How Can You Train an AI Assistant Using JavaScript?
### Introduction
In the rapidly evolving landscape of technology, artificial intelligence has emerged as a transformative force, reshaping how we interact with machines. One of the most intriguing applications of AI is the development of intelligent assistants that can understand and respond to human queries with remarkable accuracy. If you’re a developer looking to harness the power of AI in your projects, learning how to train an AI assistant in JavaScript can open up a world of possibilities. This guide will take you through the essential concepts and techniques needed to create a responsive, intelligent assistant that can enhance user experience and streamline tasks.
Training an AI assistant involves a blend of programming skills, an understanding of natural language processing (NLP), and the ability to work with various machine learning frameworks. JavaScript, being one of the most popular programming languages, offers a variety of libraries and tools that make it accessible for developers at all levels. From setting up the foundational architecture to implementing advanced features, the journey of training an AI assistant is both challenging and rewarding.
As you delve deeper into this topic, you’ll discover how to leverage JavaScript’s capabilities to build an AI assistant that not only understands user intent but also learns and adapts over time. With the right approach, you can create a virtual companion that enhances productivity, provides valuable
Understanding the Basics of AI Training
To effectively train an AI assistant in JavaScript, it is crucial to understand the foundational concepts that govern machine learning and natural language processing (NLP). At its core, AI training involves feeding data into algorithms that allow the model to learn patterns and make predictions or decisions based on that data.
Key components to consider include:
- Data Quality: High-quality, relevant data is essential for effective training. This data can include text, audio, or visual information, depending on the assistant’s capabilities.
- Model Selection: Different models can be used for various tasks (e.g., classification, regression). Selecting the right model based on the desired functionality is crucial.
- Training Process: This encompasses data preprocessing, model training, evaluation, and fine-tuning.
Setting Up Your Development Environment
To start training an AI assistant in JavaScript, you need to establish a suitable development environment. This typically involves:
- Node.js: A JavaScript runtime that allows you to run JavaScript on the server side.
- Machine Learning Libraries: Popular libraries include TensorFlow.js and Brain.js, which provide tools for building and training models directly in JavaScript.
Here’s a simple setup guide:
- Install Node.js from the official website.
- Create a new project directory and initialize a new Node.js project with `npm init`.
- Install necessary libraries:
bash
npm install @tensorflow/tfjs brain.js
Data Collection and Preparation
Data is the lifeblood of any AI training process. The following steps should be taken to ensure that your data is appropriate for training your AI assistant:
- Collect Data: Gather data relevant to the tasks you want your assistant to perform. This could include FAQs, conversation transcripts, or user queries.
- Clean Data: Remove any irrelevant or duplicate entries, and standardize formats.
- Annotate Data: Label data if necessary, particularly for supervised learning tasks.
The preparation phase can be summarized as follows:
Step | Description |
---|---|
Data Collection | Gather data from reliable sources. |
Data Cleaning | Remove noise and irrelevant information. |
Data Annotation | Label data to aid in supervised learning. |
Implementing the Training Algorithm
Once the data is prepared, you can begin implementing the training algorithm. This process typically involves:
– **Model Definition**: Define the architecture of the neural network or the algorithm you will use.
– **Training Loop**: Implement a loop that feeds data into the model, calculates loss, and updates model weights.
– **Validation**: After training, validate your model using a separate dataset to assess its performance.
Here’s a simplified code snippet using TensorFlow.js:
javascript
const tf = require(‘@tensorflow/tfjs’);
// Define a simple model
const model = tf.sequential();
model.add(tf.layers.dense({units: 1, inputShape: [1]}));
// Compile the model
model.compile({loss: ‘meanSquaredError’, optimizer: ‘sgd’});
// Prepare data
const xs = tf.tensor2d([1, 2, 3, 4], [4, 1]);
const ys = tf.tensor2d([1, 3, 5, 7], [4, 1]);
// Train the model
model.fit(xs, ys, {epochs: 10}).then(() => {
// Use the model for predictions
model.predict(tf.tensor2d([5], [1, 1])).print();
});
This code demonstrates the basic structure of setting up and training a simple model. Adjustments can be made based on the complexity of your task and the nature of your data.
By following these steps, you will be well on your way to developing an AI assistant capable of understanding and responding to user queries effectively.
Understanding AI Training Concepts
To train an AI assistant effectively in JavaScript, it is crucial to understand the foundational concepts behind AI and machine learning. Key elements include:
- Data Collection: Gathering relevant datasets that the AI can learn from. This could involve user queries, responses, and interactions.
- Model Selection: Choosing an appropriate machine learning model based on the task, such as natural language processing (NLP) for conversational agents.
- Feature Engineering: Identifying and creating relevant features from raw data that will help the model learn efficiently.
- Training and Validation: Splitting the data into training and validation sets to evaluate the model’s performance.
Setting Up the Environment
To develop an AI assistant in JavaScript, the following environment setup is recommended:
Component | Description |
---|---|
Node.js | A JavaScript runtime for building server-side applications. |
NPM (Node Package Manager) | For managing libraries and dependencies. |
TensorFlow.js | A library for training and deploying machine learning models in the browser and Node.js. |
Natural Language Processing Libraries | Such as compromise or natural for parsing and understanding text. |
Building the Model
The model building process can be broken down into several steps:
- Data Preparation:
- Clean and preprocess the data.
- Tokenize the text and convert it into numerical format.
- Model Definition:
- Use TensorFlow.js to define your model architecture. For example:
javascript
const model = tf.sequential();
model.add(tf.layers.dense({units: 16, activation: ‘relu’, inputShape: [inputDimension]}));
model.add(tf.layers.dense({units: outputDimension, activation: ‘softmax’}));
- Compilation:
- Compile the model with an optimizer and loss function.
javascript
model.compile({
optimizer: ‘adam’,
loss: ‘categoricalCrossentropy’,
metrics: [‘accuracy’]
});
Training the Model
Training involves using the prepared data to teach the model. This can be done using:
- fit() Method: Train the model on your dataset.
javascript
await model.fit(xTrain, yTrain, {
epochs: 50,
validationSplit: 0.2
});
- Callbacks: Use callbacks to monitor performance during training.
Integrating the AI Assistant
After training the model, integrate it into your JavaScript application:
– **API Setup**: Create an API endpoint to interact with your assistant.
– **User Input Handling**: Capture user queries and preprocess them for the model.
– **Response Generation**: Use the model to generate responses based on user input.
javascript
app.post(‘/query’, async (req, res) => {
const input = preprocessInput(req.body.query);
const output = model.predict(input);
const response = generateResponse(output);
res.send(response);
});
Testing and Iteration
Continuous testing and iteration are essential for refining the AI assistant:
- User Feedback: Collect feedback from users to identify areas of improvement.
- Model Retraining: Periodically retrain the model with new data to enhance accuracy.
- Performance Metrics: Monitor metrics such as accuracy and response time to assess the assistant’s effectiveness.
Expert Insights on Training AI Assistants in JavaScript
Dr. Emily Carter (AI Research Scientist, Tech Innovations Lab). “To effectively train an AI assistant using JavaScript, one must leverage frameworks like TensorFlow.js, which allows for the development of machine learning models directly in the browser. This enables real-time interaction and adaptability, essential for creating responsive AI systems.”
Michael Chen (Senior Software Engineer, AI Solutions Corp). “Incorporating natural language processing libraries such as compromise or natural into your JavaScript project is crucial. These libraries facilitate understanding and generating human-like responses, which are vital for enhancing user experience with AI assistants.”
Lisa Patel (Lead Developer, Interactive AI Technologies). “A successful AI assistant requires continuous training and refinement. Utilizing user feedback loops and integrating machine learning algorithms in JavaScript will help in improving the assistant’s performance and accuracy over time.”
Frequently Asked Questions (FAQs)
How can I start training an AI assistant in JavaScript?
Begin by selecting a suitable framework or library, such as TensorFlow.js or Brain.js. Familiarize yourself with the basics of machine learning concepts and JavaScript programming. Set up a development environment and gather training data relevant to the tasks your AI assistant will perform.
What types of machine learning models can I use for an AI assistant in JavaScript?
You can use various models, including supervised learning models like regression and classification, unsupervised models like clustering, and reinforcement learning models. The choice depends on the specific tasks your AI assistant is designed to handle, such as natural language processing or decision-making.
How do I prepare training data for my AI assistant?
Collect and preprocess data that is relevant to the tasks your assistant will perform. This may include text data for natural language understanding or structured data for decision-making. Ensure the data is clean, labeled, and representative of the scenarios your assistant will encounter.
What tools can assist in training an AI assistant using JavaScript?
Tools such as TensorFlow.js, Brain.js, and Synaptic can facilitate the training process. Additionally, libraries like Natural for natural language processing and Node.js for server-side scripting can enhance functionality and performance.
How can I evaluate the performance of my AI assistant?
Utilize metrics such as accuracy, precision, recall, and F1 score to assess the performance of your AI assistant. Implement cross-validation techniques and test the assistant with unseen data to ensure it generalizes well to new inputs.
Are there any best practices for training an AI assistant in JavaScript?
Follow best practices such as starting with a clear problem definition, ensuring high-quality training data, using appropriate model architectures, and iterating on model performance through continuous testing and refinement. Additionally, maintain a modular code structure for easier updates and maintenance.
Training an AI assistant in JavaScript involves a combination of understanding the underlying machine learning principles, selecting appropriate libraries, and implementing effective training methodologies. JavaScript offers several libraries such as TensorFlow.js and Brain.js that facilitate the development of AI models directly in the browser or on Node.js. These libraries provide tools for building neural networks, managing data, and executing training algorithms, making it easier for developers to create intelligent applications.
Another crucial aspect of training an AI assistant is data preparation. Quality data is essential for training any machine learning model. This includes gathering relevant datasets, preprocessing the data to ensure it is clean and formatted correctly, and splitting it into training and testing sets. Proper data management helps in improving the accuracy and reliability of the AI assistant, enabling it to learn effectively from the provided inputs.
Furthermore, implementing a feedback loop is vital for continuous improvement of the AI assistant. By collecting user interactions and responses, developers can refine the model over time. This iterative process allows for the adjustment of algorithms and the incorporation of new data, ensuring that the AI assistant remains relevant and effective in responding to user needs.
In summary, training an AI assistant in JavaScript requires a solid understanding of machine learning concepts, effective use
Author Profile
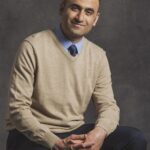
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?