How Can You Effectively Run Python in the Terminal?
Introduction
In the ever-evolving world of programming, Python has emerged as one of the most popular languages due to its simplicity and versatility. Whether you’re a seasoned developer or a curious beginner, mastering the art of running Python in the terminal is a crucial skill that opens the door to countless possibilities. Imagine having the power to execute scripts, automate tasks, and interact with your code seamlessly—all from your terminal. In this article, we’ll guide you through the essentials of running Python in the terminal, empowering you to harness the full potential of this dynamic language.
To embark on your Python journey in the terminal, it’s essential to understand the basic setup and commands that will serve as your foundation. The terminal, often overlooked by those who prefer graphical interfaces, is a powerful tool that allows you to execute commands directly and interact with your operating system. By learning how to run Python scripts and commands in this environment, you’ll gain greater control over your programming projects and enhance your workflow efficiency.
As you delve deeper into the world of terminal-based Python programming, you’ll discover various techniques for running scripts, managing virtual environments, and troubleshooting common issues. This knowledge not only boosts your confidence as a programmer but also equips you with the skills to tackle more complex projects. So, whether you’re looking to streamline your
Setting Up Your Environment
To effectively run Python in the terminal, first ensure that Python is installed on your system. You can verify this by executing a simple command in your terminal.
- For Windows, open Command Prompt and type:
python –version
- For macOS or Linux, open the terminal and type:
python3 –version
If Python is installed, you will see the version number. If not, you can download and install it from the official Python website.
Running Python Scripts
Once Python is installed, you can run Python scripts directly from the terminal. Here’s how to do it:
- Create a Python Script: Open a text editor and write your Python code. Save the file with a `.py` extension, for example, `myscript.py`.
- Navigate to the Script Location: Use the `cd` command to change the directory to where your script is located. For example:
cd path/to/your/script
- Execute the Script: You can run your script using the following commands:
- For Windows:
python myscript.py
- For macOS or Linux:
python3 myscript.py
This will execute the script, and any output will be displayed in the terminal.
Interactive Python Shell
You can also run Python in an interactive mode, which allows you to execute Python commands one at a time. To access the interactive shell:
- Type `python` or `python3` in the terminal and press Enter. You will see a prompt (>>>) where you can start typing Python code directly.
To exit the interactive shell, simply type `exit()` or press `Ctrl + D`.
Using Virtual Environments
For managing dependencies in different projects, using virtual environments is recommended. This keeps project dependencies isolated. Here’s how to set up a virtual environment:
- Install `virtualenv` (if not already installed):
pip install virtualenv
- Create a Virtual Environment:
virtualenv myenv
- Activate the Virtual Environment:
- For Windows:
myenv\Scripts\activate
- For macOS or Linux:
source myenv/bin/activate
- Run Your Python Script in the activated environment as previously described.
When you finish working in the virtual environment, you can deactivate it by simply typing `deactivate`.
Command | Description |
---|---|
python –version | Check Python version on Windows |
python3 –version | Check Python version on macOS/Linux |
python myscript.py | Run Python script on Windows |
python3 myscript.py | Run Python script on macOS/Linux |
virtualenv myenv | Create a virtual environment |
source myenv/bin/activate | Activate virtual environment on macOS/Linux |
myenv\Scripts\activate | Activate virtual environment on Windows |
Setting Up Python Environment in Terminal
To run Python in the terminal, you first need to ensure that Python is installed on your system. Follow these steps to set up your environment:
- Check Python Installation:
- Open your terminal.
- Type `python –version` or `python3 –version` and press Enter. This command will display the installed version of Python.
- If Python is not installed, download it from the [official Python website](https://www.python.org/downloads/) and follow the installation instructions for your operating system.
- Adding Python to PATH:
- During installation, ensure that the option to add Python to your system’s PATH is checked. This allows you to run Python commands directly from the terminal.
Running Python Scripts
Once Python is installed and properly configured, you can run Python scripts using the terminal. Here are the methods to execute scripts:
- Direct Command:
- Open the terminal and navigate to the directory where your Python script is located using the `cd` command. For example:
bash
cd path/to/your/script
- Execute the script by typing:
bash
python script_name.py
or for Python 3:
bash
python3 script_name.py
- Using the Python Interpreter:
- You can also run Python interactively by simply typing `python` or `python3` in the terminal:
bash
python
- This opens an interactive shell where you can execute Python commands directly.
Creating and Running Virtual Environments
Using virtual environments is a best practice for managing dependencies in Python projects. Here’s how to create and activate a virtual environment:
- Create a Virtual Environment:
- Navigate to your project directory and run:
bash
python -m venv env_name
Replace `env_name` with your desired environment name.
- Activate the Virtual Environment:
- On macOS/Linux:
bash
source env_name/bin/activate
- On Windows:
bash
.\env_name\Scripts\activate
- Deactivate the Virtual Environment:
- To exit the virtual environment, simply type:
bash
deactivate
Using Command-Line Arguments
You can pass command-line arguments to your Python scripts. Here’s how to do it:
- Accessing Arguments in Python:
- Use the `sys` module to access command-line arguments:
python
import sys
for arg in sys.argv:
print(arg)
- The first element, `sys.argv[0]`, is the script name, and subsequent elements are the arguments passed.
- Running with Arguments:
- In your terminal, run your script with arguments:
bash
python script_name.py arg1 arg2
Common Python Terminal Commands
Here are some common commands and their descriptions:
Command | Description |
---|---|
`python` or `python3` | Starts the Python interpreter |
`python script_name.py` | Runs a Python script |
`pip install package_name` | Installs a Python package |
`pip list` | Lists installed packages |
`pip freeze` | Outputs installed packages in requirements format |
These commands facilitate efficient work within the terminal environment, allowing for effective Python programming practices.
Expert Insights on Running Python in Terminal
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Running Python in the terminal is a fundamental skill for developers, as it allows for quick script execution and testing. To start, ensure Python is installed on your system and accessible in your terminal. You can verify this by typing ‘python –version’ or ‘python3 –version’ to check the installed version.
Michael Chen (Lead Developer, CodeCraft Solutions). When executing Python scripts in the terminal, it’s crucial to navigate to the directory containing your script using the ‘cd’ command. Once there, simply type ‘python script_name.py’ or ‘python3 script_name.py’ to run your program. This method is efficient for debugging and testing code snippets in real-time.
Sarah Thompson (Technical Writer, Programming Today). For those new to running Python in the terminal, I recommend creating a virtual environment first. This helps manage dependencies and avoid conflicts. Use ‘python -m venv myenv’ to create a virtual environment, activate it, and then run your scripts within this isolated space for better control over your project.
Frequently Asked Questions (FAQs)
How do I open the terminal on my computer?
To open the terminal, you can typically search for “Terminal” in your operating system’s search bar. On Windows, you can use Command Prompt or PowerShell. On macOS, you can find it in the Utilities folder within Applications. On Linux, it is usually found in the applications menu.
What command do I use to run a Python script in the terminal?
To run a Python script, navigate to the directory containing the script using the `cd` command, then type `python script_name.py` or `python3 script_name.py`, depending on your Python installation.
How can I check if Python is installed on my system?
You can check if Python is installed by typing `python –version` or `python3 –version` in the terminal. If Python is installed, it will display the version number.
What should I do if I encounter a ‘command not found’ error?
If you encounter a ‘command not found’ error, ensure that Python is installed correctly and that its installation path is added to your system’s PATH environment variable.
Can I run Python interactively in the terminal?
Yes, you can run Python interactively by simply typing `python` or `python3` in the terminal. This will open the Python interpreter, allowing you to execute Python commands directly.
How do I exit the Python interactive shell?
To exit the Python interactive shell, you can type `exit()` or press `Ctrl + D` on Unix/Linux or `Ctrl + Z` followed by `Enter` on Windows.
Running Python in the terminal is a straightforward process that allows users to execute Python scripts or commands directly from the command line interface. To begin, it is essential to have Python installed on your system, which can be verified by entering the command `python –version` or `python3 –version` in the terminal. If Python is installed, the terminal will display the version number. If not, users will need to download and install Python from the official website.
Once Python is installed, users can run scripts by navigating to the directory containing the script using the `cd` command. The script can then be executed by typing `python script_name.py` or `python3 script_name.py`, depending on the version installed. Additionally, users can enter the Python interactive shell by simply typing `python` or `python3`, allowing for immediate execution of Python commands and testing of code snippets.
For enhanced usability, it is advisable to familiarize oneself with terminal commands and options that can aid in script execution. This includes understanding how to use virtual environments for managing dependencies and ensuring that the correct version of Python is being used. By mastering these skills, users can effectively leverage the terminal to run Python programs efficiently and effectively.
Author Profile
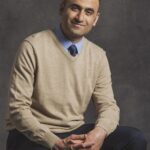
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?