How Can You Print an Array in Python: A Comprehensive Guide?
Printing arrays in Python is a fundamental skill that every programmer should master, whether you’re a novice stepping into the world of coding or an experienced developer looking to refine your skills. Arrays, which are collections of items stored at contiguous memory locations, serve as a powerful tool for organizing and manipulating data efficiently. In Python, while the native list structure is often used to represent arrays, understanding how to print these collections effectively can significantly enhance your ability to debug and present data clearly.
In this article, we will explore various methods for printing arrays in Python, highlighting the flexibility of the language and its built-in functionalities. From simple print statements to more advanced formatting techniques, we will cover the essentials that allow you to display your data in a way that is both readable and informative. Whether you’re working with numerical data, strings, or even complex objects, knowing how to print arrays can make a significant difference in your programming journey.
As we delve deeper, you’ll discover the nuances of different array-like structures in Python, including lists, tuples, and the powerful NumPy arrays. We’ll also touch on best practices for formatting output, ensuring that your printed arrays convey the intended information clearly and concisely. By the end of this article, you’ll be equipped with the knowledge and tools to print arrays like a
Using the print() Function
The most straightforward way to print an array in Python is by utilizing the built-in `print()` function. An array can be created using a list, which is a versatile data structure in Python. When you pass a list to the `print()` function, it will output the elements in a readable format.
“`python
array = [1, 2, 3, 4, 5]
print(array)
“`
Output:
“`
[1, 2, 3, 4, 5]
“`
This method will display the list with its brackets and commas, which is often suitable for debugging purposes.
Iterating Through the Array
For scenarios where you need to format the output or print each element on a new line, you can iterate through the array using a loop. This method allows for greater control over the output format.
“`python
array = [1, 2, 3, 4, 5]
for element in array:
print(element)
“`
This will print each number on a separate line:
“`
1
2
3
4
5
“`
Using the join() Method
To print the elements of an array as a single string without brackets or commas, you can convert each element to a string and use the `join()` method. This is particularly useful for creating a user-friendly output.
“`python
array = [1, 2, 3, 4, 5]
print(‘ ‘.join(map(str, array)))
“`
Output:
“`
1 2 3 4 5
“`
Using NumPy for Arrays
If you are working with numerical data and need more functionality, consider using the NumPy library. NumPy provides a more efficient way to handle arrays and offers additional printing options.
“`python
import numpy as np
array = np.array([1, 2, 3, 4, 5])
print(array)
“`
Output:
“`
[1 2 3 4 5]
“`
NumPy also allows for customized printing formats:
“`python
np.set_printoptions(linewidth=100)
print(array)
“`
Formatted Output with f-strings
For more advanced formatting, Python’s f-strings can be employed. This method allows for easy integration of values into strings, enhancing the clarity of the output.
“`python
array = [1, 2, 3, 4, 5]
for element in array:
print(f’Element: {element}’)
“`
Output:
“`
Element: 1
Element: 2
Element: 3
Element: 4
Element: 5
“`
Comparison of Methods
The following table summarizes the different methods to print an array in Python, highlighting their primary use cases:
Method | Output Format | Use Case |
---|---|---|
print() | List with brackets | Quick debugging |
Loop | Each element on a new line | Detailed output |
join() | Single line without brackets | User-friendly output |
NumPy | Efficient numerical arrays | Scientific computing |
f-strings | Custom formatted strings | Enhanced clarity |
Using the `print()` Function
The simplest way to print an array (or list) in Python is by using the built-in `print()` function. This method outputs the entire list in a single line.
“`python
my_array = [1, 2, 3, 4, 5]
print(my_array)
“`
The output will be:
“`
[1, 2, 3, 4, 5]
“`
Printing Each Element Individually
If you require more control over the format, you can print each element of the array individually using a loop. This allows for customization of how each element is displayed.
“`python
for element in my_array:
print(element)
“`
Output:
“`
1
2
3
4
5
“`
Formatting Output with `join()`
When dealing with an array of strings, you can utilize the `join()` method to create a formatted string before printing.
“`python
string_array = [‘apple’, ‘banana’, ‘cherry’]
print(“, “.join(string_array))
“`
Output:
“`
apple, banana, cherry
“`
Using List Comprehensions
List comprehensions can provide a concise way to format and print arrays. This method allows for transformations during the print operation.
“`python
squared_array = [x ** 2 for x in my_array]
print(squared_array)
“`
Output:
“`
[1, 4, 9, 16, 25]
“`
Using the `pprint` Module
For more complex data structures, such as nested arrays, the `pprint` (pretty-print) module is beneficial. It formats the output for better readability.
“`python
import pprint
nested_array = [[1, 2], [3, 4, 5], [6]]
pprint.pprint(nested_array)
“`
Output:
“`
[[1, 2],
[3, 4, 5],
[6]]
“`
Using NumPy Arrays
If you are working with numerical data, the NumPy library provides an efficient way to handle arrays. Using NumPy’s `print()` function allows for better control over the display format.
“`python
import numpy as np
numpy_array = np.array([1, 2, 3, 4, 5])
print(numpy_array)
“`
Output:
“`
[1 2 3 4 5]
“`
Customizing Print Output
You can customize the output further by specifying parameters in the `print()` function. For example, changing the separator or end character.
“`python
print(*my_array, sep=’ | ‘, end=’.’)
“`
Output:
“`
1 | 2 | 3 | 4 | 5.
“`
Using Format Strings
Another method to customize the output is through format strings, which enable you to control the appearance of each printed element.
“`python
for element in my_array:
print(f”Element: {element}”)
“`
Output:
“`
Element: 1
Element: 2
Element: 3
Element: 4
Element: 5
“`
Expert Insights on Printing Arrays in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Printing arrays in Python can be efficiently handled using the built-in print function. However, for more complex data structures, utilizing libraries such as NumPy can provide enhanced functionality and better formatting options.”
James Liu (Data Scientist, Analytics Solutions Group). “To print an array in Python, one can simply use the print() function. However, for large datasets, I recommend converting the array to a string format or using pretty-printing libraries to improve readability.”
Sarah Thompson (Educator and Python Programming Author). “Understanding how to print arrays in Python is fundamental for beginners. I always advise my students to explore different methods, such as using loops or list comprehensions, to gain a deeper understanding of Python’s capabilities.”
Frequently Asked Questions (FAQs)
How do I print a simple array in Python?
To print a simple array in Python, use the `print()` function along with the array variable. For example, `print(my_array)` will display the contents of `my_array`.
Can I print a NumPy array in Python?
Yes, you can print a NumPy array using the `print()` function. For example, if you have a NumPy array called `np_array`, simply use `print(np_array)` to display its contents.
What is the difference between printing a list and an array in Python?
In Python, lists are built-in data structures that can hold mixed data types, while arrays (from the `array` module or NumPy) are more efficient for numerical data and require elements to be of the same type. Both can be printed using the `print()` function.
How can I format the output when printing an array?
You can format the output of an array using string formatting methods such as f-strings or the `format()` method. For example, `print(f”Array contents: {my_array}”)` provides a custom message with the array contents.
Is there a way to print an array with specific formatting, like commas or new lines?
Yes, you can customize the output using the `join()` method for lists or by iterating through the array. For example, `print(“, “.join(map(str, my_array)))` will print the elements separated by commas.
How do I print multi-dimensional arrays in Python?
To print multi-dimensional arrays, you can use nested loops or the `print()` function directly if using NumPy. For example, `print(np_array)` will display the structure clearly if `np_array` is a NumPy array.
Printing an array in Python can be accomplished using various methods, depending on the type of array and the desired output format. The most common approach involves utilizing Python’s built-in data structures, such as lists or the NumPy library for numerical arrays. For basic lists, the print function suffices, while NumPy provides specialized functions for more complex array manipulations and visualizations.
When working with lists, simply using the print function will display the entire list in a straightforward manner. For multi-dimensional arrays, especially those created with NumPy, the array can be printed in a formatted way that enhances readability. Functions like `numpy.array2string()` or setting print options in NumPy can help customize the output, making it easier to interpret large datasets.
In summary, understanding the type of array you are working with is key to selecting the appropriate method for printing. Leveraging Python’s capabilities, whether through basic lists or advanced libraries like NumPy, allows for effective data representation. This knowledge is essential for efficient data handling and presentation in Python programming.
Author Profile
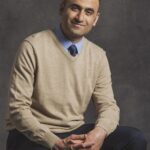
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?