How Can You Stop a Function in Python Effectively?
In the world of programming, functions are the building blocks of code, allowing developers to encapsulate logic and streamline their workflows. However, there are times when a function may need to be halted prematurely—whether due to an error, an unexpected condition, or simply the need to optimize performance. Understanding how to effectively stop a function in Python is not just a matter of good coding practice; it’s essential for creating robust and efficient applications. In this article, we will explore various techniques and strategies to gracefully terminate functions, ensuring your code runs smoothly and as intended.
When working with functions in Python, there are several scenarios where you might want to stop execution. For instance, you might encounter a situation where a function is processing data that doesn’t meet certain criteria, and continuing would lead to incorrect results or wasted resources. Additionally, functions that involve loops or long-running processes can benefit from mechanisms that allow for early termination, enhancing user experience and application performance.
In this exploration, we will delve into the different methods available for stopping a function, from using return statements to handling exceptions. Each approach has its own use cases and implications, and understanding these can empower you to write cleaner, more effective code. Whether you’re a seasoned developer or just starting out, mastering the art of function termination will elevate
Using Exceptions to Stop a Function
One of the most common methods to stop a function in Python is by raising an exception. This approach allows you to exit a function prematurely, particularly when you encounter an unexpected condition or error. You can define your own exceptions or use built-in ones like `ValueError` or `TypeError`.
Here’s an example of raising a built-in exception to stop a function:
“`python
def process_data(data):
if not isinstance(data, list):
raise TypeError(“Input must be a list”)
Function continues here if data is valid
“`
When the condition is met, the function will terminate, and the exception can be caught in the calling code.
Utilizing Return Statements
Another straightforward method to stop a function is by using the `return` statement. When `return` is executed, control is passed back to the caller, effectively ending the function’s execution.
Example of using return:
“`python
def check_number(num):
if num < 0:
return "Negative number, stopping execution."
return "Number is positive."
```
In this case, if the number is negative, the function will return a message and stop executing further.
Using Flags for Control Flow
Flags can also be used to control the execution flow of functions, particularly when dealing with loops or multiple operations within a function. This involves setting a variable to indicate whether the function should continue or stop.
Here’s an example:
“`python
def process_items(items):
stop_processing =
for item in items:
if item == ‘stop’:
stop_processing = True
break
print(item)
if stop_processing:
print(“Processing stopped.”)
“`
In this example, the function processes items until it encounters the string ‘stop’, at which point it sets a flag and breaks out of the loop.
Table of Stopping Mechanisms
Method | Description | Use Case |
---|---|---|
Exceptions | Terminate function with an error | Handling unexpected input |
Return Statement | Exit function and return a value | Conditional exit |
Flags | Control execution flow with a variable | Looping scenarios |
Using Decorators to Control Execution
Another advanced technique involves using decorators to control the execution of functions. A decorator can wrap a function and include logic to stop its execution based on certain conditions.
Example of a simple decorator:
“`python
def stop_if_negative(func):
def wrapper(num):
if num < 0:
print("Negative input, stopping execution.")
return
return func(num)
return wrapper
@stop_if_negative
def square(num):
return num * num
```
Here, the decorator checks for negative input and prevents the `square` function from executing if the condition is met.
These methods provide various ways to stop a function in Python, offering flexibility depending on the specific requirements of your application.
Using Exceptions to Stop a Function
In Python, one effective way to stop a function is by raising an exception. This technique allows you to interrupt the normal flow of execution and signal that an error or a specific condition has occurred. The following steps illustrate how to implement this approach:
- Define a custom exception (optional):
“`python
class StopFunctionException(Exception):
pass
“`
- Raise the exception within the function:
“`python
def example_function():
if some_condition:
raise StopFunctionException(“Stopping the function due to condition.”)
Additional logic here
“`
- Handle the exception outside the function:
“`python
try:
example_function()
except StopFunctionException as e:
print(e)
“`
This method allows for more controlled and meaningful stopping of functions while providing feedback on why the function was halted.
Using Return Statements
Another straightforward method to stop a function is by using the `return` statement. This method is particularly useful for terminating a function early when certain conditions are met.
- Basic usage:
“`python
def example_function():
if some_condition:
return Stops the function
Additional logic here
“`
- Returning values:
“`python
def example_function():
if some_condition:
return “Condition met, stopping function.”
return “Function executed successfully.”
“`
Utilizing `return` effectively allows you to break out of function execution without raising exceptions.
Using Flags to Control Execution Flow
Control flags can also be employed to manage whether a function continues executing or halts. This technique involves setting a variable that indicates whether the function should proceed.
- Example of using a flag:
“`python
def example_function():
should_continue = True
if some_condition:
should_continue =
if not should_continue:
return Function stops
Continue with additional logic
“`
This method provides flexibility, allowing for multiple checkpoints within the function to determine if it should continue processing.
Utilizing Decorators for Stopping Functions
Decorators can encapsulate stopping logic and can be reused across multiple functions. A decorator can be created to check conditions before executing the function.
- Example of a stopping decorator:
“`python
def stop_if(condition):
def decorator(func):
def wrapper(*args, **kwargs):
if condition:
print(“Function execution stopped.”)
return None
return func(*args, **kwargs)
return wrapper
return decorator
@stop_if(some_condition)
def example_function():
Function logic here
“`
This approach enhances code modularity and readability by separating stopping logic from business logic.
Using the `sys.exit()` Function
In cases where you want to terminate the entire program from within a function, you can use `sys.exit()`. This function raises a `SystemExit` exception, which terminates the program.
- Example:
“`python
import sys
def example_function():
if some_condition:
print(“Exiting the program.”)
sys.exit()
Additional logic here
“`
This method should be used with caution, as it will stop the entire Python interpreter, not just the function.
Expert Strategies for Stopping Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To stop a function in Python, one effective method is to use the ‘return’ statement. This allows you to exit the function immediately, returning control to the caller without executing any further lines of code within that function.”
Michael Chen (Software Engineer, CodeCraft Solutions). “In scenarios where you need to halt a function based on certain conditions, implementing exceptions can be particularly useful. By raising an exception, you can terminate the function’s execution and handle the error gracefully in the calling context.”
Laura Kim (Lead Python Instructor, LearnPython Academy). “Another approach to stopping a function is to utilize threading and the ‘Event’ class. By setting an event flag, you can signal a function to stop its execution, which is especially beneficial in long-running processes or when dealing with asynchronous tasks.”
Frequently Asked Questions (FAQs)
How can I terminate a function in Python before it completes?
You can use the `return` statement to exit a function prematurely. When `return` is executed, the function stops executing and control returns to the calling context.
Is there a way to stop a function that is running indefinitely?
To stop an indefinitely running function, you can use threading or multiprocessing to run the function in a separate thread or process. You can then terminate that thread or process using the appropriate methods.
Can I use exceptions to stop a function in Python?
Yes, you can raise an exception within a function to stop its execution. If the exception is not caught within the function, it will propagate up to the calling context, effectively halting the function.
What is the role of the `break` statement in stopping a function?
The `break` statement is used to exit loops, not functions. However, if a function contains a loop, you can use `break` to stop the loop, which may lead to the function terminating if no further code exists after the loop.
How can I stop a function from another function in Python?
You can use flags or shared variables to signal the function you want to stop. The function can periodically check this flag and exit if it detects that it should stop executing.
Are there any built-in functions to stop a function in Python?
Python does not provide built-in functions to forcibly stop a function. The best practice is to design your functions to handle termination gracefully using return statements or exceptions.
In Python, stopping a function can be achieved through various methods depending on the context and requirements of the program. The most straightforward way to terminate a function is by using the `return` statement, which exits the function and returns control to the caller. This method is particularly useful when you want to end the function early based on certain conditions, allowing for more control over the flow of execution.
Another approach to stopping a function is by raising exceptions. This is particularly useful in scenarios where an error or an unexpected condition occurs. By raising an exception, you can halt the function’s execution and propagate the error up the call stack, allowing for centralized error handling. It is essential to use exceptions judiciously to maintain code clarity and avoid unnecessary complexity.
For long-running functions or those that may need to be interrupted by user input, implementing a check within the function that listens for a specific signal or condition can be effective. This can involve using threading or asynchronous programming techniques to monitor for an interrupt signal, allowing the function to terminate gracefully when needed.
In summary, stopping a function in Python can be accomplished using the `return` statement, raising exceptions, or implementing interrupt checks. Each method serves different purposes and can be
Author Profile
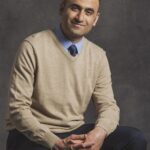
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?