How Can You Use Python to Build Your Own Website?
Creating a website can seem like a daunting task, especially if you’re new to programming or web development. However, with Python, one of the most versatile and user-friendly programming languages, you can turn your ideas into reality with relative ease. Whether you’re looking to build a personal blog, an e-commerce platform, or a portfolio to showcase your work, Python offers a plethora of frameworks and tools that can streamline the development process. In this article, we will explore how to harness the power of Python to create a dynamic and engaging website that meets your needs.
At its core, using Python to create a website involves understanding the fundamentals of web development, including front-end and back-end technologies. Python’s simplicity and readability make it an excellent choice for beginners, while its robust frameworks, such as Django and Flask, provide advanced features for seasoned developers. These frameworks not only facilitate rapid development but also ensure that your website is scalable and maintainable.
In addition to frameworks, Python’s extensive library ecosystem allows you to integrate various functionalities into your website, from database management to user authentication. As we delve deeper into the process, we will uncover the essential steps and best practices for building a website with Python, empowering you to bring your vision to life and engage users effectively. Whether you’re starting from scratch
Choosing a Web Framework
When creating a website using Python, the choice of framework is crucial as it dictates the structure and capabilities of your web application. Popular frameworks include:
- Django: A high-level framework that promotes rapid development and clean, pragmatic design. It follows the “batteries-included” philosophy, offering built-in features like an ORM, authentication, and an admin panel.
- Flask: A micro-framework that is lightweight and modular, allowing developers to choose their tools and libraries. It is ideal for smaller applications or those that require customization.
- FastAPI: A modern web framework for building APIs with Python 3.6+ based on standard Python type hints. It is designed for speed and simplicity, making it an excellent choice for high-performance applications.
Consider your project requirements when selecting a framework, as each offers distinct advantages tailored to various use cases.
Setting Up the Development Environment
Before you begin coding, it is essential to set up your development environment properly. Follow these steps:
- Install Python: Ensure you have Python installed on your machine. You can download it from the official [Python website](https://www.python.org/downloads/).
- Set up a Virtual Environment: This isolates your project dependencies. Use the following commands:
“`bash
python -m venv myprojectenv
source myprojectenv/bin/activate On Windows use `myprojectenv\Scripts\activate`
“`
- Install Your Chosen Framework: Depending on the framework selected, install it via pip. For example:
“`bash
pip install django For Django
pip install flask For Flask
pip install fastapi For FastAPI
“`
This setup helps maintain clean project management and avoid dependency conflicts.
Creating the Website Structure
After setting up your environment, the next step involves establishing the project structure. Below is an example of a basic project layout for a Django application:
Directory/File | Description |
---|---|
myproject/ | Main project directory |
myproject/settings.py | Configuration settings for the project |
myproject/urls.py | URL routing for the application |
myapp/ | A Django application directory |
myapp/models.py | Database models for the application |
myapp/views.py | Logic for handling requests and responses |
myapp/templates/ | HTML templates for rendering views |
This structure can vary depending on the framework, but it generally reflects the organization of files and directories necessary for effective development.
Building and Running the Application
To build and run your web application, you’ll need to write the necessary code within the framework’s conventions. For example, in Django, you might define a view in `views.py` like this:
“`python
from django.shortcuts import render
def home(request):
return render(request, ‘home.html’)
“`
To run the application, execute the following command in your terminal:
“`bash
python manage.py runserver
“`
For Flask, the structure is slightly different, and you might define a simple app like this:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return ‘Hello, World!’
if __name__ == ‘__main__’:
app.run(debug=True)
“`
In both cases, the development server will allow you to view your website locally, providing a foundation for further development and testing.
Choosing a Web Framework
Python offers several frameworks to facilitate web development. The most popular options include:
- Django: A high-level framework that promotes rapid development and clean, pragmatic design. It includes an ORM, built-in admin panel, and authentication.
- Flask: A micro-framework that is lightweight and flexible, ideal for small to medium applications. It allows developers to choose the libraries they want to use.
- FastAPI: Designed for building APIs with high performance, leveraging asynchronous programming capabilities. It is based on standard Python type hints.
Framework | Pros | Cons |
---|---|---|
Django | Batteries-included, ORM, built-in security | Can be heavyweight for small projects |
Flask | Lightweight, flexible, easy to learn | Requires more configuration for larger apps |
FastAPI | High performance, automatic docs generation | Newer, smaller community than Django |
Setting Up Your Environment
To start building a website with Python, you need to set up your development environment. Follow these steps:
- Install Python: Download and install the latest version of Python from the official website.
- Create a Virtual Environment: This keeps dependencies required by different projects in separate places.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
“`
- Install a Framework: Use pip to install your chosen framework.
“`bash
pip install django For Django
pip install flask For Flask
pip install fastapi For FastAPI
“`
Building Your First Web Application
The steps to create a simple web application vary depending on the framework chosen. Below is an example for each framework.
Django:
- Create a new project:
“`bash
django-admin startproject myproject
cd myproject
python manage.py runserver
“`
- Access your application at `http://127.0.0.1:8000/`.
Flask:
- Create a new application file, `app.py`:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, Flask!”
if __name__ == “__main__”:
app.run(debug=True)
“`
- Run the application:
“`bash
python app.py
“`
- Access your application at `http://127.0.0.1:5000/`.
FastAPI:
- Create a new application file, `main.py`:
“`python
from fastapi import FastAPI
app = FastAPI()
@app.get(“/”)
async def read_root():
return {“Hello”: “FastAPI”}
“`
- Run the application using Uvicorn:
“`bash
uvicorn main:app –reload
“`
- Access your application at `http://127.0.0.1:8000/`.
Adding Templates and Static Files
Web applications often require templates and static files (CSS, JavaScript). Each framework has its own approach.
Django:
- Place templates in a directory named `templates` within your app folder.
- Use the `render` function to return HTML.
Flask:
- Create a `templates` folder for HTML files.
- Use `render_template` to serve HTML pages.
FastAPI:
- Use Jinja2 for rendering templates. Set up a templates directory and configure it in your FastAPI app.
Deploying Your Application
Deployment options vary by framework, but common platforms include:
- Heroku: Offers easy deployment for all Python frameworks.
- DigitalOcean: Allows for more control over server settings.
- AWS: Provides comprehensive cloud services for scalability.
Ensure to:
- Configure your application for production (e.g., security settings).
- Set up a database if required.
- Use a WSGI server like Gunicorn or uWSGI for running your application in production.
Each framework will have specific deployment instructions, so refer to the official documentation for detailed steps.
Expert Insights on Using Python for Website Development
Dr. Emily Carter (Senior Software Engineer, Web Innovations Inc.). “Utilizing Python for web development is increasingly popular due to its simplicity and versatility. Frameworks like Django and Flask allow developers to create robust applications quickly, making Python an excellent choice for both beginners and seasoned professionals.”
Michael Chen (Lead Developer, Tech Solutions Group). “When creating a website with Python, it is crucial to understand the MVC architecture that many frameworks implement. This approach not only enhances code organization but also improves maintainability and scalability of web applications.”
Sarah Johnson (Web Development Instructor, Code Academy). “For those new to web development, starting with Python can be a game-changer. The extensive libraries and community support available make it easier to troubleshoot and learn best practices, ensuring a smoother development process.”
Frequently Asked Questions (FAQs)
What are the basic steps to create a website using Python?
To create a website using Python, start by selecting a web framework such as Flask or Django. Install the framework, set up your project structure, define your routes and views, create templates for your HTML, and finally run your application on a local server for testing.
Do I need to know HTML and CSS to create a website with Python?
Yes, knowledge of HTML and CSS is essential for creating a website with Python. HTML structures the content, while CSS styles it. Understanding these languages allows you to create visually appealing and well-structured web pages.
What is Flask and how is it used in web development?
Flask is a lightweight web framework for Python that allows developers to build web applications quickly and efficiently. It provides tools and libraries to handle routing, templates, and databases, making it suitable for both small and large applications.
Can I use Python for front-end development?
Python is primarily used for back-end development. However, frameworks like Brython and PyScript allow you to write Python code that runs in the browser, enabling some front-end functionality. Still, JavaScript remains the dominant language for front-end development.
How do I deploy a Python web application?
To deploy a Python web application, choose a hosting service that supports Python, such as Heroku, AWS, or DigitalOcean. Prepare your application for production, configure the server, and upload your code. Ensure you set up a web server like Gunicorn or uWSGI to serve your application.
What databases can I use with Python web applications?
Python web applications can connect to various databases, including relational databases like PostgreSQL and MySQL, and NoSQL databases like MongoDB. Use libraries such as SQLAlchemy or Django ORM to interact with these databases efficiently.
using Python to create a website involves several essential steps and considerations. Python offers a variety of frameworks and libraries that facilitate web development, with popular choices including Django and Flask. These frameworks provide the necessary tools to handle routing, templating, and database interactions, enabling developers to build robust and scalable web applications efficiently.
Additionally, understanding the fundamentals of HTML, CSS, and JavaScript is crucial for creating a user-friendly interface. While Python handles the backend logic, these front-end technologies are essential for designing an engaging and responsive user experience. Integrating Python with front-end frameworks or libraries can further enhance the functionality and aesthetics of the website.
Moreover, deploying the website requires knowledge of server management and cloud services. Platforms such as Heroku, AWS, or DigitalOcean can be utilized to host Python applications, ensuring they are accessible to users. Continuous integration and version control practices, such as using Git, are also important for maintaining and updating the website over time.
In summary, creating a website with Python is a multifaceted process that combines backend development, front-end design, and deployment strategies. By leveraging the strengths of Python and its associated technologies, developers can create dynamic and efficient web applications that meet the needs
Author Profile
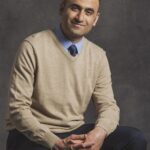
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?