers to write reusable pieces of code that can be applied to multiple elements sharing the same class, ensuring consistency and efficiency across your project. By leveraging the power of CSS, JavaScript, or even templating languages, you can manipulate the behavior and appearance of elements dynamically, enhancing both aesthetics and functionality.
As we delve deeper into this topic, we will uncover various techniques and best practices for creating these targeted snippets. From understanding the structure of classes in your code to implementing effective strategies for reusability, you will gain insights that will not only simplify your coding process but also enrich the user experience on your
Understanding the Basics of Snippets
To create a snippet that targets a specific class, it is essential to understand what a snippet is. In web development, a snippet typically refers to a small portion of reusable code or content that can be integrated into a larger system. Snippets can be used to enhance functionality, improve user experience, or optimize search engine results.
When targeting a specific class, you should consider the following aspects:
- Class Definition: The class in question must be defined in your HTML structure.
- CSS Selectors: Utilize appropriate CSS selectors to ensure your snippet interacts correctly with the targeted class.
- JavaScript Interaction: If your snippet requires dynamic behavior, you may need JavaScript to manipulate the elements associated with the targeted class.
Creating a Snippet for a Class
To create an effective snippet that targets a specific class, follow these steps:
- Identify the Target Class: Locate the class you wish to target within your HTML document. For example, consider a class named `.example-class`.
- Write the Snippet:
- For CSS: Define styles for the class.
- For JavaScript: Use the class in your functions.
Here is an example of how to create a simple CSS and JavaScript snippet that targets a class named `.example-class`.
“`html
“`
This code snippet applies a background color and text color to all elements with the class `.example-class` and adds an event listener that triggers an alert when the element is clicked.
Testing Your Snippet
After writing your snippet, it’s crucial to test it to ensure it behaves as expected. Consider the following testing strategies:
- Browser Developer Tools: Use tools like Chrome DevTools to inspect elements and test CSS/JavaScript snippets in real-time.
- Unit Testing: Implement unit tests to validate that your JavaScript functions behave correctly when interacting with the targeted class.
Testing Method | Description |
---|---|
Console Logging | Log outputs to the console to verify that the correct elements are selected. |
Visual Inspection | Manually check the webpage to see if styles are applied correctly. |
Automated Tests | Use testing frameworks like Jest to automate the testing of your snippets. |
By adhering to these guidelines and testing methods, you can create effective snippets that target specific classes, enhancing both functionality and user engagement on your website.
Understanding Snippets Targeting a Class
Creating a snippet that targets a specific class involves understanding both the structure of your HTML and the context in which the snippet will function. The goal is to extract or manipulate data associated with a particular class in your web application.
Step-by-Step Guide to Create a Snippet
- **Identify the Class**: Locate the HTML class you want to target. Inspect the element using developer tools in your browser to find its class name.
- **Choose the Right Language**: Depending on your environment (e.g., JavaScript, PHP, Python), select the appropriate method for creating your snippet.
- **Write the Code Snippet**:
- For **JavaScript**:
“`javascript
const elements = document.querySelectorAll(‘.your-class-name’);
elements.forEach(element => {
// Perform your desired action
console.log(element.textContent);
});
“`
- For **PHP**:
“`php
$dom = new DOMDocument();
@$dom->loadHTML($htmlContent); // Load your HTML content
$xpath = new DOMXPath($dom);
$elements = $xpath->query(‘//*[@class=”your-class-name”]’);
foreach ($elements as $element) {
// Perform your desired action
echo $element->nodeValue;
}
“`
- For Python (using Beautiful Soup):
“`python
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, ‘html.parser’)
elements = soup.find_all(class_=’your-class-name’)
for element in elements:
Perform your desired action
print(element.get_text())
“`
Common Use Cases for Class Targeting
- Data Extraction: Scraping specific pieces of information from a webpage.
- DOM Manipulation: Changing styles or content dynamically.
- Event Handling: Adding event listeners to elements with a specific class.
Best Practices for Targeting Classes
- Use Specific Class Names: Avoid overly generic class names to prevent unintended selections.
- Limit Scope: If possible, target a parent element to narrow the search scope and improve performance.
- Test Thoroughly: Ensure your snippet works across different browsers and devices.
Considerations for Performance and Efficiency
Factor | Considerations |
---|---|
Query Performance | Use specific selectors to reduce processing time. |
Memory Management | Clean up variables and references when no longer needed. |
Browser Compatibility | Test across various browsers to ensure consistency. |
Debugging Snippets Targeting a Class
- Console Logging: Use `console.log()` to debug and verify that the correct elements are being targeted.
- Browser Developer Tools: Utilize the Elements tab to inspect live changes and track potential issues.
- Error Handling: Implement try-catch blocks in languages that support them to gracefully handle exceptions.
By following these guidelines, you can create effective snippets that target specific classes, enhancing your web development projects or data extraction tasks.
Expert Strategies for Creating Targeted Snippets in Web Development
Dr. Emily Carter (Web Development Consultant, Tech Innovations Inc.). “To effectively create a snippet that targets a specific class, it is essential to utilize precise CSS selectors. This ensures that only the intended elements are affected, enhancing both functionality and user experience.”
Mark Thompson (SEO Specialist, Digital Growth Agency). “When crafting snippets, focus on the semantic structure of your HTML. By using appropriate tags and attributes, you can optimize your content for search engines, making it easier to target specific classes in your snippets.”
Linda Martinez (Front-End Developer, CodeCraft Solutions). “Incorporating JavaScript can greatly enhance your ability to create dynamic snippets that interact with specific classes. This approach allows for real-time updates and improved user engagement on your website.”
Frequently Asked Questions (FAQs)
What is a snippet in programming?
A snippet is a small region of reusable code or text that can be easily inserted into a larger codebase, often used to streamline coding tasks and improve efficiency.
How can I create a snippet that targets a specific class in my code?
To create a snippet targeting a specific class, define the snippet with the appropriate syntax for your programming environment, ensuring it includes the class selector and the desired properties or methods.
What tools can I use to manage code snippets targeting classes?
Popular tools for managing code snippets include Visual Studio Code, Sublime Text, and SnippetsLab. These tools offer features for organizing, searching, and inserting snippets efficiently.
Are there best practices for writing snippets that target classes?
Best practices include using clear and descriptive names for snippets, ensuring code is well-commented, and maintaining consistency in formatting and structure to enhance readability.
Can I share my snippets that target classes with others?
Yes, you can share snippets through version control systems like Git, or by exporting them from your code editor to share as files or in collaborative platforms.
How do I test a snippet that targets a class before using it in production?
To test a snippet, create a separate development environment or use unit tests to validate functionality and ensure it behaves as expected without introducing errors into the main codebase.
Creating a snippet that targets a specific class involves understanding the structure of your content and the desired outcome of the snippet. By identifying the relevant class within your HTML or code structure, you can effectively extract and display information that is pertinent to that class. This process typically includes utilizing programming languages such as JavaScript or Python, along with libraries or frameworks that facilitate DOM manipulation or data extraction.
Additionally, it is essential to consider the context in which the snippet will be used. Whether it is for enhancing user experience, improving SEO, or providing quick access to information, the snippet should be designed to fulfill its purpose efficiently. This means paying attention to the format, ensuring that it is visually appealing and easy to understand for the end-user.
Moreover, testing the snippet across different platforms and devices is crucial to ensure compatibility and functionality. By doing so, you can identify any potential issues and make necessary adjustments to optimize performance. Ultimately, a well-crafted snippet that targets a specific class can significantly enhance the overall effectiveness of your content and improve user engagement.
Author Profile
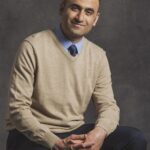
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?