How Can You Print Specific Elements from a List in Python?
In the world of programming, particularly in Python, lists are one of the most versatile and widely used data structures. They allow you to store collections of items, making it easy to manipulate and access data. However, as your lists grow in size and complexity, the ability to print specific elements becomes crucial for debugging, data analysis, and effective coding practices. Whether you’re a beginner eager to learn the ropes or an experienced developer looking to refine your skills, understanding how to efficiently extract and display specific elements from a list can significantly enhance your programming toolkit.
Printing specific elements in a Python list involves a combination of indexing, slicing, and utilizing loops or comprehensions. Each of these methods offers unique advantages depending on the context and requirements of your task. For instance, if you need to access individual elements, simple indexing can do the trick, while slicing allows you to retrieve a range of elements in one go. Moreover, leveraging loops can enable you to print elements conditionally, providing a powerful way to filter and display data based on specific criteria.
As you delve deeper into the intricacies of list manipulation, you’ll discover that Python’s rich set of built-in functions and methods can streamline the process. From list comprehensions that condense your code into elegant one-liners to the use of libraries
Accessing Specific Elements
To print specific elements from a list in Python, you can use indexing and slicing techniques. Python lists are zero-indexed, meaning the first element is at index 0. You can access elements directly by their index, or retrieve a subset of elements using slicing.
- Accessing a Single Element: To access a single element, specify its index in square brackets. For example, if you have a list `my_list = [10, 20, 30, 40, 50]`, you can print the first element with `print(my_list[0])`, which outputs `10`.
- Accessing Multiple Elements: You can retrieve multiple elements using slicing. For example, `my_list[1:4]` will return a new list containing elements from index 1 to index 3: `[20, 30, 40]`.
Printing Elements Based on Conditions
If you need to print elements that meet certain criteria, list comprehensions or filter functions are useful. For instance, if you want to print all even numbers from a list:
“`python
my_list = [10, 21, 32, 43, 54]
even_numbers = [num for num in my_list if num % 2 == 0]
print(even_numbers) Outputs: [10, 32, 54]
“`
This approach allows you to apply more complex conditions as necessary.
Using Loops to Print Specific Elements
Another method to print specific elements is through the use of loops. You can iterate over the list and apply conditions within the loop. Here’s an example:
“`python
my_list = [10, 21, 32, 43, 54]
for num in my_list:
if num > 30:
print(num) Outputs: 32, 43, 54
“`
This method provides flexibility in handling each element based on defined logic.
Table of Indexing and Slicing Examples
The following table summarizes different indexing and slicing scenarios for a given list.
Operation | Example Code | Result |
---|---|---|
Single Element Access | my_list[2] | 30 |
Slicing | my_list[1:3] | [20, 30] |
Negative Indexing | my_list[-1] | 50 |
Conditional Print | [num for num in my_list if num > 25] | [30, 40, 50] |
Utilizing these techniques, you can effectively manage and print the specific elements from lists in Python, tailoring your output to meet your needs.
Accessing Elements in a List
To print specific elements from a list in Python, first understand how to access elements using their indices. Python uses zero-based indexing, meaning the first element is at index 0.
- To access a single element:
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
print(my_list[1]) Output: banana
“`
- To access multiple elements, you can use slicing:
“`python
print(my_list[0:2]) Output: [‘apple’, ‘banana’]
“`
Using Conditional Statements
If you need to print elements based on certain conditions, utilize loops along with conditional statements.
- Example: Print only the fruits that start with the letter ‘b’:
“`python
for fruit in my_list:
if fruit.startswith(‘b’):
print(fruit) Output: banana
“`
Filtering Lists with List Comprehensions
List comprehensions provide a concise way to filter lists. This method is efficient and readable.
- Example: Print fruits that contain the letter ‘e’:
“`python
filtered_fruits = [fruit for fruit in my_list if ‘e’ in fruit]
print(filtered_fruits) Output: [‘cherry’]
“`
Using the `filter()` Function
The `filter()` function can also be used to print specific elements based on a function that returns `True` or “.
- Example: Using a lambda function to filter:
“`python
filtered = filter(lambda x: ‘a’ in x, my_list)
print(list(filtered)) Output: [‘banana’, ‘cherry’]
“`
Printing Elements Based on Their Index
To print specific elements by their index, you can create a list of desired indices and iterate through it.
- Example: Print elements at indices 0 and 2:
“`python
indices = [0, 2]
for i in indices:
print(my_list[i]) Output: apple, cherry
“`
Using `enumerate()` for Indexed Printing
The `enumerate()` function can be particularly useful when you want both the index and the value while iterating.
- Example: Print elements with their indices:
“`python
for index, fruit in enumerate(my_list):
if index % 2 == 0: Only print even indices
print(f”{index}: {fruit}”) Output: 0: apple, 2: cherry
“`
Creating a Function to Print Specific Elements
Encapsulating your logic in a function makes it reusable and clean.
- Example: Function to print elements that contain a specific substring:
“`python
def print_elements_containing(substring, lst):
for item in lst:
if substring in item:
print(item)
print_elements_containing(‘a’, my_list) Output: apple, banana
“`
Using Libraries for Advanced Filtering
For more complex data manipulation, consider using libraries like `pandas`.
- Example with `pandas`:
“`python
import pandas as pd
df = pd.DataFrame({‘fruits’: my_list})
print(df[df[‘fruits’].str.contains(‘e’)]) Output: rows containing ‘e’
“`
Expert Insights on Printing Specific Elements in Python Lists
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To print specific elements in a list in Python, one can utilize list indexing or slicing. For instance, using `print(my_list[0])` retrieves the first element, while `print(my_list[1:3])` prints elements from index 1 to 2. This flexibility allows for targeted data retrieval.”
Michael Chen (Data Scientist, Analytics Hub). “Employing list comprehensions is an efficient way to print specific elements based on conditions. For example, `print([x for x in my_list if x > 10])` will print all elements greater than 10, showcasing Python’s powerful data manipulation capabilities.”
Lisa Tran (Python Instructor, Code Academy). “Using the `filter()` function is another effective method to print specific elements. For example, `print(list(filter(lambda x: x % 2 == 0, my_list)))` will output only the even numbers from the list, demonstrating a functional programming approach within Python.”
Frequently Asked Questions (FAQs)
How can I print specific elements from a list in Python?
You can print specific elements from a list by accessing them using their index. For example, `print(my_list[0])` prints the first element, while `print(my_list[1:3])` prints elements from index 1 to 2.
What is list slicing in Python?
List slicing allows you to extract a portion of a list by specifying a start and end index. For instance, `my_list[start:end]` returns a new list containing elements from the start index up to, but not including, the end index.
Can I print elements from a list based on a condition?
Yes, you can use list comprehensions or the `filter()` function to print elements that meet specific conditions. For example, `[x for x in my_list if x > 10]` prints all elements greater than 10.
How do I print every second element in a list?
You can achieve this by using slicing with a step. For example, `print(my_list[::2])` prints every second element from the list.
Is it possible to print elements from a list that are of a certain type?
Yes, you can use a list comprehension to filter elements by type. For example, `[x for x in my_list if isinstance(x, int)]` prints all integer elements from the list.
How do I print elements from multiple lists in Python?
You can concatenate lists using the `+` operator or use the `zip()` function for paired elements. For example, `print(list1 + list2)` prints all elements from both lists, while `for a, b in zip(list1, list2): print(a, b)` prints elements from both lists in pairs.
In Python, printing specific elements from a list can be accomplished using various methods, depending on the criteria for selection. Common techniques include indexing, slicing, and using list comprehensions. Indexing allows you to access individual elements directly by their position, while slicing enables you to extract a range of elements. List comprehensions provide a concise way to filter elements based on specific conditions, enhancing both readability and efficiency in your code.
Moreover, utilizing loops, such as for-loops, can facilitate the printing of elements that meet certain criteria. This approach is particularly useful when dealing with larger datasets or when the selection criteria are complex. Additionally, the use of built-in functions like `filter()` can further streamline the process, allowing for functional programming techniques to be applied in Python.
Overall, understanding these methods equips Python developers with the tools needed to manipulate and display list data effectively. By mastering these techniques, programmers can write cleaner, more efficient code that enhances the readability and maintainability of their projects. As Python continues to evolve, these foundational skills remain essential for any developer working with data structures.
Author Profile
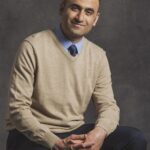
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?