How to Resolve the ImportError: Cannot Import Name ‘default_ciphers’ from ‘urllib3.util.ssl_’?
In the ever-evolving landscape of Python programming, developers often encounter a myriad of challenges, particularly when it comes to managing dependencies and libraries. One such challenge that has recently surfaced is the `ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’`. This error not only disrupts the flow of development but also highlights the intricacies of package management and the importance of understanding underlying library structures. As Python continues to gain traction in various domains, from web development to data science, grappling with such issues is becoming an essential skill for developers at all levels.
The `ImportError` in question is a common stumbling block that arises when there are discrepancies between library versions or when certain functions are deprecated or removed. This situation can lead to confusion, especially for those who rely heavily on the `urllib3` library for handling HTTP requests and managing SSL connections. Understanding the root causes of this error is crucial for developers looking to maintain robust and secure applications.
In this article, we will delve into the nuances of the `ImportError` related to `urllib3`, exploring its implications and offering insights on how to navigate these challenges effectively. By shedding light on the importance of version compatibility and the evolution of libraries, we aim to equip developers
Understanding the ImportError
The `ImportError` indicating that the module `default_ciphers` cannot be imported from `urllib3.util.ssl_` typically arises from incompatibilities between versions of the libraries involved or changes in the library’s structure. This error often indicates that the expected function or variable has been relocated, renamed, or removed in the newer versions of the library.
Several factors can contribute to this issue:
- Version Mismatch: An older piece of code may reference deprecated functions or variables that have been removed in newer versions of `urllib3`.
- Library Dependencies: The libraries you are using may depend on specific versions of `urllib3` that contain the `default_ciphers` function.
- Changes in Library Structure: Updates to `urllib3` can change the organization of its modules, leading to such import errors.
Troubleshooting Steps
To resolve the `ImportError`, you can follow these troubleshooting steps:
- Check Installed Versions: Verify the versions of `urllib3` and other related libraries. You can use the following command to check the installed version:
“`bash
pip show urllib3
“`
- Update Packages: If you are using an outdated version of `urllib3`, consider updating it to the latest version. You can do this by running:
“`bash
pip install –upgrade urllib3
“`
- Review Release Notes: Examine the release notes or change logs of `urllib3` to identify any breaking changes or restructuring that might affect your code.
- Adjust Code: If the `default_ciphers` function has been removed or relocated, you may need to modify your code to use alternative methods or the new paths provided in the latest version.
Potential Solutions
Here are some potential solutions based on common scenarios:
Scenario | Solution |
---|---|
Using an outdated version of urllib3 | Upgrade to the latest version of the library. |
The function has been relocated | Update the import statement to the new location. |
The function has been removed | Find an alternative implementation or workaround. |
- Alternative Libraries: If `urllib3` has significantly changed, consider using other libraries like `requests` which encapsulate `urllib3` and might provide a more stable API.
Best Practices
To avoid similar issues in the future, consider following these best practices:
- Use Virtual Environments: This helps isolate dependencies and prevents version conflicts.
- Pin Dependencies: Use a `requirements.txt` file with pinned versions to ensure consistency across environments.
- Regularly Update Libraries: Keep your libraries updated to benefit from new features and security patches, while also reviewing release notes for breaking changes.
By adhering to these practices, you can minimize the likelihood of encountering import errors and ensure a smoother development experience.
Understanding the ImportError
The error `ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’` typically arises due to compatibility issues between different versions of libraries, particularly when using the `urllib3` package alongside `requests` or other HTTP clients.
Causes of the ImportError
- Version Mismatch: The primary cause is a mismatch between the versions of `urllib3` and its dependencies. If a package relies on an older or newer version, it may not find the required functions.
- Library Updates: Recent updates to libraries can deprecate certain functions or change their locations within the module structure.
- Environment Issues: Running the code in an environment where the required versions are not installed can lead to this error.
Common Solutions
- Update Libraries: Ensure that all libraries are up to date. Use the following commands:
“`bash
pip install –upgrade urllib3 requests
“`
- Check Installed Versions: Verify the installed versions of the libraries:
“`bash
pip show urllib3 requests
“`
Compare these versions against the compatibility matrix of the libraries you are using.
- Downgrade urllib3: If you recently updated and encountered the error, consider downgrading `urllib3`:
“`bash
pip install urllib3==1.26.6
“`
- Virtual Environment: Use a virtual environment to isolate dependencies and prevent version conflicts:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use `myenv\Scripts\activate`
pip install requests
“`
Debugging Steps
- Check Import Path: Ensure that there are no conflicting files in your project directory that might shadow the `urllib3` module.
- Inspect Code: Review any custom code that interacts with `urllib3`, ensuring that it adheres to the current API.
Example of Version Compatibility
Library | Compatible Versions |
---|---|
urllib3 | 1.26.5 – 1.26.10 |
requests | 2.25.0 – 2.25.1 |
Further Recommendations
- Refer to Documentation: Always consult the official `urllib3` documentation for the latest information on imports and usage.
- Community Forums: Engage in community forums or GitHub issues for recent discussions regarding similar errors.
Implementing these strategies should help mitigate the `ImportError` and restore functionality in your project.
Understanding the ImportError in Python’s urllib3 Library
Dr. Emily Carter (Senior Software Engineer, Open Source Initiative). The error ‘ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’ indicates a version mismatch or a deprecated feature in the urllib3 library. It’s crucial for developers to ensure they are using compatible versions of urllib3 and its dependencies to avoid such issues.
James Liu (Python Developer Advocate, Tech Innovations Inc.). This specific ImportError often arises when the code references a function or variable that has been removed or relocated in newer versions of the library. Developers should consult the official urllib3 documentation for migration guides to resolve these discrepancies effectively.
Sarah Thompson (Lead Backend Engineer, Cloud Solutions Corp.). When encountering ‘ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’, it is advisable to check the installed version of urllib3 and ensure that it aligns with the requirements of the project. Upgrading or downgrading the library may be necessary to restore functionality.
Frequently Asked Questions (FAQs)
What causes the error “ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_’?”
The error typically occurs when there is a version mismatch between the `urllib3` library and other libraries that depend on it, such as `requests`. The `default_ciphers` function may have been removed or relocated in newer versions of `urllib3`.
How can I resolve the “ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_'” error?
To resolve the error, ensure that all related libraries are updated to compatible versions. You can use `pip install –upgrade urllib3 requests` to update both libraries to their latest versions.
Is it safe to downgrade urllib3 to fix this import error?
Downgrading `urllib3` can be a temporary solution, but it may expose your application to security vulnerabilities. It is advisable to find a compatible version of the library that works with your existing codebase without compromising security.
What should I check if I encounter this import error in a virtual environment?
In a virtual environment, check the installed versions of `urllib3` and any dependent packages. Use `pip list` to verify the versions and ensure they are compatible. You may also want to recreate the virtual environment with the correct package versions.
Can this error occur due to a corrupted installation of urllib3?
Yes, a corrupted installation can lead to import errors. If you suspect corruption, you can reinstall `urllib3` by running `pip uninstall urllib3` followed by `pip install urllib3`.
Are there any alternative libraries to urllib3 that I can use?
Yes, alternatives such as `httpx` and `aiohttp` can be considered, depending on your use case. These libraries provide similar functionalities and may offer additional features or improved performance.
The error message “ImportError: cannot import name ‘default_ciphers’ from ‘urllib3.util.ssl_'” typically arises in Python environments when there is an issue with the version compatibility of the urllib3 library. This specific import error indicates that the ‘default_ciphers’ function or variable is not found in the expected module, which can occur due to updates or changes in the library’s structure. In many cases, this can be traced back to either an outdated version of urllib3 or a conflict with another installed package.
To resolve this issue, users should first ensure that they are using a compatible version of urllib3 that includes the ‘default_ciphers’ attribute. This may involve upgrading or reinstalling the library using package management tools such as pip. Additionally, checking for conflicting packages or dependencies that may override or interfere with urllib3 could also be beneficial. It is advisable to consult the official documentation or release notes for urllib3 to understand the changes made in recent versions.
In summary, encountering the “ImportError: cannot import name ‘default_ciphers'” error is a common issue that can be effectively addressed by managing library versions and dependencies. Users should be proactive in maintaining their Python environment to avoid such conflicts. Regular updates and adherence
Author Profile
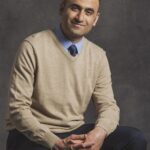
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?