How Can You Print a Dictionary in Python Effectively?
### Introduction
In the world of programming, dictionaries are a fundamental data structure in Python, allowing developers to store and manipulate data in an organized way. Whether you’re a seasoned coder or just starting your journey with Python, understanding how to effectively print a dictionary is a crucial skill that can enhance your ability to debug and present data. Printing a dictionary not only provides a clear view of its contents but also helps in verifying the structure and values stored within it.
As you delve into the nuances of printing dictionaries, you’ll discover various methods that cater to different needs and preferences. From simple print statements to more advanced formatting techniques, each approach offers unique advantages that can elevate your coding experience. Moreover, mastering these techniques can significantly improve your ability to communicate information clearly, making your code more readable and maintainable.
In this article, we will explore the diverse ways to print a dictionary in Python. You’ll learn about the built-in functions and formatting options that can help you display your data in a way that is both informative and aesthetically pleasing. So, whether you’re looking to print a small dictionary for quick debugging or a large dataset for analysis, this guide will equip you with the knowledge you need to present your data effectively.
Using the print() Function
The simplest way to print a dictionary in Python is by using the built-in `print()` function. This will output the dictionary in its standard representation, showing the keys and their corresponding values. For example:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(my_dict)
This will produce the following output:
{‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
While this method is straightforward, it may not be formatted in a visually appealing way, especially for larger dictionaries.
Pretty Printing with pprint
For improved readability, especially with nested dictionaries or larger data structures, Python offers the `pprint` module, which stands for “pretty-print”. This module formats the output to be more human-readable. Here’s how to use it:
python
import pprint
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’, ‘hobbies’: [‘reading’, ‘hiking’]}
pprint.pprint(my_dict)
The output will be more structured:
{‘age’: 30,
‘city’: ‘New York’,
‘hobbies’: [‘reading’, ‘hiking’],
‘name’: ‘Alice’}
Custom Formatting
If you require specific formatting for your dictionary output, you can iterate over its items and format them as you see fit. Below is an example of how to print each key-value pair on a new line:
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(f”{key}: {value}”)
This will yield:
name: Alice
age: 30
city: New York
Using JSON for Structured Output
For dictionaries that need to be printed in a structured format, the `json` module can be used. It allows you to serialize the dictionary into a JSON-formatted string, which is both readable and structured:
python
import json
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(json.dumps(my_dict, indent=4))
This will output the dictionary as:
json
{
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
Comparison of Printing Methods
The following table compares the methods of printing dictionaries in Python:
Method | Output Style | Use Case |
---|---|---|
print() | Standard | Quick output for small dictionaries |
pprint | Formatted | Readable output for larger or nested dictionaries |
Custom Loop | Flexible | Specific formatting needs |
json.dumps() | JSON Structure | Structured output, ideal for APIs |
By selecting the appropriate method based on your requirements, you can effectively present dictionary data in Python, enhancing both readability and usability.
Methods to Print a Dictionary in Python
Printing a dictionary in Python can be accomplished through various methods depending on the desired output format. Below are some common approaches:
Using the print() Function
The simplest way to display a dictionary is to utilize the built-in `print()` function. This will output the dictionary in its standard representation.
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(my_dict)
Output:
{‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
Formatted Printing with f-strings
For more controlled formatting, especially when combining dictionary values with strings, you can use f-strings (available in Python 3.6 and later).
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(f”Name: {my_dict[‘name’]}, Age: {my_dict[‘age’]}, City: {my_dict[‘city’]}”)
Output:
Name: Alice, Age: 30, City: New York
Using the json Module for Pretty Printing
The `json` module allows for pretty-printing of dictionaries, which formats the output in a more readable manner. This is especially useful for larger dictionaries.
python
import json
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(json.dumps(my_dict, indent=4))
Output:
{
“name”: “Alice”,
“age”: 30,
“city”: “New York”
}
Iterating Over Dictionary Items
If you require a more customized output, iterating through the dictionary items can provide flexibility. You can access keys and values individually.
python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
for key, value in my_dict.items():
print(f”{key}: {value}”)
Output:
name: Alice
age: 30
city: New York
Using the pprint Module
The `pprint` module provides a capability to print nested dictionaries in a more human-readable format.
python
from pprint import pprint
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’, ‘hobbies’: [‘reading’, ‘hiking’, ‘coding’]}
pprint(my_dict)
Output:
{‘age’: 30,
‘city’: ‘New York’,
‘hobbies’: [‘reading’, ‘hiking’, ‘coding’],
‘name’: ‘Alice’}
Tabular Representation Using PrettyTable
To display dictionary data in a table format, the `PrettyTable` module can be employed. This is particularly useful for displaying lists of dictionaries.
python
from prettytable import PrettyTable
my_dict_list = [
{‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’},
{‘name’: ‘Bob’, ‘age’: 25, ‘city’: ‘Los Angeles’}
]
table = PrettyTable()
table.field_names = my_dict_list[0].keys()
for item in my_dict_list:
table.add_row(item.values())
print(table)
Output:
+——-+—–+————-+
name | age | city |
---|
+——-+—–+————-+
Alice | 30 | New York |
---|---|---|
Bob | 25 | Los Angeles |
+——-+—–+————-+
Using these methods, you can effectively print dictionaries in Python based on your specific needs and desired output formatting.
Expert Insights on Printing Dictionaries in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Printing a dictionary in Python can be accomplished using the built-in `print()` function. However, for better readability, especially with larger dictionaries, utilizing the `pprint` module is highly recommended as it formats the output in a more structured manner.”
Michael Chen (Lead Software Engineer, CodeCrafters). “When printing dictionaries, it’s essential to consider the data types of the keys and values. For instance, if the dictionary contains nested dictionaries or lists, employing a recursive function to print them can enhance clarity and ensure that all elements are visible.”
Sarah Thompson (Data Scientist, Analytics Hub). “In addition to the standard printing methods, using JSON serialization via the `json` module can be beneficial. This approach allows for a more human-readable format, especially when dealing with complex data structures, by converting the dictionary into a JSON string before printing.”
Frequently Asked Questions (FAQs)
How can I print a dictionary in Python?
You can print a dictionary in Python using the `print()` function. For example, `print(my_dict)` will display the contents of the dictionary `my_dict`.
What is the output format when printing a dictionary?
When you print a dictionary, the output is shown in key-value pairs enclosed in curly braces, such as `{‘key1’: ‘value1’, ‘key2’: ‘value2’}`.
Can I format the output of a dictionary when printing?
Yes, you can format the output using the `json` module. For example, `import json; print(json.dumps(my_dict, indent=4))` will print the dictionary in a more readable format with indentation.
How do I print only the keys or values of a dictionary?
To print only the keys, use `print(my_dict.keys())`. To print only the values, use `print(my_dict.values())`.
Is there a way to print a dictionary in a sorted order?
Yes, you can print a dictionary in sorted order by using the `sorted()` function. For example, `print(dict(sorted(my_dict.items())))` will print the dictionary sorted by keys.
Can I print a dictionary with a specific separator between items?
Yes, you can achieve this by using a loop or a comprehension. For example, `print(“, “.join(f”{k}: {v}” for k, v in my_dict.items()))` will print each key-value pair separated by a comma.
In Python, printing a dictionary can be accomplished in several straightforward ways, depending on the desired output format. The most basic method involves using the built-in `print()` function, which outputs the dictionary in its default string representation. This representation includes the keys and values in a format that closely resembles the dictionary’s syntax in code.
For more structured outputs, developers can utilize the `json` module to print dictionaries in a JSON format, which is often more readable. By using `json.dumps()` with the `indent` parameter, one can achieve a neatly formatted string representation of the dictionary. Additionally, the `pprint` module provides a way to print nested dictionaries in a more visually appealing manner, making it easier to analyze complex data structures.
Key takeaways from the discussion include the versatility of Python’s printing capabilities for dictionaries. Understanding the different methods available allows developers to choose the most appropriate approach based on their specific needs, whether for debugging, logging, or user-facing output. Overall, mastering these techniques enhances the efficiency and clarity of data presentation in Python programming.
Author Profile
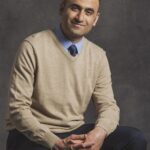
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?