How Can You Run a Perl Script in Linux?
In the world of programming and scripting, Perl stands out as a versatile and powerful language, particularly favored for tasks such as text processing, system administration, and web development. Whether you’re a seasoned developer or a curious newcomer, knowing how to run a Perl script in Linux can open up a realm of possibilities for automating tasks and enhancing productivity. With its rich set of features and extensive libraries, Perl enables users to tackle complex problems with elegant solutions.
Running a Perl script in a Linux environment is a straightforward process, but it involves understanding a few key concepts. From ensuring that Perl is installed on your system to setting the appropriate permissions for your script, there are essential steps to follow. Additionally, knowing how to execute your script from the command line and handle any potential errors will empower you to harness the full potential of Perl in your projects.
As you delve deeper into the mechanics of running Perl scripts, you’ll discover the nuances that make this language both powerful and user-friendly. Whether you’re looking to automate mundane tasks, process large datasets, or simply explore the capabilities of Perl, mastering the execution of scripts in Linux is the first step toward unlocking a wealth of opportunities in your programming journey.
Prerequisites for Running a Perl Script
Before executing a Perl script in a Linux environment, ensure that the following prerequisites are met:
- Perl Installation: Confirm that Perl is installed on your system. Most Linux distributions come with Perl pre-installed. You can check the installation by running:
“`bash
perl -v
“`
- Script Permissions: The script file must have the appropriate permissions to be executed. You can set the executable permission using:
“`bash
chmod +x your_script.pl
“`
Running a Perl Script
There are several methods to run a Perl script in Linux. The most common approaches include:
- Using the Perl Interpreter Directly:
You can execute the script directly through the Perl interpreter by specifying the script’s path. For example:
“`bash
perl /path/to/your_script.pl
“`
- Executing a Script with Shebang:
If your script includes a shebang (`!`) line at the top, you can run it directly. Ensure that the first line of your script is:
“`perl
!/usr/bin/perl
“`
After setting the executable permission, execute the script as follows:
“`bash
/path/to/your_script.pl
“`
- Running with Command-Line Options:
Perl scripts can accept command-line arguments. You can pass these arguments when running the script:
“`bash
perl /path/to/your_script.pl arg1 arg2
“`
Common Issues and Troubleshooting
When running Perl scripts, you may encounter common issues. Here are some troubleshooting tips:
- Script Not Found: Ensure the path to the script is correct. Use absolute paths when possible to avoid confusion.
- Permission Denied: If you receive a permission error, verify that the script has executable permissions (use `chmod +x`).
- Syntax Errors: If your script has syntax errors, Perl will output the line number and error message. Review your code for common mistakes such as missing semicolons or unmatched brackets.
Error Type | Possible Cause | Solution |
---|---|---|
File Not Found | Incorrect path | Verify the file path |
Permission Denied | Insufficient permissions | Change permissions using chmod |
Syntax Error | Code mistakes | Check for typos and structure |
Best Practices for Writing and Running Perl Scripts
To ensure smooth execution and maintainability of your Perl scripts, consider the following best practices:
- Use Descriptive Names: Name your scripts and variables clearly to reflect their functionality.
- Comment Your Code: Add comments to explain complex sections of your code, which aids in readability and future maintenance.
- Test Your Scripts: Before deployment, test your scripts thoroughly in a controlled environment to catch any errors or unexpected behavior.
- Version Control: Utilize version control systems like Git to track changes in your scripts over time, allowing for easier collaboration and rollback if necessary.
Prerequisites for Running a Perl Script
To effectively run a Perl script in a Linux environment, ensure that the following prerequisites are met:
- Perl Installation: Confirm that Perl is installed on your system. You can check this by running:
“`bash
perl -v
“`
If Perl is installed, this command will return the version information.
- Script Permissions: Ensure that the script file has the appropriate execute permissions. You can modify the permissions with:
“`bash
chmod +x script.pl
“`
- Text Editor: Familiarity with a text editor (e.g., `nano`, `vim`, or `gedit`) to create or edit your Perl script.
Creating a Perl Script
To create a simple Perl script, follow these steps:
- Open a terminal.
- Use your preferred text editor to create a new file:
“`bash
nano myscript.pl
“`
- Add the following code to the file:
“`perl
!/usr/bin/perl
use strict;
use warnings;
print “Hello, World!\n”;
“`
- Save and exit the editor (in `nano`, press `CTRL + X`, then `Y`, and `Enter`).
Running a Perl Script
There are multiple methods to run a Perl script in Linux:
- Direct Execution:
If the script is executable, you can run it directly from the terminal:
“`bash
./myscript.pl
“`
- Using the Perl Interpreter:
If the script lacks executable permissions or you prefer to specify the interpreter, use:
“`bash
perl myscript.pl
“`
Passing Arguments to a Perl Script
To pass command-line arguments to your Perl script, you can access them using the `@ARGV` array. For example, modify your script as follows:
“`perl
!/usr/bin/perl
use strict;
use warnings;
my $name = $ARGV[0] // ‘World’;
print “Hello, $name!\n”;
“`
You can run this script with an argument like this:
“`bash
perl myscript.pl Alice
“`
This would output: `Hello, Alice!`
Debugging Perl Scripts
If you encounter issues when running your Perl script, consider the following debugging techniques:
- Use Warnings and Strict: Always include `use strict;` and `use warnings;` at the beginning of your scripts to catch common mistakes.
- Print Debugging: Insert print statements to output variable values at various points in your script.
- Perl Debugger: Utilize the built-in debugger by running:
“`bash
perl -d myscript.pl
“`
This will allow you to step through your script interactively.
Common Perl Shebang Line
The shebang line at the top of your script indicates which interpreter to use. Here’s a common shebang line for Perl scripts:
“`perl
!/usr/bin/perl
“`
You may also find this alternative, which automatically uses the system’s Perl interpreter:
“`perl
!/usr/bin/env perl
“`
This method is particularly useful in environments where Perl might not be located in the same directory across different systems.
Expert Insights on Running Perl Scripts in Linux
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a Perl script in Linux, ensure that you have Perl installed on your system. You can check this by typing ‘perl -v’ in the terminal. Once confirmed, navigate to the directory containing your script and execute it using the command ‘perl scriptname.pl’.”
Michael Thompson (Linux Systems Administrator, Open Source Solutions). “It’s crucial to set the correct permissions for your Perl script. Use ‘chmod +x scriptname.pl’ to make it executable. After that, you can run it directly with ‘./scriptname.pl’ if you include the shebang line at the top of your script.”
Sarah Lee (DevOps Engineer, CloudTech Corp.). “For more complex Perl scripts, consider using a virtual environment or a version manager like Perlbrew. This allows you to manage dependencies and versions effectively, ensuring that your script runs smoothly across different Linux distributions.”
Frequently Asked Questions (FAQs)
How do I check if Perl is installed on my Linux system?
You can check if Perl is installed by running the command `perl -v` in the terminal. This command will display the version of Perl if it is installed.
What is the command to run a Perl script in Linux?
To run a Perl script, use the command `perl script_name.pl`, replacing `script_name.pl` with the actual name of your Perl script file.
Do I need to set permissions to execute a Perl script?
Yes, you need to set the execute permission on the script file. Use the command `chmod +x script_name.pl` to make it executable.
Can I run a Perl script without specifying ‘perl’ in the command?
Yes, if the script has a shebang line (e.g., `!/usr/bin/perl`) at the top and has executable permissions, you can run it directly by typing `./script_name.pl`.
What should I do if I encounter a “Permission denied” error?
If you encounter a “Permission denied” error, ensure that the script has the correct permissions set. Use `chmod +x script_name.pl` to grant execute permissions.
How can I pass arguments to a Perl script in Linux?
You can pass arguments by including them after the script name in the command line, like this: `perl script_name.pl arg1 arg2`. Inside the script, access these arguments using the `@ARGV` array.
Running a Perl script in Linux is a straightforward process that involves a few essential steps. First, ensure that Perl is installed on your system, which is typically pre-installed on most Linux distributions. You can verify this by executing the command `perl -v` in the terminal. If Perl is not installed, it can be easily added using the package manager specific to your distribution.
Once you have confirmed that Perl is installed, the next step is to create your Perl script. This can be done using any text editor, such as `nano`, `vim`, or `gedit`. It is crucial to begin your script with the shebang line `!/usr/bin/perl`, which indicates the script should be executed using the Perl interpreter. After writing your script, save it with a `.pl` file extension to signify that it is a Perl script.
To execute the script, you must set the appropriate permissions to make it executable. This can be accomplished by running the command `chmod +x your_script.pl`. After setting the permissions, you can run the script directly by typing `./your_script.pl` in the terminal. Alternatively, you can execute it by explicitly invoking Perl with the command `perl your_script.pl`, which does
Author Profile
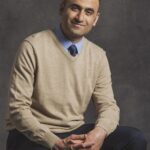
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?