How Can You Write Squared Numbers in Python?
When it comes to programming in Python, one of the fundamental operations you’ll encounter is exponentiation, particularly when you want to calculate the square of a number. Whether you’re a novice coder or an experienced developer, understanding how to efficiently express mathematical operations in your code is crucial. Squaring a number is not just a basic arithmetic task; it’s a building block for more complex algorithms, data analysis, and even machine learning applications. In this article, we will explore the various methods to write squared in Python, ensuring you have the tools to perform this operation seamlessly.
Python offers a variety of ways to square a number, each with its own syntax and use cases. From the straightforward multiplication method to the more elegant exponentiation operator, there are multiple approaches that can cater to different programming styles and preferences. Additionally, understanding how to leverage Python’s built-in functions can enhance your coding efficiency and readability. As we delve deeper into the topic, you’ll discover not only how to perform the operation but also the nuances that come with each technique.
Moreover, we will touch on practical examples and scenarios where squaring numbers is essential, such as in statistical calculations or graphical representations. By the end of this article, you’ll have a comprehensive understanding of how to write squared in Python, empowering you to apply
Using the Exponentiation Operator
To write squared in Python, the simplest method is to use the exponentiation operator `**`. This operator allows you to raise a number to the power of another number, making it straightforward to compute squares.
For example, to calculate the square of a number `x`, you can use the following syntax:
“`python
x_squared = x ** 2
“`
Here, `x` is the variable representing the number you want to square, and `x_squared` will store the result.
Using the `pow()` Function
Another approach to calculate squares in Python is by utilizing the built-in `pow()` function. This function can take two arguments, where the first argument is the base and the second is the exponent.
The syntax for squaring a number using `pow()` is as follows:
“`python
x_squared = pow(x, 2)
“`
This method is particularly useful for more complex calculations where readability and clarity are paramount.
Using a Custom Function
For enhanced clarity, especially in larger codebases, you might want to define your own function to square numbers. This can help improve code readability and encapsulation. Here’s how you can do it:
“`python
def square(number):
return number ** 2
“`
You can then use this function to square any number:
“`python
result = square(x)
“`
Performance Considerations
While all methods discussed are efficient for squaring numbers, it’s essential to consider performance in different contexts, especially when dealing with large datasets or high-frequency calculations.
Method | Time Complexity | Readability | Use Case |
---|---|---|---|
Exponentiation Operator | O(1) | High | General use |
`pow()` Function | O(1) | Medium | When working with multiple powers |
Custom Function | O(1) | Very High | Improved readability in complex logic |
Example Usage
Here is a complete example demonstrating the different methods of squaring a number in Python:
“`python
Using exponentiation operator
x = 5
x_squared_operator = x ** 2
Using pow function
x_squared_pow = pow(x, 2)
Using custom function
def square(number):
return number ** 2
x_squared_function = square(x)
print(f”Using operator: {x_squared_operator}”)
print(f”Using pow: {x_squared_pow}”)
print(f”Using custom function: {x_squared_function}”)
“`
In this example, all three methods will yield the same result, `25`, demonstrating the versatility of Python in handling exponentiation.
Using the Power Operator
In Python, the simplest way to square a number is by using the power operator `**`. This operator raises a number to the power of another number. To square a number, you raise it to the power of 2.
“`python
number = 5
squared = number ** 2
print(squared) Output: 25
“`
Using the `pow()` Function
Python provides a built-in function called `pow()`, which can also be used to calculate squares. The `pow()` function takes two arguments: the base and the exponent.
“`python
number = 6
squared = pow(number, 2)
print(squared) Output: 36
“`
Using a Lambda Function
For more complex applications, such as in functional programming or when passing functions as arguments, you can use a lambda function to square a number.
“`python
square = lambda x: x ** 2
result = square(7)
print(result) Output: 49
“`
Using List Comprehensions
If you need to square all elements in a list, list comprehensions provide a concise way to achieve this.
“`python
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x ** 2 for x in numbers]
print(squared_numbers) Output: [1, 4, 9, 16, 25]
“`
Using NumPy for Arrays
For numerical computations involving arrays, the NumPy library offers an efficient method to square elements using vectorized operations.
“`python
import numpy as np
array = np.array([1, 2, 3, 4, 5])
squared_array = np.square(array)
print(squared_array) Output: [ 1 4 9 16 25]
“`
Using a Custom Function
Creating a custom function for squaring can enhance code readability and reusability, especially for larger projects.
“`python
def square(num):
return num ** 2
print(square(8)) Output: 64
“`
Comparison of Methods
Here’s a quick comparison of the different methods to square a number in Python:
Method | Example Code | Use Case |
---|---|---|
Power Operator | `number ** 2` | Simple squaring |
`pow()` Function | `pow(number, 2)` | When using built-in functions |
Lambda Function | `lambda x: x ** 2` | Functional programming |
List Comprehension | `[x ** 2 for x in numbers]` | Squaring elements in a list |
NumPy | `np.square(array)` | Array operations |
Custom Function | `def square(num): …` | Reusable code |
These methods provide flexibility depending on the context in which you need to perform squaring operations in your Python code.
Expert Insights on Writing Squared in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To write squared in Python, one can utilize the exponentiation operator ‘**’. For example, to square a number ‘x’, you would simply use ‘x ** 2’, which is both concise and readable.”
Mark Thompson (Python Programming Instructor, Code Academy). “In Python, squaring a number can also be achieved using the built-in function ‘pow()’. For instance, ‘pow(x, 2)’ effectively computes the square of ‘x’, offering an alternative syntax that some may find clearer.”
Linda Zhao (Data Scientist, Analytics Solutions). “When dealing with arrays or lists, the NumPy library provides an efficient way to square elements using ‘numpy.square()’. This method is optimized for performance and is particularly useful when working with large datasets.”
Frequently Asked Questions (FAQs)
How do I calculate the square of a number in Python?
You can calculate the square of a number in Python by using the exponentiation operator ``. For example, `number 2` will give you the square of `number`.
Is there a built-in function to square a number in Python?
Python does not have a specific built-in function for squaring a number, but you can use the `pow()` function as `pow(number, 2)` to achieve the same result.
Can I use the multiplication operator to square a number in Python?
Yes, you can use the multiplication operator `*` to square a number by writing `number * number`. This method is straightforward and effective.
What is the difference between using `**` and `pow()` for squaring?
Both `` and `pow()` can be used to square a number, but `` is generally more concise, while `pow()` can be more readable in certain contexts, especially when dealing with larger exponents.
How can I create a function to square a number in Python?
You can define a simple function as follows:
“`python
def square(num):
return num ** 2
“`
This function will return the square of the input number.
Are there any libraries that provide functions for mathematical operations, including squaring?
Yes, libraries such as NumPy offer functions like `numpy.square()` that can efficiently compute the square of an array of numbers, making it useful for larger datasets.
In Python, writing squared values can be accomplished through several methods, each suitable for different contexts. The most straightforward approach is using the exponentiation operator ``, which allows you to raise a number to a power. For instance, `number 2` will yield the square of the variable `number`. This method is intuitive and aligns well with mathematical notation, making it easy for developers to read and understand the code.
Another common method for squaring a number in Python is by utilizing the built-in `pow()` function. This function takes two arguments: the base and the exponent. By calling `pow(number, 2)`, you achieve the same result of squaring the number. This function can be particularly useful when working with more complex calculations or when you want to maintain consistency in function usage throughout your code.
Additionally, Python’s libraries, such as NumPy, offer optimized functions for squaring numbers, especially when dealing with arrays or large datasets. Using `numpy.square(array)` allows for efficient computation across multiple values simultaneously. This is particularly advantageous in scientific computing and data analysis, where performance can be a critical factor.
In summary, Python provides multiple ways to write squared values, including the exponent
Author Profile
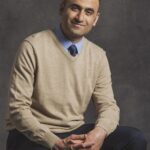
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?