How Can You Return a String in Python? A Comprehensive Guide
In the world of programming, strings are one of the fundamental building blocks that allow us to manipulate and display text. Whether you’re crafting a simple script or developing a complex application, knowing how to return a string in Python is an essential skill that can enhance your coding efficiency and effectiveness. This versatile language offers a variety of ways to work with strings, making it a favorite among developers for its readability and ease of use.
Understanding how to return strings in Python involves grasping the concept of functions, which serve as the backbone of code organization and reusability. By encapsulating logic within functions, you can streamline your code and make it more manageable. When a function returns a string, it can provide output that can be utilized elsewhere in your program, allowing for dynamic interactions and data processing.
As we delve deeper into this topic, we will explore the various methods and best practices for returning strings in Python. From basic return statements to more advanced techniques involving string formatting and manipulation, you’ll gain insights that will empower you to write cleaner, more efficient code. Whether you’re a beginner or looking to refine your skills, mastering the art of returning strings is a crucial step in your Python programming journey.
Returning a String from a Function
In Python, functions can return strings just like they return any other type of data. To return a string, you simply use the `return` statement followed by the string you wish to return. This allows you to encapsulate logic within the function and retrieve the resulting string for further use.
Here’s a basic example of a function that returns a string:
“`python
def greet(name):
return “Hello, ” + name + “!”
“`
In this example, when you call `greet(“Alice”)`, it will return the string `”Hello, Alice!”`.
Using Return Values
Once a function returns a string, you can store that string in a variable, print it, or pass it to another function. Here’s how you might use the returned value:
“`python
message = greet(“Bob”)
print(message) Output: Hello, Bob!
“`
This illustrates that the returned string can be assigned to a variable for later use.
Returning Multiple Strings
In some cases, you may want to return multiple strings from a single function. This can be achieved by returning them as a tuple. Here’s an example:
“`python
def get_full_name(first_name, last_name):
return first_name, last_name
“`
You can then unpack the returned values:
“`python
first, last = get_full_name(“John”, “Doe”)
print(first, last) Output: John Doe
“`
Table of String Return Examples
The following table summarizes different scenarios for returning strings from functions:
Function Purpose | Return Statement | Example Call | Returned String |
---|---|---|---|
Greeting | return “Hello, ” + name | greet(“Alice”) | Hello, Alice! |
Full Name | return first_name, last_name | get_full_name(“John”, “Doe”) | John, Doe (as tuple) |
Farewell | return “Goodbye, ” + name | farewell(“Bob”) | Goodbye, Bob! |
Best Practices for Returning Strings
When returning strings from functions, consider the following best practices:
- Clarity: Ensure the purpose of the returned string is clear and descriptive.
- Consistency: Maintain consistent return types in functions to avoid confusion.
- Error Handling: Implement error handling to manage cases where the input might lead to unexpected output.
By adhering to these principles, you can enhance the readability and maintainability of your code.
Returning a String from a Function
In Python, functions can return strings just like any other data type. The `return` statement is used to send a value back to the caller of the function. Here’s a simple example:
“`python
def greet(name):
return f”Hello, {name}!”
“`
In this example, the function `greet` takes a parameter `name` and returns a greeting string. You can call this function and capture the returned string as follows:
“`python
message = greet(“Alice”)
print(message) Output: Hello, Alice!
“`
Returning Multiple Strings
A function can return multiple strings by using tuples or lists. Here’s how to implement both methods:
Using a Tuple:
“`python
def get_full_name(first_name, last_name):
return first_name, last_name
“`
Using a List:
“`python
def get_names():
return [“Alice”, “Bob”, “Charlie”]
“`
You can unpack the returned values or access them directly:
“`python
first, last = get_full_name(“Alice”, “Smith”)
names_list = get_names()
print(names_list[0]) Output: Alice
“`
Returning Strings Based on Conditions
You can control the string returned by a function based on certain conditions. This is particularly useful for generating dynamic outputs. For example:
“`python
def evaluate_score(score):
if score >= 90:
return “Excellent”
elif score >= 75:
return “Good”
elif score >= 50:
return “Fair”
else:
return “Poor”
“`
This function evaluates a score and returns a string that reflects the performance level. Calling the function yields:
“`python
result = evaluate_score(85)
print(result) Output: Good
“`
Returning Formatted Strings
Python provides several ways to format strings before returning them. You can use f-strings, the `format()` method, or the `%` operator. Here’s how to use each method:
Using f-strings:
“`python
def format_message(name, age):
return f”{name} is {age} years old.”
“`
Using the `format()` method:
“`python
def format_message(name, age):
return “{} is {} years old.”.format(name, age)
“`
Using the `%` operator:
“`python
def format_message(name, age):
return “%s is %d years old.” % (name, age)
“`
All three functions will return strings in the same format when called, for example:
“`python
print(format_message(“Alice”, 30)) Output: Alice is 30 years old.
“`
Returning Strings from Class Methods
In object-oriented programming, classes can also have methods that return strings. Here’s an example of a class with a method that returns a string:
“`python
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
return f”{self.name} says hello!”
“`
When you create an instance of the class and call the `speak` method, it returns a string:
“`python
dog = Animal(“Buddy”)
print(dog.speak()) Output: Buddy says hello!
“`
Returning Strings in a JSON Response
For web applications, functions often return strings in JSON format. Utilizing the `json` library facilitates this process. For example:
“`python
import json
def create_response(data):
return json.dumps({“message”: data})
“`
This function converts a Python dictionary to a JSON string. When invoked:
“`python
response = create_response(“Operation successful”)
print(response) Output: {“message”: “Operation successful”}
“`
Utilizing these methods allows for flexible string handling and output in various programming contexts.
Expert Insights on Returning Strings in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Returning a string in Python is a fundamental concept that can be achieved using the `return` statement within a function. It is essential to ensure that the function is defined correctly to encapsulate the logic that generates the string.”
James Liu (Python Developer, CodeCraft Solutions). “Utilizing the `return` keyword is not just about sending a string back; it is about understanding the flow of data within your program. Properly returning strings can enhance code readability and maintainability.”
Sarah Thompson (Technical Writer, Python Programming Journal). “When writing functions that return strings, it is crucial to consider the context in which the string will be used. Clear documentation and examples can significantly aid users in understanding how to effectively implement string returns in their own code.”
Frequently Asked Questions (FAQs)
How do I return a string from a function in Python?
To return a string from a function in Python, define the function using the `def` keyword, and use the `return` statement followed by the string you want to return. For example:
“`python
def greet():
return “Hello, World!”
“`
Can I return multiple strings from a function in Python?
Yes, you can return multiple strings from a function by using a tuple or a list. For instance:
“`python
def get_names():
return “Alice”, “Bob” returns a tuple
“`
What is the difference between returning a string and printing a string in Python?
Returning a string sends the value back to the caller of the function, while printing a string outputs it directly to the console. Use `return` when you need to use the string later, and `print` when you want to display it immediately.
Can I return a string that is generated dynamically in Python?
Yes, you can generate a string dynamically within a function and return it. For example:
“`python
def create_message(name):
return f”Hello, {name}!”
“`
What happens if I forget to use the return statement in a function?
If you forget to use the `return` statement, the function will return `None` by default. This means that no value will be passed back to the caller, which may lead to unexpected behavior if a return value is expected.
Is it possible to return a string from a lambda function in Python?
Yes, a lambda function can return a string. A lambda function is defined using the `lambda` keyword followed by parameters and an expression. For example:
“`python
greet = lambda: “Hello, World!”
“`
In Python, returning a string from a function is a straightforward process that involves defining a function using the `def` keyword and utilizing the `return` statement to send a string value back to the caller. This allows for modular code design, enabling developers to encapsulate functionality and reuse code efficiently. The syntax is simple: define the function, process any necessary logic, and then return the desired string result.
One of the key advantages of returning strings from functions is the ability to create dynamic and flexible applications. By returning strings, functions can provide feedback, generate messages, or produce formatted outputs based on input parameters. This capability is essential for tasks such as data manipulation, user interaction, and generating reports, making it a fundamental aspect of Python programming.
In summary, understanding how to return a string in Python is crucial for effective coding practices. It enhances code readability and maintainability while promoting the reuse of logic. As developers become more proficient in utilizing return statements, they can create more sophisticated and user-friendly applications that leverage the power of string manipulation and processing.
Author Profile
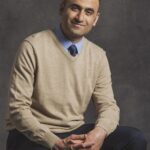
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?