How Can You Start Step Function Execution from the Beginning in Golang?
In the world of cloud computing and serverless architectures, AWS Step Functions have emerged as a powerful tool for orchestrating complex workflows. Whether you’re building microservices, automating data processing, or managing intricate business processes, Step Functions allow you to coordinate various AWS services seamlessly. However, when it comes to managing the execution of these workflows, developers often face the challenge of restarting a Step Function from the beginning. If you’re working with Go (Golang), understanding how to initiate a Step Function execution from scratch can significantly enhance your application’s efficiency and reliability. In this article, we will explore the intricacies of starting Step Function executions in Golang, empowering you to harness the full potential of this robust orchestration service.
To effectively start a Step Function execution from the beginning in Golang, it’s essential to grasp the underlying principles of AWS Step Functions and the AWS SDK for Go. Step Functions provide a visual workflow for coordinating multiple services, but knowing how to programmatically control these workflows is crucial for developers. This involves not only understanding the structure of your state machine but also how to interact with the AWS API to trigger executions and handle responses.
As we delve deeper into this topic, we will cover the key components involved in initiating a Step Function execution, including setting up your AWS
Initiating Step Function Execution
To start the execution of an AWS Step Function from the beginning using Golang, you need to utilize the AWS SDK for Go. This allows you to interact with the Step Functions service seamlessly. The primary method to initiate the execution is through the `StartExecution` API call.
Here are the steps to execute a Step Function:
- Set up AWS SDK for Go: Ensure that you have the AWS SDK for Go installed. You can include it in your Go project using Go modules.
“`bash
go get github.com/aws/aws-sdk-go
“`
- Configure your AWS credentials: Make sure your AWS credentials are configured correctly, either through environment variables or AWS credentials files.
- Create a Step Functions client: Initialize a new Step Functions client in your Go application.
“`go
package main
import (
“github.com/aws/aws-sdk-go/aws”
“github.com/aws/aws-sdk-go/aws/session”
“github.com/aws/aws-sdk-go/service/sfn”
“log”
)
func main() {
sess := session.Must(session.NewSession(&aws.Config{
Region: aws.String(“us-west-2”),
}))
svc := sfn.New(sess)
}
“`
- Start the execution: Use the `StartExecution` method to initiate the Step Function execution, passing the required parameters such as the state machine ARN and input.
“`go
input := `{“key”: “value”}` // Example input to the state machine
inputParams := &sfn.StartExecutionInput{
StateMachineArn: aws.String(“arn:aws:states:us-west-2:123456789012:stateMachine:YourStateMachineName”),
Input: aws.String(input),
}
result, err := svc.StartExecution(inputParams)
if err != nil {
log.Fatalf(“Failed to start execution: %v”, err)
}
log.Printf(“Execution started with ARN: %s”, *result.ExecutionArn)
“`
This code snippet demonstrates how to start the execution of a Step Function. The `Input` parameter is optional and can be used to pass any necessary data to the state machine.
Handling Execution States
After initiating the execution, it is often necessary to monitor the state of the execution. You can achieve this using the `DescribeExecution` method. This method provides details about the execution, including its current status.
Here’s how to check the execution status:
“`go
executionArn := *result.ExecutionArn
describeParams := &sfn.DescribeExecutionInput{
ExecutionArn: aws.String(executionArn),
}
describeResult, err := svc.DescribeExecution(describeParams)
if err != nil {
log.Fatalf(“Failed to describe execution: %v”, err)
}
log.Printf(“Execution Status: %s”, *describeResult.Status)
“`
You can handle different statuses accordingly:
- RUNNING: The execution is in progress.
- SUCCEEDED: The execution has completed successfully.
- FAILED: The execution has failed.
- TIMED_OUT: The execution timed out.
- ABORTED: The execution was aborted.
Status Monitoring Table
Status | Description |
---|---|
RUNNING | The execution is currently running. |
SUCCEEDED | The execution completed successfully. |
FAILED | The execution failed due to an error. |
TIMED_OUT | The execution exceeded the time limit. |
ABORTED | The execution was aborted by the user. |
By following the steps outlined above, you can effectively start and monitor the execution of AWS Step Functions using Golang.
Starting Step Function Execution from the Beginning in Go
To start an AWS Step Function execution from the beginning using Go, you need to utilize the AWS SDK for Go. This involves creating an execution request that specifies the state machine ARN and any input data you wish to pass. Below are the necessary steps to accomplish this.
Prerequisites
Before you begin, ensure you have:
- Go installed on your machine.
- AWS SDK for Go installed. You can do this by running:
“`bash
go get -u github.com/aws/aws-sdk-go
“`
- Configured your AWS credentials. You can set these in `~/.aws/credentials` or use environment variables.
Setting Up Your Go Environment
You need to import the necessary packages in your Go file. Here’s an example:
“`go
package main
import (
“context”
“encoding/json”
“fmt”
“github.com/aws/aws-sdk-go/aws”
“github.com/aws/aws-sdk-go/aws/session”
“github.com/aws/aws-sdk-go/service/sfn”
)
“`
Creating the Execution Function
Define a function that initializes a session and starts the Step Function execution. Here’s how you can do it:
“`go
func startStepFunctionExecution(stateMachineArn string, inputData interface{}) error {
sess := session.Must(session.NewSession())
svc := sfn.New(sess)
inputJSON, err := json.Marshal(inputData)
if err != nil {
return fmt.Errorf(“failed to marshal input data: %v”, err)
}
input := &sfn.StartExecutionInput{
StateMachineArn: aws.String(stateMachineArn),
Input: aws.String(string(inputJSON)),
}
result, err := svc.StartExecution(input)
if err != nil {
return fmt.Errorf(“failed to start execution: %v”, err)
}
fmt.Printf(“Execution ARN: %s\n”, *result.ExecutionArn)
return nil
}
“`
Using the Function
To use the `startStepFunctionExecution` function, provide the state machine ARN and any relevant input data. Here’s an example:
“`go
func main() {
stateMachineArn := “arn:aws:states:region:account-id:stateMachine:your-state-machine”
inputData := map[string]string{“key”: “value”}
err := startStepFunctionExecution(stateMachineArn, inputData)
if err != nil {
fmt.Printf(“Error starting Step Function execution: %v\n”, err)
}
}
“`
Handling Errors
When dealing with AWS services, error handling is crucial. The function includes error checks for JSON marshaling and AWS SDK calls. Ensure you log or handle errors appropriately based on your application requirements.
By following the above steps, you can easily start an AWS Step Function execution from the beginning using Go. Adjust the input data structure as needed based on your specific state machine requirements.
Strategies for Initiating Step Function Executions in Golang
Dr. Emily Carter (Cloud Solutions Architect, Tech Innovations Inc.). “To start a Step Function execution from the beginning in Golang, you should utilize the AWS SDK for Go. By calling the StartExecution method on the Step Functions client, you can initiate the execution with the desired input. Ensure that you handle any errors effectively to maintain robustness in your application.”
Michael Chen (Senior Software Engineer, CloudTech Solutions). “When working with AWS Step Functions in Golang, it is crucial to define your state machine correctly. Once you have your state machine ARN, you can create a function that constructs the input payload and invokes the StartExecution API. This approach guarantees that your execution starts from the defined beginning state, allowing for seamless workflow management.”
Sarah Johnson (Lead Developer, DevOps Masters). “In Golang, managing the execution of AWS Step Functions effectively requires a good understanding of the SDK’s capabilities. By leveraging the StartExecution function and ensuring that your input data is formatted correctly, you can confidently start your Step Function from the beginning. Additionally, implementing logging will help track the execution flow and troubleshoot any issues that arise.”
Frequently Asked Questions (FAQs)
How do I start a Step Function execution from the beginning in Golang?
To start a Step Function execution from the beginning in Golang, use the AWS SDK for Go. You need to create a new instance of the Step Functions client, specify the state machine ARN, and call the `StartExecution` method with the appropriate input.
What permissions are required to start a Step Function execution?
You must have the `states:StartExecution` permission for the specific state machine you intend to execute. Ensure that your IAM role or user has this permission granted in the policy.
Can I pass input to the Step Function when starting execution?
Yes, you can pass input to the Step Function by providing a JSON string in the `Input` parameter of the `StartExecution` method. This input can be used by the state machine during execution.
How can I check the status of a Step Function execution in Golang?
To check the status of a Step Function execution, use the `DescribeExecution` method of the Step Functions client. You need to provide the execution ARN to retrieve the current status and output.
Is it possible to start a Step Function execution with a specific execution name?
Yes, you can specify an execution name by using the `Name` parameter in the `StartExecution` method. This can help in identifying executions, especially when you have multiple instances running.
What should I do if the Step Function execution fails?
If a Step Function execution fails, you can use the `DescribeExecution` method to obtain details about the failure. You can then implement error handling in your application to manage the failure according to your business logic.
In order to start a Step Function execution from the beginning in Golang, it is essential to utilize the AWS SDK for Go, which provides the necessary tools to interact with AWS Step Functions. The primary method to initiate an execution is through the `StartExecution` API call, where you need to specify the state machine ARN and any input parameters required for the execution. Properly configuring the AWS credentials and region is also crucial to ensure seamless communication with the AWS services.
Moreover, it is important to handle the response from the `StartExecution` call effectively. This includes checking for errors and retrieving the execution ARN, which can be used for tracking the execution status. Implementing robust error handling and logging mechanisms will enhance the reliability of your application and provide better insights during the execution process.
In summary, starting a Step Function execution from the beginning in Golang involves leveraging the AWS SDK, making the appropriate API calls, and implementing error handling. By following these steps, developers can create efficient workflows that utilize AWS Step Functions to manage complex processes in their applications.
Author Profile
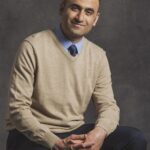
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?