How Can You Easily Repeat Actions in Python?
In the world of programming, repetition is a fundamental concept that allows developers to execute a block of code multiple times without the need for redundancy. Whether you’re automating tasks, processing data, or creating dynamic applications, understanding how to repeat actions efficiently in Python is essential. This powerful language offers several methods to achieve repetition, each with its own unique advantages and use cases. As you delve into the intricacies of loops and iterations, you’ll discover how to harness Python’s capabilities to streamline your code and enhance its functionality.
When it comes to repeating actions in Python, the most common tools at your disposal are loops. These constructs enable you to execute a sequence of statements multiple times, based on specific conditions or a predetermined number of iterations. Python provides various types of loops, each tailored for different scenarios—from simple tasks to complex algorithms. Understanding the nuances of these loops will empower you to write cleaner, more efficient code that can adapt to a wide range of programming challenges.
Moreover, repetition in Python isn’t limited to traditional loops; you can also explore advanced techniques such as list comprehensions and generator expressions. These methods not only offer a more concise way to handle repetitive tasks but also enhance performance and readability. As we explore the various ways to implement repetition in Python, you’ll gain
Using Loops for Repetition
In Python, the most common way to repeat actions is through loops. The two primary types of loops used are `for` loops and `while` loops. Each serves different purposes, allowing for flexibility depending on the scenario.
A `for` loop iterates over a sequence (like a list, tuple, or string) or an iterable object, executing a block of code for each item. The syntax is as follows:
“`python
for item in iterable:
Code block to execute
“`
For example, to print each number in a list:
“`python
numbers = [1, 2, 3, 4, 5]
for number in numbers:
print(number)
“`
A `while` loop, on the other hand, continues executing a block of code as long as a specified condition is true. The syntax is:
“`python
while condition:
Code block to execute
“`
Here’s an example that prints numbers until it reaches 5:
“`python
count = 1
while count <= 5:
print(count)
count += 1
```
Using the `range()` Function
The `range()` function is particularly useful when you need to repeat an action a specific number of times. This function generates a sequence of numbers, which can be iterated over using a `for` loop.
The syntax of `range()` can be summarized as:
“`python
range(start, stop, step)
“`
- start: The starting value (inclusive).
- stop: The ending value (exclusive).
- step: The increment (default is 1).
For example, to repeat an action ten times, you could write:
“`python
for i in range(10):
print(“This is repetition number”, i + 1)
“`
List Comprehensions for Repetition
List comprehensions provide a concise way to create lists and can also be used for repeating actions with a return value. The syntax is:
“`python
[expression for item in iterable]
“`
For instance, to create a list of squares from 0 to 9, you can do:
“`python
squares = [x**2 for x in range(10)]
print(squares)
“`
This method not only repeats the action of squaring but also constructs a new list in a single line of code.
Using Functions to Encapsulate Repetition
Functions can encapsulate repetitive tasks, allowing you to call them multiple times without repeating code. Here’s an example function that prints a message a specified number of times:
“`python
def repeat_message(message, times):
for _ in range(times):
print(message)
repeat_message(“Hello, World!”, 3)
“`
This function enhances code reusability and maintainability by centralizing the repetition logic.
Summary Table of Loop Types
Loop Type | Use Case | Example Syntax |
---|---|---|
for loop | Iterate over items in a sequence or iterable | for item in iterable: |
while loop | Repeat as long as a condition is true | while condition: |
list comprehension | Generate lists with a single line of code | [expression for item in iterable] |
Using Loops for Repetition
In Python, loops are a primary mechanism for repeating actions. The two most common types of loops are `for` loops and `while` loops.
For Loops
A `for` loop iterates over a sequence (like a list, tuple, or string) and executes a block of code for each item in the sequence.
Example:
“`python
for i in range(5):
print(i)
“`
This code will output:
“`
0
1
2
3
4
“`
- The `range(5)` function generates a sequence of numbers from 0 to 4.
- The loop executes the `print(i)` statement five times, once for each number.
While Loops
A `while` loop continues to execute as long as a specified condition evaluates to `True`.
Example:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
This code will yield the same output as the `for` loop:
```
0
1
2
3
4
```
- The `while` loop checks the condition `count < 5` before each iteration.
- The variable `count` is incremented within the loop to avoid an infinite loop.
Using List Comprehensions
List comprehensions provide a concise way to create lists and can include repetition through iterations.
Example:
“`python
squares = [x**2 for x in range(5)]
print(squares)
“`
Output:
“`
[0, 1, 4, 9, 16]
“`
- This single line generates a list of squares for numbers from 0 to 4.
- It’s a compact and efficient way to create lists based on existing lists or ranges.
Using the `repeat` Function from `itertools`
The `itertools` module includes a `repeat` function that allows you to repeat a value indefinitely or a specified number of times.
Example:
“`python
from itertools import repeat
for item in repeat(“Python”, 3):
print(item)
“`
Output:
“`
Python
Python
Python
“`
- The `repeat` function takes a value and an optional count.
- If no count is specified, it will repeat indefinitely, which can be useful in certain contexts.
Using String Multiplication
In Python, strings can be repeated using the multiplication operator (`*`).
Example:
“`python
result = “Hello ” * 3
print(result)
“`
Output:
“`
Hello Hello Hello
“`
- This method is straightforward and effective for string repetition.
Combining Loops and Conditions
Loops can be combined with conditional statements to create more complex repetition logic.
Example:
“`python
for i in range(10):
if i % 2 == 0:
print(i)
“`
Output:
“`
0
2
4
6
8
“`
- In this example, the loop iterates through numbers 0 to 9, but only prints even numbers using the `if` statement.
Summary of Repetition Techniques
Technique | Description |
---|---|
For Loop | Iterates over a sequence or range. |
While Loop | Continues until a condition is . |
List Comprehensions | Creates lists using a concise syntax. |
`itertools.repeat` | Repeats a value indefinitely or a specified number of times. |
String Multiplication | Repeats strings using the multiplication operator. |
Loop with Conditions | Combines loops with `if` statements for selective repetition. |
Mastering Repetition in Python: Insights from Experts
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “In Python, repetition can be efficiently handled using loops, such as ‘for’ and ‘while’ loops. Understanding the structure of these loops is crucial for writing clean and effective code.”
Michael Chen (Python Developer, Tech Innovations Inc.). “Utilizing list comprehensions is another powerful way to repeat operations in Python. They provide a concise way to create lists while applying an expression to each item in an iterable.”
Sarah Thompson (Data Scientist, Analytics Hub). “For tasks requiring repetition of code blocks, defining functions is essential. This approach not only promotes code reuse but also enhances readability and maintainability in Python projects.”
Frequently Asked Questions (FAQs)
How can I repeat a block of code in Python?
You can repeat a block of code in Python using loops, such as `for` loops and `while` loops. A `for` loop iterates over a sequence, while a `while` loop continues until a specified condition is no longer true.
What is the syntax for a for loop in Python?
The syntax for a `for` loop in Python is as follows:
“`python
for variable in iterable:
code to execute
“`
This structure allows you to iterate over items in a list, tuple, string, or any iterable object.
How do I use a while loop to repeat code?
A `while` loop repeats code as long as a specified condition is true. The syntax is:
“`python
while condition:
code to execute
“`
Ensure that the condition eventually becomes to avoid infinite loops.
Can I repeat code a specific number of times in Python?
Yes, you can repeat code a specific number of times using the `range()` function in a `for` loop. For example:
“`python
for i in range(n): where n is the number of repetitions
code to execute
“`
What is the difference between a for loop and a while loop?
A `for` loop is typically used when the number of iterations is known or finite, while a `while` loop is used when the number of iterations is uncertain and depends on a condition being met.
How can I repeat a function call multiple times in Python?
You can repeat a function call by placing it inside a loop. For example:
“`python
def my_function():
function code
for i in range(n): where n is the number of times to call the function
my_function()
“`
This structure allows you to execute the function multiple times efficiently.
In Python, repetition can be achieved through various constructs, primarily utilizing loops such as ‘for’ and ‘while’. The ‘for’ loop is particularly useful for iterating over sequences like lists, tuples, and strings, allowing for concise and readable code. On the other hand, the ‘while’ loop provides flexibility by continuing to execute a block of code as long as a specified condition remains true. Both constructs are fundamental for performing repetitive tasks efficiently in Python programming.
Additionally, Python offers built-in functions such as ‘range()’ that facilitate the creation of sequences for iteration. This function can be particularly useful in ‘for’ loops to repeat actions a specific number of times. Moreover, list comprehensions provide a powerful way to generate lists through concise syntax, effectively combining iteration and conditional logic in a single line of code.
Understanding how to implement repetition in Python is essential for developing efficient algorithms and automating tasks. By mastering loops and related constructs, programmers can enhance their code’s functionality and maintainability. Ultimately, the ability to repeat actions effectively is a cornerstone of programming in Python, enabling developers to write cleaner and more efficient code.
Author Profile
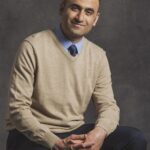
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?