How Can You Raise a ValueError in Python?
In the world of programming, error handling is a crucial skill that can make the difference between a robust application and one riddled with bugs. Among the various types of errors that can arise during the execution of a Python program, the `ValueError` stands out as a common yet significant issue. This error typically surfaces when a function receives an argument of the right type but an inappropriate value, leading to unexpected behavior. Understanding how to intentionally raise a `ValueError` can empower developers to enforce data integrity and provide clearer feedback to users, ultimately enhancing the overall user experience.
Raising a `ValueError` in Python is not just about signaling that something has gone wrong; it’s about communicating specific expectations and constraints within your code. By leveraging this exception, developers can create more predictable and manageable applications. This practice is particularly useful in scenarios where input validation is critical, such as when dealing with user inputs, processing data from external sources, or ensuring that function arguments meet certain criteria.
In this article, we will explore the nuances of raising a `ValueError`, including the various contexts in which it can be applied and best practices for implementing it effectively. Whether you’re a seasoned programmer or just starting your journey in Python, mastering the art of error handling will undoubtedly elevate your coding skills and
Understanding ValueError in Python
ValueError is a built-in exception in Python that is raised when an operation or function receives an argument that has the right type but an inappropriate value. For instance, passing a string that cannot be converted to an integer will trigger a ValueError. Understanding when and how to raise this exception is crucial for effective error handling in your programs.
When to Raise a ValueError
Raising a ValueError is appropriate in various scenarios, including but not limited to:
- When user input does not conform to expected formats.
- When data processing functions encounter unexpected values.
- When a function’s preconditions are not met.
For example, if a function is designed to accept a positive integer, and a negative integer is provided, it would be prudent to raise a ValueError.
How to Raise a ValueError
Raising a ValueError in Python is straightforward. You can use the `raise` statement followed by the `ValueError` class. Here’s the syntax:
python
raise ValueError(“Error message describing the issue”)
Example Code Snippets
Consider the following example that checks if a number is positive:
python
def check_positive(number):
if number < 0:
raise ValueError("The number must be positive.")
return number
In this code, if a user provides a negative number, the function will raise a ValueError with a descriptive message.
Best Practices for Raising ValueError
When raising a ValueError, follow these best practices to enhance code readability and maintainability:
- Always provide a clear and concise error message.
- Ensure the raised exception is relevant to the context.
- Use custom messages to aid in debugging.
Here is a table summarizing common situations in which you might raise a ValueError along with example messages:
Scenario | Example Message |
---|---|
Invalid input type | “Expected an integer, got a string.” |
Out of range value | “Value must be between 1 and 100.” |
Invalid format | “Date must be in YYYY-MM-DD format.” |
Raising a ValueError is an essential part of robust error handling in Python. By understanding when and how to raise this exception, you can create more reliable and maintainable code.
Understanding ValueError in Python
In Python, a `ValueError` is raised when a built-in operation or function receives an argument that has the right type but an inappropriate value. Common examples include attempting to convert a string to an integer when the string does not represent a valid integer.
### Common Scenarios for ValueError
- Type Conversion: Trying to convert a non-numeric string to an integer.
- Mathematical Operations: Performing operations that do not make sense for the input values, such as taking the square root of a negative number.
- Data Parsing: Extracting data from user input or files that do not meet expected formats.
### Raising a ValueError
To raise a `ValueError`, you can use the `raise` statement followed by the `ValueError` class. This can be performed in your own functions or methods to enforce specific conditions.
python
def check_positive(value):
if value < 0:
raise ValueError("Value must be positive.")
return value
### Example of Raising ValueError
Consider the following example where a function checks if an input string can be converted to an integer. If it cannot, a `ValueError` is raised:
python
def convert_to_integer(s):
try:
return int(s)
except ValueError:
raise ValueError(f"Cannot convert '{s}' to an integer.")
### Best Practices for Raising ValueError
- Clarity: Always provide a clear and descriptive message when raising a `ValueError`.
- Validation: Validate input data before performing operations to avoid unintentional errors.
- Documentation: Document your functions to inform users of the conditions under which a `ValueError` may be raised.
### Handling ValueError
When working with functions that may raise a `ValueError`, you can use `try-except` blocks to handle the error gracefully:
python
try:
result = convert_to_integer(“abc”)
except ValueError as e:
print(f”Error: {e}”)
This code snippet will catch the `ValueError` raised by the `convert_to_integer` function and print an error message without crashing the program.
### Summary of Key Points
Aspect | Details |
---|---|
Exception Type | ValueError |
When to Use | When an operation receives an argument of the right type but inappropriate value |
Raising Syntax | `raise ValueError(“Your message here”)` |
Handling | Use `try-except` to catch and manage the error |
By adhering to these guidelines, you can effectively utilize and manage `ValueError` in your Python applications, ensuring that your code remains robust and user-friendly.
Expert Insights on Raising Value Errors in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Raising a ValueError in Python is crucial when a function receives an argument of the right type but an inappropriate value. This ensures that the code behaves predictably and helps maintain data integrity.”
Michael Thompson (Python Developer, CodeCraft Academy). “To raise a ValueError, one should use the ‘raise’ statement followed by the ValueError class. This practice not only aids in debugging but also provides clear feedback to the user about incorrect inputs.”
Linda Zhang (Data Scientist, Analytics Hub). “Incorporating custom messages when raising a ValueError can significantly enhance user experience. It allows users to understand the specific nature of the error, facilitating quicker resolutions.”
Frequently Asked Questions (FAQs)
How do I raise a ValueError in Python?
You can raise a ValueError in Python using the `raise` statement followed by the `ValueError` class. For example: `raise ValueError(“Invalid value provided”)`.
When should I use ValueError in my code?
Use ValueError when a function receives an argument of the correct type but an inappropriate value. This helps indicate that the input is semantically incorrect.
Can I customize the message when raising a ValueError?
Yes, you can customize the message by passing a string as an argument to the ValueError. For instance: `raise ValueError(“Custom error message”)`.
Is it possible to catch a ValueError in Python?
Yes, you can catch a ValueError using a try-except block. For example:
python
try:
# code that may raise ValueError
except ValueError as e:
print(f”Caught an error: {e}”)
What are some common scenarios where ValueError is raised?
Common scenarios include converting a string to an integer with `int()` when the string is not a valid number, or when using functions that expect a specific range of values.
Can I create my own exception class that inherits from ValueError?
Yes, you can create a custom exception class by inheriting from ValueError. This allows you to define specific error conditions while maintaining the characteristics of a ValueError. For example:
python
class MyCustomError(ValueError):
pass
Raising a ValueError in Python is a straightforward process that involves using the `raise` statement. This exception is typically used to indicate that a function has received an argument of the right type but an inappropriate value. By explicitly raising a ValueError, developers can signal to users of their code that something has gone wrong due to invalid input, thereby enhancing the robustness and clarity of their programs.
To raise a ValueError, you can use the syntax `raise ValueError(“Your error message here”)`. This allows you to provide a custom error message that can help users understand the nature of the issue. It is essential to implement this in functions where input validation is critical, ensuring that the program behaves predictably and provides informative feedback when faced with incorrect values.
In summary, effectively raising a ValueError is an important aspect of error handling in Python. It not only helps maintain the integrity of your code but also improves the user experience by providing clear communication about what went wrong. By following best practices for input validation and exception handling, developers can create more reliable and user-friendly applications.
Author Profile
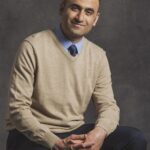
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?