How Can You Effectively Use an API in Python?
In today’s digital landscape, APIs (Application Programming Interfaces) have become the backbone of software development, enabling seamless communication between different applications and services. Whether you’re looking to integrate social media functionalities, access vast databases, or automate tasks, understanding how to use an API in Python can open up a world of possibilities. Python, with its simplicity and versatility, is an ideal language for interacting with APIs, allowing developers to harness the power of external services with minimal effort.
As we delve into the intricacies of API usage in Python, you’ll discover the fundamental concepts that underpin API interactions. From understanding the different types of APIs to grasping the essential HTTP methods, you’ll gain insights into how data is exchanged between your Python applications and external services. Additionally, we’ll explore the libraries and tools that can simplify the process, making it easier for you to implement API calls and handle responses effectively.
By the end of this article, you’ll be equipped with the knowledge to confidently integrate various APIs into your Python projects. Whether you’re a beginner eager to learn or an experienced developer looking to refresh your skills, mastering API usage in Python will empower you to create more dynamic and feature-rich applications. Get ready to unlock the potential of APIs and elevate your programming capabilities!
Understanding API Requests
To interact with an API in Python, it is essential to understand how requests are structured. Generally, an API request consists of the following components:
- Endpoint: The URL where the API is accessed.
- Method: The type of request being made (e.g., GET, POST, PUT, DELETE).
- Headers: Additional information sent with the request, often used for authentication.
- Parameters: Data sent to the API, either in the URL (query parameters) or the body of the request.
Making API Calls with `requests` Library
Python’s `requests` library is a powerful tool for making HTTP requests. It simplifies the process of sending requests and handling responses. To begin using the `requests` library, ensure it is installed in your environment:
“`bash
pip install requests
“`
Here is an example of how to make a simple GET request:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json() Parse JSON response
print(data)
“`
For POST requests, you can send data as follows:
“`python
import requests
url = ‘https://api.example.com/data’
payload = {‘key1’: ‘value1’, ‘key2’: ‘value2’}
response = requests.post(url, json=payload)
data = response.json()
print(data)
“`
Handling API Responses
Responses from APIs typically include status codes and data. The status code indicates the result of the request:
Status Code | Description |
---|---|
200 | OK |
201 | Created |
400 | Bad Request |
401 | Unauthorized |
404 | Not Found |
500 | Internal Server Error |
You can access the status code and content of the response as follows:
“`python
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f”Error: {response.status_code} – {response.text}”)
“`
Authentication with APIs
Many APIs require authentication to ensure that only authorized users can access their resources. Common methods include:
- API Key: A unique key provided when registering for the API.
- Bearer Token: A token used in the Authorization header for OAuth 2.0.
- Basic Auth: A simple username and password encoded in Base64.
Example of using an API key in the headers:
“`python
headers = {
‘Authorization’: ‘Bearer YOUR_API_KEY’
}
response = requests.get(‘https://api.example.com/protected’, headers=headers)
“`
Working with JSON Data
APIs often communicate using JSON, a lightweight data interchange format. In Python, you can easily work with JSON data using the built-in `json` module or directly with the `requests` library:
“`python
import json
Convert Python object to JSON string
json_data = json.dumps({‘key’: ‘value’})
Parse JSON string to Python object
parsed_data = json.loads(json_data)
“`
utilizing an API in Python involves understanding requests, handling responses, managing authentication, and working with data formats such as JSON. By leveraging the `requests` library and following best practices, you can effectively integrate APIs into your applications.
Understanding APIs
An API (Application Programming Interface) allows different software applications to communicate with each other. In Python, APIs are typically used to retrieve data from a web service or send data to it. APIs generally utilize HTTP requests and return data in formats such as JSON or XML.
Setting Up Your Environment
Before using an API in Python, ensure you have the necessary packages installed. The most commonly used library for making HTTP requests in Python is `requests`. Install it using pip if you haven’t already:
“`bash
pip install requests
“`
Making a Basic API Request
To interact with an API, you’ll typically use the `requests` library to send a request. The following example demonstrates how to make a GET request to a sample API:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json() assuming the response is in JSON format
print(data)
“`
In this code:
- `requests.get()` sends a GET request to the specified URL.
- `response.json()` parses the response content into a Python dictionary.
Handling Parameters
Many APIs require parameters to customize the data retrieved. You can pass these parameters as a dictionary to the `params` argument in your request:
“`python
params = {‘key’: ‘value’, ‘another_key’: ‘another_value’}
response = requests.get(‘https://api.example.com/data’, params=params)
“`
Authentication Methods
APIs often require authentication. Common methods include:
- API Keys: Included in request headers or as URL parameters.
- OAuth: A more complex method that involves obtaining access tokens.
Example of using an API key in the headers:
“`python
headers = {‘Authorization’: ‘Bearer YOUR_API_KEY’}
response = requests.get(‘https://api.example.com/data’, headers=headers)
“`
Error Handling
When working with APIs, it is important to handle errors gracefully. Check the status code of the response to determine if the request was successful:
“`python
if response.status_code == 200:
data = response.json()
else:
print(f”Error: {response.status_code} – {response.text}”)
“`
Here’s a brief overview of common HTTP status codes:
Status Code | Meaning |
---|---|
200 | OK |
400 | Bad Request |
401 | Unauthorized |
403 | Forbidden |
404 | Not Found |
500 | Internal Server Error |
Working with JSON Data
APIs often return data in JSON format. Python’s `json` library can help you manipulate this data. For example, you can extract specific fields as follows:
“`python
value = data[‘key’] Accessing a specific field
print(value)
“`
Best Practices
- Rate Limiting: Respect the API’s rate limits to avoid being blocked.
- Documentation: Always refer to the API documentation for detailed information about endpoints, parameters, and response formats.
- Version Control: Use the versioning specified in the API to ensure compatibility with your requests.
By following these guidelines, you can effectively utilize APIs in Python, enabling seamless integration and data exchange between applications.
Expert Insights on Using APIs in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Using APIs in Python is a straightforward process, primarily facilitated by libraries such as Requests. Understanding the structure of the API, including endpoints and required parameters, is crucial for effective integration.”
Michael Patel (Lead Data Scientist, Data Solutions Group). “When working with APIs in Python, it is essential to handle authentication methods, such as OAuth or API keys, properly. This ensures secure access to the data and services provided by the API.”
Sarah Thompson (API Integration Specialist, CloudTech Services). “Error handling is a vital aspect of using APIs in Python. Implementing robust error-checking mechanisms allows developers to manage unexpected responses and maintain application stability.”
Frequently Asked Questions (FAQs)
What is an API?
An API, or Application Programming Interface, is a set of rules and protocols that allows different software applications to communicate with each other. It defines the methods and data formats that applications can use to request and exchange information.
How do I install the necessary libraries to use an API in Python?
To use an API in Python, you typically need the `requests` library. You can install it using pip by running the command `pip install requests` in your terminal or command prompt.
How do I make a GET request to an API using Python?
To make a GET request, use the `requests.get()` method. For example:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.json()
“`
How can I handle API authentication in Python?
API authentication can be handled using headers. For example, if the API requires a token, include it in the headers:
“`python
headers = {‘Authorization’: ‘Bearer YOUR_TOKEN’}
response = requests.get(‘https://api.example.com/data’, headers=headers)
“`
What should I do if I receive an error response from the API?
If you receive an error response, check the status code and the response content. Use `response.status_code` to identify the error type and `response.text` to read the error message. Implement error handling using try-except blocks to manage exceptions gracefully.
How can I parse JSON data returned by an API in Python?
To parse JSON data, use the `json()` method on the response object. For example:
“`python
response = requests.get(‘https://api.example.com/data’)
data = response.json()
“`
This converts the JSON response into a Python dictionary for easy manipulation.
Using an API in Python involves several key steps that facilitate communication between your application and the service you wish to interact with. Initially, you need to identify the API you want to use and understand its documentation, which typically includes information on endpoints, request methods, authentication, and response formats. Familiarity with these elements is crucial for successful implementation.
Once you have a grasp of the API’s structure, you can utilize libraries such as `requests` to send HTTP requests. This library simplifies the process of making GET, POST, PUT, and DELETE requests, allowing you to easily interact with the API. Additionally, handling responses is essential; you should parse the returned data, often in JSON format, to extract the information you need for your application.
Moreover, error handling is a vital aspect of working with APIs. You should implement checks for response status codes and handle exceptions gracefully to ensure your application can respond appropriately to various scenarios, such as rate limiting or service downtime. By following these practices, you can effectively integrate APIs into your Python applications, enhancing their functionality and user experience.
In summary, mastering the use of APIs in Python requires understanding the API documentation, utilizing the right libraries for HTTP requests, and implementing robust error
Author Profile
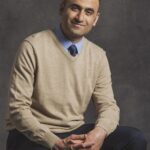
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?