How Can You Easily Remove ‘n’ from a String in Python?
Strings are a fundamental aspect of programming in Python, serving as the building blocks for text manipulation and data processing. Whether you’re cleaning up user input, formatting data for display, or preparing strings for analysis, knowing how to efficiently modify strings is crucial. One common task that developers encounter is the need to remove specific characters from strings, such as the letter ‘n’. This seemingly simple operation can be approached in various ways, each with its own advantages and use cases. In this article, we will explore the methods to remove ‘n’ from strings in Python, equipping you with the knowledge to handle similar challenges in your coding journey.
When it comes to string manipulation in Python, the versatility of the language shines through. Removing characters like ‘n’ can be accomplished using built-in methods that are both efficient and easy to implement. From leveraging string methods to utilizing list comprehensions, Python offers a range of techniques that cater to different scenarios. Understanding these methods not only enhances your coding skills but also empowers you to write cleaner, more efficient code.
As we delve deeper into the topic, we will examine various approaches to removing ‘n’ from strings, considering factors such as performance and readability. Whether you’re a beginner looking to grasp the basics or an experienced programmer seeking to refine
Methods for Removing ‘n’ from a String
To remove the character ‘n’ from a string in Python, several methods can be employed, each suited to different use cases. Below are the most common approaches:
Using String Replace Method
The simplest way to remove ‘n’ from a string is by using the built-in `replace()` method. This method creates a new string where all occurrences of a specified substring are replaced with another substring. To remove ‘n’, you can replace it with an empty string.
“`python
original_string = “banana”
modified_string = original_string.replace(‘n’, ”)
print(modified_string) Output: “baa”
“`
Using List Comprehension
List comprehension provides a concise way to construct lists in Python. By filtering out the character ‘n’, you can create a new string without it.
“`python
original_string = “banana”
modified_string = ”.join([char for char in original_string if char != ‘n’])
print(modified_string) Output: “baa”
“`
Using Regular Expressions
For more complex scenarios, such as removing multiple characters or using patterns, the `re` module can be utilized. Here’s how to remove ‘n’ using regular expressions:
“`python
import re
original_string = “banana”
modified_string = re.sub(‘n’, ”, original_string)
print(modified_string) Output: “baa”
“`
Comparison of Methods
Each method has its advantages depending on the context. Below is a comparison of the methods discussed:
Method | Advantages | Disadvantages |
---|---|---|
String Replace | Simple and straightforward | Replaces all occurrences; not suitable for conditional removal |
List Comprehension | Flexible; allows for conditions | Less readable for beginners |
Regular Expressions | Powerful for complex patterns | Overhead of importing module; can be less efficient for simple tasks |
Conclusion on Usage
Choosing the right method to remove ‘n’ from a string depends on the specific requirements of your application. For simple replacements, `replace()` is usually sufficient. For more complex needs, consider list comprehensions or regular expressions.
Methods to Remove ‘n’ from a String in Python
Removing specific characters from a string in Python can be efficiently achieved using several methods. Here are some common techniques:
Using the `replace()` Method
The `replace()` method allows you to replace specified characters in a string with another character or an empty string. To remove ‘n’, you can replace it with an empty string:
“`python
original_string = “banana”
modified_string = original_string.replace(‘n’, ”)
print(modified_string) Output: “baa”
“`
Using List Comprehension
List comprehension provides a concise way to create lists by filtering out unwanted characters. This technique can be used to build a new string without ‘n’:
“`python
original_string = “banana”
modified_string = ”.join([char for char in original_string if char != ‘n’])
print(modified_string) Output: “baa”
“`
Using `filter()` Function
The `filter()` function can also be utilized to remove specific characters. It filters the elements of an iterable based on a function’s return value:
“`python
original_string = “banana”
modified_string = ”.join(filter(lambda x: x != ‘n’, original_string))
print(modified_string) Output: “baa”
“`
Using Regular Expressions
For more complex string manipulations, the `re` module can be employed. This is particularly useful when you need to remove multiple characters or patterns:
“`python
import re
original_string = “banana”
modified_string = re.sub(‘n’, ”, original_string)
print(modified_string) Output: “baa”
“`
Performance Considerations
When choosing a method to remove characters from a string, consider the following factors:
Method | Complexity | Best Use Case |
---|---|---|
`replace()` | O(n) | Simple character replacement |
List comprehension | O(n) | Custom filtering and transformations |
`filter()` | O(n) | Functional programming style |
Regular expressions | O(n) | Complex patterns and multiple characters |
Each method has its advantages, and the choice depends on the specific requirements of your task. For straightforward replacements, `replace()` is often the simplest. For more complex scenarios, consider using regular expressions or list comprehensions for their flexibility and power.
Expert Insights on Removing Characters from Strings in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To effectively remove a specific character, such as ‘n’, from a string in Python, one can utilize the string method `replace()`. This method allows for a straightforward approach by replacing the target character with an empty string, thus achieving the desired result.”
James Liu (Python Developer, Tech Innovators Inc.). “Using list comprehensions is another efficient way to remove unwanted characters from a string. By iterating through each character and including only those that do not match ‘n’, developers can create a new string that omits the specified character.”
Sarah Thompson (Data Scientist, Analytics Hub). “For those working with larger datasets, utilizing regular expressions through the `re` module can provide a powerful solution. The `re.sub()` function allows for more complex patterns and can be particularly useful when needing to remove multiple instances of ‘n’ or other characters in a single operation.”
Frequently Asked Questions (FAQs)
How can I remove all occurrences of the letter ‘n’ from a string in Python?
You can use the `replace()` method of a string. For example: `my_string.replace(‘n’, ”)` will return a new string with all ‘n’ characters removed.
Is there a way to remove ‘n’ using regular expressions in Python?
Yes, you can use the `re` module. The code `import re; re.sub(‘n’, ”, my_string)` will remove all occurrences of ‘n’ from `my_string`.
Can I remove ‘n’ from a string while keeping the case sensitivity in mind?
Yes, you can modify the `replace()` method to handle both cases by using: `my_string.replace(‘n’, ”).replace(‘N’, ”)` or by using regular expressions with the `flags=re.IGNORECASE` option.
What if I want to remove ‘n’ only from the beginning of the string?
You can use the `lstrip()` method, which removes specified characters from the left side of the string. For example: `my_string.lstrip(‘n’)` removes ‘n’ from the start only.
Is there a method to remove ‘n’ from a string based on its position?
Yes, you can use slicing to remove ‘n’ based on its index. For instance, `my_string[:index] + my_string[index+1:]` removes ‘n’ at the specified `index`.
How do I remove ‘n’ from a list of strings in Python?
You can use a list comprehension: `[s.replace(‘n’, ”) for s in my_list]` will create a new list with ‘n’ removed from each string in `my_list`.
Removing a specific character, such as ‘n’, from a string in Python can be accomplished using several methods. The most common approaches include using the `replace()` method, list comprehensions, and the `filter()` function. Each of these methods allows for efficient modification of strings, catering to different use cases and preferences in coding style.
The `replace()` method is straightforward and effective for this task. It allows you to specify the character you wish to remove and replace it with an empty string. This method is particularly useful for cases where you want to remove all occurrences of the character in one simple line of code. On the other hand, list comprehensions provide a more flexible approach, allowing for the construction of a new string by including only those characters that do not match the specified character. This method is beneficial when you need to apply additional conditions or transformations to the string.
Additionally, the `filter()` function can be employed to achieve similar results. By passing a lambda function that excludes the unwanted character, you can create a new string from the original. This method is particularly advantageous when working with more complex filtering criteria. Overall, Python offers multiple efficient ways to remove characters from strings, enabling developers to choose the method that best fits their
Author Profile
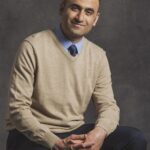
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?