How Can You Write a Python Script? A Step-by-Step Guide for Beginners
### Introduction
In today’s digital landscape, the ability to write a Python script is an invaluable skill that opens doors to a myriad of opportunities. Whether you’re looking to automate mundane tasks, analyze data, or even develop complex applications, Python’s simplicity and versatility make it a go-to language for beginners and seasoned programmers alike. But where do you start? How do you transform your ideas into functional code? This article will guide you through the essential steps to crafting your own Python scripts, empowering you to harness the full potential of this dynamic programming language.
Writing a Python script is not just about knowing the syntax; it’s about understanding the logic behind programming concepts and how they can be applied to solve real-world problems. From setting up your development environment to structuring your code effectively, there are fundamental principles that every aspiring coder should grasp. As you embark on your scripting journey, you’ll discover the importance of planning your project, choosing the right libraries, and debugging your code to ensure smooth execution.
As you delve deeper into the world of Python scripting, you’ll learn how to create scripts that are not only functional but also efficient and maintainable. This knowledge will empower you to tackle increasingly complex challenges, whether in data analysis, web development, or automation. So, let’s take the first step together and explore
Setting Up Your Python Environment
To begin writing a Python script, it is essential to have a proper development environment configured. This involves installing Python and choosing an Integrated Development Environment (IDE) or text editor that suits your needs.
- Download Python: Visit the official Python website at [python.org](https://www.python.org/) to download the latest version. Ensure that you select the installer that matches your operating system.
- Install Python: Follow the installation instructions provided. During installation, make sure to check the box that says “Add Python to PATH” to avoid issues with running Python from the command line.
- Choose an IDE/Text Editor: Some popular choices include:
- PyCharm
- Visual Studio Code
- Jupyter Notebook
- Sublime Text
Each of these editors has unique features, so select one that aligns with your workflow.
Writing Your First Script
Once your environment is set up, you can start writing your first Python script. Open your chosen IDE or text editor, and follow these steps:
- Create a New File: Open a new file and save it with a `.py` extension. For example, `hello_world.py`.
- Write Code: Enter the following code into your file:
python
print(“Hello, World!”)
- Run the Script: To execute your script, use the command line or terminal:
- Navigate to the directory where your script is saved.
- Run the command:
python hello_world.py
This simple script will display “Hello, World!” in the console, confirming that your setup is functioning correctly.
Understanding Python Syntax
Python has a clear and readable syntax. Familiarizing yourself with the basic elements of Python syntax is crucial for effective scripting. Here are some fundamental concepts:
– **Variables**: Used to store data values.
– **Data Types**: Common types include:
- Integers (`int`)
- Floating-point numbers (`float`)
- Strings (`str`)
- Booleans (`bool`)
Example of variable declaration:
python
name = “Alice”
age = 30
is_student =
– **Control Structures**: These include conditions and loops that control the flow of the program.
– **If Statements**:
python
if age >= 18:
print(“Adult”)
else:
print(“Minor”)
- For Loops:
python
for i in range(5):
print(i)
Common Python Libraries
Python’s extensive library ecosystem allows you to perform a variety of tasks without having to write everything from scratch. Here are some commonly used libraries:
Library | Purpose |
---|---|
NumPy | Numerical operations |
Pandas | Data manipulation and analysis |
Matplotlib | Data visualization |
Requests | HTTP requests |
Flask | Web development |
To install a library, use pip, Python’s package installer. For example, to install NumPy, run:
bash
pip install numpy
By leveraging these libraries, you can enhance your scripts significantly, making them more powerful and efficient.
Debugging Your Script
Debugging is an essential part of the development process. Here are some techniques to identify and fix errors in your scripts:
- Print Statements: Use print statements to output variable values and program flow.
- IDE Debugging Tools: Most IDEs come with debugging tools that allow you to step through your code.
- Error Messages: Read error messages carefully; they often provide clues about what went wrong.
By applying these techniques, you can streamline the debugging process and improve your coding efficiency.
Setting Up Your Environment
To write a Python script, you first need to set up your development environment. This involves installing Python and choosing a code editor.
- Install Python:
Download the latest version from the official Python website (python.org). Follow the installation instructions specific to your operating system. Ensure you check the option to add Python to your system PATH during installation.
- Choose a Code Editor:
Select a text editor or integrated development environment (IDE) suited to your needs. Popular options include:
- Visual Studio Code: A versatile and widely used editor with support for extensions.
- PyCharm: A powerful IDE specifically designed for Python development.
- Jupyter Notebook: Ideal for data analysis and interactive coding.
Creating Your First Python Script
Once your environment is set up, you can create your first Python script.
- Open your code editor.
- Create a new file: Save it with a `.py` extension (e.g., `my_script.py`).
- Write your code: Start with a simple print statement.
python
print(“Hello, World!”)
- Save the file: Use the save option in your editor.
Running the Python Script
To execute your Python script, follow these steps:
- Using Command Line:
- Open your command prompt or terminal.
- Navigate to the directory where your script is saved using the `cd` command.
- Run the script by typing:
bash
python my_script.py
- Using an IDE:
- Most IDEs have a built-in run option. Look for a run button or use a shortcut (often F5 or Shift + F10).
Basic Syntax and Structure
Understanding Python’s basic syntax is essential for writing effective scripts. Here are some fundamental concepts:
Element | Description |
---|---|
Variables | Used to store data (e.g., `name = “Alice”`). |
Data Types | Common types include integers, floats, strings, lists, etc. |
Control Flow | Use `if`, `for`, and `while` statements to control execution. |
Functions | Defined using the `def` keyword (e.g., `def my_function():`). |
Common Python Script Components
When writing Python scripts, certain components are commonly used. Familiarize yourself with these:
– **Comments**: Begin with `#` to annotate code.
python
# This is a comment
– **Functions**: Encapsulate reusable code.
python
def greet(name):
print(f”Hello, {name}!”)
– **Loops**: Iterate over sequences.
python
for i in range(5):
print(i)
– **Conditionals**: Control the flow based on conditions.
python
if x > 10:
print(“x is greater than 10”)
Debugging and Error Handling
Debugging is an integral part of writing scripts. Use the following techniques:
- Print Statements: Use `print()` to track variable values.
- Exceptions: Handle errors gracefully with `try` and `except`.
python
try:
# Code that may throw an error
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero!”)
- Debugging Tools: Utilize built-in debugging tools in your IDE, such as breakpoints and step execution.
Best Practices for Writing Python Scripts
Adopting best practices enhances code quality and maintainability:
- Use Meaningful Names: Choose descriptive variable and function names.
- Keep Code Modular: Break scripts into functions or modules.
- Follow PEP 8: Adhere to Python’s style guide for consistency.
- Document Your Code: Use docstrings to explain functions and modules.
By following these guidelines, you can develop clean, efficient, and maintainable Python scripts.
Expert Insights on Writing Python Scripts
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When writing a Python script, it’s essential to start with a clear understanding of the problem you are trying to solve. A well-defined problem statement will guide your coding process and help you structure your script effectively.”
Mark Thompson (Lead Python Developer, CodeCraft Solutions). “Utilizing Python’s extensive libraries can significantly enhance your script’s functionality. Familiarize yourself with libraries such as NumPy for numerical tasks and Pandas for data manipulation to streamline your coding efforts.”
Jessica Patel (Educational Technology Consultant, LearnPython.org). “For beginners, I recommend breaking down your script into smaller, manageable functions. This modular approach not only makes your code easier to read and debug but also promotes reusability in future projects.”
Frequently Asked Questions (FAQs)
What are the basic steps to write a Python script?
To write a Python script, start by choosing a text editor or an Integrated Development Environment (IDE) such as PyCharm or VSCode. Create a new file with a `.py` extension, write your Python code, and save the file. Finally, run the script using a command line or terminal by typing `python filename.py`.
What is the purpose of the shebang line in a Python script?
The shebang line, typically `#!/usr/bin/env python3`, is used at the beginning of a script to indicate the interpreter that should be used to execute the file. It allows the script to be run as an executable without explicitly invoking the Python interpreter.
How do I handle errors in a Python script?
To handle errors in a Python script, utilize try-except blocks. Place the code that may raise an exception within the try block, and define how to handle the exception in the except block. This approach prevents the script from crashing and allows for graceful error management.
Can I use external libraries in my Python script?
Yes, you can use external libraries in your Python script. Install the desired library using pip, Python’s package manager, by running `pip install library_name` in the terminal. Then, import the library in your script using the `import` statement.
How can I make my Python script executable on Unix-like systems?
To make your Python script executable on Unix-like systems, ensure the script has the correct shebang line at the top. Then, change the file permissions using the command `chmod +x filename.py`. You can then run the script directly from the terminal.
What are some best practices for writing Python scripts?
Best practices for writing Python scripts include using meaningful variable and function names, adhering to PEP 8 style guidelines, writing modular code by defining functions, including comments and documentation, and using version control systems like Git to track changes.
Writing a Python script involves several essential steps that contribute to the overall effectiveness and efficiency of the code. First, it is crucial to define the purpose of the script clearly. Understanding what problem the script aims to solve or what task it should perform lays the groundwork for the subsequent coding process. This initial step ensures that the script remains focused and relevant to its intended use.
Next, selecting the appropriate development environment is vital for writing and testing the script. Python offers various integrated development environments (IDEs) and text editors that can enhance productivity and facilitate debugging. Popular options include PyCharm, Visual Studio Code, and Jupyter Notebook, each providing unique features that cater to different programming needs and preferences.
Additionally, structuring the code logically and utilizing comments effectively are key practices in Python scripting. A well-organized script not only improves readability but also makes maintenance easier in the long run. Comments serve as documentation, helping others (or the original author at a later time) understand the code’s functionality and reasoning behind specific implementations.
Finally, testing the script thoroughly is essential to ensure its reliability and correctness. Implementing unit tests and debugging techniques can help identify and resolve issues before deployment. By following these steps—defining the
Author Profile
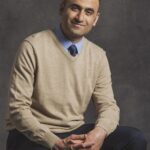
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?