How Can You Effectively Subscribe to a URL in Angular?
Understanding Subscription in Angular
In Angular, subscribing to a URL often involves making HTTP requests, typically through the `HttpClient` service. This service allows you to interact with REST APIs and handle asynchronous data streams effectively.
Setting Up HttpClient in Angular
To use `HttpClient`, ensure that you have imported the `HttpClientModule` in your application’s main module. Follow these steps:
- Open your `app.module.ts` file.
- Import `HttpClientModule` from `@angular/common/http`.
- Add `HttpClientModule` to the `imports` array.
“`typescript
import { HttpClientModule } from ‘@angular/common/http’;
@NgModule({
declarations: [
// your components
],
imports: [
HttpClientModule,
// other modules
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
“`
Creating a Service for HTTP Requests
To manage your HTTP requests efficiently, create a service. Here’s how:
- Generate a service using Angular CLI:
“`bash
ng generate service my-service
“`
- Implement the HTTP request in the service. For example, to subscribe to a URL:
“`typescript
import { Injectable } from ‘@angular/core’;
import { HttpClient } from ‘@angular/common/http’;
import { Observable } from ‘rxjs’;
@Injectable({
providedIn: ‘root’
})
export class MyService {
private apiUrl = ‘https://api.example.com/data’;
constructor(private http: HttpClient) { }
getData(): Observable
return this.http.get
}
}
“`
Subscribing to the Observable
Once the service is set up, you can subscribe to the data in any component. Here’s how to do it:
- Inject the service into your component.
- Call the method and subscribe to the observable.
“`typescript
import { Component, OnInit } from ‘@angular/core’;
import { MyService } from ‘./my-service.service’;
@Component({
selector: ‘app-my-component’,
templateUrl: ‘./my-component.component.html’,
})
export class MyComponent implements OnInit {
data: any;
constructor(private myService: MyService) { }
ngOnInit(): void {
this.myService.getData().subscribe(
(response) => {
this.data = response;
console.log(‘Data received:’, this.data);
},
(error) => {
console.error(‘Error fetching data’, error);
}
);
}
}
“`
Handling Responses and Errors
When subscribing to an observable, it is crucial to manage both successful responses and potential errors. Use the following strategies:
– **Success Callback**: Process the response data as needed.
– **Error Callback**: Handle any errors gracefully, possibly informing the user.
“`typescript
this.myService.getData().subscribe(
(response) => {
// Handle successful response
},
(error) => {
// Handle error
}
);
“`
Unsubscribing from Observables
To prevent memory leaks, it’s important to unsubscribe from observables when they are no longer needed. Utilize the `ngOnDestroy` lifecycle hook for cleanup.
“`typescript
import { Subscription } from ‘rxjs’;
export class MyComponent implements OnInit, OnDestroy {
private subscription: Subscription;
ngOnInit(): void {
this.subscription = this.myService.getData().subscribe(
// success and error handling
);
}
ngOnDestroy(): void {
this.subscription.unsubscribe();
}
}
“`
Best Practices for HTTP Requests
- Always handle errors to improve user experience.
- Use environment variables for URLs to avoid hardcoding.
- Consider using `async`/`await` with `toPromise()` for cleaner code in some scenarios.
- Leverage Angular’s built-in interceptors for common tasks such as logging and authentication.
By following these practices and structures, you can effectively subscribe to URLs and manage data in Angular applications.
Expert Insights on Subscribing to URLs in Angular
Dr. Emily Carter (Senior Software Engineer, Angular Development Group). “To effectively subscribe to a URL in Angular, developers should utilize the HttpClient module, which provides a robust way to handle HTTP requests. It is essential to manage subscriptions properly to avoid memory leaks, particularly when dealing with observable streams.”
Michael Chen (Frontend Architect, Tech Innovations Inc.). “Using Angular’s Router, developers can subscribe to URL changes by leveraging the ActivatedRoute service. This allows for dynamic updates to the component based on the current route, ensuring a responsive user experience.”
Sarah Thompson (Angular Consultant, CodeCraft Solutions). “It is crucial to implement unsubscribe logic in Angular applications when working with observables. Utilizing the takeUntil operator or the AsyncPipe can greatly enhance performance and maintainability when subscribing to URL changes.”
Frequently Asked Questions (FAQs)
How do I subscribe to a URL in Angular?
To subscribe to a URL in Angular, you typically use the HttpClient service. First, inject HttpClient into your component or service, then use the `get()` method to make a request to the desired URL. Finally, subscribe to the observable returned by the `get()` method to handle the response.
What is the purpose of subscribing to a URL in Angular?
Subscribing to a URL allows you to initiate an HTTP request and handle the response asynchronously. This is essential for retrieving data from a server, enabling dynamic content updates in your application.
Can I subscribe to multiple URLs simultaneously in Angular?
Yes, you can subscribe to multiple URLs simultaneously using the `forkJoin` operator from RxJS. This operator allows you to combine multiple observables and wait for all of them to complete before processing the results.
What happens if I do not unsubscribe from an HTTP request in Angular?
If you do not unsubscribe from an HTTP request, it may lead to memory leaks in your application. Although Angular automatically handles HTTP observables, it’s a good practice to manage subscriptions, especially for long-lived components.
How can I handle errors when subscribing to a URL in Angular?
You can handle errors by providing an error callback in the `subscribe()` method. Additionally, you can use the `catchError` operator from RxJS within your service to manage errors more gracefully and return a user-friendly response.
Is it possible to use async/await syntax with HTTP requests in Angular?
Yes, you can use async/await syntax with HTTP requests in Angular by converting the observable to a promise using the `toPromise()` method. This allows you to write asynchronous code in a more synchronous style, improving readability.
Subscribing to a URL in Angular typically involves utilizing the HttpClient module to make HTTP requests to a specified endpoint. This process allows developers to interact with external APIs or services, retrieve data, and manage it effectively within their Angular applications. By leveraging observables provided by the HttpClient, developers can subscribe to these requests to handle asynchronous data streams, enabling a reactive programming approach that is essential for modern web applications.
To successfully subscribe to a URL, developers should first import the HttpClientModule in their application module. Following this, they can inject the HttpClient service into their components or services. By calling methods such as `get()`, `post()`, or `put()`, developers can initiate requests to the desired URL and subscribe to the observable returned. This subscription allows them to process the response data, handle errors, and implement loading states, thereby enhancing user experience.
subscribing to a URL in Angular is a straightforward yet powerful technique that facilitates seamless data retrieval and interaction with external services. By understanding the fundamentals of HttpClient and observables, developers can create dynamic applications that respond efficiently to user actions and data changes. Mastering this aspect of Angular development is crucial for building robust, data-driven applications that meet the
Author Profile
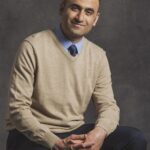
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?