How Can You Run Python Code Every Minute with Precision?
In the fast-paced world of programming, the ability to execute code at precise intervals can be a game-changer. Whether you’re monitoring system performance, collecting data from APIs, or automating repetitive tasks, running code every minute can streamline your workflow and enhance efficiency. Python, with its robust libraries and straightforward syntax, makes it easy to implement this functionality. But how exactly can you ensure that your code runs at exactly one-minute intervals?
In this article, we will explore various methods to achieve this in Python, catering to both beginners and experienced developers. From leveraging built-in modules to utilizing third-party libraries, we’ll guide you through the different approaches you can take to set up your code for consistent execution. Along the way, we’ll discuss the importance of precision in timing and how to handle potential pitfalls that may arise when scheduling tasks.
As we delve deeper into the topic, you’ll discover practical examples and best practices that will empower you to run your code every minute with confidence. Whether you’re automating a simple task or developing a more complex application, mastering this skill will undoubtedly elevate your programming prowess. Get ready to unlock the potential of time-based automation in Python!
Using the `time` Module
To execute code every minute, the `time` module in Python is a straightforward solution. You can use the `sleep` function to pause execution for a specified duration. Here’s a simple example demonstrating this approach:
python
import time
while True:
# Your code here
print(“This code runs every minute.”)
time.sleep(60) # Sleep for 60 seconds
This method is simple to implement and works well for scripts that do not require precise timing.
Using the `schedule` Library
For more advanced scheduling, the `schedule` library can be used. It allows for more flexibility and readability, making it easier to schedule tasks at different intervals. You can install it via pip if it’s not already available in your environment:
bash
pip install schedule
Here’s how to use it:
python
import schedule
import time
def job():
print(“This code runs every minute.”)
# Schedule the job every minute
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1) # Sleep for 1 second
This approach is particularly useful when you want to manage multiple jobs or more complex scheduling logic.
Using `threading.Timer`
Another method is using `threading.Timer`, which can be set to call a function after a specified delay. This method is more suitable when you need to run tasks in the background without blocking the main thread.
Here’s an implementation:
python
import threading
def job():
print(“This code runs every minute.”)
threading.Timer(60, job).start() # Schedule the next execution
job() # Start the first execution
This method is effective for scenarios where you want your main program to remain responsive while executing tasks at regular intervals.
Comparison Table of Methods
Method | Pros | Cons |
---|---|---|
time.sleep() | Simple to implement | Blocking; not suitable for concurrent tasks |
schedule Library | Flexible; easy to read and manage | Requires external library |
threading.Timer | Non-blocking; allows for background processing | More complex; requires careful management of threads |
Each method has its own advantages and disadvantages, and the choice depends on the specific requirements of your application.
Using the `time` Module
To execute code every minute precisely, one of the simplest methods is to utilize the `time` module in Python. This module allows you to control the execution time of your code effectively. Below is a basic structure for running a function every minute:
python
import time
def job():
print(“Executing job…”)
while True:
job()
time.sleep(60) # Sleep for 60 seconds
In this example:
- The `job()` function contains the code that needs to be executed.
- The `while True` loop ensures continuous execution.
- `time.sleep(60)` pauses the execution for one minute between calls.
Using the `schedule` Library
For more advanced scheduling, the `schedule` library provides a user-friendly interface. First, install the library if it is not already available:
bash
pip install schedule
Here is an example of how to use it:
python
import schedule
import time
def job():
print(“Executing job…”)
schedule.every(1).minutes.do(job)
while True:
schedule.run_pending()
time.sleep(1) # Sleep to prevent excessive CPU usage
This method offers the following benefits:
- Readability: The code is more intuitive and easier to understand.
- Flexibility: You can easily adjust the timing and frequency of jobs.
Using Threads for Non-blocking Execution
If you want to run a background job every minute without blocking the main thread, consider using the `threading` module. Here’s how to implement this:
python
import threading
import time
def job():
print(“Executing job…”)
threading.Timer(60, job).start() # Schedule the job again after 60 seconds
job() # Initial call to start the job
In this implementation:
- The `job()` function schedules itself to run again after 60 seconds using `threading.Timer`.
- This approach allows your program to remain responsive while the job runs in the background.
Using `APScheduler` for Advanced Scheduling
For more complex scheduling needs, consider using `APScheduler`. To install it, use:
bash
pip install APScheduler
Here’s an example of how to set up a job that runs every minute:
python
from apscheduler.schedulers.blocking import BlockingScheduler
def job():
print(“Executing job…”)
scheduler = BlockingScheduler()
scheduler.add_job(job, ‘interval’, minutes=1)
scheduler.start()
Advantages of using `APScheduler` include:
- Persistence: It can save job states to a database.
- Job Management: You can pause, resume, or remove jobs easily.
Comparative Summary of Methods
Method | Simplicity | Flexibility | Background Execution |
---|---|---|---|
`time.sleep` | Simple | Low | No |
`schedule` | Moderate | Moderate | No |
`threading.Timer` | Moderate | Low | Yes |
`APScheduler` | Complex | High | Yes |
Each method has its own strengths and is suitable for different scenarios depending on the complexity and requirements of the task at hand.
Expert Insights on Running Code Every Minute in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To run code every minute in Python, utilizing the `schedule` library is highly effective. This library allows for simple scheduling of tasks with a clear syntax, making it easy to implement and manage recurring jobs.”
Michael Chen (Python Developer, CodeCrafters). “For precise timing, leveraging the `time` module along with a while loop can be beneficial. By using `time.sleep(60)`, you can ensure that your code executes every minute, although it is crucial to account for execution time to maintain accuracy.”
Lisa Patel (Data Scientist, AI Solutions Group). “If your application requires more sophisticated scheduling, consider using `APScheduler`. This library provides advanced features such as job persistence and can handle complex scheduling scenarios, making it ideal for production environments.”
Frequently Asked Questions (FAQs)
How can I run a Python script every minute?
You can use the `schedule` library to run a Python script every minute. First, install the library using `pip install schedule`, then create a job that executes your function and call `schedule.run_pending()` in a loop.
Is there a built-in way to run code at regular intervals in Python?
Python does not have a built-in method for scheduling tasks at regular intervals. However, you can use the `time.sleep()` function in a loop to achieve similar functionality, though this is less efficient than using a scheduling library.
What is the best way to ensure precision when running code every minute?
For precise execution, consider using the `schedule` library along with `time` to calculate the time taken by the task and adjust the sleep time accordingly. This helps maintain consistent intervals.
Can I use threading to run code every minute in Python?
Yes, you can use the `threading` module to run a function in a separate thread that executes every minute. This allows your main program to continue running without blocking.
What are the limitations of using `time.sleep()` for scheduling tasks?
Using `time.sleep()` can lead to drift over time, especially if the execution time of the task varies. It does not account for the time taken by the task itself, which can result in less accurate scheduling.
Are there alternatives to the `schedule` library for running code periodically in Python?
Yes, alternatives include `APScheduler`, which provides a more robust scheduling framework, and using cron jobs on Unix-like systems or Task Scheduler on Windows for executing Python scripts at regular intervals.
Running code at precise intervals, such as every minute, is a common requirement in various programming tasks. In Python, there are several approaches to achieve this, including the use of the `time` module, the `schedule` library, and threading techniques. Each method has its own advantages and can be chosen based on the specific needs of the application, such as simplicity, accuracy, or the ability to handle concurrent tasks.
The most straightforward method involves using a loop combined with `time.sleep()`, which pauses execution for a specified duration. However, this approach may lead to slight inaccuracies due to the time taken to execute the code within the loop. For more precise scheduling, libraries like `schedule` provide a higher-level interface that can manage job execution with better accuracy and less manual overhead.
For applications that require multitasking, using threading or asynchronous programming can be beneficial. This allows the main program to continue running while executing tasks at set intervals. It is essential to consider the nature of the tasks being performed and the potential impact on performance when selecting the appropriate method for running code every minute.
Author Profile
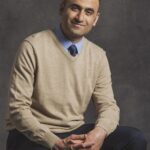
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?