How Can You Effectively Manage Events in Kubernetes Using a Dynamic Informer?
In the ever-evolving landscape of cloud-native applications, Kubernetes has emerged as a powerhouse for container orchestration. As organizations increasingly rely on this robust platform to manage their microservices, the need for efficient event handling becomes paramount. Enter the dynamic informer—a powerful tool in the Kubernetes ecosystem that allows developers and operators to listen for changes in resource states and respond accordingly. Whether you’re scaling applications, automating responses to changes, or simply monitoring the health of your clusters, mastering event management with a dynamic informer can significantly enhance your operational efficiency and responsiveness.
At its core, managing events in Kubernetes involves understanding how resources interact and change over time. Dynamic informers provide a way to watch these resources and receive notifications about their state changes, enabling you to react in real-time. By leveraging the informer’s capabilities, you can build applications that are not only aware of their environment but can also adapt to changes seamlessly. This is particularly useful in scenarios where rapid response is crucial, such as scaling workloads based on demand or implementing self-healing mechanisms in your applications.
Furthermore, the dynamic informer simplifies the complexity of event handling by abstracting the intricacies of the Kubernetes API. It allows developers to focus on building their applications rather than getting bogged down by the underlying mechanics of resource monitoring. As we delve
Understanding Dynamic Informers
Dynamic informers in Kubernetes are a powerful tool for monitoring and responding to changes in resources. They allow you to create an event-driven architecture that can efficiently handle updates to Kubernetes objects. This mechanism utilizes the Kubernetes API to watch for changes and react accordingly, enabling more responsive and adaptive applications.
Dynamic informers work by registering for updates on specific resource types, such as Pods, Services, or Deployments. When an event occurs, such as the creation or deletion of a resource, the informer sends notifications to registered handlers, allowing your application to respond to these changes in real time.
Setting Up Dynamic Informers
To set up a dynamic informer, you typically follow these steps:
- Create a Kubernetes Client: Use the official client-go library to create a client that can interact with the Kubernetes API.
- Define the Informer: Specify the resource type you want to watch and create an informer for it.
- Register Event Handlers: Attach event handlers to the informer to define how your application should respond to create, update, or delete events.
- Start the Informer: Begin the informer to start receiving events.
The following code snippet illustrates how to create a dynamic informer for Pods:
“`go
import (
“context”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/tools/cache”
“k8s.io/client-go/tools/clientcmd”
)
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfigPath)
if err != nil {
panic(err)
}
clientset, err := kubernetes.NewForConfig(config)
if err != nil {
panic(err)
}
informer := cache.NewSharedInformer(
cache.NewListWatchFromClient(clientset.CoreV1().RESTClient(), “pods”, “”, fields.Everything()),
&v1.Pod{},
0, // No resync period
)
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) { /* Handle addition */ },
UpdateFunc: func(oldObj, newObj interface{}) { /* Handle update */ },
DeleteFunc: func(obj interface{}) { /* Handle deletion */ },
})
stopCh := make(chan struct{})
defer close(stopCh)
informer.Run(stopCh)
“`
Event Handling
When an event occurs, the registered event handlers will be triggered. The `AddFunc`, `UpdateFunc`, and `DeleteFunc` are critical for defining how your application reacts.
- AddFunc: Executes when a new resource is added.
- UpdateFunc: Executes when an existing resource is updated.
- DeleteFunc: Executes when a resource is deleted.
Properly implementing these functions ensures that your application remains in sync with the state of the Kubernetes cluster.
Best Practices for Managing Events
To effectively manage events with dynamic informers, consider the following best practices:
- Batch Processing: When handling updates, consider batching events to minimize the number of operations on your application.
- Error Handling: Implement robust error handling in your event handlers to prevent application crashes.
- Resource Cleanup: Ensure that resources are properly released when they are no longer needed.
- Concurrency Control: Be mindful of concurrency when updating shared resources.
Performance Considerations
Dynamic informers can consume resources, especially in large clusters. Here are some performance considerations:
Aspect | Recommendation |
---|---|
Resync Period | Use a sensible resync period to reduce load on the API server. |
Event Filter | Apply filters to limit the scope of events to only those of interest. |
Resource Limits | Set appropriate resource limits for your pods to prevent resource exhaustion. |
By following these guidelines and utilizing dynamic informers effectively, you can build responsive and efficient applications that adapt seamlessly to changes within the Kubernetes environment.
Understanding Dynamic Informers in Kubernetes
Dynamic informers in Kubernetes provide a powerful way to interact with the Kubernetes API by allowing users to watch for changes in resources. They are particularly useful for managing events related to specific resources without needing to poll the API continuously.
Key Components of Dynamic Informers
- Informers: These are responsible for watching resources and keeping a local cache of the objects.
- Event Handlers: Functions that are triggered when an event occurs, such as an object being added, updated, or deleted.
- Shared Informer Factory: This provides a way to create and manage informers efficiently, reducing the number of connections to the API server.
Setting Up a Dynamic Informer
- Create a Shared Informer Factory: Use the client-go library to create a shared informer factory for a specific Kubernetes client.
“`go
factory := informers.NewSharedInformerFactory(clientset, time.Second*30)
“`
- Define the Informer: Choose the resource type you want to watch, such as Pods or Deployments.
“`go
podInformer := factory.Core().V1().Pods().Informer()
“`
- Set Up Event Handlers: Define handlers for add, update, and delete events.
“`go
podInformer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: func(obj interface{}) { /* Handle add event */ },
UpdateFunc: func(oldObj, newObj interface{}) { /* Handle update event */ },
DeleteFunc: func(obj interface{}) { /* Handle delete event */ },
})
“`
- Start the Informer: Begin watching for events.
“`go
factory.Start(stopCh)
“`
Best Practices for Managing Events
- Minimize API Calls: Use informers to reduce the number of direct API calls to the Kubernetes server.
- Error Handling: Implement robust error handling in your event handlers to avoid crashes.
- Resource Cleanup: Make sure to clean up resources and stop informers when they are no longer needed.
- Rate Limiting: Use rate limiting to manage the frequency of event handler execution, especially for high-frequency events.
Example Use Case: Monitoring Pod Events
To monitor Pod events, you would set up a dynamic informer as follows:
Step | Code Example |
---|---|
Create Factory | `factory := informers.NewSharedInformerFactory(clientset, time.Second*30)` |
Create Pod Informer | `podInformer := factory.Core().V1().Pods().Informer()` |
Add Handlers | `podInformer.AddEventHandler(…)` |
Start Informer | `factory.Start(stopCh)` |
Conclusion
Dynamic informers are essential for efficiently managing events in Kubernetes. By following the outlined setup and best practices, developers can effectively monitor and respond to changes in the Kubernetes environment, ensuring that applications remain responsive and resilient.
Strategies for Effective Event Management with Dynamic Informers in Kubernetes
Dr. Emily Tran (Kubernetes Specialist, Cloud Innovations Inc.). “To effectively manage events with a dynamic informer in Kubernetes, it is crucial to understand the lifecycle of the informer. Implementing a well-structured event handling mechanism allows for efficient resource utilization and minimizes latency in your applications.”
James Patel (DevOps Engineer, Tech Solutions Group). “Utilizing dynamic informers can significantly enhance event-driven architectures in Kubernetes. By leveraging the capabilities of informers to watch for changes in resources, teams can automate responses and streamline workflows, ultimately improving operational efficiency.”
Linda Chen (Cloud Native Architect, Future Technologies). “Integrating dynamic informers within your Kubernetes setup requires careful planning and testing. It is essential to monitor the performance of your informers and adjust their configurations based on the scale of your application to ensure they handle events effectively without overwhelming the system.”
Frequently Asked Questions (FAQs)
What is a dynamic informer in Kubernetes?
A dynamic informer in Kubernetes is a component that watches for changes in resources and provides notifications about those changes. It allows developers to manage and react to events for various Kubernetes objects dynamically.
How do I set up a dynamic informer in my Kubernetes application?
To set up a dynamic informer, you need to use the Kubernetes client-go library. You can create a dynamic client, specify the resource you want to watch, and then use the `NewSharedIndexInformer` method to start watching the resource.
What are the benefits of using a dynamic informer?
Dynamic informers provide real-time updates about resource changes, reduce the need for polling, and improve resource management efficiency. They enhance application responsiveness by enabling immediate reactions to events.
Can I filter events with a dynamic informer?
Yes, you can filter events by specifying a field selector or label selector when creating the informer. This allows you to receive notifications only for specific resources that match your criteria.
How do I handle events received from a dynamic informer?
You can handle events by implementing event handlers that respond to add, update, or delete notifications. These handlers can be registered with the informer to execute specific logic when an event occurs.
Are there any performance considerations when using dynamic informers?
Yes, performance considerations include the number of watched resources and the complexity of event handlers. It’s essential to optimize event processing logic and manage resource usage to avoid overwhelming the system.
Managing events in Kubernetes using a dynamic informer is a powerful approach that allows developers and operators to effectively monitor and respond to changes in the cluster. Dynamic informers leverage the Kubernetes API to provide real-time notifications about resource changes, enabling applications to react promptly to events such as the creation, modification, or deletion of resources. This capability is essential for building responsive and resilient applications within a Kubernetes environment.
One of the key advantages of using dynamic informers is their ability to reduce the overhead associated with polling the Kubernetes API. Instead of continuously querying the API for updates, dynamic informers establish a watch on specific resources, receiving updates only when changes occur. This not only improves efficiency but also minimizes the load on the API server, allowing for better resource utilization across the cluster.
Furthermore, implementing a dynamic informer requires an understanding of the Kubernetes client-go library, which provides the necessary tools to create and manage informers. Developers should be familiar with concepts such as the shared informer factory and the event handlers that process notifications. By leveraging these components, teams can build robust event-driven architectures that enhance the overall functionality and responsiveness of their applications.
managing events with a dynamic informer in Kubernetes is a strategic approach that enhances the responsiveness
Author Profile
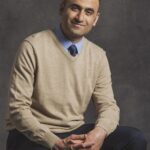
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?