How Can You Easily Read an XML File in Python?
In today’s data-driven world, XML (eXtensible Markup Language) serves as a vital format for storing and transporting structured information. Whether you’re dealing with web services, configuration files, or data interchange between systems, understanding how to read XML files in Python can significantly enhance your programming toolkit. Python, with its rich ecosystem of libraries and straightforward syntax, makes it easier than ever to parse and manipulate XML data. If you’ve ever found yourself grappling with complex data structures or seeking a reliable way to extract information from XML files, you’re in the right place.
Reading XML files in Python involves leveraging powerful libraries that simplify the process of parsing and navigating through the XML structure. With options like `xml.etree.ElementTree`, `lxml`, and `xml.dom.minidom`, developers can choose the most suitable tool for their specific needs. Each library offers unique features and functionalities, enabling you to handle everything from simple data extraction to more complex manipulations of XML content.
As you delve deeper into the topic, you’ll discover various techniques for efficiently reading and processing XML files, including handling namespaces, iterating through elements, and extracting attributes. By mastering these skills, you’ll not only enhance your ability to work with XML data but also broaden your understanding of data representation in programming. Get ready
Using the ElementTree Module
The ElementTree module is a standard Python library for parsing and creating XML data. It provides a simple and efficient way to navigate and manipulate XML trees. To read an XML file with ElementTree, follow these steps:
- Import the ElementTree module.
- Load the XML file.
- Parse the XML content into an ElementTree object.
- Access the elements using methods provided by the module.
Here is a sample code snippet demonstrating how to read an XML file using ElementTree:
“`python
import xml.etree.ElementTree as ET
Load and parse the XML file
tree = ET.parse(‘example.xml’)
root = tree.getroot()
Iterate through elements and print their tags and attributes
for child in root:
print(child.tag, child.attrib)
“`
Using the minidom Module
The minidom module is another option for reading XML files in Python. It is part of the xml package and provides a Document Object Model (DOM) interface. This approach is slightly more complex but allows for more detailed manipulation of XML documents.
To use minidom to read an XML file, follow these steps:
- Import the minidom module.
- Use the `parse` method to load the XML file.
- Access elements using methods such as `getElementsByTagName`.
Example of reading an XML file with minidom:
“`python
from xml.dom import minidom
Load and parse the XML file
doc = minidom.parse(‘example.xml’)
Get all elements by tag name
elements = doc.getElementsByTagName(‘tagname’)
Print each element’s text content
for element in elements:
print(element.firstChild.nodeValue)
“`
Using the lxml Library
The lxml library is a powerful third-party library that provides extensive capabilities for XML and HTML processing. It is not included in the standard library, so it needs to be installed separately using pip.
To read an XML file with lxml, follow these steps:
- Install the lxml library.
- Import the library.
- Use the `etree` module to parse the XML file.
Here is an example of reading an XML file using lxml:
“`python
from lxml import etree
Load and parse the XML file
tree = etree.parse(‘example.xml’)
Access elements using XPath
elements = tree.xpath(‘//tagname’)
Print the text content of each element
for element in elements:
print(element.text)
“`
Comparison of XML Parsing Methods
The choice of XML parsing method can depend on your specific needs and the complexity of the XML data. Below is a comparison table highlighting key features of each method:
Feature | ElementTree | minidom | lxml |
---|---|---|---|
Standard Library | Yes | Yes | No |
Ease of Use | High | Medium | High |
Performance | Good | Fair | Excellent |
XPath Support | No | No | Yes |
By evaluating these options, you can select the most suitable method for reading XML files in your Python projects.
Understanding XML in Python
XML (eXtensible Markup Language) is a versatile format for storing and transporting data. Python provides several libraries to read XML files, each with its distinct features and advantages. The most commonly used libraries include `xml.etree.ElementTree`, `lxml`, and `minidom`.
Using xml.etree.ElementTree
The `xml.etree.ElementTree` module is part of Python’s standard library and offers a simple way to parse and create XML data.
Basic Usage:
- Import the module:
“`python
import xml.etree.ElementTree as ET
“`
- Load an XML file:
“`python
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
- Accessing elements:
“`python
for child in root:
print(child.tag, child.attrib)
“`
Example:
“`python
import xml.etree.ElementTree as ET
tree = ET.parse(‘example.xml’)
root = tree.getroot()
for child in root:
print(child.tag, child.attrib)
“`
Using lxml for Advanced Parsing
`lxml` is a powerful library that provides extensive support for XML and HTML processing.
Installation:
“`bash
pip install lxml
“`
Basic Usage:
“`python
from lxml import etree
tree = etree.parse(‘file.xml’)
root = tree.getroot()
“`
XPath Queries:
`lxml` supports XPath for complex queries, allowing you to select nodes with specific criteria.
Example:
“`python
results = root.xpath(‘//tagname[@attribute=”value”]’)
for result in results:
print(result.text)
“`
Using minidom for DOM Parsing
`minidom`, another module in the standard library, provides a DOM (Document Object Model) interface for XML.
Basic Usage:
“`python
from xml.dom import minidom
doc = minidom.parse(‘file.xml’)
elements = doc.getElementsByTagName(‘tagname’)
“`
Extracting Data:
“`python
for element in elements:
print(element.firstChild.data)
“`
Comparison of Libraries
The following table summarizes key features of the discussed libraries:
Feature | xml.etree.ElementTree | lxml | minidom |
---|---|---|---|
Standard Library | Yes | No | Yes |
XPath Support | Limited | Full | No |
Performance | Moderate | High | Moderate |
Ease of Use | Simple | Slightly Complex | Moderate |
Best For | Simple XML files | Complex XML handling | Basic XML parsing |
Best Practices
When working with XML files in Python, consider the following best practices:
- Validate XML Structure: Ensure the XML file adheres to its schema.
- Error Handling: Use try-except blocks to handle potential parsing errors.
- Use Context Managers: When dealing with file operations, use context managers to ensure files are properly closed.
- Avoid Memory Issues: For large XML files, consider using iterparse to process elements one at a time to save memory.
By following these practices, you can efficiently read and manipulate XML data in Python, leveraging the strengths of each library as needed.
Expert Insights on Reading XML Files in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “To effectively read an XML file in Python, utilizing the built-in `xml.etree.ElementTree` module is highly recommended. It provides a simple and efficient way to parse XML data, allowing for easy navigation through elements and attributes.”
Michael Chen (Software Engineer, CodeMasters). “For those looking to handle more complex XML structures, I suggest using the `lxml` library. It not only offers extensive support for XPath and XSLT but also enhances performance when dealing with large XML files.”
Sarah Thompson (Python Developer, Open Source Projects). “When reading XML files, it is crucial to consider error handling. Implementing try-except blocks can help manage potential parsing errors, ensuring that your application remains robust and user-friendly.”
Frequently Asked Questions (FAQs)
How can I read an XML file in Python?
You can read an XML file in Python using libraries such as `xml.etree.ElementTree`, `lxml`, or `xml.dom.minidom`. The `ElementTree` module is commonly used for its simplicity and ease of use.
What is the basic syntax for reading an XML file using ElementTree?
The basic syntax involves importing the `ElementTree` module and using `ElementTree.parse()` to read the XML file. For example:
“`python
import xml.etree.ElementTree as ET
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
Can I read XML files with different encodings in Python?
Yes, you can read XML files with different encodings by specifying the encoding when opening the file. Use the `open()` function with the appropriate encoding parameter, such as `open(‘file.xml’, ‘r’, encoding=’utf-8′)`.
What are the advantages of using the lxml library for XML parsing?
The `lxml` library offers several advantages, including better performance, support for XPath, and the ability to handle large XML files efficiently. It also provides a more feature-rich API compared to the built-in libraries.
How can I extract specific elements from an XML file in Python?
You can extract specific elements using methods like `.find()`, `.findall()`, or `.iter()` from the `ElementTree` module. For example:
“`python
specific_element = root.find(‘tag_name’)
“`
Is it possible to modify an XML file after reading it in Python?
Yes, you can modify an XML file after reading it by changing the elements in the parsed tree and then writing the changes back to the file using `ElementTree.write()`. For example:
“`python
tree.write(‘modified_file.xml’)
“`
Reading an XML file in Python can be accomplished using various libraries, with the most common being `xml.etree.ElementTree`, `lxml`, and `xml.dom.minidom`. Each of these libraries offers distinct advantages, depending on the complexity of the XML data and the specific requirements of the task. For simple XML parsing, `ElementTree` is often sufficient, while `lxml` provides more advanced features, including support for XPath and XSLT. The `xml.dom.minidom` library is useful for those who prefer a Document Object Model (DOM) approach to manipulate XML data.
To read an XML file, one typically begins by importing the chosen library and then loading the XML content. For instance, with `ElementTree`, you can parse the XML file using `ElementTree.parse()` and navigate through the elements using methods like `find()` and `iter()`. This allows for easy extraction of data from the XML structure. In contrast, `lxml` offers more robust handling of XML namespaces and schema validation, making it suitable for more complex XML documents.
Key takeaways include the importance of selecting the right library based on the specific needs of the project. For straightforward XML parsing, `ElementTree`
Author Profile
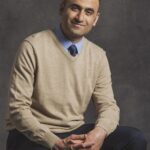
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?