How Can You Effectively Test an SMTP Server Using PowerShell?
In today’s digital landscape, email remains a cornerstone of communication for businesses and individuals alike. However, ensuring that your email system operates smoothly is crucial to maintaining effective communication. One of the key components of this system is the Simple Mail Transfer Protocol (SMTP) server, which is responsible for sending and receiving emails. But how do you verify that your SMTP server is functioning correctly? Enter PowerShell, a powerful scripting language that can help you test and troubleshoot your SMTP server with ease. In this article, we will explore the essential techniques and commands that will empower you to assess your SMTP server’s performance and reliability.
Testing an SMTP server using PowerShell can streamline the process of diagnosing email issues, allowing you to quickly identify and resolve problems that may disrupt your communication flow. By leveraging PowerShell’s robust capabilities, you can perform a variety of tests, from checking server connectivity to sending test emails. This not only enhances your troubleshooting efficiency but also provides a deeper understanding of your email infrastructure.
Whether you are an IT professional managing a corporate email system or a tech-savvy individual wanting to ensure your personal email setup is robust, mastering SMTP testing with PowerShell can be a game-changer. In the following sections, we will delve into the tools and techniques necessary to effectively test
Testing SMTP Server with PowerShell
To test an SMTP server using PowerShell, you can utilize the `System.Net.Mail` namespace, which provides classes for sending emails and interacting with the SMTP server. This method allows you to verify server connectivity and functionality directly from your PowerShell console.
First, you will need to create an SMTP client object, configure it with the server’s details, and send a test email. Here’s a straightforward example:
“`powershell
Define SMTP server and credentials
$smtpServer = “smtp.yourserver.com”
$smtpPort = 587
$smtpUser = “yourusername”
$smtpPass = “yourpassword”
Create the MailMessage object
$mailMessage = New-Object System.Net.Mail.MailMessage
$mailMessage.From = “[email protected]”
$mailMessage.To.Add(“[email protected]”)
$mailMessage.Subject = “Test Email”
$mailMessage.Body = “This is a test email sent using PowerShell.”
Create the SmtpClient object
$smtpClient = New-Object Net.Mail.SmtpClient($smtpServer, $smtpPort)
$smtpClient.EnableSsl = $true
$smtpClient.Credentials = New-Object System.Net.NetworkCredential($smtpUser, $smtpPass)
Send the email
try {
$smtpClient.Send($mailMessage)
Write-Host “Email sent successfully.”
} catch {
Write-Host “Failed to send email: $_”
}
“`
This script defines the SMTP server settings, including the server address, port, and user credentials. The `MailMessage` object is created to represent the email being sent, and the `SmtpClient` object manages the sending process.
SMTP Server Testing Options
In addition to sending test emails, you can perform connectivity tests to ensure that the SMTP server is reachable. Here are some common methods:
- Telnet Command: Use Telnet to connect to the SMTP server on the specified port.
- PowerShell Test-NetConnection: Use the `Test-NetConnection` cmdlet to check the server’s response.
Here’s how to use these methods:
Using Telnet:
- Open Command Prompt.
- Type `telnet smtp.yourserver.com 587` and press Enter.
- If connected, you will see a response indicating that the connection was successful.
Using Test-NetConnection:
“`powershell
Test-NetConnection -ComputerName smtp.yourserver.com -Port 587
“`
This command checks the connectivity to the SMTP server, returning information such as the TCP connection status.
SMTP Configuration Table
To aid in setting up your SMTP client, refer to the following configuration table:
Parameter | Description | Example Value |
---|---|---|
SMTP Server | The address of the SMTP server. | smtp.yourserver.com |
Port | The port number used for SMTP communication. | 587 |
Username | Authentication username for the SMTP server. | yourusername |
Password | Authentication password for the SMTP server. | yourpassword |
SSL | Indicates if SSL should be used for secure communication. | true |
Utilizing these tools and methods, you can effectively test the functionality and connectivity of your SMTP server using PowerShell.
Testing SMTP Server Using PowerShell
To test an SMTP server using PowerShell, you can utilize the `System.Net.Mail` namespace, which allows you to create and send emails programmatically. This method is effective for verifying SMTP server connectivity and functionality.
Prerequisites
Before conducting tests, ensure you have the following:
- PowerShell installed on your system.
- Access to the SMTP server with the correct hostname and port.
- Valid credentials, if the SMTP server requires authentication.
- An email address for the sender and recipient.
Basic SMTP Test Script
You can create a simple script to send a test email. Below is a sample PowerShell script for testing the SMTP server.
“`powershell
Define SMTP server details
$smtpServer = “smtp.yourserver.com”
$smtpPort = 587
$smtpUser = “yourusername”
$smtpPass = “yourpassword”
$from = “[email protected]”
$to = “[email protected]”
$subject = “SMTP Test Email”
$body = “This is a test email sent from PowerShell.”
Create a mail message object
$mailMessage = New-Object System.Net.Mail.MailMessage
$mailMessage.From = $from
$mailMessage.To.Add($to)
$mailMessage.Subject = $subject
$mailMessage.Body = $body
Create an SMTP client and send the email
$smtpClient = New-Object Net.Mail.SmtpClient($smtpServer, $smtpPort)
$smtpClient.EnableSsl = $true
$smtpClient.Credentials = New-Object System.Net.NetworkCredential($smtpUser, $smtpPass)
try {
$smtpClient.Send($mailMessage)
Write-Host “Email sent successfully.”
} catch {
Write-Host “Failed to send email: $_”
}
“`
Script Breakdown
Component | Description |
---|---|
`$smtpServer` | The hostname or IP address of your SMTP server. |
`$smtpPort` | The port number (commonly 25, 587, or 465). |
`$smtpUser` | Username for authentication, if required. |
`$smtpPass` | Password for authentication. |
`$from` | The sender’s email address. |
`$to` | The recipient’s email address. |
`$subject` | Subject line of the test email. |
`$body` | Body content of the email. |
`EnableSsl` | Set to `$true` to use SSL for the connection. |
`Send` method | Sends the email and handles exceptions. |
Handling Errors
When testing your SMTP server, you may encounter various errors. Common issues include:
- Authentication Errors: Ensure credentials are correct.
- Connection Errors: Verify the SMTP server address and port.
- SSL/TLS Errors: Check if the SMTP server requires SSL/TLS and adjust settings accordingly.
- Firewall Issues: Ensure that your firewall allows outgoing connections on the SMTP port.
To provide more detailed error information, you can enhance your error handling in the script:
“`powershell
catch {
Write-Host “Failed to send email: $_.Exception.Message”
}
“`
This modification will display the specific error message associated with the failure, aiding in troubleshooting.
Testing Without Sending Emails
If you need to verify SMTP server functionality without sending actual emails, consider using the `Telnet` command or specialized PowerShell modules like `MailKit`. However, this approach requires more technical knowledge and setup. Use the above script for straightforward email testing scenarios.
Expert Insights on Testing SMTP Servers with PowerShell
Jordan Miller (Senior Network Engineer, Tech Solutions Inc.). “Testing an SMTP server using PowerShell is essential for ensuring reliable email delivery. Utilizing the ‘Send-MailMessage’ cmdlet allows you to quickly validate SMTP connectivity and troubleshoot issues effectively.”
Lisa Chen (IT Security Consultant, CyberSafe Advisors). “When testing SMTP servers, it is crucial to consider security protocols. PowerShell can be leveraged to test both secure and non-secure connections, ensuring that your configurations comply with best practices for email security.”
Mark Thompson (Systems Administrator, CloudTech Solutions). “I recommend using PowerShell scripts to automate SMTP testing. By creating a script that checks for successful connections and validates responses from the server, you can streamline your monitoring process and quickly identify any issues.”
Frequently Asked Questions (FAQs)
How can I test an SMTP server using PowerShell?
You can test an SMTP server using PowerShell by utilizing the `Send-MailMessage` cmdlet. This cmdlet allows you to send a test email to verify the server’s functionality.
What parameters are required for the Send-MailMessage cmdlet?
The essential parameters include `-SmtpServer` (to specify the SMTP server address), `-From` (the sender’s email address), `-To` (the recipient’s email address), and `-Subject` (the email subject). Optionally, you can include `-Body` and `-Credential` for authentication.
Can I check the SMTP server response using PowerShell?
Yes, you can check the SMTP server response by using the `Test-NetConnection` cmdlet with the `-Port` parameter set to 25, 587, or 465, depending on the server configuration. This will help determine if the server is reachable.
What if I encounter an error while testing the SMTP server?
If you encounter an error, ensure that the SMTP server address is correct, the required ports are open, and your firewall settings allow outbound connections. Additionally, verify your credentials if authentication is required.
Is it possible to send attachments using PowerShell when testing SMTP?
Yes, you can send attachments by using the `-Attachments` parameter in the `Send-MailMessage` cmdlet. Specify the file path of the attachment you wish to include in the email.
How can I automate SMTP testing using PowerShell scripts?
You can automate SMTP testing by creating a PowerShell script that includes the `Send-MailMessage` cmdlet and any necessary logic to handle multiple test cases, logging results, and error handling for comprehensive testing.
Testing an SMTP server using PowerShell is a straightforward process that can help ensure your email server is functioning correctly. By leveraging PowerShell’s capabilities, users can send test emails and verify the server’s response. This is particularly useful for troubleshooting issues related to email delivery and server configuration. The process typically involves creating a simple script that utilizes the .NET framework to establish a connection to the SMTP server, authenticate if necessary, and send a test message.
One of the key takeaways from this discussion is the importance of understanding the SMTP protocol and the parameters required for a successful connection. Users should be familiar with the server address, port number, and any authentication credentials needed. Additionally, knowing how to interpret the responses from the SMTP server can provide valuable insights into potential issues, such as incorrect settings or server downtime.
Moreover, the flexibility of PowerShell allows for the automation of testing tasks, making it easier for administrators to regularly check the health of their SMTP servers. By creating scripts that can be scheduled to run at intervals, organizations can proactively monitor their email systems and address any issues before they impact users. Overall, testing an SMTP server with PowerShell is an essential skill for IT professionals managing email infrastructure.
Author Profile
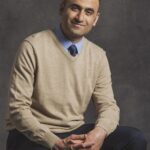
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?