How Can You Effectively Sort Data in AG Grid?
Sorting data is a fundamental aspect of data presentation, especially when dealing with large datasets in modern web applications. In the realm of front-end development, AG Grid has emerged as a powerful and flexible solution for displaying tabular data with ease. Whether you’re building a complex enterprise application or a simple dashboard, understanding how to sort data effectively within AG Grid can significantly enhance user experience and data accessibility. In this article, we will delve into the various methods and best practices for implementing sorting in AG Grid, empowering you to create intuitive and responsive data tables.
AG Grid offers a robust sorting feature that allows users to organize data in a manner that best suits their needs. With built-in support for both ascending and descending order, developers can easily enable sorting on specific columns, giving users the flexibility to view their data in a structured way. The grid’s sorting capabilities are not only powerful but also customizable, allowing for tailored sorting logic based on the data type or specific requirements of your application.
Moreover, AG Grid’s sorting functionality extends beyond simple alphabetical or numerical order. It provides advanced options such as multi-column sorting, which enables users to prioritize their data views by selecting multiple columns to sort simultaneously. This feature is particularly beneficial for datasets with hierarchical relationships, allowing for a more nuanced and detailed presentation of information
Sorting Basics in AG Grid
AG Grid offers a highly flexible sorting mechanism that allows users to sort data in various ways. By default, sorting is enabled on all columns, making it easy to organize data according to specific criteria. Users can sort by clicking on the column headers, which toggles between ascending, descending, and unsorted states.
To implement sorting, you can utilize the following attributes in your grid options:
- sortable: Set this attribute to `true` for any column you wish to be sortable.
- sort: This attribute allows you to define the default sort order when the grid is initially loaded. Options include `asc` for ascending or `desc` for descending.
Here’s a simple example of how to define a sortable column in AG Grid:
“`javascript
const columnDefs = [
{ headerName: “Make”, field: “make”, sortable: true },
{ headerName: “Model”, field: “model”, sortable: true },
{ headerName: “Price”, field: “price”, sortable: true }
];
“`
Advanced Sorting Options
AG Grid supports more complex sorting options that can be customized based on specific requirements. These include multi-sorting, sorting with custom comparator functions, and using value getters.
Multi-Sorting: Users can sort by multiple columns by holding the Shift key while clicking the column headers. The order in which columns are clicked determines the sort priority.
Custom Sorters: If the default sorting does not meet your needs, AG Grid allows you to define custom sorting logic. You can create a comparator function for your columns to define how values should be compared. Below is an example of a custom sorting function:
“`javascript
function customSort(a, b) {
return a.length – b.length; // Sort by string length
}
const columnDefs = [
{ headerName: “Name”, field: “name”, sortable: true, comparator: customSort }
];
“`
Sorting API Methods
AG Grid provides a range of API methods to control sorting programmatically. The key methods include:
- api.sortColumn(column, sort)
- api.getSortModel()
- api.setSortModel(sortModel)
These methods can be used to manipulate the sort state of the grid dynamically.
Method | Description |
---|---|
sortColumn(column, sort) | Sorts the specified column in the given order (asc/desc). |
getSortModel() | Returns the current sort model, indicating which columns are sorted and their order. |
setSortModel(sortModel) | Sets the sort model, allowing you to define multiple columns and their sort orders. |
Sorting in Server-Side Row Model
For applications using AG Grid’s server-side row model, sorting can be managed on the server. When the user sorts a column, the grid sends a request to the server with the sort parameters, and the server is responsible for returning the sorted data.
To implement this, ensure that your server-side logic can handle sort requests. The grid will provide sorting information as follows:
“`javascript
gridOptions.api.getSortModel();
“`
This method retrieves the current sort model, which can be sent to the server to fetch the sorted dataset efficiently.
In summary, AG Grid’s sorting capabilities are robust and adaptable, allowing for both basic and advanced sorting configurations to meet various application needs.
Sorting in AG Grid
AG Grid provides robust options for sorting data within your grid. Sorting enhances data visibility and allows users to quickly find relevant information. The sorting feature can be customized extensively through grid properties.
Enabling Sorting
To enable sorting on columns, set the `sortable` property to `true` in your column definitions. This can be done as follows:
“`javascript
const columnDefs = [
{ headerName: “Make”, field: “make”, sortable: true },
{ headerName: “Model”, field: “model”, sortable: true },
{ headerName: “Price”, field: “price”, sortable: true }
];
“`
By default, AG Grid supports sorting on all columns that have this property enabled.
Sort Order
AG Grid supports both ascending and descending sort orders. The sort order can be configured through the `sort` property in the column definition. Here’s how to set it:
“`javascript
const columnDefs = [
{ headerName: “Make”, field: “make”, sortable: true, sort: ‘asc’ },
{ headerName: “Model”, field: “model”, sortable: true, sort: ‘desc’ }
];
“`
Multi-Sorting
AG Grid allows users to sort on multiple columns simultaneously. To enable multi-sorting, set the `suppressMultiSort` property to “. Users can then hold down the Shift key while clicking on column headers to add them to the sort criteria.
“`javascript
gridOptions = {
suppressMultiSort: ,
columnDefs: columnDefs
};
“`
Custom Sorting
For custom sorting logic, use the `comparator` function in the column definition. This function receives the values of the cells to compare and should return:
- A negative number if the first value is less than the second
- Zero if they are equal
- A positive number if the first value is greater than the second
Example of a custom comparator:
“`javascript
const columnDefs = [
{
headerName: “Custom Sort”,
field: “customField”,
sortable: true,
comparator: (valueA, valueB) => {
return valueA.length – valueB.length; // Sort by string length
}
}
];
“`
Sorting API
AG Grid provides a sorting API that allows programmatic control over sorting. Key methods include:
- `api.setSortModel(sortModel)`: Sets the current sort model.
- `api.getSortModel()`: Retrieves the current sort model.
Example of using the API to set sorting:
“`javascript
gridOptions.api.setSortModel([
{ colId: ‘make’, sort: ‘asc’ },
{ colId: ‘price’, sort: ‘desc’ }
]);
“`
Preserving Sorting State
AG Grid can preserve the sorting state even when data is refreshed. This can be achieved by using the `getSortModel` method to save the current sort state and then applying it after the data refresh.
“`javascript
const sortModel = gridOptions.api.getSortModel();
// After data refresh
gridOptions.api.setSortModel(sortModel);
“`
Sorting with Server-Side Data
When using server-side data, AG Grid can send sort parameters to the server for processing. The grid will handle the sorting based on the server response. Ensure that your server understands the sorting parameters provided by the grid.
“`javascript
const dataSource = {
getRows: (params) => {
const sortModel = params.sortModel;
// Send sortModel to your backend
}
};
“`
Implementing these features will enhance the user experience and ensure your data is easily navigable within AG Grid.
Expert Insights on Sorting in AG Grid
Dr. Emily Chen (Data Visualization Specialist, Tech Insights Journal). “Sorting in AG Grid is a powerful feature that allows users to manipulate data efficiently. By leveraging the grid’s built-in sorting capabilities, developers can enhance user experience significantly, ensuring that data presentation aligns with user needs.”
Michael Thompson (Frontend Development Lead, CodeCraft Solutions). “Implementing sorting in AG Grid is straightforward, yet it requires an understanding of the grid’s API. Utilizing the ‘sortable’ property on columns and managing the sort order programmatically can lead to a more dynamic and responsive interface.”
Sarah Patel (Senior Software Engineer, DataGrid Innovations). “To sort effectively in AG Grid, it is crucial to consider the data types being handled. Different data types may require specific sorting algorithms, and AG Grid offers customization options that allow developers to define sorting behavior tailored to their datasets.”
Frequently Asked Questions (FAQs)
How do I enable sorting in AG Grid?
To enable sorting in AG Grid, set the `sortable` property to `true` in the column definitions. This allows users to sort the data by clicking on the column headers.
Can I customize the sorting behavior in AG Grid?
Yes, AG Grid allows customization of sorting behavior by using the `comparator` function in the column definition. This function can define custom sorting logic based on specific requirements.
Is it possible to sort multiple columns in AG Grid?
AG Grid supports multi-column sorting. Users can hold down the Shift key while clicking on additional column headers to apply sorting on multiple columns simultaneously.
How can I programmatically sort data in AG Grid?
You can programmatically sort data by using the `api.sortColumn()` method. This method allows you to specify the column and the sort direction, enabling dynamic sorting based on application logic.
What happens to the sorting state when data is refreshed in AG Grid?
When data is refreshed, AG Grid maintains the current sorting state unless explicitly reset. If you want to clear sorting after a data refresh, you can call the `api.setSortModel([])` method.
Can I disable sorting for specific columns in AG Grid?
Yes, you can disable sorting for specific columns by setting the `sortable` property to “ in the column definition. This prevents users from sorting those columns.
Sorting in AG Grid is a powerful feature that enhances data management and user experience. AG Grid provides built-in sorting capabilities that allow users to organize data in ascending or descending order based on any column. This functionality can be easily implemented through grid options, and it supports both single and multi-column sorting, giving users flexibility in how they view their data.
To enable sorting, developers can set the `sortable` property to `true` for the desired columns in the grid configuration. Additionally, AG Grid offers various sorting algorithms, such as text, number, and date sorting, which can be customized to meet specific requirements. The grid also supports external sorting, allowing developers to implement custom sorting logic when necessary.
Moreover, AG Grid provides user-friendly features such as sorting icons and the ability to reset sorting with a simple click. This enhances the overall usability of the grid, making it easier for users to interact with large datasets. Overall, mastering sorting in AG Grid is essential for creating efficient and user-centric applications that facilitate data analysis.
Author Profile
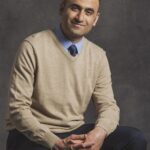
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?