How Can I Run a Python File from the Terminal?
In the world of programming, Python stands out as one of the most versatile and user-friendly languages, making it a favorite among beginners and seasoned developers alike. Whether you’re automating mundane tasks, developing web applications, or diving into data science, knowing how to execute your Python scripts from the terminal is an essential skill. This seemingly simple action opens up a realm of possibilities, allowing you to leverage the full power of your code directly from the command line.
Running a Python file from the terminal may seem daunting at first, especially if you’re accustomed to graphical interfaces. However, mastering this process not only enhances your coding efficiency but also deepens your understanding of how programming environments operate. By executing scripts in the terminal, you gain immediate feedback and control over your code, making it easier to troubleshoot and refine your projects.
In this article, we’ll explore the straightforward steps involved in running a Python file from the terminal, along with some tips and tricks to streamline your workflow. Whether you’re looking to run simple scripts or complex applications, understanding how to navigate the terminal is a crucial step in your programming journey. Get ready to unlock the full potential of your Python projects with just a few keystrokes!
Running Python Files from the Terminal
To execute a Python file from the terminal, you need to follow a series of straightforward steps. The most common method involves using the Python interpreter directly.
First, ensure you have Python installed on your system. You can verify this by running the following command in your terminal:
“`bash
python –version
“`
or, for Python 3 specifically:
“`bash
python3 –version
“`
If Python is installed, the terminal will display the version number. If not, you will need to install it from the official Python website or through a package manager.
Once you confirm that Python is installed, you can run your Python script using the following commands:
- Open your terminal.
- Navigate to the directory containing your Python file using the `cd` command. For example:
“`bash
cd path/to/your/directory
“`
- Run your Python file by typing:
“`bash
python filename.py
“`
or, if you’re using Python 3:
“`bash
python3 filename.py
“`
Additional Options
You may also use various flags to modify the execution of your Python scripts. Here are some commonly used options:
- `-m` : Allows you to run a module as a script.
- `-c` : Allows you to pass a command as a string to the Python interpreter.
- `-i` : Launches an interactive session after executing the script.
Example Command Usage
Command | Description |
---|---|
`python filename.py` | Runs the specified Python script. |
`python3 -m module_name` | Executes a module as a script. |
`python -c “print(‘Hello World!’)”` | Executes the provided command string. |
Troubleshooting Common Issues
While running a Python file, you may encounter some common issues. Here are a few troubleshooting tips:
- File Not Found Error: Ensure you are in the correct directory and the filename is spelled correctly.
- Permission Denied: Check file permissions; you may need to change them using `chmod`.
- Python Version Issues: Ensure you are using the correct version of Python that your script is compatible with.
By following these steps and utilizing the provided options, you can efficiently run your Python scripts from the terminal, enhancing your workflow and productivity.
Using the Python Interpreter
To run a Python file from the terminal, you can utilize the Python interpreter. This method is straightforward and universally applicable across different operating systems.
- Open your terminal or command prompt.
- Navigate to the directory containing your Python file using the `cd` command. For example:
“`bash
cd path/to/your/file
“`
- Once in the correct directory, execute the following command:
“`bash
python filename.py
“`
or, if you are using Python 3 specifically:
“`bash
python3 filename.py
“`
Ensure that `filename.py` is replaced with the actual name of your Python file.
Setting Up Environment Variables
In some cases, you may want to run Python files without specifying the `python` or `python3` command. This can be achieved by adding Python to your system’s PATH environment variable.
For Windows:
- Search for “Environment Variables” in your start menu.
- Select “Edit the system environment variables.”
- Click on “Environment Variables.”
- Under “System variables,” find and select the “Path” variable, then click “Edit.”
- Add the path to your Python installation and its Scripts folder (e.g., `C:\Python39;C:\Python39\Scripts`).
- Click OK to save changes.
For macOS and Linux:
- Open a terminal.
- Edit your shell configuration file (e.g., `.bashrc`, `.bash_profile`, or `.zshrc`) using a text editor.
“`bash
nano ~/.bashrc
“`
- Add the following line to export the PATH:
“`bash
export PATH=”$PATH:/usr/local/bin/python3″
“`
- Save the changes and apply them:
“`bash
source ~/.bashrc
“`
Running Python Scripts in Background
If you want to run your Python script in the background, especially on Unix-like systems, you can append an ampersand (`&`) to the command.
“`bash
python filename.py &
“`
This allows you to continue using the terminal for other commands while the script runs.
Executing Python Scripts with Arguments
If your Python script requires command-line arguments, you can pass them directly after the filename.
“`bash
python filename.py arg1 arg2
“`
In your script, you can access these arguments using the `sys` module:
“`python
import sys
arg1 = sys.argv[1]
arg2 = sys.argv[2]
“`
This allows for dynamic input processing when executing the script.
Using Virtual Environments
For projects that rely on specific packages or dependencies, consider using a virtual environment. This isolates your project’s environment, preventing conflicts.
- Create a virtual environment:
“`bash
python -m venv myenv
“`
- Activate the virtual environment:
- Windows:
“`bash
myenv\Scripts\activate
“`
- macOS/Linux:
“`bash
source myenv/bin/activate
“`
- Run your Python file as usual:
“`bash
python filename.py
“`
Common Errors and Troubleshooting
When running Python files from the terminal, you may encounter several common issues:
Error Message | Possible Cause | Solution |
---|---|---|
`python: command not found` | Python is not installed or not in PATH. | Install Python or add it to your PATH. |
`SyntaxError` | Typo or syntax issue in your script. | Review and correct the code. |
`ModuleNotFoundError` | Missing external libraries. | Install the required packages using pip. |
By following these guidelines, you can effectively run Python scripts from the terminal while managing environments and arguments efficiently.
Expert Insights on Running Python Files from the Terminal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run a Python file from the terminal, you simply need to navigate to the directory containing your script using the ‘cd’ command and then execute it by typing ‘python filename.py’ or ‘python3 filename.py’ depending on your Python installation. This straightforward process is essential for efficient coding and testing.”
Mark Thompson (Lead Python Developer, CodeCrafters). “Understanding how to run a Python file from the terminal is crucial for any developer. Ensure that your terminal is configured correctly with the appropriate Python version. Utilizing virtual environments can also help manage dependencies effectively when executing scripts.”
Linda Garcia (Technical Writer, Python Programming Journal). “Running Python scripts from the terminal not only enhances productivity but also provides immediate feedback on your code. It’s important to familiarize yourself with command-line arguments and how they can be passed to your script for more dynamic execution.”
Frequently Asked Questions (FAQs)
How do I run a Python file from the terminal?
To run a Python file from the terminal, navigate to the directory containing the file using the `cd` command, then execute the file by typing `python filename.py` or `python3 filename.py`, depending on your Python installation.
What should I do if I receive a “command not found” error?
If you encounter a “command not found” error, ensure that Python is installed on your system and that the installation path is added to your system’s environment variables. You can verify the installation by typing `python –version` or `python3 –version`.
Can I run a Python file without specifying the Python version?
Yes, if the Python interpreter is set as the default version in your environment, you can simply type `python filename.py`. Otherwise, you must specify the version, such as `python3 filename.py`.
What if my Python file requires command-line arguments?
To run a Python file with command-line arguments, use the syntax `python filename.py arg1 arg2`, replacing `arg1` and `arg2` with the actual arguments your script requires.
How can I run a Python script in the background?
To run a Python script in the background, append an ampersand (`&`) at the end of your command, like this: `python filename.py &`. This allows the script to execute while freeing up the terminal for other commands.
Is there a way to run a Python script interactively from the terminal?
Yes, you can run a Python script interactively by using the command `python -i filename.py`. This allows you to execute the script and then enter commands in the Python shell afterward.
Running a Python file from the terminal is a straightforward process that can significantly enhance your productivity and streamline your workflow. To execute a Python script, you typically need to open your terminal or command prompt, navigate to the directory where your Python file is located, and then use the appropriate command to run it. The command usually takes the form of `python filename.py` or `python3 filename.py`, depending on your system configuration and the version of Python you are using.
It is essential to ensure that Python is correctly installed on your machine and that the environment variables are set up properly, especially for Windows users. Additionally, using a virtual environment can help manage dependencies and avoid conflicts with other projects. Familiarity with command-line navigation and basic terminal commands is also beneficial for efficiently running Python scripts.
In summary, mastering the ability to run Python files from the terminal not only empowers developers to execute scripts quickly but also fosters a deeper understanding of the programming environment. By following the correct procedures and utilizing best practices, such as working within virtual environments, users can maximize their efficiency and maintain organized project structures.
Author Profile
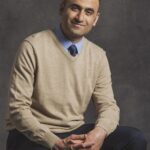
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?