How Can You Effectively Use the Mod Function in Python?
### Introduction
In the world of programming, understanding how to manipulate numbers is a fundamental skill, and one of the most useful operations you can perform is the modulus function, commonly referred to as the “mod” function. Whether you’re building a simple calculator, developing a game, or working on a complex algorithm, the ability to determine the remainder of a division operation can unlock a plethora of possibilities. In Python, this functionality is not only straightforward but also incredibly versatile, allowing you to tackle a variety of problems with ease.
The mod function in Python is a powerful tool that can help you manage tasks ranging from determining even or odd numbers to creating looping patterns in your code. By using the modulus operator, you can find out how much is left over after dividing one number by another, which can be particularly useful in scenarios like scheduling, gaming mechanics, or even cryptography. This article will guide you through the essentials of using the mod function, exploring its syntax and practical applications in real-world programming.
As we delve deeper into the intricacies of the mod function, you’ll discover how it can simplify your coding tasks and enhance your problem-solving skills. From basic examples to more advanced use cases, we’ll cover everything you need to know to effectively implement this function in your Python projects. Get
Using the Mod Function in Python
The modulus operator in Python is represented by the percent sign `%`. This operator returns the remainder of a division operation. It is a fundamental mathematical function commonly used in programming for various applications, such as determining even or odd numbers, cycling through lists, and more.
To use the modulus operator in Python, you can follow this syntax:
python
result = a % b
Where `a` is the dividend and `b` is the divisor. The `result` will contain the remainder of the division of `a` by `b`.
Examples of Mod Function
Here are several examples demonstrating how to use the modulus operator effectively:
python
# Example 1: Basic Modulus Operation
print(10 % 3) # Output: 1
# Example 2: Even or Odd Check
number = 20
if number % 2 == 0:
print(f”{number} is even.”)
else:
print(f”{number} is odd.”)
# Example 3: Cycling through a List
colors = [‘red’, ‘green’, ‘blue’]
for i in range(10):
print(colors[i % len(colors)]) # Cycles through the list
Common Use Cases for Modulus
The modulus operator serves various purposes, including:
- Determining Even or Odd Numbers: By checking if a number `% 2` equals 0, you can determine if it is even.
- Creating Cycles: In cases like iterating over a list, using modulus helps in wrapping around when the index exceeds the list length.
- Divisibility Checks: It can be used to check if a number is divisible by another (e.g., `x % y == 0`).
Modulus with Negative Numbers
Python’s handling of the modulus operator with negative numbers can be slightly different than in some other programming languages. Here’s how it works:
python
print(-10 % 3) # Output: 2
print(10 % -3) # Output: -2
print(-10 % -3) # Output: -1
This behavior can be summarized as follows:
Expression | Result |
---|---|
-10 % 3 | 2 |
10 % -3 | -2 |
-10 % -3 | -1 |
Understanding this behavior is crucial when performing calculations involving negative numbers.
Mod Function Usage
Using the modulus operator in Python is straightforward and versatile. Its ability to return remainders makes it a powerful tool for various programming tasks, from basic arithmetic to more complex algorithms.
Understanding the Mod Function in Python
The modulo operator in Python, represented by the percentage sign (`%`), is used to compute the remainder of a division operation. This is particularly useful in various programming scenarios, including checking for even or odd numbers, cycling through a list, or implementing algorithms that require periodicity.
Basic Syntax
The syntax for the modulo operation is straightforward:
python
result = a % b
Where:
- `a` is the dividend.
- `b` is the divisor.
- `result` will hold the remainder of the division of `a` by `b`.
Examples of Mod Function Usage
Here are some practical examples illustrating how the mod function can be used in different contexts:
- Finding the Remainder:
python
print(10 % 3) # Output: 1
print(15 % 4) # Output: 3
- Checking for Even or Odd Numbers:
python
number = 7
if number % 2 == 0:
print(“Even”)
else:
print(“Odd”) # Output: Odd
- Cycling Through a List:
python
items = [‘a’, ‘b’, ‘c’, ‘d’]
index = 5
print(items[index % len(items)]) # Output: ‘b’
Common Use Cases
The modulo function is commonly applied in various programming scenarios:
- Determining Leap Years:
- A year is a leap year if it is divisible by 4 but not by 100, unless it is also divisible by 400.
python
year = 2024
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
print(“Leap Year”)
- Implementing Circular Buffers:
- When managing data structures that require wrapping around, such as circular queues.
- Game Development:
- For managing player turns or determining outcomes based on score divisions.
Performance Considerations
While the modulo operation is generally efficient, its performance can vary based on the size of the numbers involved. Here are key points to consider:
- Time Complexity: The modulo operation is O(1), meaning it executes in constant time.
- Integer Size: Operations on very large integers may affect performance. Python handles large integers natively, but performance can degrade with excessively large values.
Handling Negative Numbers
When using the modulo operation with negative numbers, it is crucial to be aware of how Python handles the sign:
- The result of the modulo operation takes the sign of the divisor. For example:
python
print(-10 % 3) # Output: 2
print(10 % -3) # Output: -2
print(-10 % -3) # Output: -1
This behavior can sometimes lead to unexpected results, so it is important to handle cases involving negative numbers carefully.
The modulo function is a versatile tool in Python, providing a simple yet powerful means of performing operations that require the determination of remainders. Understanding its application and behavior, especially with negative numbers, enhances its utility in programming tasks.
Expert Insights on Using the Mod Function in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The mod function in Python, represented by the ‘%’ operator, is essential for determining the remainder of a division operation. It is particularly useful in scenarios such as checking for even or odd numbers, where you can simply use ‘number % 2’ to ascertain the parity.”
Michael Chen (Data Scientist, Analytics Hub). “In data analysis, the mod function can be invaluable for categorizing data points. For instance, when working with time series data, using ‘timestamp % 60’ can help in grouping data into minute intervals, facilitating easier analysis of trends.”
Sarah Patel (Software Engineer, CodeCraft Solutions). “Understanding the mod function is crucial for algorithm design, especially in problems involving cycles or periodicity. For example, in a circular queue implementation, using ‘index % size’ ensures that the index wraps around correctly, preventing out-of-bounds errors.”
Frequently Asked Questions (FAQs)
What is the mod function in Python?
The mod function in Python, represented by the percentage symbol `%`, calculates the remainder of the division of one number by another.
How do you use the mod operator in Python?
To use the mod operator, write an expression in the format `a % b`, where `a` is the dividend and `b` is the divisor. The result will be the remainder of `a` divided by `b`.
Can you provide an example of using the mod function?
Certainly. For example, `7 % 3` would return `1`, as 7 divided by 3 equals 2 with a remainder of 1.
Is there a built-in mod function in Python?
Yes, Python also provides a built-in function called `math.fmod()`, which can be used for floating-point numbers. It behaves similarly to the `%` operator but is specifically designed for floating-point arithmetic.
What are common use cases for the mod function?
Common use cases include determining if a number is even or odd, cycling through a list, and implementing algorithms that require periodic behavior, such as hash functions.
Are there any differences between the `%` operator and `math.fmod()`?
Yes, the `%` operator follows the rules of integer division, while `math.fmod()` returns the remainder with the same sign as the divisor. This can lead to different results for negative numbers.
The modulo function, commonly referred to as the mod function, is an essential mathematical operation in Python that returns the remainder of a division between two numbers. In Python, the mod operation is performed using the percentage symbol (%) and can be applied to both integers and floating-point numbers. This operation is particularly useful in various programming scenarios, such as determining even or odd numbers, cycling through a range of values, and implementing algorithms that require periodic behavior.
To utilize the mod function effectively, one must understand its syntax and behavior. The basic syntax is straightforward: `result = a % b`, where `a` is the dividend and `b` is the divisor. The result will be the remainder after dividing `a` by `b`. Additionally, Python’s handling of negative numbers in the modulo operation may differ from other programming languages, as it ensures that the result always has the same sign as the divisor. This characteristic can be crucial when designing algorithms that rely on consistent behavior across different input values.
In summary, the mod function in Python is a powerful tool that facilitates various programming tasks. By mastering its usage, developers can enhance their code’s efficiency and clarity. Understanding the nuances of how the mod function operates, especially with negative numbers, allows
Author Profile
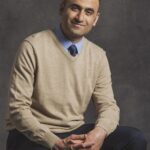
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?