How Can You Save a JSON File Effectively?
In today’s data-driven world, the ability to manage and manipulate data formats is essential for developers, data analysts, and tech enthusiasts alike. One such format that has gained immense popularity is JSON (JavaScript Object Notation). Known for its simplicity and readability, JSON serves as a lightweight way to store and exchange data, making it a favorite among web applications and APIs. But once you’ve crafted the perfect JSON structure, how do you ensure it is saved correctly for future use? In this article, we will explore the various methods and best practices for saving JSON files, empowering you to handle your data with confidence.
Saving a JSON file may seem straightforward, but there are several considerations to keep in mind, depending on the programming language and environment you’re using. From understanding file paths to choosing the right encoding, each step plays a crucial role in ensuring that your data is stored accurately and securely. Whether you’re working with JavaScript, Python, or another language, grasping the nuances of file handling is key to effective data management.
Moreover, the implications of saving JSON files extend beyond mere storage; they can impact data integrity, accessibility, and performance. As we delve deeper into the topic, you’ll discover various techniques tailored to different scenarios, including saving JSON data locally, sending it to a server,
Saving JSON Files in Python
To save a JSON file in Python, you typically utilize the built-in `json` module, which provides a straightforward interface for encoding and decoding JSON data. Follow these steps to save a JSON object to a file:
- Import the JSON Module: Start by importing the `json` module into your script.
- Prepare Your Data: Create a Python dictionary or list that you want to save as a JSON file.
- Open a File in Write Mode: Use the `open()` function to create a new file or overwrite an existing file.
- Use `json.dump()`: Call the `json.dump()` method to write the JSON data to the file.
Here is an example of how to execute these steps:
“`python
import json
data = {
“name”: “John”,
“age”: 30,
“city”: “New York”
}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
Saving JSON Files in JavaScript
In JavaScript, saving a JSON file can be accomplished in various environments, such as within a web browser or Node.js. The approach varies slightly depending on the context.
In the Browser:
- Create a JSON Object: Define your data as a JSON object.
- Convert the Object to a String: Use `JSON.stringify()` to convert the object into a JSON string.
- Create a Blob: Use the `Blob` constructor to create a blob of the JSON string.
- Use the `URL.createObjectURL()` method: Create a downloadable link.
- Programmatically Click the Link: Trigger the download.
Here’s an example:
“`javascript
const data = {
name: “John”,
age: 30,
city: “New York”
};
const jsonString = JSON.stringify(data);
const blob = new Blob([jsonString], { type: ‘application/json’ });
const url = URL.createObjectURL(blob);
const a = document.createElement(‘a’);
a.href = url;
a.download = ‘data.json’;
document.body.appendChild(a);
a.click();
document.body.removeChild(a);
“`
In Node.js:
- Use the `fs` Module: Import the `fs` module to handle file operations.
- Stringify Your Data: Convert your data into a JSON string using `JSON.stringify()`.
- Write to a File: Use `fs.writeFileSync()` or `fs.writeFile()` to save the JSON string.
Example code in Node.js:
“`javascript
const fs = require(‘fs’);
const data = {
name: “John”,
age: 30,
city: “New York”
};
fs.writeFileSync(‘data.json’, JSON.stringify(data));
“`
Comparison Table for Saving JSON in Different Languages
Language | Method | Code Snippet |
---|---|---|
Python | json.dump() |
import json data = {"key": "value"} with open('file.json', 'w') as f: json.dump(data, f) |
JavaScript (Browser) | Blob and URL.createObjectURL() |
const data = {"key": "value"}; const jsonString = JSON.stringify(data); const blob = new Blob([jsonString], { type: 'application/json' }); const url = URL.createObjectURL(blob); |
Node.js | fs.writeFileSync() |
const fs = require('fs'); const data = {"key": "value"}; fs.writeFileSync('file.json', JSON.stringify(data)); |
Methods to Save a JSON File
Saving a JSON file can be accomplished through various programming languages and tools. Below are some common methods across different platforms.
Using Python
In Python, the `json` module provides a straightforward way to save data in JSON format.
“`python
import json
data = {
“name”: “John”,
“age”: 30,
“city”: “New York”
}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file, indent=4)
“`
This code snippet does the following:
- Imports the `json` module.
- Creates a dictionary named `data`.
- Opens a file named `data.json` in write mode.
- Uses `json.dump()` to write the dictionary to the file with an indentation of 4 spaces for readability.
Using JavaScript
In JavaScript, particularly in a Node.js environment, you can use the `fs` module to save JSON data.
“`javascript
const fs = require(‘fs’);
const data = {
name: “John”,
age: 30,
city: “New York”
};
fs.writeFile(‘data.json’, JSON.stringify(data, null, 4), (err) => {
if (err) throw err;
console.log(‘Data saved to data.json’);
});
“`
The process involves:
- Requiring the `fs` module.
- Creating a JavaScript object.
- Using `fs.writeFile()` to save the stringified version of the object to `data.json`, with 4 spaces for indentation.
Using Command Line Tools
For users comfortable with command line interfaces, you can use tools like `curl` or `echo` to create a JSON file.
“`bash
echo ‘{“name”: “John”, “age”: 30, “city”: “New York”}’ > data.json
“`
This command:
- Uses `echo` to output the JSON string.
- Redirects the output to `data.json`, creating the file if it doesn’t exist.
Using Online JSON Editors
There are several online platforms that allow you to create and save JSON files directly through a web interface. Some popular options include:
- [jsoneditoronline.org](https://jsoneditoronline.org)
- [jsonlint.com](https://jsonlint.com)
Steps to save a JSON file online:
- Visit the chosen website.
- Input or paste your JSON data.
- Use the “Download” or “Save” option provided by the tool to download your JSON file to your local machine.
Using Text Editors
You can also create and save a JSON file using any text editor. This process is universal and applies to various editors like Notepad, Sublime Text, or VSCode.
- Open your preferred text editor.
- Input your JSON data.
- Use the “Save As” option, and ensure to set the file type as “All Files” (if required).
- Name your file with a `.json` extension, for example, `data.json`.
Considerations for Valid JSON
When saving JSON data, ensure that:
- The data is properly formatted.
- Strings are enclosed in double quotes.
- Use commas appropriately between key-value pairs.
- Objects are enclosed in curly braces `{}` and arrays in square brackets `[]`.
Common JSON Errors | Description |
---|---|
Missing Quotes | Keys and string values must be enclosed in double quotes. |
Trailing Comma | No trailing commas are allowed after the last item in objects or arrays. |
Incorrect Data Types | Ensure values are of correct types: strings, numbers, arrays, objects, or booleans. |
Expert Insights on Saving JSON Files
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When saving a JSON file, it is crucial to ensure that the data structure adheres to JSON syntax rules. Utilizing programming languages like Python or JavaScript can simplify this process, as they provide built-in libraries that facilitate seamless serialization of data into JSON format.”
Michael Chen (Software Engineer, CodeCraft Solutions). “To save a JSON file effectively, always specify the correct file extension and ensure that your data is properly formatted. Tools like Postman or programming environments like Node.js can be particularly useful for testing and saving JSON data accurately.”
Linda Thompson (Web Developer, Digital Trends). “It is essential to handle exceptions when saving JSON files to avoid data loss. Implementing error handling in your code not only safeguards your data but also enhances the reliability of your application when writing JSON files.”
Frequently Asked Questions (FAQs)
How can I save a JSON file using Python?
To save a JSON file in Python, use the `json` module. First, convert your data into a JSON format using `json.dumps()` or `json.dump()`, then write it to a file using the `open()` function with the ‘w’ mode.
What is the correct file extension for JSON files?
The correct file extension for JSON files is `.json`. This extension indicates that the file contains data formatted in JavaScript Object Notation.
Can I save a JSON file using a text editor?
Yes, you can save a JSON file using any text editor. Simply write your JSON data in the editor and save the file with a `.json` extension.
What tools can I use to validate a JSON file before saving?
You can use online JSON validators like JSONLint or built-in tools in code editors like Visual Studio Code, which provide syntax highlighting and error detection for JSON files.
Is it possible to save JSON data in a database?
Yes, many databases support storing JSON data, such as MongoDB and PostgreSQL. You can convert your JSON data into the appropriate format and use database commands to save it.
How do I save a JSON file in JavaScript?
In JavaScript, you can save a JSON file by converting an object to a JSON string using `JSON.stringify()`, then using the `Blob` and `URL.createObjectURL()` methods to create a downloadable link for the user.
Saving a JSON file is a straightforward process that can be accomplished using various programming languages and tools. The primary steps involve creating a JSON object, converting it into a string format, and then writing that string to a file with the appropriate .json extension. This process is essential for data interchange between applications, as JSON (JavaScript Object Notation) is a widely accepted format for representing structured data.
Different programming environments offer built-in libraries and functions to facilitate the saving of JSON files. For instance, in Python, the `json` module provides methods like `json.dump()` to write JSON data directly to a file. Similarly, JavaScript can use the `File` API or Node.js’s `fs` module to achieve the same result. Understanding these methods and their usage is crucial for developers working with data serialization and storage.
mastering the technique of saving JSON files is vital for effective data management and communication in software development. By leveraging the appropriate tools and libraries, developers can ensure that their data is stored in a structured and accessible format. This not only enhances the efficiency of data handling but also promotes interoperability between different systems and applications.
Author Profile
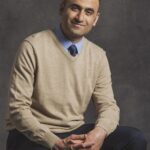
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?