How Can You Style a JavaScript Dropdown Using Styled Components?
In the world of modern web development, creating visually appealing and highly functional user interfaces is paramount. Among the myriad of UI components, dropdowns stand out as essential elements that enhance user experience by offering a streamlined way to select options. However, as developers, we often find ourselves grappling with the challenge of styling these dropdowns to match our unique design visions. Enter Styled Components, a powerful library that allows us to write CSS directly within our JavaScript, making it easier than ever to create dynamic and visually cohesive dropdown menus. In this article, we will explore how to effectively style dropdowns using Styled Components in JavaScript, transforming them from basic selections into eye-catching interactive elements.
Styled Components revolutionizes the way we approach styling in React applications by enabling component-level styles that are scoped and maintainable. This approach not only simplifies the styling process but also enhances the readability of our code. When it comes to dropdowns, using Styled Components allows developers to encapsulate styles within the component itself, ensuring that the dropdown’s appearance is consistent and easily adjustable. By leveraging props and themes, we can create versatile dropdowns that adapt to various design requirements, making them a perfect fit for any project.
As we delve deeper into the intricacies of styling dropdowns with Styled Components
Styling a Dropdown with Styled Components
To create a visually appealing dropdown using Styled Components in JavaScript, you can leverage the power of CSS-in-JS to manage styles directly within your component files. Here’s a breakdown of how to achieve this.
Creating a Basic Dropdown Component
Start by defining your dropdown component. This will consist of a button that, when clicked, reveals a list of options. You can use Styled Components to style both the button and the dropdown list.
“`javascript
import React, { useState } from ‘react’;
import styled from ‘styled-components’;
const DropdownContainer = styled.div`
position: relative;
display: inline-block;
`;
const DropdownButton = styled.button`
background-color: 4CAF50; /* Green */
color: white;
padding: 10px 20px;
font-size: 16px;
border: none;
cursor: pointer;
border-radius: 5px;
&:hover {
background-color: 45a049;
}
`;
const DropdownList = styled.ul`
display: ${props => (props.isOpen ? ‘block’ : ‘none’)};
position: absolute;
background-color: white;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
margin: 0;
padding: 0;
list-style-type: none;
border-radius: 5px;
`;
const DropdownItem = styled.li`
padding: 10px;
color: black;
&:hover {
background-color: f1f1f1;
cursor: pointer;
}
`;
“`
Implementing the Dropdown Logic
Within your component, manage the state to control the visibility of the dropdown list. This is typically done using React’s `useState` hook.
“`javascript
const Dropdown = () => {
const [isOpen, setIsOpen] = useState();
const toggleDropdown = () => {
setIsOpen(!isOpen);
};
return (
);
};
“`
Adding Accessibility Features
When creating dropdowns, it’s essential to consider accessibility. Below are some points to improve the dropdown’s accessibility:
– **Keyboard Navigation**: Allow users to navigate through options using keyboard arrow keys.
– **ARIA Attributes**: Add `aria-expanded` to indicate the dropdown state and `role=”listbox”` for screen readers.
– **Focus Management**: Ensure the dropdown can be closed with the `Escape` key.
Here’s an example of how to implement these features:
“`javascript
const Dropdown = () => {
const [isOpen, setIsOpen] = useState();
const toggleDropdown = () => {
setIsOpen(!isOpen);
};
const handleKeyDown = (event) => {
if (event.key === ‘Escape’) {
setIsOpen();
}
};
return (
Select an option
);
};
“`
Styling Options and Customization
You can further customize your dropdown by adjusting the colors, padding, and hover effects. Below is a table comparing basic styling options.
Property | Default Value | Description |
---|---|---|
Background Color | 4CAF50 | Color of the dropdown button |
Text Color | White | Color of the text in the dropdown button |
Hover Background Color | 45a049 | Color when hovering over the dropdown button |
Dropdown Background Color | White | Background color of the dropdown list |
By adjusting these properties, you can create a dropdown that aligns with your application’s design.
Creating a Styled Components Dropdown
To create a dropdown using Styled Components in a React application, you will utilize the flexibility of CSS-in-JS to style your components dynamically. Below are the steps and code snippets to implement a functional and styled dropdown.
Setting Up the Dropdown Component
- **Install Styled Components**: Ensure that you have Styled Components installed in your project. You can do this by running:
“`bash
npm install styled-components
“`
- **Create the Dropdown Component**: Start by defining a new component for the dropdown.
“`javascript
import React, { useState } from ‘react’;
import styled from ‘styled-components’;
const DropdownContainer = styled.div`
position: relative;
display: inline-block;
`;
const DropdownButton = styled.button`
background-color: 4CAF50; /* Green */
color: white;
padding: 10px;
font-size: 16px;
border: none;
cursor: pointer;
&:hover {
background-color: 45a049;
}
`;
const DropdownContent = styled.div`
display: ${props => (props.open ? ‘block’ : ‘none’)};
position: absolute;
background-color: white;
min-width: 160px;
box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2);
z-index: 1;
`;
const DropdownItem = styled.div`
color: black;
padding: 12px 16px;
text-decoration: none;
&:hover {
background-color: f1f1f1;
}
`;
const Dropdown = () => {
const [isOpen, setIsOpen] = useState();
const toggleDropdown = () => {
setIsOpen(!isOpen);
};
return (
Dropdown
);
};
export default Dropdown;
“`
Styling the Dropdown
The styling can be customized by modifying the properties in the styled components. Here are some key attributes you can adjust:
Styled Component | Property | Description |
---|---|---|
`DropdownButton` | `background-color` | Changes the background color of the button. |
`DropdownContent` | `box-shadow` | Adds a shadow effect to the dropdown. |
`DropdownItem` | `color` | Sets the text color for each dropdown item. |
`padding` | Adjusts spacing within each item. |
Handling Click Events
To enhance user experience, it is advisable to close the dropdown when a user clicks outside of it. You can achieve this by adding an event listener.
“`javascript
import { useEffect } from ‘react’;
const useOutsideClick = (ref, handler) => {
useEffect(() => {
const listener = (event) => {
if (!ref.current || ref.current.contains(event.target)) {
return;
}
handler();
};
document.addEventListener(‘mousedown’, listener);
return () => {
document.removeEventListener(‘mousedown’, listener);
};
}, [ref, handler]);
};
const Dropdown = () => {
const [isOpen, setIsOpen] = useState();
const ref = useRef();
useOutsideClick(ref, () => {
setIsOpen();
});
return (
Dropdown
);
};
“`
This implementation ensures that the dropdown remains user-friendly and visually appealing, enhancing the overall functionality of your application.
Expert Insights on Styling JavaScript Dropdowns with Styled Components
Emily Carter (Frontend Developer, Tech Innovations Inc.). “When styling dropdowns using Styled Components, it is crucial to leverage the power of props to create dynamic styles that respond to user interactions. This approach not only enhances the user experience but also maintains the scalability of your components.”
Michael Chen (UI/UX Designer, Creative Solutions Group). “Utilizing Styled Components for dropdowns allows for a clean separation of concerns. By encapsulating styles within the component, developers can ensure that their dropdowns are consistent across the application while still being customizable based on the context in which they are used.”
Laura Simmons (JavaScript Engineer, CodeCraft Academy). “To achieve a polished look for dropdowns, I recommend using transitions and animations within Styled Components. This not only makes the dropdowns visually appealing but also provides a smoother interaction for users, which is essential for modern web applications.”
Frequently Asked Questions (FAQs)
How can I create a dropdown using styled-components in JavaScript?
To create a dropdown using styled-components, define a styled component for the dropdown container and its items. Use the `select` and `option` HTML elements or custom components, applying styles through styled-components for customization.
What are styled-components in JavaScript?
Styled-components are a library for React that allows developers to use component-level styles in their applications. It utilizes tagged template literals to style components, enhancing modularity and maintainability.
Can I customize the dropdown styles with styled-components?
Yes, you can fully customize dropdown styles by creating styled components for each part of the dropdown, including the button and options. You can apply CSS properties like colors, fonts, and spacing to achieve the desired look.
How do I handle dropdown state in a styled-components dropdown?
To manage dropdown state, use React’s `useState` hook to track whether the dropdown is open or closed. Update the state based on user interactions, such as clicking the dropdown button or selecting an item.
Is it possible to use animations with styled-components for dropdowns?
Yes, you can implement animations in styled-components by using CSS transitions or animations. Define keyframes or transition properties within your styled components to create smooth opening and closing effects for the dropdown.
What are the best practices for accessibility in styled-components dropdowns?
Ensure accessibility by using semantic HTML elements, such as `button` for the dropdown trigger and `ul` for the options list. Implement keyboard navigation and ARIA attributes to enhance usability for users with disabilities.
styling a dropdown component using styled-components in JavaScript involves a combination of leveraging the capabilities of styled-components and understanding the structure of the dropdown itself. By creating styled components for the dropdown container, options, and any other necessary elements, developers can achieve a visually appealing and functional dropdown that aligns with their application’s design requirements. The use of styled-components allows for scoped styles, which helps in avoiding conflicts with other styles in the application.
Key takeaways include the importance of maintaining a clear hierarchy in the dropdown’s structure and ensuring that styles are applied consistently across different states, such as hover and active. Additionally, utilizing props within styled-components can enhance the interactivity and responsiveness of the dropdown, allowing for dynamic styling based on user interactions or application state. This approach not only improves user experience but also enhances code maintainability.
Furthermore, integrating accessibility features into the dropdown is crucial for ensuring that all users can interact with the component effectively. This includes managing keyboard navigation and providing appropriate ARIA attributes. By focusing on both aesthetics and functionality, developers can create dropdown components that are not only stylish but also user-friendly and inclusive.
Author Profile
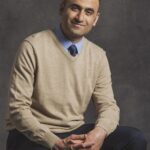
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?