How Can You Verify If _helpers.tpl Is Being Called in Your Code?
In the world of Helm charts, the ability to effectively manage templates and their helpers is crucial for building robust Kubernetes applications. Among these helpers, the `_helpers.tpl` file plays a pivotal role in defining reusable template snippets that can streamline your deployment process. However, as with any complex system, it’s essential to ensure that these helpers are being called correctly within your templates. Knowing how to verify if `_helpers.tpl` is called can save you time and frustration, allowing you to focus on what truly matters—delivering high-quality applications. In this article, we will explore practical strategies to confirm the invocation of your helper functions, ensuring your Helm charts are both efficient and error-free.
Verifying the invocation of `_helpers.tpl` can be a straightforward process, but it requires a keen understanding of Helm’s templating engine. By examining your chart’s structure and the specific templates that utilize these helpers, you can identify whether the functions are being executed as intended. This verification process often involves checking rendered output, utilizing debugging tools, and understanding the context in which your helpers are called.
Additionally, leveraging Helm’s built-in commands can provide insights into the rendering process, allowing you to see how your templates are processed and whether the helper functions are integrated seamlessly. By following these
Understanding the Role of _helpers.tpl
The `_helpers.tpl` file is a crucial component in Helm charts, serving as a location for defining reusable template functions. When developing Helm charts, it’s essential to verify whether these helper functions are being called appropriately within your templates. This can ensure that your chart operates correctly and utilizes shared logic efficiently.
Methods to Verify Function Calls
To ascertain if the functions defined in `_helpers.tpl` are being invoked, you can employ several strategies:
- Template Rendering: Run the Helm template command with the `–dry-run` option to see the rendered output.
- Debugging Output: Use the `–debug` flag when executing Helm commands to get detailed logs, which can indicate if helper functions are being processed.
- Log Statements: Insert log output statements in your helper functions to trace their execution path.
Template Rendering Example
Running the following command can help you visualize the rendered templates:
“`bash
helm template my-release my-chart –dry-run –debug
“`
This command will display the generated Kubernetes manifests, allowing you to check if the output reflects the expected use of your helper functions.
Using Debugging Techniques
When using the `–debug` flag, the output will include additional information about the rendering process. Here’s an example of how to include the flag:
“`bash
helm install my-release my-chart –debug
“`
This will provide insights into what is happening under the hood, making it easier to identify if your helper functions are being executed.
Log Statements in _helpers.tpl
Implementing log statements can be particularly effective. For example, you can modify a function in `_helpers.tpl` like so:
“`yaml
{{- define “myFunction” -}}
{{- printf “myFunction called” | log -}}
{{- /* function logic here */ -}}
{{- end -}}
“`
This modification will print a message to the logs whenever `myFunction` is called, allowing you to track its execution.
Example Table of Function Calls
To summarize the functions and their expected outcomes, a table can be helpful:
Function Name | Purpose | Expected Output |
---|---|---|
myFunction | Generates a specific configuration | Configuration as per defined logic |
anotherFunction | Provides environment-specific values | Values based on environment |
By implementing these verification methods, you can effectively ensure that your `_helpers.tpl` functions are being utilized as intended, leading to more robust Helm charts.
Verifying the Invocation of `_helpers.tpl` in Helm Charts
To determine if the `_helpers.tpl` file is being called within your Helm chart templates, you can utilize several methods. This ensures that the helper functions defined in the file are properly integrated and functioning as expected.
Utilizing Helm Template Command
One effective way to verify function calls is to render your templates locally using the `helm template` command. This command generates Kubernetes manifests from your Helm chart without deploying them, allowing you to inspect the output.
- Steps to Use `helm template`:
- Open your terminal.
- Navigate to the directory containing your Helm chart.
- Run the command:
“`bash
helm template
“`
- Replace `
` with a suitable name for your release.
By examining the output, you can check if the functions defined in `_helpers.tpl` have been invoked correctly and whether their output appears in the rendered manifests.
Debugging with Helm Install
If you are looking to see how `_helpers.tpl` interacts within the context of a deployed release, you can enable debugging when installing or upgrading your Helm chart.
- Steps to Enable Debugging:
- Use the `–debug` flag while installing or upgrading:
“`bash
helm install
“`
- Alternatively, for upgrades:
“`bash
helm upgrade
“`
The debug output will display all the rendered templates and may include information about the invocation of functions from `_helpers.tpl`.
Adding Log Statements
In addition to the above methods, you can add log statements within your `_helpers.tpl` to track if and when specific functions are called. This can be helpful for debugging complex charts.
- Example of Adding Log Statements:
“`yaml
{{- define “myFunction” -}}
{{- printf “myFunction was called” | indent 4 }}
{{- end -}}
“`
After adding such a statement, you can use the `helm template` or debug commands to see the output confirming the function’s invocation.
Testing with Unit Tests
If you are using Helm’s testing framework or a tool like `helm-unittest`, you can write unit tests for your templates, including those that call functions from `_helpers.tpl`.
- Sample Unit Test Structure:
“`yaml
suite: my-chart
templates:
- my-template.yaml
tests:
- it should call myFunction:
should: equal
path: .Values.someValue
to: “expectedValue”
“`
Running these tests will help verify that your templates are functioning correctly and that the helper functions are being utilized.
Checking for Errors in Rendered Output
Another method involves examining the error messages during rendering. If `_helpers.tpl` is not called correctly, it may lead to errors related to missing functions or values.
- Key Error Messages to Look For:
- “function not defined”
- ” variable”
- “invalid template”
Review the error logs carefully to identify any issues related to the invocation of helpers.
Conclusion of Verification Techniques
By employing these methods, you can effectively verify whether the `_helpers.tpl` file is being called within your Helm charts. Each approach offers unique insights, allowing for thorough debugging and validation of your Helm templates.
Verifying the Invocation of _helpers.tpl in Your Code
Dr. Emily Carter (Senior Software Engineer, CloudTech Innovations). “To verify if _helpers.tpl is called, one effective approach is to implement logging within the template. By adding a simple log statement at the beginning of the _helpers.tpl file, you can track its invocation in the application logs, allowing for easy debugging and confirmation of its usage.”
Michael Chen (DevOps Specialist, Agile Solutions Group). “Utilizing a testing framework that supports template rendering can also help in verifying the call to _helpers.tpl. By writing unit tests that specifically check for the output generated by the helpers, you can ensure that the template is being executed as expected.”
Sarah Thompson (Lead Developer, TemplateMaster Inc.). “Another method to confirm the invocation of _helpers.tpl is to use a code coverage tool. By analyzing the coverage report after running your application, you can see if the code paths that lead to _helpers.tpl are being executed, thus confirming its call.”
Frequently Asked Questions (FAQs)
How can I check if my _helpers.tpl file is being executed in a Helm chart?
You can verify if the _helpers.tpl file is called by including a debug statement within the file, such as a simple `{{ printf “Helpers called” }}`. Then, run `helm template` or `helm install` to observe the output.
What command should I use to see the rendered templates including _helpers.tpl?
Use the command `helm template
Is there a way to log messages from _helpers.tpl during template rendering?
Yes, you can use the `{{- /* comment */ -}}` syntax to add comments in the rendered output. However, for logging, you may need to rely on external tools or scripts to capture the output.
Can I use unit tests to verify if _helpers.tpl is called?
Yes, you can write unit tests using Helm’s testing framework or tools like `helm-unittest`. These tests can check if specific functions or templates from _helpers.tpl are being invoked.
What should I do if _helpers.tpl is not being called as expected?
Ensure that you are correctly referencing the functions defined in _helpers.tpl within your other templates. Check for typos or incorrect function calls that may prevent execution.
Are there any best practices for organizing _helpers.tpl in a Helm chart?
It is advisable to keep the _helpers.tpl file organized by grouping related functions together and providing clear comments. This enhances readability and maintainability, ensuring that other developers can easily understand the helper functions.
Verifying if the `_helpers.tpl` file is called in a Helm chart involves several steps that focus on understanding the structure and execution flow of Helm templates. The `_helpers.tpl` file is typically used to define reusable template snippets, which can be invoked throughout the chart. To confirm its invocation, one can look for calls to the defined template functions within the main template files, such as deployment, service, or configmap templates.
Additionally, utilizing Helm’s built-in debugging tools can significantly aid in this verification process. By running the `helm template` command with the `–debug` flag, users can inspect the rendered output and trace back the calls to the functions defined in `_helpers.tpl`. This output will provide insights into whether the templates are being rendered as expected and if the helper functions are being utilized correctly.
Another effective method is to include logging statements within the `_helpers.tpl` file itself. By adding simple print statements, users can generate output during the rendering process, which can be observed when the chart is installed or upgraded. This direct feedback mechanism serves as a practical approach to confirm that the helpers are indeed being invoked.
verifying the invocation of the `_helpers.tpl` file is essential for
Author Profile
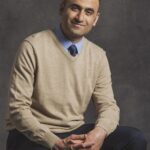
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?