How Can You Write a Matrix in Python?
In the world of programming, particularly in data science and numerical computing, matrices play a crucial role in handling complex data structures. Whether you’re working on machine learning algorithms, performing statistical analysis, or simply manipulating data, understanding how to effectively create and manage matrices in Python is essential. With its rich ecosystem of libraries and tools, Python offers a variety of ways to work with matrices, making it a favorite among developers and data scientists alike. This article will guide you through the various methods of writing and manipulating matrices in Python, ensuring you have the skills to tackle any matrix-related task with confidence.
Overview
When it comes to writing matrices in Python, there are several approaches you can take, each suited to different needs and preferences. From using native Python lists to leveraging powerful libraries like NumPy, the options available allow for flexibility and efficiency in your coding practices. Understanding the strengths and limitations of each method is key to selecting the right one for your specific project.
Moreover, matrices are not just about storage; they also involve operations such as addition, multiplication, and transposition. By mastering these operations, you can perform complex calculations and analyses that are fundamental in fields such as data science, machine learning, and scientific computing. As we delve deeper into this topic, you will
Using Lists to Represent Matrices
In Python, the simplest way to represent a matrix is by using a list of lists. Each inner list represents a row in the matrix. This approach is intuitive and easy to implement, especially for small matrices. Here’s how you can create a 2×3 matrix:
“`python
matrix = [[1, 2, 3],
[4, 5, 6]]
“`
To access elements in the matrix, you can use indexing. For example, to access the element in the first row and second column, you would write:
“`python
element = matrix[0][1] This will return 2
“`
Using NumPy for Matrix Operations
For more complex matrix operations, the NumPy library is highly recommended. It provides efficient storage and operations for large matrices. First, you need to install NumPy if you haven’t already:
“`bash
pip install numpy
“`
Once installed, you can create matrices using NumPy’s `array` function:
“`python
import numpy as np
matrix = np.array([[1, 2, 3],
[4, 5, 6]])
“`
NumPy allows you to perform various operations on matrices, such as addition, multiplication, and transposition. Here’s a quick overview of some common operations:
- Addition: `matrix + matrix`
- Multiplication: `matrix @ matrix` (for matrix multiplication)
- Transpose: `matrix.T`
Creating Matrices with List Comprehensions
You can also create matrices using list comprehensions, which can make your code cleaner and more concise. Here’s an example of creating a 3×3 identity matrix:
“`python
identity_matrix = [[1 if i == j else 0 for j in range(3)] for i in range(3)]
“`
This method is particularly useful for generating larger matrices or those with specific patterns.
Example of Matrix Operations with NumPy
Below is an example demonstrating various operations with NumPy matrices. Let’s create two matrices and perform addition and multiplication:
“`python
import numpy as np
A = np.array([[1, 2],
[3, 4]])
B = np.array([[5, 6],
[7, 8]])
Addition
C = A + B
Matrix Multiplication
D = A @ B
print(“Matrix C (Addition):\n”, C)
print(“Matrix D (Multiplication):\n”, D)
“`
The output will be:
“`
Matrix C (Addition):
[[ 6 8]
[10 12]]
Matrix D (Multiplication):
[[19 22]
[43 50]]
“`
Matrix Representation in Tabular Form
When dealing with matrices, especially for presentation purposes, it can be useful to visualize them in a tabular format. Below is a representation of a 2×3 matrix using HTML:
Column 1 | Column 2 | Column 3 |
---|---|---|
1 | 2 | 3 |
4 | 5 | 6 |
This table format is beneficial for representing matrices in web applications or documentation, enhancing readability and comprehension.
Creating a Matrix Using Lists
In Python, the most straightforward way to create a matrix is by using nested lists. Each inner list represents a row of the matrix.
“`python
Example of a 3×3 matrix
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
“`
To access elements in the matrix, you can use indexing:
“`python
element = matrix[1][2] Accesses the element in the second row, third column (value 6)
“`
Using NumPy for Matrix Operations
For more complex matrix operations, the NumPy library is highly recommended. It provides a powerful array structure and numerous mathematical functions.
To use NumPy, install it via pip if it is not already available:
“`bash
pip install numpy
“`
Once installed, you can create matrices as follows:
“`python
import numpy as np
Creating a 3×3 matrix
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
“`
NumPy allows for easy manipulation of matrices, including addition, multiplication, and transposition:
- Addition: You can add two matrices of the same dimensions.
- Multiplication: Matrix multiplication is done using the `@` operator or the `dot()` method.
- Transposition: Use the `.T` attribute to get the transpose of a matrix.
Creating a Sparse Matrix
In scenarios where the matrix is large but contains many zeros, it may be more efficient to use sparse matrices. The `scipy.sparse` module allows for the creation and manipulation of sparse matrices.
“`python
from scipy.sparse import csr_matrix
Creating a sparse matrix
data = [1, 2, 3]
row_indices = [0, 1, 2]
col_indices = [0, 2, 1]
sparse_matrix = csr_matrix((data, (row_indices, col_indices)), shape=(3, 3))
“`
This representation is efficient in terms of both memory usage and computational performance.
Matrix Operations with Lists and NumPy
Here’s a brief overview of common operations you might perform on matrices with lists and NumPy:
Operation | Using Lists | Using NumPy |
---|---|---|
Addition | Manually iterate and add elements | `matrix1 + matrix2` |
Multiplication | Nested loops for element-wise product | `matrix1 @ matrix2` or `np.dot(matrix1, matrix2)` |
Transposition | List comprehension or loops | `matrix.T` |
Determinant | Not straightforward, manual method | `np.linalg.det(matrix)` |
Visualizing Matrices
For visual representation, libraries like Matplotlib can be used to display matrices as heatmaps. Here’s a simple example:
“`python
import matplotlib.pyplot as plt
plt.imshow(matrix, cmap=’hot’, interpolation=’nearest’)
plt.colorbar()
plt.show()
“`
This will create a heatmap that visually represents the values in the matrix, aiding in understanding its structure and properties.
Expert Insights on Writing Matrices in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When writing matrices in Python, utilizing libraries such as NumPy is essential. NumPy provides efficient and convenient methods for creating and manipulating matrices, making it the go-to choice for data analysis and scientific computing.”
Michael Chen (Software Engineer, Python Programming Group). “For beginners, I recommend starting with simple lists to represent matrices. However, as your projects grow in complexity, transitioning to NumPy arrays will enhance performance and functionality significantly.”
Sarah Johnson (Academic Researcher, Computational Mathematics Institute). “It is crucial to understand the underlying structure of matrices when programming in Python. Mastering the use of multidimensional arrays will not only improve your coding skills but also enable you to implement advanced algorithms effectively.”
Frequently Asked Questions (FAQs)
How can I create a matrix in Python?
You can create a matrix in Python using nested lists or by utilizing libraries such as NumPy. For example, a simple 2×2 matrix can be created using nested lists: `matrix = [[1, 2], [3, 4]]`.
What is the NumPy library and how does it help with matrices?
NumPy is a powerful library for numerical computing in Python. It provides support for large multidimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays efficiently.
How do I install NumPy?
You can install NumPy using pip by running the command `pip install numpy` in your terminal or command prompt.
How can I perform matrix operations using NumPy?
Matrix operations such as addition, subtraction, multiplication, and inversion can be performed using NumPy functions. For example, to multiply two matrices, you can use `numpy.dot(matrix1, matrix2)`.
What are the advantages of using NumPy for matrix operations over nested lists?
NumPy provides optimized performance for large datasets, better memory efficiency, and a wide range of built-in functions for mathematical operations, making it preferable for complex calculations compared to nested lists.
Can I convert a list of lists into a NumPy array?
Yes, you can convert a list of lists into a NumPy array using the `numpy.array()` function. For example, `numpy_array = np.array([[1, 2], [3, 4]])` converts a nested list into a NumPy array.
Writing matrices in Python can be accomplished using various methods, depending on the specific requirements of your project. The most common approaches include utilizing built-in lists, employing the NumPy library for more advanced operations, and leveraging libraries like pandas for data manipulation. Each method has its advantages, and the choice largely depends on the complexity of the matrix operations you intend to perform and the efficiency you require.
Using Python’s built-in lists is straightforward for basic matrix representation. However, for numerical computations, NumPy is the preferred option due to its powerful array handling capabilities and performance optimization. NumPy allows for easy creation, manipulation, and mathematical operations on matrices, making it ideal for scientific computing. Additionally, pandas can be used for matrix-like data structures, particularly when working with tabular data, providing robust data analysis tools.
understanding how to write and manipulate matrices in Python is essential for various applications in data science, machine learning, and numerical analysis. By selecting the right approach—whether it be lists, NumPy arrays, or pandas DataFrames—you can efficiently handle matrix operations and enhance your programming capabilities in Python. Familiarity with these tools will significantly benefit your projects and improve your overall coding proficiency.
Author Profile
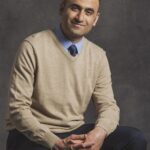
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?