How Can You Easily Switch to Another Python Version?
Switching between different versions of Python can be a crucial skill for developers, data scientists, and anyone working with Python-based applications. As projects evolve, so do their dependencies, and sometimes, a specific version of Python is required to ensure compatibility or to leverage new features. Whether you’re looking to test your code against multiple Python versions or you need to maintain legacy systems, knowing how to switch between Python versions seamlessly can save you time and headaches.
In the world of programming, flexibility is key. Python’s versatility allows developers to choose from a variety of versions, each with its own set of features and improvements. However, managing these versions can be daunting, especially for those who are new to the language or who are used to a single version. Fortunately, there are several tools and methods available that can simplify this process, allowing you to easily switch between versions without disrupting your workflow.
From using version management tools like pyenv to leveraging built-in features in popular IDEs, the options for switching Python versions are plentiful. Understanding these methods not only enhances your productivity but also empowers you to work on diverse projects with varying requirements. In the following sections, we will delve deeper into the practical steps and tools that can help you navigate the world of Python version management with confidence.
Using Pyenv to Manage Python Versions
One of the most effective methods for switching between different Python versions is by using `pyenv`. This tool allows you to easily install multiple Python versions and switch between them as needed. To use `pyenv`, follow these steps:
- Install Pyenv: You can install `pyenv` using the following command on Unix-based systems:
bash
curl https://pyenv.run | bash
- Add Pyenv to your shell: Update your shell configuration file (e.g., `.bashrc`, `.zshrc`) with the following lines:
bash
export PATH=”$HOME/.pyenv/bin:$PATH”
eval “$(pyenv init –path)”
eval “$(pyenv init -)”
eval “$(pyenv virtualenv-init -)”
- Restart your shell: Apply the changes by restarting your terminal or running `source ~/.bashrc` or `source ~/.zshrc`.
- Install a specific Python version: Use `pyenv` to install the desired version:
bash
pyenv install 3.9.7
- Set the global or local Python version: You can set the default Python version for all projects or just for a specific one:
- To set globally:
bash
pyenv global 3.9.7
- To set locally (in a project directory):
bash
pyenv local 3.9.7
Using Virtual Environments
Another approach to manage multiple Python versions is through virtual environments. Virtual environments allow you to create isolated environments for different projects, each with its own dependencies and Python version. Here’s how to create a virtual environment with a specific Python version:
- Install the desired Python version: Ensure you have the target version installed on your system. You can check installed versions with:
bash
python –version
- Create a virtual environment: Use the `venv` module or `virtualenv` to create a virtual environment with a specific Python version:
bash
python3.9 -m venv myenv
- Activate the virtual environment: To start using the environment, activate it with:
- On macOS/Linux:
bash
source myenv/bin/activate
- On Windows:
cmd
myenv\Scripts\activate
- Deactivate the virtual environment: When done, you can deactivate it with:
bash
deactivate
Comparison of Version Management Tools
Tool | Description | Advantages | Disadvantages |
---|---|---|---|
Pyenv | Manages multiple Python versions | Easy switching, lightweight | Requires additional setup |
Virtualenv | Creates isolated environments for projects | Dependency management | Needs to be activated manually |
Conda | Package manager that manages environments and dependencies | Cross-language support | Heavier than other tools |
Using Docker for Version Isolation
For those who prefer containerization, Docker can be used to run applications in isolated environments with specific Python versions. This approach is particularly useful for deploying applications in production. Here’s how to use Docker for Python version management:
- Create a Dockerfile: Define the Python version in a `Dockerfile`:
Dockerfile
FROM python:3.9
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD [“python”, “app.py”]
- Build the Docker image: Run the following command to build the image:
bash
docker build -t my-python-app .
- Run the container: Start a container from the built image:
bash
docker run my-python-app
By utilizing these methods, you can effectively manage and switch between different Python versions according to your project requirements.
Using pyenv for Python Version Management
One of the most efficient ways to switch between different Python versions is by using `pyenv`. This tool allows you to easily manage multiple Python versions and set global or local versions for your projects.
Installation Steps:
- Install Dependencies: Depending on your operating system, you may need to install some dependencies. For instance, on Ubuntu, you would run:
bash
sudo apt update
sudo apt install -y build-essential libssl-dev libbz2-dev libreadline-dev libsqlite3-dev wget curl llvm libffi-dev liblzma-dev python-openssl git
- Install pyenv:
You can install `pyenv` using Git:
bash
curl https://pyenv.run | bash
- Configure Your Shell:
Add the following lines to your `.bashrc` or `.zshrc` file:
bash
export PATH=”$HOME/.pyenv/bin:$PATH”
eval “$(pyenv init –path)”
eval “$(pyenv init -)”
eval “$(pyenv virtualenv-init -)”
- Restart Your Shell:
Reload your shell configuration:
bash
source ~/.bashrc
Switching Python Versions:
- To see all available Python versions:
bash
pyenv install –list
- To install a specific version:
bash
pyenv install 3.8.10
- To set a global Python version:
bash
pyenv global 3.8.10
- To set a local Python version for a specific project:
bash
cd your_project_directory
pyenv local 3.9.5
Checking the Current Version:
You can check which Python version you are currently using with:
bash
python –version
Using Virtual Environments
Virtual environments allow you to create isolated environments for different projects, enabling you to manage dependencies and Python versions separately.
**Creating a Virtual Environment**:
- **Using venv**:
To create a virtual environment, use:
bash
python3 -m venv myenv
- **Activating the Environment**:
- On Windows:
bash
myenv\Scripts\activate
- On macOS/Linux:
bash
source myenv/bin/activate
- **Installing a Specific Python Version**:
If you need a specific Python version, ensure you have it installed and then create the virtual environment with that version:
bash
python3.8 -m venv myenv
- **Deactivating the Environment**:
To deactivate the virtual environment, simply run:
bash
deactivate
**Managing Dependencies**:
Once inside a virtual environment, you can install packages using `pip` without affecting other projects.
- To install a package:
bash
pip install package_name
- To freeze your dependencies:
bash
pip freeze > requirements.txt
Using Docker for Python Version Isolation
Docker allows you to run applications in isolated containers, which can include specific Python versions.
Setting Up a Docker Container:
- Install Docker: Follow the instructions on the official Docker website for your operating system.
- Create a Dockerfile:
Here’s an example of a simple Dockerfile specifying a Python version:
dockerfile
FROM python:3.9-slim
WORKDIR /app
COPY . .
RUN pip install -r requirements.txt
CMD [“python”, “your_script.py”]
- Build the Docker Image:
Run the following command in the directory where your Dockerfile is located:
bash
docker build -t my-python-app .
- Run the Container:
To start your container:
bash
docker run my-python-app
This approach provides a completely isolated environment, ensuring that dependencies and Python versions do not conflict.
Expert Insights on Switching Python Versions
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Switching Python versions can be streamlined by utilizing tools such as `pyenv`, which allows for easy management of multiple Python installations. It is essential to ensure that your environment is configured correctly to avoid conflicts between versions.
Mark Thompson (Lead Developer, Open Source Projects). When transitioning to another Python version, it is crucial to review your existing codebase for compatibility issues. Utilizing virtual environments can help isolate dependencies and ensure that your application runs smoothly on the new version without affecting other projects.
Lisa Chen (Python Educator and Author). I recommend using `pipenv` or `venv` to create isolated environments for different Python versions. This practice not only simplifies the process of switching versions but also enhances project organization and dependency management.
Frequently Asked Questions (FAQs)
How can I check which Python versions are installed on my system?
You can check installed Python versions by running the command `python –version` or `python3 –version` in your terminal or command prompt. Additionally, you can use `py -0` on Windows to list all installed versions.
What tools can I use to manage multiple Python versions?
Common tools for managing multiple Python versions include `pyenv`, `Anaconda`, and `virtualenv`. These tools allow you to easily switch between different Python versions and manage environments.
How do I switch Python versions using pyenv?
To switch Python versions with `pyenv`, first install the desired version using `pyenv install
Can I switch Python versions in a virtual environment?
Yes, you can switch Python versions in a virtual environment by creating a new environment with the desired version. Use `python -m venv
What should I do if my scripts are not compatible with the new Python version?
If your scripts are not compatible with the new Python version, you may need to modify them to address any deprecated features or syntax changes. Consult the official Python documentation for guidance on migrating code between versions.
Is it possible to switch Python versions on Windows?
Yes, you can switch Python versions on Windows by using the Python Launcher (`py`). You can specify the version by running commands like `py -3.8 script.py` to execute a script with Python 3.8. Alternatively, you can use tools like `pyenv-win` for version management.
Switching to another Python version can be essential for compatibility with specific projects or libraries. Users have several methods at their disposal, including using version management tools like Pyenv, Conda, or virtual environments. Each of these methods provides a structured approach to managing multiple Python installations, making it easier to switch between versions as needed.
Pyenv is particularly popular for its simplicity and effectiveness, allowing users to install and switch between different Python versions seamlessly. Conda, on the other hand, is a robust package and environment management system that not only allows for version switching but also manages dependencies efficiently. Virtual environments, created using tools like venv or virtualenv, provide isolated spaces for projects, ensuring that each project can use its required Python version without interference.
In summary, the choice of method for switching Python versions largely depends on the user’s specific needs and workflow preferences. Understanding the advantages of each approach can significantly enhance productivity and ensure that projects run smoothly across different environments. By leveraging these tools, developers can maintain flexibility and control over their development setups.
Author Profile
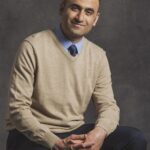
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?