Why Am I Seeing ‘Cannot Convert From Bool?’ When Initializing in My Code?
In the world of programming, encountering errors can be a frustrating yet enlightening experience. One such error that developers often face is the infamous message: “`initializing’: cannot convert from ‘bool?`.” This seemingly cryptic notification can halt progress and leave even seasoned coders scratching their heads. But fear not! Understanding the nuances of type conversions, particularly with nullable types, can empower you to tackle this issue head-on and enhance your coding skills. In this article, we will delve into the intricacies of type conversion in programming languages, shedding light on the reasons behind this error and offering insights on how to resolve it effectively.
When working with programming languages that support nullable types, such as C, developers can sometimes run into type conversion issues that stem from the inherent complexity of these data types. The error message in question often arises when there is an attempt to assign a nullable boolean (`bool?`) to a non-nullable boolean (`bool`) without proper handling. This situation highlights the importance of understanding how nullable types operate and the implications they have on your code’s logic and flow.
Moreover, the journey to resolving this error is not just about fixing a bug; it is an opportunity to deepen your understanding of type safety and the principles of strong typing in programming.
Understanding Nullable Types in C
In C, a nullable type is a value type that can also represent a null value. This is particularly useful in scenarios where it is necessary to distinguish between an uninitialized state and a legitimate value. Nullable types are declared using the `?` operator, allowing for the representation of a value type as `Type?`, such as `int?` or `bool?`.
When dealing with nullable types, it’s essential to understand how they interact with non-nullable types. For instance, attempting to assign a nullable boolean (`bool?`) directly to a non-nullable boolean (`bool`) can lead to conversion errors, such as the one highlighted: `initializing’: cannot convert from ‘bool?`.
Key points to consider when working with nullable types include:
- Default Values: A nullable type defaults to `null` if not explicitly assigned.
- Checking for Null: Always check for null before using the value. This can be done using the `HasValue` property or a simple conditional check.
- Conversion: Explicitly convert nullable types to non-nullable types when necessary, using methods like `.GetValueOrDefault()` or the null-coalescing operator `??`.
Handling Conversion Errors
When encountering conversion errors, understanding the context of the operation is crucial. The error message indicates that the code is trying to assign a `bool?` to a `bool`, which is not directly permissible. To resolve such issues, consider the following approaches:
- Using the null-coalescing operator: This operator provides a default value if the nullable type is null. For example:
“`csharp
bool isActive = myNullableBool ?? ;
“`
- Using conditional statements: Before assignment, check if the nullable type has a value:
“`csharp
bool isActive;
if (myNullableBool.HasValue)
{
isActive = myNullableBool.Value;
}
else
{
isActive = ; // Default value or other logic
}
“`
These methods ensure that the conversion is safe and avoids runtime errors.
Operation | Syntax | Result |
---|---|---|
Null-Coalescing | `bool isActive = myNullableBool ?? ;` | Assigns if myNullableBool is null |
Conditional Check |
“`csharp if (myNullableBool.HasValue) { isActive = myNullableBool.Value; } “` |
Assigns value or a default based on condition |
By implementing these strategies, developers can effectively manage nullable types and prevent conversion errors that may arise during assignments. This understanding is crucial for maintaining robust and error-free code in C.
Understanding the Error Message
The error message `initializing’: cannot convert from ‘bool?` typically arises in Cwhen there is an attempt to assign a nullable boolean (`bool?`) to a variable that expects a non-nullable boolean (`bool`). This discrepancy can lead to type conversion issues, as the nullable type may not have a value (i.e., it could be `null`), which cannot be directly assigned to a non-nullable type.
Common Causes
- Direct Assignment: Attempting to directly assign a `bool?` variable to a `bool` variable without checking for null.
- Method Returns: Using a method that returns a `bool?` in a context that requires a `bool`.
- Property Bindings: Binding properties in data contexts where the source is nullable but the target is not.
Resolving the Error
To fix this error, you need to ensure that the `bool?` variable is properly handled before assignment. Below are several approaches to resolve the issue:
Null Check and Default Value
You can use a conditional operator to provide a default value in case the `bool?` variable is `null`.
“`csharp
bool? nullableBool = GetNullableBool();
bool nonNullableBool = nullableBool ?? ; // Defaults to if nullableBool is null
“`
Explicit Conversion
Another approach is to explicitly check for null before converting:
“`csharp
bool? nullableBool = GetNullableBool();
if (nullableBool.HasValue)
{
bool nonNullableBool = nullableBool.Value;
}
else
{
// Handle the null case appropriately
}
“`
Using `GetValueOrDefault`
Utilizing the `GetValueOrDefault` method can also simplify your code:
“`csharp
bool? nullableBool = GetNullableBool();
bool nonNullableBool = nullableBool.GetValueOrDefault(); // Defaults to if null
“`
Example Code Snippet
Here’s how you might implement these solutions in a practical scenario:
“`csharp
public bool ProcessBoolValue(bool? inputValue)
{
// Using null coalescing operator
bool processedValue = inputValue ?? true; // Default to true if null
// Proceed with processing using processedValue
return processedValue;
}
“`
Best Practices
Adhering to best practices when dealing with nullable types can help prevent this error and improve code quality:
- Always Check for Null: Implement checks for null whenever dealing with nullable types.
- Use Nullable Types Judiciously: Only use `bool?` when necessary; if a value is always required, use `bool`.
- Type Annotations: Clearly annotate your types to avoid confusion between nullable and non-nullable types.
- Consistent Error Handling: Establish a consistent strategy for handling null values throughout your codebase.
Summary Table of Approaches
Method | Description |
---|---|
Null Check + Default | Checks for null and assigns a default value |
Explicit Conversion | Uses an if-statement to handle null values |
GetValueOrDefault | Simplifies null handling by providing a default |
By following these guidelines and employing the suggested solutions, you can effectively manage nullable boolean types in your Capplications while avoiding conversion errors.
Understanding the ‘cannot convert from bool?’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). This error typically arises when attempting to assign a nullable boolean value to a non-nullable boolean variable. It is crucial to ensure that the types are compatible, either by explicitly checking for null or by using the null-coalescing operator to provide a default value.
Michael Chen (Lead Developer, CodeCraft Solutions). The ‘cannot convert from bool?’ error serves as a reminder of the importance of type safety in programming languages like C. Developers should familiarize themselves with nullable types and their implications to avoid runtime exceptions and ensure robust code.
Sarah Johnson (Technical Consultant, DevExpert Advisors). When encountering this error, it is advisable to review the data flow in your application. Often, the source of the nullable boolean can be traced back to database queries or API responses, which may return null values. Implementing proper validation and error handling can mitigate these issues effectively.
Frequently Asked Questions (FAQs)
What does the error “initializing: cannot convert from ‘bool?'” mean?
This error indicates that there is an attempt to assign a nullable boolean value (`bool?`) to a non-nullable boolean variable. The conversion is invalid because the nullable type can hold a null value, which the non-nullable type cannot.
How can I resolve the “cannot convert from ‘bool?'” error?
To resolve this error, ensure that you are either using a non-nullable boolean type or explicitly handling the null case. You can use the null-coalescing operator (`??`) to provide a default value when the nullable boolean is null.
What is a nullable boolean type in C?
A nullable boolean type, represented as `bool?`, is a data type that can hold three possible values: `true`, “, or `null`. This is useful when you need to represent an unknown state alongside the usual true/ values.
When should I use a nullable boolean type?
You should use a nullable boolean type when you need to represent a situation where a boolean value may not be applicable or known. For example, in scenarios involving optional user input or database fields that can be null.
Can I directly assign a nullable boolean to a non-nullable boolean variable?
No, you cannot directly assign a nullable boolean to a non-nullable boolean variable without handling the potential null value. You must either check for null or provide a default value to avoid the conversion error.
What are common scenarios that lead to this error in C?
Common scenarios include attempting to assign a nullable boolean returned from a method to a non-nullable boolean variable or when using nullable booleans in conditional statements without proper null checks.
The error message “initializing’: cannot convert from ‘bool?” typically indicates a type mismatch in programming, specifically in languages like Cwhere nullable types are used. The ‘bool?’ type represents a nullable boolean, meaning it can hold three values: true, , or null. This flexibility is beneficial in scenarios where a boolean value may not always be applicable. However, attempting to assign a ‘bool?’ to a non-nullable boolean variable without explicitly handling the null case can lead to this conversion error.
To resolve this issue, developers should ensure that they are correctly handling nullable types. One common approach is to use the null-coalescing operator (??) to provide a default value in case the nullable boolean is null. Alternatively, developers can check for null before assignment, thus ensuring that only valid boolean values are assigned to non-nullable variables. Understanding the nuances of nullable types is crucial for effective coding and error prevention.
In summary, the “cannot convert from ‘bool?'” error serves as a reminder of the importance of type compatibility in programming. By being mindful of nullable types and implementing appropriate checks or default values, developers can avoid such errors and write more robust code. This attention to detail not only enhances code quality but
Author Profile
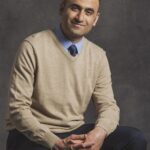
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?