How Can You Effectively Save Your Python Projects?
In the ever-evolving landscape of programming, Python has emerged as a powerhouse, renowned for its simplicity and versatility. Whether you’re a seasoned developer or a curious beginner, the ability to save your work efficiently is paramount. But what does it truly mean to “save” in Python? Is it merely about storing code, or does it encompass a broader spectrum of practices that ensure your projects remain intact and accessible? In this article, we will delve into the essential methods and best practices for saving your Python work, equipping you with the knowledge to safeguard your creations and streamline your coding experience.
When working with Python, saving your scripts and data is just the tip of the iceberg. Beyond the conventional act of writing code and saving files, there are various strategies to preserve your work effectively. From utilizing integrated development environments (IDEs) that offer auto-save features to employing version control systems like Git, understanding these tools can dramatically enhance your workflow. Additionally, knowing how to manage data persistence—whether through databases or file formats—plays a crucial role in ensuring that your applications can store and retrieve information seamlessly.
Moreover, saving in Python isn’t limited to just code and data; it also involves maintaining the integrity of your development environment. This includes managing dependencies and ensuring that your projects
Saving Python Scripts
To save a Python script, you will typically use a text editor or an integrated development environment (IDE). Python files are saved with the `.py` file extension, which allows the Python interpreter to recognize them as executable scripts.
Here are the steps to save a Python script in a text editor:
- Open your preferred text editor (e.g., Visual Studio Code, Sublime Text, or even Notepad).
- Write your Python code in the editor.
- Click on the “File” menu and select “Save As.”
- In the dialog that appears, choose the directory where you want to save the file.
- Enter your desired filename followed by `.py` (for example, `my_script.py`).
- Click “Save.”
If you are using an IDE, the process is similar but may include additional features such as project management and debugging tools.
Saving Data in Python
In Python, you can save data in various formats depending on your requirements, including text files, CSV files, JSON, and databases. Each method has its own use case, advantages, and disadvantages.
Common Methods for Saving Data:
- Text Files (.txt): Simple to implement; suitable for basic data storage.
- CSV Files (.csv): Ideal for tabular data; can be easily opened in spreadsheet applications.
- JSON Files (.json): Useful for saving structured data; widely used for APIs and web applications.
- Databases: For large datasets requiring complex queries; can use SQLite, MySQL, PostgreSQL, etc.
Example: Saving Data to a CSV File
To save data into a CSV file, you can use the built-in `csv` module. Below is a simple example:
“`python
import csv
data = [
[‘Name’, ‘Age’, ‘City’],
[‘Alice’, 30, ‘New York’],
[‘Bob’, 25, ‘Los Angeles’],
]
with open(‘data.csv’, mode=’w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
This code snippet creates a CSV file named `data.csv` and writes two rows of data to it.
Saving Python Environment Configuration
When developing Python applications, managing dependencies is crucial. You can save your Python environment configuration using a `requirements.txt` file. This file lists all the packages and their versions required for your project.
To create a `requirements.txt` file, use the following command:
“`bash
pip freeze > requirements.txt
“`
This command captures the current environment’s package list. Below is an example of the structure of a `requirements.txt` file:
Package | Version |
---|---|
numpy | 1.21.2 |
pandas | 1.3.3 |
You can then install the required packages in another environment using:
“`bash
pip install -r requirements.txt
“`
This method ensures that your project dependencies are consistent across different environments.
Saving Python Scripts
To save your Python scripts, you can follow several methods depending on the environment you are using. The most common environments include IDEs (Integrated Development Environments) like PyCharm, Jupyter Notebooks, and simple text editors.
Using an Integrated Development Environment (IDE)
When using an IDE such as PyCharm or Visual Studio Code, the process is typically straightforward:
– **Open the IDE**: Launch your IDE of choice.
– **Create a New File**: Click on `File` > `New File` or use the shortcut (usually Ctrl + N).
– **Write Your Code**: Type your Python code in the newly created file.
– **Save the File**:
- Click on `File` > `Save As…`
- Choose your desired location.
- Name your file with a `.py` extension (e.g., `script.py`).
Saving in Jupyter Notebooks
Jupyter Notebooks allow for interactive coding and saving in a specific format:
– **Open Jupyter Notebook**: Launch Jupyter Notebook from your command line or Anaconda Navigator.
– **Create a New Notebook**: Select `New` > `Python 3` from the top right corner.
- Write Your Code: Enter your Python code in the cells provided.
- Save the Notebook:
- Click on the disk icon in the toolbar or use the shortcut (Ctrl + S).
- Your notebook will be saved with a `.ipynb` extension.
Saving Python Files in Text Editors
If you are using a simple text editor like Notepad or Sublime Text:
– **Open Text Editor**: Launch your preferred text editor.
– **Write Your Code**: Type your Python script.
– **Save the File**:
- Click on `File` > `Save As…`
- Select the location where you want to save the file.
- Ensure you name your file with a `.py` extension (e.g., `script.py`).
Version Control with Git
Using version control systems like Git enhances your file-saving process by allowing you to track changes:
- Initialize a Git Repository:
- Open your command line and navigate to your project folder.
- Use the command `git init` to create a new repository.
- Add Files to Staging Area:
- Use `git add filename.py` to stage your files for commit.
- Commit Your Changes:
- Use `git commit -m “Your commit message”` to save your changes with a message.
- Push to Remote Repository:
- If using a service like GitHub, use `git push origin main` to upload your code.
Automating Saves with Scripts
You can also automate saving your Python scripts by writing a small Python script that saves your work at regular intervals. This can be done using the following libraries:
- Using `time` and `os`:
- Set a timer for automatic saving.
- Use `os` to copy the current file to a backup location.
“`python
import time
import os
import shutil
def auto_save(original_file_path, backup_folder):
while True:
time.sleep(300) Save every 5 minutes
shutil.copy(original_file_path, backup_folder)
“`
Backup Strategies
Implementing effective backup strategies ensures that your work is preserved:
- Cloud Storage: Use services like Google Drive, Dropbox, or OneDrive to store your files.
- External Drives: Regularly back up your scripts to an external hard drive.
- Automated Backup Software: Consider using software that automatically backs up your files at scheduled intervals.
By utilizing these methods, you can ensure that your Python scripts are saved and backed up effectively, minimizing the risk of data loss.
Strategies for Efficient Python Resource Management
Dr. Emily Carter (Lead Data Scientist, Tech Innovations Inc.). “To save Python effectively, one should focus on optimizing code efficiency. This includes using built-in functions, avoiding unnecessary loops, and leveraging libraries such as NumPy and Pandas for data manipulation, which can significantly reduce memory usage and execution time.”
James Liu (Senior Software Engineer, Cloud Solutions Corp.). “Implementing proper resource management practices, such as using context managers for file operations and memory management, can greatly enhance the performance of Python applications. This not only saves memory but also ensures that resources are released promptly, preventing leaks.”
Dr. Sarah Thompson (Professor of Computer Science, University of Tech). “Educating developers on the importance of profiling and monitoring their Python applications is crucial. Utilizing tools like cProfile and memory_profiler allows for identifying bottlenecks and optimizing resource usage, ultimately leading to more efficient Python programming.”
Frequently Asked Questions (FAQs)
How can I save a Python script?
You can save a Python script by using a text editor or an Integrated Development Environment (IDE). Simply write your code and select “Save” from the file menu, or use the shortcut Ctrl+S (Cmd+S on Mac), ensuring to save the file with a `.py` extension.
What is the command to save a file in Python?
In Python, you can save data to a file using the built-in `open()` function along with the `write()` method. For example, `with open(‘filename.txt’, ‘w’) as file: file.write(‘Your content here’)` will create or overwrite the specified file with the provided content.
How do I save data to a CSV file in Python?
To save data to a CSV file in Python, you can use the `csv` module. First, import the module, then create a `csv.writer` object and use the `writerow()` or `writerows()` methods to write data to the file.
Can I save Python objects using serialization?
Yes, you can save Python objects using serialization with the `pickle` module. Use `pickle.dump(object, file)` to serialize and save the object to a file, and `pickle.load(file)` to read it back.
What is the best way to save configuration settings in Python?
The best way to save configuration settings in Python is by using the `configparser` module for INI files or the `json` module for JSON files. Both methods allow for structured storage and easy retrieval of configuration data.
How can I save a Jupyter Notebook?
In Jupyter Notebook, you can save your work by clicking on the disk icon in the toolbar or by using the keyboard shortcut Ctrl+S (Cmd+S on Mac). This saves the notebook in its current state, preserving all code and outputs.
In summary, saving Python scripts and projects is a fundamental practice for developers and data scientists alike. Properly saving your work ensures that you can revisit and modify your code as needed. It is essential to utilize version control systems, such as Git, to track changes over time and facilitate collaboration with others. Additionally, employing cloud storage solutions can provide an extra layer of security and accessibility, allowing you to access your work from any location.
Another crucial aspect of saving Python projects involves organizing your files effectively. This includes using meaningful naming conventions for files and directories, which can help you quickly identify the purpose of each component. Furthermore, documenting your code with comments and README files enhances clarity and usability, making it easier for others (or yourself) to understand the project in the future.
Lastly, regular backups are vital to prevent data loss. Utilizing automated backup solutions can safeguard your work against unforeseen circumstances, such as hardware failures or accidental deletions. By adopting these practices, you can ensure that your Python projects are not only saved but also well-organized and secure, ultimately leading to a more efficient development process.
Author Profile
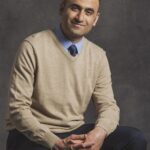
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?