How Can You Sort a Dictionary by Value in Python?
In the world of Python programming, dictionaries are a fundamental data structure that allows for the efficient storage and retrieval of key-value pairs. However, as your data grows in complexity, you may find yourself needing to organize this information in a more meaningful way. Sorting a dictionary by its values can be a game-changer, enabling you to analyze and present your data with clarity and precision. Whether you’re handling user scores, product prices, or any other numerical dataset, mastering the art of sorting dictionaries can elevate your coding skills and enhance the functionality of your applications.
Sorting a dictionary by value in Python is not just a matter of aesthetics; it can significantly impact the way you interpret and utilize the data at hand. With Python’s rich set of built-in functions and libraries, you can achieve this task with ease and efficiency. The process involves leveraging techniques that allow you to extract, sort, and present the values in a way that aligns with your specific needs. Understanding how to manipulate dictionaries in this manner opens up a world of possibilities for data analysis and reporting.
As you delve into the intricacies of sorting dictionaries, you’ll discover various methods and best practices that can streamline your workflow. From using built-in functions to exploring more advanced sorting techniques, you’ll gain insights that will empower you to handle data more
Sorting a Dictionary by Value
To sort a dictionary by its values in Python, you can utilize the built-in `sorted()` function. This function allows you to specify a custom sorting key, enabling you to sort the dictionary based on its values rather than its keys.
The basic syntax to sort a dictionary by values is as follows:
“`python
sorted_dict = dict(sorted(original_dict.items(), key=lambda item: item[1]))
“`
Here, `original_dict` is the dictionary you want to sort. The `items()` method returns a view of the dictionary’s items, which is then passed to `sorted()`. The `key` argument uses a lambda function that specifies that the second element of each item (the value) should be used for sorting.
Sorting in Ascending and Descending Order
By default, the `sorted()` function sorts in ascending order. If you want to sort the dictionary in descending order, you can set the `reverse` parameter to `True`.
Here’s how you can do both:
- Ascending Order:
“`python
sorted_dict_asc = dict(sorted(original_dict.items(), key=lambda item: item[1]))
“`
- Descending Order:
“`python
sorted_dict_desc = dict(sorted(original_dict.items(), key=lambda item: item[1], reverse=True))
“`
Example of Sorting a Dictionary by Value
Consider the following example dictionary:
“`python
grades = {‘Alice’: 88, ‘Bob’: 95, ‘Charlie’: 78, ‘David’: 92}
“`
You can sort this dictionary by values as shown below:
“`python
Ascending order
sorted_grades_asc = dict(sorted(grades.items(), key=lambda item: item[1]))
Descending order
sorted_grades_desc = dict(sorted(grades.items(), key=lambda item: item[1], reverse=True))
“`
The sorted results will be:
- Ascending Order:
“`python
{‘Charlie’: 78, ‘Alice’: 88, ‘David’: 92, ‘Bob’: 95}
“`
- Descending Order:
“`python
{‘Bob’: 95, ‘David’: 92, ‘Alice’: 88, ‘Charlie’: 78}
“`
Handling Ties in Values
In cases where multiple keys have the same value, the sorting will maintain the order of keys as they appear in the original dictionary. However, if you want to specify a secondary sorting criterion, you can modify the key function to include the keys as well:
“`python
sorted_dict_ties = dict(sorted(original_dict.items(), key=lambda item: (item[1], item[0])))
“`
This approach will sort primarily by value and use the key as a tiebreaker, which can be useful when values are not unique.
Sorting with a Table Representation
When displaying sorted dictionaries, it can be helpful to visualize the data in a tabular format. Below is an example of how you might represent sorted data:
Name | Score |
---|---|
Charlie | 78 |
Alice | 88 |
David | 92 |
Bob | 95 |
This table represents the dictionary sorted in ascending order by value, allowing for easy visualization of the data.
Sorting a Dictionary by Value
In Python, dictionaries store key-value pairs, and sorting them by their values can be accomplished using several methods. The most common approach involves utilizing the built-in `sorted()` function, which is both efficient and straightforward.
Using `sorted()` Function
The `sorted()` function allows you to sort the dictionary by its values. This can be achieved using a lambda function as the key parameter.
“`python
my_dict = {‘apple’: 3, ‘banana’: 1, ‘cherry’: 2}
Sorting by value
sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))
print(sorted_dict)
“`
In this example, `my_dict.items()` retrieves the key-value pairs, and the lambda function specifies that sorting should be based on the second element of each pair (the value).
Sorting in Descending Order
To sort the dictionary in descending order, you can set the `reverse` parameter to `True`.
“`python
Sorting by value in descending order
sorted_dict_desc = dict(sorted(my_dict.items(), key=lambda item: item[1], reverse=True))
print(sorted_dict_desc)
“`
This method will rearrange the dictionary such that items with higher values appear first.
Using `collections.OrderedDict` for Preserving Order
If you’re using Python versions prior to 3.7, where dictionary order is not guaranteed, you may want to use `collections.OrderedDict` to maintain the order of the sorted dictionary.
“`python
from collections import OrderedDict
Sorting and preserving order
ordered_sorted_dict = OrderedDict(sorted(my_dict.items(), key=lambda item: item[1]))
print(ordered_sorted_dict)
“`
This ensures that the order of elements remains consistent as per the sorted values.
Sorting by Values with Custom Criteria
You may want to apply custom sorting logic depending on specific conditions. In such cases, the lambda function can be modified accordingly.
“`python
Custom sorting by value
custom_sorted_dict = dict(sorted(my_dict.items(), key=lambda item: -item[1] if item[0].startswith(‘b’) else item[1]))
print(custom_sorted_dict)
“`
This example sorts items starting with the letter ‘b’ in descending order while sorting others in ascending order.
Example: Sorting a Dictionary of Mixed Values
When dealing with mixed data types, ensure that your sorting criteria accounts for the types involved. Here is a brief example:
“`python
mixed_dict = {‘apple’: 3, ‘banana’: 1, ‘cherry’: ‘two’}
Sorting with error handling
sorted_mixed_dict = dict(sorted(mixed_dict.items(), key=lambda item: (str(item[1]))))
print(sorted_mixed_dict)
“`
In this scenario, converting all values to strings ensures that the sorting process can handle different data types without errors.
Final Thoughts
Sorting a dictionary by its values in Python can be executed efficiently using various methods tailored to specific needs and scenarios. Understanding how to utilize the `sorted()` function alongside lambda expressions provides a robust framework for managing dictionary data effectively.
Expert Insights on Sorting Dictionaries by Value in Python
Dr. Emily Chen (Senior Data Scientist, Tech Innovations Inc.). “Sorting a dictionary by value in Python can be efficiently achieved using the built-in `sorted()` function combined with a lambda function. This method allows for clear and concise code that is easy to read and maintain.”
Michael Thompson (Python Developer, CodeCrafters). “Utilizing the `operator` module’s `itemgetter` function can enhance performance when sorting large dictionaries. This approach is particularly useful in data-heavy applications where speed is crucial.”
Sarah Patel (Software Engineer, Data Solutions Group). “When sorting dictionaries by value, it is important to consider whether you need ascending or descending order. Leveraging the `reverse` parameter in the `sorted()` function can help achieve the desired outcome efficiently.”
Frequently Asked Questions (FAQs)
How can I sort a dictionary by its values in Python?
You can sort a dictionary by its values using the `sorted()` function along with a lambda function. For example: `sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))`.
Is it possible to sort a dictionary in descending order by value?
Yes, you can sort a dictionary in descending order by setting the `reverse` parameter to `True` in the `sorted()` function. For example: `sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1], reverse=True))`.
What data type does the sorted dictionary return?
The `sorted()` function returns a list of tuples representing the sorted items. When converting back to a dictionary, it returns a dictionary object in Python 3.7 and later, which maintains the insertion order.
Can I sort a dictionary with non-comparable values?
No, if the values in the dictionary are non-comparable (e.g., a mix of strings and integers), Python will raise a `TypeError`. Ensure that the values are of a comparable type before sorting.
Are there any built-in methods to sort dictionaries in Python?
Python does not have a built-in method specifically for sorting dictionaries by value. However, you can use the `sorted()` function as described, or utilize libraries like `pandas` for more complex sorting operations.
How can I sort a dictionary by value while preserving the original dictionary?
To preserve the original dictionary, create a new sorted dictionary using the `sorted()` function without modifying the original. For example: `sorted_dict = dict(sorted(my_dict.items(), key=lambda item: item[1]))`.
Sorting a dictionary by its values in Python can be accomplished using various methods. The most common approach involves utilizing the built-in `sorted()` function, which allows for sorting based on the values while maintaining the key-value relationship. By applying a lambda function as the sorting key, one can easily specify that the sorting should be done according to the dictionary’s values rather than its keys.
Another effective method to sort a dictionary by value is to use the `items()` method, which returns a view of the dictionary’s items as tuples. This can be particularly useful when combined with the `sorted()` function, as it allows for a straightforward transformation of the dictionary into a sorted list of tuples. Furthermore, this method can also be adjusted to sort in descending order by setting the `reverse` parameter to `True`.
It is also worth noting that Python 3.7 and later versions maintain the insertion order of dictionaries, making it easier to work with sorted dictionaries. For those who require a sorted dictionary structure, the `collections.OrderedDict` can be utilized to maintain the order after sorting. Overall, these methods provide flexibility and efficiency for sorting dictionaries by value in Python, catering to various programming needs.
Author Profile
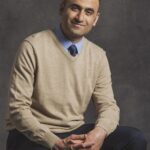
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?