How Can You Run a Python Script on Linux?
Introduction
In the world of programming, Python has emerged as one of the most versatile and widely-used languages, beloved by beginners and seasoned developers alike. Whether you’re automating mundane tasks, developing web applications, or analyzing data, knowing how to run a Python script on a Linux system is an essential skill that can enhance your productivity and streamline your workflow. Linux, known for its stability and flexibility, provides a powerful environment for executing Python scripts, making it a favorite among developers.
Understanding how to effectively run Python scripts in Linux opens up a realm of possibilities for both personal and professional projects. From the command line to integrated development environments, there are various methods to execute your code, each with its own set of advantages. Whether you’re working with simple scripts or complex applications, mastering these techniques will empower you to harness the full potential of Python in a Linux environment.
As we delve deeper into this topic, we’ll explore the fundamental steps and best practices for running Python scripts on Linux. You’ll learn about the tools and commands that make the process seamless, as well as tips for troubleshooting common issues. With this knowledge at your fingertips, you’ll be well-equipped to take your Python programming skills to the next level.
Using the Terminal to Execute Python Scripts
To run a Python script in Linux, you primarily utilize the terminal. This command-line interface allows you to execute scripts efficiently. First, ensure that Python is installed on your system. You can check this by typing `python –version` or `python3 –version` in the terminal. If Python is correctly installed, the terminal will return the version number.
Once you confirm Python’s installation, navigate to the directory containing your Python script. You can change directories using the `cd` command. For example:
bash
cd /path/to/your/script
After reaching the desired directory, you can run the script using either `python` or `python3`, depending on the version you want to use. The command structure is as follows:
bash
python script_name.py
or
bash
python3 script_name.py
Making Your Python Script Executable
To streamline the process of running a Python script, you can make it executable. This involves modifying the file’s permissions and adding a shebang at the top of your script. Follow these steps:
- Open your script in a text editor and add the following shebang line at the very top:
python
#!/usr/bin/env python3
- Save the changes and exit the editor.
- Change the script’s permissions to make it executable:
bash
chmod +x script_name.py
Now, you can execute the script directly by entering `./script_name.py` in the terminal.
Running Python Scripts with Arguments
In many cases, you may need to pass arguments to your Python scripts. This can be accomplished seamlessly through the command line. Here’s how to do it:
bash
python script_name.py arg1 arg2 arg3
Inside your script, you can access these arguments using the `sys` module:
python
import sys
arg1 = sys.argv[1] # First argument
arg2 = sys.argv[2] # Second argument
To illustrate this, consider the following example script:
python
# example.py
import sys
print(f”Hello, {sys.argv[1]}!”)
You would run it as follows:
bash
python example.py Alice
This will output: `Hello, Alice!`
Using Virtual Environments
When working on multiple Python projects, it’s advisable to use virtual environments to manage dependencies efficiently. You can create a virtual environment using the `venv` module as follows:
- Navigate to your project directory.
- Create a virtual environment:
bash
python3 -m venv env
- Activate the virtual environment:
bash
source env/bin/activate
When activated, any Python scripts you run will use the packages installed in this environment.
Common Errors and Troubleshooting
While running Python scripts, you may encounter some common issues. Below is a table outlining typical errors and their solutions:
Error | Solution |
---|---|
Permission denied | Check if the script is executable using `chmod +x script_name.py`. |
Command not found | Ensure Python is installed and added to your PATH. |
SyntaxError | Check the script for typos or incorrect syntax. |
ModuleNotFoundError | Verify that the necessary packages are installed in your environment. |
By addressing these common errors, you can enhance your scripting experience and improve workflow efficiency.
Running Python Scripts in Linux
To execute a Python script in a Linux environment, follow these straightforward steps:
Prerequisites
Before running a Python script, ensure that Python is installed on your system. You can verify the installation by executing the following command in the terminal:
bash
python –version
or for Python 3:
bash
python3 –version
If Python is not installed, you can install it using your package manager. For example:
- Debian/Ubuntu:
bash
sudo apt update
sudo apt install python3
- Fedora:
bash
sudo dnf install python3
- CentOS/RHEL:
bash
sudo yum install python3
Creating a Python Script
- Open a text editor, such as `nano` or `vim`.
- Write your Python code and save the file with a `.py` extension. For example:
bash
nano my_script.py
Example content of `my_script.py`:
python
print(“Hello, World!”)
Making the Script Executable
To run the script directly, make it executable:
- Change the file’s permissions:
bash
chmod +x my_script.py
- Add a shebang at the top of your script to specify the interpreter:
python
#!/usr/bin/env python3
Running the Script
You can execute the script in several ways:
- Directly from the terminal:
bash
./my_script.py
- Using Python explicitly:
bash
python my_script.py
or for Python 3:
bash
python3 my_script.py
Running Scripts with Arguments
If your script requires command-line arguments, you can pass them directly:
bash
python my_script.py arg1 arg2
In your script, you can access these arguments using the `sys` module:
python
import sys
print(sys.argv[1]) # This will print the first argument
Using Virtual Environments
For projects with specific dependencies, consider using a virtual environment:
- Install the `venv` module if not already available:
bash
sudo apt install python3-venv # For Debian/Ubuntu
- Create a virtual environment:
bash
python3 -m venv myenv
- Activate the virtual environment:
bash
source myenv/bin/activate
- Install necessary packages:
bash
pip install package_name
- Run your script as before:
bash
python my_script.py
Debugging Python Scripts
To troubleshoot issues in your Python script, you can use:
- Print statements to track variable values.
- The `pdb` module for interactive debugging:
python
import pdb; pdb.set_trace()
Utilizing these techniques will help ensure your Python scripts run smoothly in a Linux environment.
Expert Guidance on Running Python Scripts in Linux
Dr. Emily Chen (Senior Software Engineer, Open Source Initiative). “To run a Python script on a Linux system, you first need to ensure that Python is installed. You can check this by running ‘python –version’ or ‘python3 –version’ in the terminal. Once confirmed, navigate to the directory containing your script using the ‘cd’ command, and execute it with ‘python script_name.py’ or ‘python3 script_name.py’ depending on your Python version.”
Mark Johnson (Linux System Administrator, Tech Solutions Inc.). “When executing Python scripts in Linux, it is essential to set the correct permissions. Use ‘chmod +x script_name.py’ to make your script executable. After that, you can run it directly by typing ‘./script_name.py’ if you have included the shebang line at the top of your script, which specifies the Python interpreter.”
Lisa Patel (DevOps Engineer, Cloud Innovations). “For more complex Python scripts that require specific environments or dependencies, consider using virtual environments. You can create one with ‘python3 -m venv myenv’ and activate it with ‘source myenv/bin/activate’. This ensures that your script runs with the correct libraries without affecting the global Python installation.”
Frequently Asked Questions (FAQs)
How do I run a Python script in the terminal on Linux?
To run a Python script in the terminal, navigate to the directory containing the script using the `cd` command. Then, execute the script by typing `python script_name.py` or `python3 script_name.py`, depending on your Python version.
What permissions do I need to run a Python script on Linux?
You need execute permissions for the script file. You can set the appropriate permissions using the command `chmod +x script_name.py`. This allows you to run the script directly if you use `./script_name.py`.
Can I run a Python script without specifying the Python interpreter?
Yes, you can run a Python script without explicitly calling the interpreter if you include a shebang line at the top of the script. For example, adding `#!/usr/bin/env python3` allows you to execute the script with `./script_name.py` after setting the executable permission.
What should I do if I encounter a “command not found” error?
If you encounter a “command not found” error, ensure that Python is installed on your system. You can check this by running `python –version` or `python3 –version`. If Python is not installed, you can install it using your package manager (e.g., `sudo apt install python3` for Ubuntu).
How can I pass arguments to a Python script in Linux?
You can pass arguments to a Python script by including them after the script name in the command line. For example, `python script_name.py arg1 arg2`. Inside your script, you can access these arguments using the `sys.argv` list from the `sys` module.
Is it possible to schedule a Python script to run automatically on Linux?
Yes, you can schedule a Python script to run automatically using cron jobs. Open the crontab editor with `crontab -e` and add a line specifying the schedule and the command to run your script, such as `0 * * * * /usr/bin/python3 /path/to/script_name.py` to run it hourly.
Running a Python script on a Linux system involves several straightforward steps. First, ensure that Python is installed on your machine. Most Linux distributions come with Python pre-installed, but you can verify this by opening a terminal and typing `python –version` or `python3 –version`. If Python is not installed, you can easily install it using your package manager.
Once you have confirmed that Python is available, you need to navigate to the directory containing your script using the terminal. This can be done using the `cd` command followed by the path to the directory. After reaching the correct directory, you can execute your script by typing `python script_name.py` or `python3 script_name.py`, depending on the version of Python you wish to use.
Additionally, it’s important to ensure that your script has the appropriate permissions to be executed. You can modify the permissions using the `chmod` command. For example, running `chmod +x script_name.py` will make your script executable. After setting the permissions, you can run the script directly by typing `./script_name.py` if you include the shebang line at the top of your script.
In summary, running a Python script in Linux is a
Author Profile
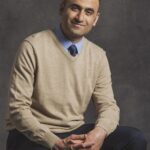
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?