How Can You Skip a Line in Python?
Introduction
In the world of programming, the ability to manipulate text output is a fundamental skill that can enhance the readability and presentation of your code. Whether you’re crafting a simple script or developing a complex application, knowing how to format your output effectively can make all the difference. One common formatting task is skipping a line in Python, which can help create clear separations between different sections of output, making it easier for users to digest information. This article will guide you through the various methods to achieve this, ensuring your Python projects are both functional and visually appealing.
When working with Python, you may often find yourself needing to improve the clarity of your printed output. Skipping a line can serve as a powerful tool to enhance the structure of your text, allowing for better organization and a more polished appearance. This simple yet effective technique can be applied in various scenarios, from console applications to more intricate user interfaces.
Understanding how to skip lines not only contributes to cleaner output but also aids in creating a user-friendly experience. As you delve into the methods available for achieving this in Python, you’ll discover that the language offers several straightforward options, each suited to different contexts and preferences. Get ready to unlock the potential of your text formatting skills and take your Python programming to the next level!
Using Newline Characters
In Python, you can skip a line by using newline characters. The most common way to create a new line in your output is by using the `\n` character. This character can be used in strings to indicate where a line break should occur.
For example:
python
print(“Hello, World!\nThis is a new line.”)
This code will output:
Hello, World!
This is a new line.
You can insert multiple newline characters to create additional blank lines. For instance, `\n\n` will skip two lines.
Using the Print Function
Another straightforward method to skip a line in Python is by using multiple `print()` statements. Each call to `print()` outputs a new line. By including an empty `print()` call, you can create a blank line in your output.
Example:
python
print(“First line.”)
print() # This will print a blank line
print(“Second line.”)
The output will be:
First line.
Second line.
Using String Formatting
String formatting methods can also be employed to include newline characters effectively. Python provides several ways to format strings that can incorporate newlines.
- f-Strings (Python 3.6+): You can utilize f-strings to insert newlines easily.
python
name = “Alice”
message = f”Hello, {name}!\nWelcome to the program.”
print(message)
- `str.format()` Method: This method also supports newlines.
python
greeting = “Hello, {}!\nEnjoy your day.”
print(greeting.format(“Bob”))
Table of Newline Methods
Method | Description | Example |
---|---|---|
Newline Character | Insert ‘\n’ in strings | print(“Line 1\nLine 2”) |
Multiple print() | Use empty print() for blank lines | print(“A”); print(); print(“B”) |
f-Strings | Embed newlines in formatted strings | f”Hello!\nGoodbye!” |
str.format() | Format strings with newlines | “Hello!\n{}”.format(“World”) |
By utilizing these methods, you can effectively control line spacing in your Python scripts, enhancing the readability of your output.
Methods to Skip a Line in Python
In Python, skipping a line can be accomplished in several ways, depending on the context and the output medium. Below are the most common methods used in various scenarios.
Using Print Statement
When printing to the console, you can skip a line by adding an additional newline character (`\n`) in your print function. This method is straightforward and widely used for creating space between outputs.
python
print(“First Line”)
print(“\n”) # This will skip a line
print(“Third Line”)
Alternatively, you can achieve the same effect by utilizing the `end` parameter of the print function:
python
print(“First Line”, end=”\n\n”) # Skips a line after the first line
print(“Third Line”)
Using Multiline Strings
When dealing with multiline strings, you can easily include blank lines by incorporating `\n` within the triple quotes. This approach is particularly useful when generating formatted text.
python
multiline_string = “””Line One
Line Three”””
print(multiline_string)
Writing to Files
When writing to files, skipping a line can be performed similarly by writing newline characters. Here’s how to do it:
python
with open(“output.txt”, “w”) as file:
file.write(“First Line\n\n”)
file.write(“Third Line”)
This code will create a text file named `output.txt` with an empty line between the first and third lines.
Using Loops
In loops, you can conditionally skip a line based on certain criteria. For instance, if you want to skip a line after every third iteration, you can use the following:
python
for i in range(1, 10):
print(f”Line {i}”)
if i % 3 == 0:
print() # Skips a line after every third line
Using Format Strings
With formatted strings, you can embed newlines as well. Here’s an example using f-strings:
python
line1 = “First Line”
line2 = “Second Line”
formatted_output = f”{line1}\n\n{line2}”
print(formatted_output)
Summary of Methods
Method | Description |
---|---|
Print with `\n` | Directly adds newline characters in print statements. |
Print with `end` | Uses the `end` parameter to specify custom endings. |
Multiline Strings | Allows inclusion of newlines in formatted strings. |
File Writing | Uses `\n` in file operations to create blank lines. |
Loops | Conditionally skips lines within loops. |
Format Strings | Embeds newlines in formatted output. |
These methods allow flexibility when handling output in Python, ensuring that developers can format their output according to specific requirements.
Expert Insights on Skipping Lines in Python
Dr. Emily Carter (Senior Software Engineer, CodeCrafters Inc.). “In Python, skipping a line can be accomplished by using the newline character `\n` within print statements. This is particularly useful for formatting output in a more readable manner, allowing developers to create clear separations between different sections of text.”
James Liu (Python Instructor, Tech Academy). “To skip a line in Python, one can utilize multiple print statements or include the newline character in a single print statement. This technique enhances the readability of console outputs, especially when displaying results or logs.”
Sarah Thompson (Lead Developer, Innovative Solutions). “Using `print()` with an empty call, such as `print()`, is an effective way to insert a blank line in your output. This method is straightforward and widely used in Python programming to improve the structure of printed information.”
Frequently Asked Questions (FAQs)
How can I skip a line when printing in Python?
You can skip a line in Python by using the `print()` function with an empty string. For example, `print(“”)` will produce a blank line.
Is there a specific character to skip a line in Python strings?
Yes, you can use the newline character `\n` within strings to create a line break. For instance, `print(“Hello\nWorld”)` will output “Hello” on one line and “World” on the next.
Can I skip multiple lines in Python?
Yes, you can skip multiple lines by using multiple newline characters. For example, `print(“Line 1\n\nLine 2”)` will insert one blank line between “Line 1” and “Line 2”.
How do I skip a line in a file while writing in Python?
When writing to a file, you can include a newline character `\n` in your write statements. For example, `file.write(“Line 1\n\nLine 2”)` will create a blank line in the file.
What is the difference between `print()` and `print(“\n”)`?
The `print()` function without arguments outputs a newline, while `print(“\n”)` explicitly prints a newline character. Both result in moving to the next line, but the former is more concise.
Can I use triple quotes to skip lines in Python?
Yes, triple quotes can be used for multi-line strings, which inherently include line breaks. For example, `print(“””Line 1\n\nLine 2″””)` will produce a blank line between “Line 1” and “Line 2”.
In Python, skipping a line in output can be accomplished in several straightforward ways. The most common method is to use the newline character `\n`, which can be included in strings to create a line break. For instance, using `print(“Hello\nWorld”)` will display “Hello” on one line and “World” on the next. This method is versatile and can be used in various contexts, including within formatted strings.
Another approach to achieve line skipping is by utilizing multiple `print()` statements. By calling `print()` with no arguments, a blank line is printed, effectively creating a space between outputs. For example, `print(“Hello”)` followed by `print()` and then `print(“World”)` will result in “Hello” on one line, a blank line, and “World” on the next line. This method is particularly useful for enhancing readability in console outputs.
Additionally, Python’s f-strings and formatted string literals can also incorporate line breaks seamlessly. By embedding `\n` within an f-string, developers can craft more complex outputs that require specific formatting. This capability allows for greater control over how information is presented in the console, making it easier to convey messages clearly and effectively.
Author Profile
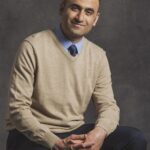
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?